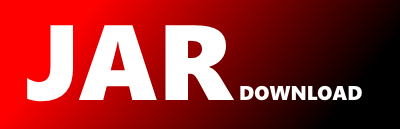
org.apache.james.imap.message.response.FetchResponse Maven / Gradle / Ivy
/****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one *
* or more contributor license agreements. See the NOTICE file *
* distributed with this work for additional information *
* regarding copyright ownership. The ASF licenses this file *
* to you under the Apache License, Version 2.0 (the *
* "License"); you may not use this file except in compliance *
* with the License. You may obtain a copy of the License at *
* *
* http://www.apache.org/licenses/LICENSE-2.0 *
* *
* Unless required by applicable law or agreed to in writing, *
* software distributed under the License is distributed on an *
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY *
* KIND, either express or implied. See the License for the *
* specific language governing permissions and limitations *
* under the License. *
****************************************************************/
package org.apache.james.imap.message.response;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.mail.Flags;
import org.apache.james.imap.api.message.response.ImapResponseMessage;
import org.apache.james.imap.message.Literal;
import org.apache.james.mailbox.MessageSequenceNumber;
import org.apache.james.mailbox.MessageUid;
import org.apache.james.mailbox.ModSeq;
import org.apache.james.mailbox.model.MessageId;
import org.apache.james.mailbox.model.ThreadId;
public final class FetchResponse implements ImapResponseMessage {
private final MessageSequenceNumber messageNumber;
private final Flags flags;
private final MessageUid uid;
private final Date internalDate;
private final Optional saveDate;
private final Long size;
private final List elements;
private final Envelope envelope;
private final Structure body;
private final Structure bodystructure;
private final ModSeq modSeq;
private final MessageId emailId;
private final ThreadId threadId;
public FetchResponse(MessageSequenceNumber messageNumber, Flags flags, MessageUid uid, Optional saveDate, ModSeq modSeq, Date internalDate, Long size, Envelope envelope, Structure body, Structure bodystructure, List elements, MessageId emailId, ThreadId threadId) {
this.messageNumber = messageNumber;
this.flags = flags;
this.uid = uid;
this.internalDate = internalDate;
this.saveDate = saveDate;
this.size = size;
this.envelope = envelope;
this.elements = elements;
this.body = body;
this.bodystructure = bodystructure;
this.modSeq = modSeq;
this.emailId = emailId;
this.threadId = threadId;
}
/**
* Gets the structure of this message.
*
* @return Structure
, or null if the FETCH
did not
* include BODY
*/
public Structure getBody() {
return body;
}
/**
* Gets the structure of this message.
*
* @return Structure
, or null if the FETCH
did not
* include BODYSTRUCTURE
*/
public Structure getBodyStructure() {
return bodystructure;
}
/**
* Gets the number of the message whose details have been fetched.
*
* @return message number
*/
public MessageSequenceNumber getMessageNumber() {
return messageNumber;
}
/**
* Gets the fetched flags.
*
* @return {@link Flags} fetched, or null if the FETCH
did not
* include FLAGS
*/
public Flags getFlags() {
return flags;
}
/**
* Gets the unique id for the fetched message.
*
* @return message uid, or null if the FETCH
did not include
* UID
*/
public MessageUid getUid() {
return uid;
}
/**
* Gets the internal date for the fetched message.
*
* @return the internalDate, or null if the FETCH
did not
* include INTERNALDATE
*/
public Date getInternalDate() {
return internalDate;
}
/**
* Gets the size for the fetched message.
*
* @return the size, or null if the FETCH
did not include
* SIZE
*/
public Long getSize() {
return size;
}
/**
* Gets the envelope for the fetched message
*
* @return the envelope, or null if the FETCH
did not include
* ENVELOPE
*/
public Envelope getEnvelope() {
return envelope;
}
/**
* TODO: replace
*
* @return List
of BodyElement
's, or null if the
* FETCH
did not include body elements
*/
public List getElements() {
return elements;
}
/**
* Return the mod-sequence for the message or null if the FETCH
did not
* include it
*
* @return modSeq
*/
public ModSeq getModSeq() {
return modSeq;
}
public MessageId getEmailId() {
return emailId;
}
public ThreadId getThreadId() {
return threadId;
}
public Optional getSaveDate() {
return saveDate;
}
/**
* Describes the message structure.
*/
public interface Structure {
/**
* Gets the MIME media type.
*
* @return media type, or null if default
*/
String getMediaType();
/**
* Gets the MIME content subtype
*
* @return subtype of null if default
*/
String getSubType();
/**
* Gets body type parameters.
*
* @return parameters, or null
*/
List getParameters();
/**
* Gets Content-ID
.
*
* @return MIME content ID, possibly null
*/
String getId();
/**
* Gets Content-Description
.
*
* @return MIME Content-Description
, possibly null
*/
String getDescription();
/**
* Gets content transfer encoding.
*
* @return MIME Content-Transfer-Encoding
, possibly null
*/
String getEncoding();
/**
* Gets the size of message body the in octets.
*
* @return number of octets in the message.
*/
long getOctets();
/**
* Gets the number of lines fo transfer encoding for a TEXT
* type.
*
* @return number of lines when TEXT
, -1 otherwise
*/
long getLines();
/**
* Gets Content-MD5
.
*
* @return Content-MD5 or null if BODY
FETCH or not present
*/
String getMD5();
/**
* Gets header field-value from Content-Disposition
.
*
* @return map of field value String
indexed by field name
* String
or null if BODY
FETCH or not
* present
*/
Map getDispositionParams();
/**
* Gets header field-value from Content-Disposition
.
*
* @return disposition or null if BODY
FETCH or not present
*/
String getDisposition();
/**
* Gets MIME Content-Language
's.
*
* @return List of Content-Language
name
* String
's possibly null or null when
* BODY
FETCH
*/
List getLanguages();
/**
* Gets Content-Location
.
*
* @return Content-Location possibly null; or null when
* BODY
FETCH
*/
String getLocation();
/**
* Iterates parts of a composite media type.
*
* @return Structure
Iterator
when composite
* type, null otherwise
*/
Iterator parts();
/**
* Gets the envelope of an embedded mail.
*
* @return Envelope
when message/rfc822
* otherwise null
*/
Envelope getEnvelope();
/**
* Gets the envelope of an embedded mail.
*
* @return Structure
when when message/rfc822
* otherwise null
*/
Structure getBody();
}
/**
* BODY FETCH element content.
*/
public interface BodyElement extends Literal {
/**
* The full name of the element fetched. As per FETCH
* command input.
*
* @return name, not null
*/
String getName();
}
/**
* ENVELOPE content.
*/
public interface Envelope {
/**
* Gets the envelope date
. This is the value of the RFC822
* date
header.
*
* @return envelope Date or null if this attribute is NIL
*/
String getDate();
/**
* Gets the envelope subject
. This is the value of the
* RFC822 subject
header.
*
* @return subject, or null if this attribute is NIL
*/
String getSubject();
/**
* Gets the envelope from
addresses.
*
* @return from addresses, not null
*/
Address[] getFrom();
/**
* Gets the envelope sender
addresses.
*
* @return sender
addresses, not null
*/
Address[] getSender();
/**
* Gets the envelope reply-to
addresses.
*
* @return reply-to
, not null
*/
Address[] getReplyTo();
/**
* Gets the envelope to
addresses.
*
* @return to
, or null if NIL
*/
Address[] getTo();
/**
* Gets the envelope cc
addresses.
*
* @return cc
, or null if NIL
*/
Address[] getCc();
/**
* Gets the envelope bcc
addresses.
*
* @return bcc
, or null if NIL
*/
Address[] getBcc();
/**
* Gets the envelope in-reply-to
.
*
* @return in-reply-to
or null if NIL
*/
String getInReplyTo();
/**
* Gets the envelope message
*
* @return the message id
*/
String getMessageId();
/**
* Values an envelope address.
*/
interface Address {
/**
* Gets the personal name.
*
* @return personal name, or null if the personal name is
* NIL
*/
String getPersonalName();
/**
* Gets the SMTP source route.
*
* @return SMTP at-domain-list, or null if the list if
* NIL
*/
String getAtDomainList();
/**
* Gets the mailbox name.
*
* @return the mailbox name or the group name when
* {@link #getHostName()} is null
*/
String getMailboxName();
/**
* Gets the host name.
*
* @return the host name, or null when this address marks the start
* or end of a group
*/
String getHostName();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy