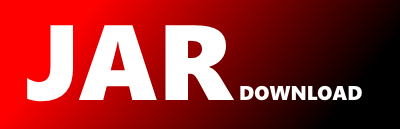
org.apache.juddi.v3.client.config.ClientConfig Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2001-2009 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.juddi.v3.client.config;
import org.apache.commons.configuration.CompositeConfiguration;
import org.apache.commons.configuration.Configuration;
import org.apache.commons.configuration.ConfigurationException;
import org.apache.commons.configuration.PropertiesConfiguration;
import org.apache.commons.configuration.SystemConfiguration;
import org.apache.commons.configuration.reloading.FileChangedReloadingStrategy;
import org.apache.log4j.Logger;
/**
* Handles the client configuration of the uddi-client. By default it first
* looks at system properties.
*
* @author Kurt T Stam
*/
public class ClientConfig
{
private final static String UDDI_PROPERTIES = "uddi.properties";
private Logger log = Logger.getLogger(ClientConfig.class);
private Configuration config;
private static ClientConfig instance=null;
/**
* Constructor (note Singleton pattern).
* @throws ConfigurationException
*/
private ClientConfig() throws ConfigurationException
{
loadConfiguration();
}
/**
* Does the actual work of reading the configuration from System
* Properties and/or juddiv3.properties file. When the juddiv3.properties
* file is updated the file will be reloaded. By default the reloadDelay is
* set to 1 second to prevent excessive date stamp checking.
*/
private void loadConfiguration() throws ConfigurationException
{
//Properties from system properties
CompositeConfiguration compositeConfig = new CompositeConfiguration();
compositeConfig.addConfiguration(new SystemConfiguration());
//Properties from file
PropertiesConfiguration propConfig = new PropertiesConfiguration(UDDI_PROPERTIES);
long refreshDelay = propConfig.getLong(Property.UDDI_CONFIGURATION_RELOAD_DELAY, 1000l);
log.debug("Setting refreshDelay to " + refreshDelay);
FileChangedReloadingStrategy fileChangedReloadingStrategy = new FileChangedReloadingStrategy();
fileChangedReloadingStrategy.setRefreshDelay(refreshDelay);
propConfig.setReloadingStrategy(fileChangedReloadingStrategy);
compositeConfig.addConfiguration(propConfig);
//Making the new configuration globally accessible.
config = compositeConfig;
}
/**
* Obtains the reference to the Singleton instance.
*
* @return the APplicationConfuration Singleton Instance.
* @throws ConfigurationException
*/
private static ClientConfig getInstance() throws ConfigurationException
{
if (instance==null) {
instance = new ClientConfig();
}
return instance;
}
/**
* Hook to receive configuration reload events from an external application.
*
* @throws ConfigurationException
*/
public static void reloadConfig() throws ConfigurationException
{
getInstance().loadConfiguration();
}
/**
* The object from which property values can be obtained.
* @return the commons Configuration interface
* @throws ConfigurationException
*/
public static Configuration getConfiguration() throws ConfigurationException
{
return getInstance().config;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy