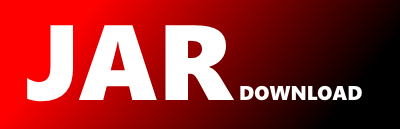
org.jclouds.profitbricks.domain.AutoValue_Storage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of profitbricks Show documentation
Show all versions of profitbricks Show documentation
jclouds components to access an implementation of ProfitBricks
package org.jclouds.profitbricks.domain;
import java.util.Date;
import java.util.List;
import javax.annotation.processing.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Storage extends Storage {
private final String id;
private final String name;
private final float size;
private final Date creationTime;
private final Date lastModificationTime;
private final ProvisioningState state;
private final List serverIds;
private final Boolean bootDevice;
private final Storage.BusType busType;
private final Integer deviceNumber;
private AutoValue_Storage(
String id,
@Nullable String name,
float size,
@Nullable Date creationTime,
@Nullable Date lastModificationTime,
@Nullable ProvisioningState state,
@Nullable List serverIds,
@Nullable Boolean bootDevice,
@Nullable Storage.BusType busType,
@Nullable Integer deviceNumber) {
this.id = id;
this.name = name;
this.size = size;
this.creationTime = creationTime;
this.lastModificationTime = lastModificationTime;
this.state = state;
this.serverIds = serverIds;
this.bootDevice = bootDevice;
this.busType = busType;
this.deviceNumber = deviceNumber;
}
@Override
public String id() {
return id;
}
@Nullable
@Override
public String name() {
return name;
}
@Override
public float size() {
return size;
}
@Nullable
@Override
public Date creationTime() {
return creationTime;
}
@Nullable
@Override
public Date lastModificationTime() {
return lastModificationTime;
}
@Nullable
@Override
public ProvisioningState state() {
return state;
}
@Nullable
@Override
public List serverIds() {
return serverIds;
}
@Nullable
@Override
public Boolean bootDevice() {
return bootDevice;
}
@Nullable
@Override
public Storage.BusType busType() {
return busType;
}
@Nullable
@Override
public Integer deviceNumber() {
return deviceNumber;
}
@Override
public String toString() {
return "Storage{"
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "size=" + size + ", "
+ "creationTime=" + creationTime + ", "
+ "lastModificationTime=" + lastModificationTime + ", "
+ "state=" + state + ", "
+ "serverIds=" + serverIds + ", "
+ "bootDevice=" + bootDevice + ", "
+ "busType=" + busType + ", "
+ "deviceNumber=" + deviceNumber
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Storage) {
Storage that = (Storage) o;
return (this.id.equals(that.id()))
&& ((this.name == null) ? (that.name() == null) : this.name.equals(that.name()))
&& (Float.floatToIntBits(this.size) == Float.floatToIntBits(that.size()))
&& ((this.creationTime == null) ? (that.creationTime() == null) : this.creationTime.equals(that.creationTime()))
&& ((this.lastModificationTime == null) ? (that.lastModificationTime() == null) : this.lastModificationTime.equals(that.lastModificationTime()))
&& ((this.state == null) ? (that.state() == null) : this.state.equals(that.state()))
&& ((this.serverIds == null) ? (that.serverIds() == null) : this.serverIds.equals(that.serverIds()))
&& ((this.bootDevice == null) ? (that.bootDevice() == null) : this.bootDevice.equals(that.bootDevice()))
&& ((this.busType == null) ? (that.busType() == null) : this.busType.equals(that.busType()))
&& ((this.deviceNumber == null) ? (that.deviceNumber() == null) : this.deviceNumber.equals(that.deviceNumber()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= (name == null) ? 0 : name.hashCode();
h$ *= 1000003;
h$ ^= Float.floatToIntBits(size);
h$ *= 1000003;
h$ ^= (creationTime == null) ? 0 : creationTime.hashCode();
h$ *= 1000003;
h$ ^= (lastModificationTime == null) ? 0 : lastModificationTime.hashCode();
h$ *= 1000003;
h$ ^= (state == null) ? 0 : state.hashCode();
h$ *= 1000003;
h$ ^= (serverIds == null) ? 0 : serverIds.hashCode();
h$ *= 1000003;
h$ ^= (bootDevice == null) ? 0 : bootDevice.hashCode();
h$ *= 1000003;
h$ ^= (busType == null) ? 0 : busType.hashCode();
h$ *= 1000003;
h$ ^= (deviceNumber == null) ? 0 : deviceNumber.hashCode();
return h$;
}
@Override
public Storage.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends Storage.Builder {
private String id;
private String name;
private Float size;
private Date creationTime;
private Date lastModificationTime;
private ProvisioningState state;
private List serverIds;
private Boolean bootDevice;
private Storage.BusType busType;
private Integer deviceNumber;
Builder() {
}
private Builder(Storage source) {
this.id = source.id();
this.name = source.name();
this.size = source.size();
this.creationTime = source.creationTime();
this.lastModificationTime = source.lastModificationTime();
this.state = source.state();
this.serverIds = source.serverIds();
this.bootDevice = source.bootDevice();
this.busType = source.busType();
this.deviceNumber = source.deviceNumber();
}
@Override
public Storage.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public Storage.Builder name(@Nullable String name) {
this.name = name;
return this;
}
@Override
public Storage.Builder size(float size) {
this.size = size;
return this;
}
@Override
public Storage.Builder creationTime(@Nullable Date creationTime) {
this.creationTime = creationTime;
return this;
}
@Override
public Storage.Builder lastModificationTime(@Nullable Date lastModificationTime) {
this.lastModificationTime = lastModificationTime;
return this;
}
@Override
public Storage.Builder state(@Nullable ProvisioningState state) {
this.state = state;
return this;
}
@Override
public Storage.Builder serverIds(@Nullable List serverIds) {
this.serverIds = serverIds;
return this;
}
@Override
public Storage.Builder bootDevice(@Nullable Boolean bootDevice) {
this.bootDevice = bootDevice;
return this;
}
@Override
public Storage.Builder busType(@Nullable Storage.BusType busType) {
this.busType = busType;
return this;
}
@Override
public Storage.Builder deviceNumber(@Nullable Integer deviceNumber) {
this.deviceNumber = deviceNumber;
return this;
}
@Override
Storage autoBuild() {
String missing = "";
if (this.id == null) {
missing += " id";
}
if (this.size == null) {
missing += " size";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_Storage(
this.id,
this.name,
this.size,
this.creationTime,
this.lastModificationTime,
this.state,
this.serverIds,
this.bootDevice,
this.busType,
this.deviceNumber);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy