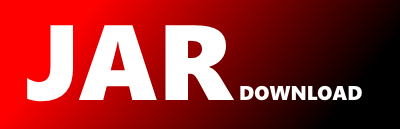
org.apache.jena.fuseki.main.FusekiLib Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.jena.fuseki.main;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.apache.jena.fuseki.access.AccessCtl_AllowGET;
import org.apache.jena.fuseki.access.AccessCtl_Deny;
import org.apache.jena.fuseki.access.AccessCtl_GSP_R;
import org.apache.jena.fuseki.access.AccessCtl_SPARQL_QueryDataset;
import org.apache.jena.fuseki.server.DataAccessPointRegistry;
import org.apache.jena.fuseki.server.Endpoint;
import org.apache.jena.fuseki.server.Operation;
import org.apache.jena.fuseki.servlets.ActionService;
import org.apache.jena.fuseki.servlets.GSP_RW;
import org.apache.jena.fuseki.servlets.HttpAction;
import org.apache.jena.riot.WebContent;
/** Actions on and about a {@link FusekiServer} */
public class FusekiLib {
/**
* Return a collection of the names registered. This collection does not change as the
* server changes.
*/
public static Collection names(FusekiServer server) {
DataAccessPointRegistry dataAccessPoints = DataAccessPointRegistry.get(server.getServletContext());
int N = dataAccessPoints.size();
Stream stream = DataAccessPointRegistry.get(server.getServletContext()).keys().stream();
// Correct size, no reallocate.
List names = stream.collect(Collectors.toCollection(() -> new ArrayList<>(N)));
return names;
}
/**
* Return a {@code FusekiServer.Builder} setup for data access control.
*/
public static FusekiServer.Builder fusekiBuilderAccessCtl(Function determineUser) {
FusekiServer.Builder builder = FusekiServer.create();
return fusekiBuilderAccessCtl(builder, determineUser);
}
/**
* Modify a {@code FusekiServer.Builder} setup for data access control.
*/
public static FusekiServer.Builder fusekiBuilderAccessCtl(FusekiServer.Builder builder, Function determineUser) {
// Replace the standard operations with the SecurityRegistry processing ones.
builder.registerOperation(Operation.Query, WebContent.contentTypeSPARQLQuery, new AccessCtl_SPARQL_QueryDataset(determineUser));
builder.registerOperation(Operation.GSP_R, new AccessCtl_GSP_R(determineUser));
// Block updates (can just not register these operations).
builder.registerOperation(Operation.Update, WebContent.contentTypeSPARQLUpdate, new AccessCtl_Deny("Update"));
builder.registerOperation(Operation.GSP_RW, new AccessCtl_AllowGET(new GSP_RW(), "GSP Write"));
builder.registerOperation(Operation.GSP_RW, new AccessCtl_GSP_R(determineUser));
return builder;
}
/**
* Modify in-place existing {@link Endpoint Endpoints} so that the read-operations for
* query/GSP/Quads go to the data-filtering versions of the {@link ActionService ActionServices}.
*/
public static void modifyForAccessCtl(DataAccessPointRegistry dapRegistry, Function determineUser) {
dapRegistry.forEach((name, dap) -> {
dap.getDataService().forEachEndpoint(ep->{
Operation op = ep.getOperation();
modifyForAccessCtl(ep, determineUser);
});
});
}
/**
* Modify in-place existing an {@link Endpoint} so that the read-operations for
* query/GSP/Quads go to the data-filtering versions of the {@link ActionService ActionServices}.
* Any other operations are replaced with "access denied".
*/
public static void modifyForAccessCtl(Endpoint endpoint, Function determineUser) {
endpoint.setProcessor( controlledProc(endpoint.getOperation(), determineUser) );
}
private static ActionService controlledProc(Operation op, Function determineUser) {
if ( Operation.Query.equals(op) )
return new AccessCtl_SPARQL_QueryDataset(determineUser);
if ( Operation.GSP_R.equals(op) )
return new AccessCtl_GSP_R(determineUser);
if ( Operation.GSP_RW.equals(op) )
return new AccessCtl_GSP_R(determineUser);
return new AccessCtl_Deny("Not supported for graph level access control: "+op.getDescription());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy