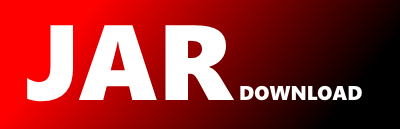
org.apache.james.mailbox.model.Quota Maven / Gradle / Ivy
/****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one *
* or more contributor license agreements. See the NOTICE file *
* distributed with this work for additional information *
* regarding copyright ownership. The ASF licenses this file *
* to you under the Apache License, Version 2.0 (the *
* "License"); you may not use this file except in compliance *
* with the License. You may obtain a copy of the License at *
* *
* http://www.apache.org/licenses/LICENSE-2.0 *
* *
* Unless required by applicable law or agreed to in writing, *
* software distributed under the License is distributed on an *
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY *
* KIND, either express or implied. See the License for the *
* specific language governing permissions and limitations *
* under the License. *
****************************************************************/
package org.apache.james.mailbox.model;
import java.util.Map;
import org.apache.james.core.quota.QuotaValue;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableMap;
public class Quota> {
public enum Scope {
Domain,
Global,
User
}
public static > Builder builder() {
return new Builder<>();
}
public static class Builder> {
private final ImmutableMap.Builder limitsByScope;
private T computedLimit;
private T used;
private Builder() {
limitsByScope = ImmutableMap.builder();
}
public Builder computedLimit(T limit) {
this.computedLimit = limit;
return this;
}
public Builder used(T used) {
this.used = used;
return this;
}
public Builder limitsByScope(Map limits) {
limitsByScope.putAll(limits);
return this;
}
public Builder limitForScope(T limit, Scope scope) {
limitsByScope.put(scope, limit);
return this;
}
public Quota build() {
Preconditions.checkState(used != null);
Preconditions.checkState(computedLimit != null);
return new Quota<>(used, computedLimit, limitsByScope.build());
}
}
private final T limit;
private final ImmutableMap limitByScope;
private final T used;
private Quota(T used, T max, ImmutableMap limitByScope) {
this.used = used;
this.limit = max;
this.limitByScope = limitByScope;
}
public T getLimit() {
return limit;
}
public T getUsed() {
return used;
}
public double getRatio() {
if (limit.isUnlimited()) {
return 0;
}
return Double.valueOf(used.asLong()) / Double.valueOf(limit.asLong());
}
public ImmutableMap getLimitByScope() {
return limitByScope;
}
public Quota addValueToQuota(T value) {
return new Quota<>(used.add(value), limit, limitByScope);
}
/**
* Tells us if the quota is reached
*
* @return True if the user over uses the resource of this quota
*/
public boolean isOverQuota() {
return isOverQuotaWithAdditionalValue(0);
}
public boolean isOverQuotaWithAdditionalValue(long additionalValue) {
Preconditions.checkArgument(additionalValue >= 0);
return limit.isLimited() && used.add(additionalValue).isGreaterThan(limit);
}
@Override
public String toString() {
return used + "/" + limit;
}
@Override
public boolean equals(Object o) {
if (o == null || ! (o instanceof Quota)) {
return false;
}
Quota> other = (Quota>) o;
return Objects.equal(used, other.getUsed())
&& Objects.equal(limit,other.getLimit());
}
@Override
public int hashCode() {
return Objects.hashCode(used, limit);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy