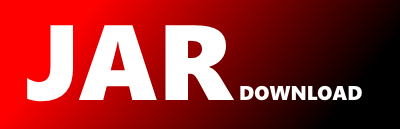
org.jclouds.packet.domain.AutoValue_Device_CreateDevice Maven / Gradle / Ivy
package org.jclouds.packet.domain;
import java.util.Map;
import java.util.Set;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Device_CreateDevice extends Device.CreateDevice {
private final String hostname;
private final String plan;
private final String billingCycle;
private final String facility;
private final Map features;
private final String operatingSystem;
private final Boolean locked;
private final String userdata;
private final Set tags;
private AutoValue_Device_CreateDevice(
String hostname,
String plan,
String billingCycle,
String facility,
Map features,
String operatingSystem,
Boolean locked,
String userdata,
Set tags) {
this.hostname = hostname;
this.plan = plan;
this.billingCycle = billingCycle;
this.facility = facility;
this.features = features;
this.operatingSystem = operatingSystem;
this.locked = locked;
this.userdata = userdata;
this.tags = tags;
}
@Override
public String hostname() {
return hostname;
}
@Override
public String plan() {
return plan;
}
@Override
public String billingCycle() {
return billingCycle;
}
@Override
public String facility() {
return facility;
}
@Override
public Map features() {
return features;
}
@Override
public String operatingSystem() {
return operatingSystem;
}
@Override
public Boolean locked() {
return locked;
}
@Override
public String userdata() {
return userdata;
}
@Override
public Set tags() {
return tags;
}
@Override
public String toString() {
return "CreateDevice{"
+ "hostname=" + hostname + ", "
+ "plan=" + plan + ", "
+ "billingCycle=" + billingCycle + ", "
+ "facility=" + facility + ", "
+ "features=" + features + ", "
+ "operatingSystem=" + operatingSystem + ", "
+ "locked=" + locked + ", "
+ "userdata=" + userdata + ", "
+ "tags=" + tags
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Device.CreateDevice) {
Device.CreateDevice that = (Device.CreateDevice) o;
return (this.hostname.equals(that.hostname()))
&& (this.plan.equals(that.plan()))
&& (this.billingCycle.equals(that.billingCycle()))
&& (this.facility.equals(that.facility()))
&& (this.features.equals(that.features()))
&& (this.operatingSystem.equals(that.operatingSystem()))
&& (this.locked.equals(that.locked()))
&& (this.userdata.equals(that.userdata()))
&& (this.tags.equals(that.tags()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= hostname.hashCode();
h$ *= 1000003;
h$ ^= plan.hashCode();
h$ *= 1000003;
h$ ^= billingCycle.hashCode();
h$ *= 1000003;
h$ ^= facility.hashCode();
h$ *= 1000003;
h$ ^= features.hashCode();
h$ *= 1000003;
h$ ^= operatingSystem.hashCode();
h$ *= 1000003;
h$ ^= locked.hashCode();
h$ *= 1000003;
h$ ^= userdata.hashCode();
h$ *= 1000003;
h$ ^= tags.hashCode();
return h$;
}
static final class Builder extends Device.CreateDevice.Builder {
private String hostname;
private String plan;
private String billingCycle;
private String facility;
private Map features;
private String operatingSystem;
private Boolean locked;
private String userdata;
private Set tags;
Builder() {
}
@Override
public Device.CreateDevice.Builder hostname(String hostname) {
if (hostname == null) {
throw new NullPointerException("Null hostname");
}
this.hostname = hostname;
return this;
}
@Override
public Device.CreateDevice.Builder plan(String plan) {
if (plan == null) {
throw new NullPointerException("Null plan");
}
this.plan = plan;
return this;
}
@Override
public Device.CreateDevice.Builder billingCycle(String billingCycle) {
if (billingCycle == null) {
throw new NullPointerException("Null billingCycle");
}
this.billingCycle = billingCycle;
return this;
}
@Override
public Device.CreateDevice.Builder facility(String facility) {
if (facility == null) {
throw new NullPointerException("Null facility");
}
this.facility = facility;
return this;
}
@Override
public Device.CreateDevice.Builder features(Map features) {
if (features == null) {
throw new NullPointerException("Null features");
}
this.features = features;
return this;
}
@Override
Map features() {
if (features == null) {
throw new IllegalStateException("Property \"features\" has not been set");
}
return features;
}
@Override
public Device.CreateDevice.Builder operatingSystem(String operatingSystem) {
if (operatingSystem == null) {
throw new NullPointerException("Null operatingSystem");
}
this.operatingSystem = operatingSystem;
return this;
}
@Override
public Device.CreateDevice.Builder locked(Boolean locked) {
if (locked == null) {
throw new NullPointerException("Null locked");
}
this.locked = locked;
return this;
}
@Override
public Device.CreateDevice.Builder userdata(String userdata) {
if (userdata == null) {
throw new NullPointerException("Null userdata");
}
this.userdata = userdata;
return this;
}
@Override
public Device.CreateDevice.Builder tags(Set tags) {
if (tags == null) {
throw new NullPointerException("Null tags");
}
this.tags = tags;
return this;
}
@Override
Set tags() {
if (tags == null) {
throw new IllegalStateException("Property \"tags\" has not been set");
}
return tags;
}
@Override
Device.CreateDevice autoBuild() {
String missing = "";
if (this.hostname == null) {
missing += " hostname";
}
if (this.plan == null) {
missing += " plan";
}
if (this.billingCycle == null) {
missing += " billingCycle";
}
if (this.facility == null) {
missing += " facility";
}
if (this.features == null) {
missing += " features";
}
if (this.operatingSystem == null) {
missing += " operatingSystem";
}
if (this.locked == null) {
missing += " locked";
}
if (this.userdata == null) {
missing += " userdata";
}
if (this.tags == null) {
missing += " tags";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_Device_CreateDevice(
this.hostname,
this.plan,
this.billingCycle,
this.facility,
this.features,
this.operatingSystem,
this.locked,
this.userdata,
this.tags);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy