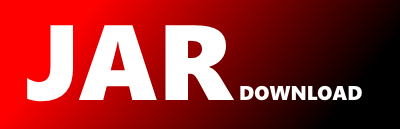
org.apache.juneau.AnnotationProvider Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau;
import static org.apache.juneau.internal.ConsumerUtils.*;
import java.lang.annotation.*;
import java.lang.reflect.*;
import java.util.function.*;
import org.apache.juneau.internal.*;
/**
* Interface that provides the ability to look up annotations on classes/methods/constructors/fields.
*
* See Also:
*
*/
public interface AnnotationProvider {
/**
* Disable annotation caching.
*/
boolean DISABLE_ANNOTATION_CACHING = Boolean.getBoolean("juneau.disableAnnotationCaching");
/**
* Default metadata provider.
*/
@SuppressWarnings("unchecked") AnnotationProvider DEFAULT = new AnnotationProvider() {
private final TwoKeyConcurrentCache,Class extends Annotation>,Annotation[]> classAnnotationCache = new TwoKeyConcurrentCache<>(DISABLE_ANNOTATION_CACHING, Class::getAnnotationsByType);
private final TwoKeyConcurrentCache,Class extends Annotation>,Annotation[]> declaredClassAnnotationCache = new TwoKeyConcurrentCache<>(DISABLE_ANNOTATION_CACHING, Class::getDeclaredAnnotationsByType);
private final TwoKeyConcurrentCache,Annotation[]> methodAnnotationCache = new TwoKeyConcurrentCache<>(DISABLE_ANNOTATION_CACHING, Method::getAnnotationsByType);
private final TwoKeyConcurrentCache,Annotation[]> fieldAnnotationCache = new TwoKeyConcurrentCache<>(DISABLE_ANNOTATION_CACHING, Field::getAnnotationsByType);
private final TwoKeyConcurrentCache,Class extends Annotation>,Annotation[]> constructorAnnotationCache = new TwoKeyConcurrentCache<>(DISABLE_ANNOTATION_CACHING, Constructor::getAnnotationsByType);
@Override /* MetaProvider */
public void forEachAnnotation(Class type, Class> onClass, Predicate filter, Consumer action) {
if (type != null && onClass != null)
for (A a : annotations(type, onClass))
consume(filter, action, a);
}
@Override /* MetaProvider */
public A firstAnnotation(Class type, Class> onClass, Predicate filter) {
if (type != null && onClass != null)
for (A a : annotations(type, onClass))
if (test(filter, a))
return a;
return null;
}
@Override /* MetaProvider */
public A lastAnnotation(Class type, Class> onClass, Predicate filter) {
A x = null;
if (type != null && onClass != null)
for (A a : annotations(type, onClass))
if (test(filter, a))
x = a;
return x;
}
@Override /* MetaProvider */
public void forEachDeclaredAnnotation(Class type, Class> onClass, Predicate filter, Consumer action) {
if (type != null && onClass != null)
for (A a : declaredAnnotations(type, onClass))
consume(filter, action, a);
}
@Override /* MetaProvider */
public A firstDeclaredAnnotation(Class type, Class> onClass, Predicate filter) {
if (type != null && onClass != null)
for (A a : declaredAnnotations(type, onClass))
if (test(filter, a))
return a;
return null;
}
@Override /* MetaProvider */
public A lastDeclaredAnnotation(Class type, Class> onClass, Predicate filter) {
A x = null;
if (type != null && onClass != null)
for (A a : declaredAnnotations(type, onClass))
if (test(filter, a))
x = a;
return x;
}
@Override /* MetaProvider */
public void forEachAnnotation(Class type, Method onMethod, Predicate filter, Consumer action) {
if (type != null && onMethod != null)
for (A a : annotations(type, onMethod))
consume(filter, action, a);
}
@Override /* MetaProvider */
public A firstAnnotation(Class type, Method onMethod, Predicate filter) {
if (type != null && onMethod != null)
for (A a : annotations(type, onMethod))
if (test(filter, a))
return a;
return null;
}
@Override /* MetaProvider */
public A lastAnnotation(Class type, Method onMethod, Predicate filter) {
A x = null;
if (type != null && onMethod != null)
for (A a : annotations(type, onMethod))
if (test(filter, a))
x = a;
return x;
}
@Override /* MetaProvider */
public void forEachAnnotation(Class type, Field onField, Predicate filter, Consumer action) {
if (type != null && onField != null)
for (A a : annotations(type, onField))
consume(filter, action, a);
}
@Override /* MetaProvider */
public A firstAnnotation(Class type, Field onField, Predicate filter) {
if (type != null && onField != null)
for (A a : annotations(type, onField))
if (test(filter, a))
return a;
return null;
}
@Override /* MetaProvider */
public A lastAnnotation(Class type, Field onField, Predicate filter) {
A x = null;
if (type != null && onField != null)
for (A a : annotations(type, onField))
if (test(filter, a))
x = a;
return x;
}
@Override /* MetaProvider */
public void forEachAnnotation(Class type, Constructor> onConstructor, Predicate filter, Consumer action) {
if (type != null && onConstructor != null)
for (A a : annotations(type, onConstructor))
consume(filter, action, a);
}
@Override /* MetaProvider */
public A firstAnnotation(Class type, Constructor> onConstructor, Predicate filter) {
if (type != null && onConstructor != null)
for (A a : annotations(type, onConstructor))
if (test(filter, a))
return a;
return null;
}
@Override /* MetaProvider */
public A lastAnnotation(Class type, Constructor> onConstructor, Predicate filter) {
A x = null;
if (type != null && onConstructor != null)
for (A a : annotations(type, onConstructor))
if (test(filter, a))
x = a;
return x;
}
private A[] annotations(Class type, Class> onClass) {
return (A[])classAnnotationCache.get(onClass, type);
}
private A[] declaredAnnotations(Class type, Class> onClass) {
return (A[])declaredClassAnnotationCache.get(onClass, type);
}
private A[] annotations(Class type, Method onMethod) {
return (A[])methodAnnotationCache.get(onMethod, type);
}
private A[] annotations(Class type, Field onField) {
return (A[])fieldAnnotationCache.get(onField, type);
}
private A[] annotations(Class type, Constructor> onConstructor) {
return (A[])constructorAnnotationCache.get(onConstructor, type);
}
};
/**
* Performs an action on the matching annotations on the specified class.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onClass The class to search on.
* @param filter A predicate to apply to the entries to determine if action should be performed. Can be null .
* @param action An action to perform on the entry.
*/
void forEachAnnotation(Class type, Class> onClass, Predicate filter, Consumer action);
/**
* Finds the first matching annotation on the specified class.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onClass The class to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if not found.
*/
A firstAnnotation(Class type, Class> onClass, Predicate filter);
/**
* Finds the last matching annotation on the specified class.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onClass The class to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if not found.
*/
A lastAnnotation(Class type, Class> onClass, Predicate filter);
/**
* Performs an action on the matching declared annotations on the specified class.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onClass The class to search on.
* @param filter A predicate to apply to the entries to determine if action should be performed. Can be null .
* @param action An action to perform on the entry.
*/
void forEachDeclaredAnnotation(Class type, Class> onClass, Predicate filter, Consumer action);
/**
* Finds the first matching declared annotations on the specified class.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onClass The class to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if no annotations matched.
*/
A firstDeclaredAnnotation(Class type, Class> onClass, Predicate filter);
/**
* Finds the last matching declared annotations on the specified class.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onClass The class to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if no annotations matched.
*/
A lastDeclaredAnnotation(Class type, Class> onClass, Predicate filter);
/**
* Performs an action on the matching annotations on the specified method.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onMethod The method to search on.
* @param filter A predicate to apply to the entries to determine if action should be performed. Can be null .
* @param action An action to perform on the entry.
*/
void forEachAnnotation(Class type, Method onMethod, Predicate filter, Consumer action);
/**
* Finds the first matching annotation on the specified method.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onMethod The method to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if no annotations matched.
*/
A firstAnnotation(Class type, Method onMethod, Predicate filter);
/**
* Finds the last matching annotation on the specified method.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onMethod The method to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if no annotations matched.
*/
A lastAnnotation(Class type, Method onMethod, Predicate filter);
/**
* Performs an action on the matching annotations on the specified field.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onField The field to search on.
* @param filter A predicate to apply to the entries to determine if action should be performed. Can be null .
* @param action An action to perform on the entry.
*/
void forEachAnnotation(Class type, Field onField, Predicate filter, Consumer action);
/**
* Finds the first matching annotation on the specified field.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onField The field to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if no annotations matched.
*/
A firstAnnotation(Class type, Field onField, Predicate filter);
/**
* Finds the last matching annotation on the specified field.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onField The field to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if no annotations matched.
*/
A lastAnnotation(Class type, Field onField, Predicate filter);
/**
* Performs an action on the matching annotations on the specified constructor.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onConstructor The constructor to search on.
* @param filter A predicate to apply to the entries to determine if action should be performed. Can be null .
* @param action An action to perform on the entry.
*/
void forEachAnnotation(Class type, Constructor> onConstructor, Predicate filter, Consumer action);
/**
* Finds the first matching annotation on the specified constructor.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onConstructor The constructor to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if no annotations matched.
*/
A firstAnnotation(Class type, Constructor> onConstructor, Predicate filter);
/**
* Finds the last matching annotation on the specified constructor.
*
* @param The annotation type to find.
* @param type The annotation type to find.
* @param onConstructor The constructor to search on.
* @param filter A predicate to apply to the entries to determine if value should be used. Can be null .
* @return The matched annotation, or null if no annotations matched.
*/
A lastAnnotation(Class type, Constructor> onConstructor, Predicate filter);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy