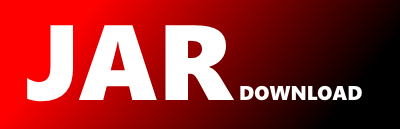
org.apache.juneau.BeanContextable Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau;
import static org.apache.juneau.collections.JsonMap.*;
import java.beans.*;
import java.io.*;
import java.lang.annotation.*;
import java.lang.reflect.*;
import java.util.*;
import java.util.function.*;
import org.apache.juneau.annotation.*;
import org.apache.juneau.collections.*;
import org.apache.juneau.internal.*;
import org.apache.juneau.swap.*;
import org.apache.juneau.utils.*;
/**
* Context class for classes that use {@link BeanContext} objects.
*
*
* This abstraction exists to allow different kinds of subclasses (e.g. JsonSerilalizer, XmlParser...) to share bean context objects since
* bean context objects are heavyweight objects that cache metadata about encountered beans.
*
*
Notes:
* - This class is thread safe and reusable.
*
*/
public abstract class BeanContextable extends Context {
//-----------------------------------------------------------------------------------------------------------------
// Builder
//-----------------------------------------------------------------------------------------------------------------
/**
* Builder class.
*/
@FluentSetters(ignore={"annotations","debug"})
public abstract static class Builder extends Context.Builder {
BeanContext.Builder bcBuilder;
BeanContext bc;
/**
* Constructor.
*
* All default settings.
*/
protected Builder() {
this.bcBuilder = BeanContext.create();
registerBuilders(bcBuilder);
}
/**
* Copy constructor.
*
* @param copyFrom The bean to copy from.
*/
protected Builder(BeanContextable copyFrom) {
super(copyFrom);
this.bcBuilder = copyFrom.getBeanContext().copy();
registerBuilders(bcBuilder);
}
/**
* Copy constructor.
*
* @param copyFrom The builder to copy from.
*/
protected Builder(Builder copyFrom) {
super(copyFrom);
this.bcBuilder = copyFrom.bcBuilder.copy();
this.bc = copyFrom.bc;
registerBuilders(bcBuilder);
}
@Override /* Context.Builder */
public abstract Builder copy();
@Override /* Context.Builder */
public HashKey hashKey() {
return HashKey.of(
super.hashKey(),
bcBuilder.hashKey(),
bc == null ? 0 : bc.hashKey
);
}
/**
* Returns the inner bean context builder.
*
* @return The inner bean context builder.
*/
public BeanContext.Builder beanContext() {
return bcBuilder;
}
/**
* Applies an operation to the inner bean context builder.
*
* @param operation The operation to apply.
* @return This object.
*/
public final Builder beanContext(Consumer operation) {
operation.accept(beanContext());
return this;
}
//-----------------------------------------------------------------------------------------------------------------
// Properties
//-----------------------------------------------------------------------------------------------------------------
/**
* Overrides the bean context builder.
*
*
* Used when sharing bean context builders across multiple context objects.
* For example, {@link org.apache.juneau.jsonschema.JsonSchemaGenerator.Builder} uses this to apply common bean settings with the JSON
* serializer and parser.
*
* @param value The new value for this setting.
* @return This object.
*/
@FluentSetter
public Builder beanContext(BeanContext.Builder value) {
this.bcBuilder = value;
return this;
}
/**
* Specifies an already-instantiated bean context to use.
*
*
* Provides an optimization for cases where serializers and parsers can use an existing
* bean context without having to go through beanContext .copy().build() .
* An example is {@link BeanContext#getBeanToStringSerializer()}.
*
* @param value The bean context to use.
* @return This object.
*/
@FluentSetter
public Builder beanContext(BeanContext value) {
this.bc = value;
return this;
}
/**
* Minimum bean class visibility.
*
*
* Classes are not considered beans unless they meet the minimum visibility requirements.
* For example, if the visibility is PUBLIC and the bean class is protected , then the class
* will not be interpreted as a bean class and be serialized as a string.
* Use this setting to reduce the visibility requirement.
*
*
Example:
*
* // A bean with a protected class and one field.
* protected class MyBean {
* public String foo = "bar" ;
* }
*
* // Create a serializer that's capable of serializing the class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanClassVisibility(PROTECTED )
* .build();
*
* // Produces: {"foo","bar"}
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
*
* - The {@link Bean @Bean} annotation can be used on a non-public bean class to override this setting.
*
- The {@link BeanIgnore @BeanIgnore} annotation can also be used on a public bean class to ignore it as a bean.
*
*
* See Also:
*
* - {@link BeanConfig#beanClassVisibility()}
*
*
* @param value
* The new value for this setting.
*
The default is {@link Visibility#PUBLIC}.
* @return This object.
*/
@FluentSetter
public Builder beanClassVisibility(Visibility value) {
bcBuilder.beanClassVisibility(value);
return this;
}
/**
* Minimum bean constructor visibility.
*
*
* Only look for constructors with the specified minimum visibility.
*
*
* This setting affects the logic for finding no-arg constructors for bean. Normally, only public no-arg
* constructors are used. Use this setting if you want to reduce the visibility requirement.
*
*
Example:
*
* // A bean with a protected constructor and one field.
* public class MyBean {
* public String foo ;
*
* protected MyBean() {}
* }
*
* // Create a parser capable of calling the protected constructor.
* ReaderParser parser = ReaderParser
* .create ()
* .beanConstructorVisibility(PROTECTED )
* .build();
*
* // Use it.
* MyBean bean = parser .parse("{foo:'bar'}" , MyBean.class );
*
*
* Notes:
* - The {@link Beanc @Beanc} annotation can also be used to expose a non-public constructor.
*
- The {@link BeanIgnore @BeanIgnore} annotation can also be used on a public bean constructor to ignore it.
*
*
* See Also:
* - {@link BeanConfig#beanConstructorVisibility()}
*
*
* @param value
* The new value for this setting.
*
The default is {@link Visibility#PUBLIC}.
* @return This object.
*/
@FluentSetter
public Builder beanConstructorVisibility(Visibility value) {
bcBuilder.beanConstructorVisibility(value);
return this;
}
/**
* Minimum bean field visibility.
*
*
* Only look for bean fields with the specified minimum visibility.
*
*
* This affects which fields on a bean class are considered bean properties. Normally only public fields are considered.
* Use this setting if you want to reduce the visibility requirement.
*
*
Example:
*
* // A bean with a protected field.
* public class MyBean {
* protected String foo = "bar" ;
* }
*
* // Create a serializer that recognizes the protected field.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanFieldVisibility(PROTECTED )
* .build();
*
* // Produces: {"foo":"bar"}
* String json = serializer .serialize(new MyBean());
*
*
*
* Bean fields can be ignored as properties entirely by setting the value to {@link Visibility#NONE}
*
*
* // Disable using fields as properties entirely.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanFieldVisibility(NONE )
* .build();
*
*
* Notes:
* - The {@link Beanp @Beanp} annotation can also be used to expose a non-public field.
*
- The {@link BeanIgnore @BeanIgnore} annotation can also be used on a public bean field to ignore it as a bean property.
*
*
* See Also:
* - {@link BeanConfig#beanFieldVisibility()}
*
*
* @param value
* The new value for this setting.
*
The default is {@link Visibility#PUBLIC}.
* @return This object.
*/
@FluentSetter
public Builder beanFieldVisibility(Visibility value) {
bcBuilder.beanFieldVisibility(value);
return this;
}
/**
* Bean interceptor.
*
*
* Bean interceptors can be used to intercept calls to getters and setters and alter their values in transit.
*
*
Example:
*
* // Interceptor that strips out sensitive information.
* public class AddressInterceptor extends BeanInterceptor<Address> {
*
* public Object readProperty(Address bean , String name , Object value ) {
* if ("taxInfo" .equals(name ))
* return "redacted" ;
* return value ;
* }
*
* public Object writeProperty(Address bean , String name , Object value ) {
* if ("taxInfo" .equals(name ) && "redacted" .equals(value ))
* return TaxInfoUtils.lookup (bean .getStreet(), bean .getCity(), bean .getState());
* return value ;
* }
* }
*
* // Our bean class.
* public class Address {
* public String getTaxInfo() {...}
* public void setTaxInfo(String value ) {...}
* }
*
* // Register filter on serializer or parser.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanInterceptor(Address.class , AddressInterceptor.class )
* .build();
*
* // Produces: {"taxInfo":"redacted"}
* String json = serializer .serialize(new Address());
*
*
* See Also:
* - {@link BeanInterceptor}
*
- {@link Bean#interceptor() Bean(interceptor)}
*
*
* @param on The bean that the filter applies to.
* @param value
* The new value for this setting.
* @return This object.
*/
@FluentSetter
public Builder beanInterceptor(Class> on, Class extends BeanInterceptor>> value) {
bcBuilder.beanInterceptor(on, value);
return this;
}
/**
* BeanMap.put() returns old property value.
*
*
* When enabled, then the {@link BeanMap#put(String,Object) BeanMap.put()} method will return old property
* values. Otherwise, it returns null .
*
*
* Disabled by default because it introduces a slight performance penalty during serialization.
*
*
Example:
*
* // Create a context that creates BeanMaps with normal put() behavior.
* BeanContext context = BeanContext
* .create ()
* .beanMapPutReturnsOldValue()
* .build();
*
* BeanMap<MyBean> beanMap = context .createSession().toBeanMap(new MyBean());
* beanMap .put("foo" , "bar" );
* Object oldValue = beanMap .put("foo" , "baz" ); // oldValue == "bar"
*
*
* See Also:
* - {@link BeanConfig#beanMapPutReturnsOldValue()}
*
- {@link BeanContext.Builder#beanMapPutReturnsOldValue()}
*
*
* @return This object.
*/
@FluentSetter
public Builder beanMapPutReturnsOldValue() {
bcBuilder.beanMapPutReturnsOldValue();
return this;
}
/**
* Minimum bean method visibility.
*
*
* Only look for bean methods with the specified minimum visibility.
*
*
* This affects which methods are detected as getters and setters on a bean class. Normally only public getters and setters are considered.
* Use this setting if you want to reduce the visibility requirement.
*
*
Example:
*
* // A bean with a protected getter.
* public class MyBean {
* public String getFoo() { return "foo" ; }
* protected String getBar() { return "bar" ; }
* }
*
* // Create a serializer that looks for protected getters and setters.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanMethodVisibility(PROTECTED )
* .build();
*
* // Produces: {"foo":"foo","bar":"bar"}
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
* - The {@link Beanp @Beanp} annotation can also be used to expose a non-public method.
*
- The {@link BeanIgnore @BeanIgnore} annotation can also be used on a public bean getter/setter to ignore it as a bean property.
*
*
* See Also:
* - {@link BeanConfig#beanMethodVisibility()}
*
*
* @param value
* The new value for this setting.
*
The default is {@link Visibility#PUBLIC}
* @return This object.
*/
@FluentSetter
public Builder beanMethodVisibility(Visibility value) {
bcBuilder.beanMethodVisibility(value);
return this;
}
/**
* Beans require no-arg constructors.
*
*
* When enabled, a Java class must implement a default no-arg constructor to be considered a bean.
* Otherwise, the bean will be serialized as a string using the {@link Object#toString()} method.
*
*
Example:
*
* // A bean without a no-arg constructor.
* public class MyBean {
*
* // A property method.
* public String foo = "bar" ;
*
* // A no-arg constructor
* public MyBean(String foo ) {
* this .foo = foo ;
* }
*
* @Override
* public String toString() {
* return "bar" ;
* }
* }
*
* // Create a serializer that ignores beans without default constructors.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beansRequireDefaultConstructor()
* .build();
*
* // Produces: "bar"
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
* - The {@link Bean @Bean} annotation can be used on a bean class to override this setting.
*
- The {@link BeanIgnore @BeanIgnore} annotation can also be used on a class to ignore it as a bean.
*
*
* See Also:
* - {@link BeanConfig#beansRequireDefaultConstructor()}
*
- {@link BeanContext.Builder#beansRequireDefaultConstructor()}
*
*
* @return This object.
*/
@FluentSetter
public Builder beansRequireDefaultConstructor() {
bcBuilder.beansRequireDefaultConstructor();
return this;
}
/**
* Beans require Serializable interface.
*
*
* When enabled, a Java class must implement the {@link Serializable} interface to be considered a bean.
* Otherwise, the bean will be serialized as a string using the {@link Object#toString()} method.
*
*
Example:
*
* // A bean without a Serializable interface.
* public class MyBean {
*
* // A property method.
* public String foo = "bar" ;
*
* @Override
* public String toString() {
* return "bar" ;
* }
* }
*
* // Create a serializer that ignores beans not implementing Serializable.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beansRequireSerializable()
* .build();
*
* // Produces: "bar"
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
* - The {@link Bean @Bean} annotation can be used on a bean class to override this setting.
*
- The {@link BeanIgnore @BeanIgnore} annotation can also be used on a class to ignore it as a bean.
*
*
* See Also:
* - {@link BeanConfig#beansRequireSerializable()}
*
- {@link BeanContext.Builder#beansRequireSerializable()}
*
*
* @return This object.
*/
@FluentSetter
public Builder beansRequireSerializable() {
bcBuilder.beansRequireSerializable();
return this;
}
/**
* Beans require setters for getters.
*
*
* When enabled, ignore read-only properties (properties with getters but not setters).
*
*
Example:
*
* // A bean without a Serializable interface.
* public class MyBean {
*
* // A read/write property.
* public String getFoo() { return "foo" ; }
* public void setFoo(String foo ) { ... }
*
* // A read-only property.
* public String getBar() { return "bar" ; }
* }
*
* // Create a serializer that ignores bean properties without setters.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beansRequireSettersForGetters()
* .build();
*
* // Produces: {"foo":"foo"}
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
* - The {@link Beanp @Beanp} annotation can be used on the getter to override this setting.
*
- The {@link BeanIgnore @BeanIgnore} annotation can also be used on getters to ignore them as bean properties.
*
*
* See Also:
* - {@link BeanConfig#beansRequireSettersForGetters()}
*
- {@link BeanContext.Builder#beansRequireSettersForGetters()}
*
*
* @return This object.
*/
@FluentSetter
public Builder beansRequireSettersForGetters() {
bcBuilder.beansRequireSettersForGetters();
return this;
}
/**
* Beans don't require at least one property.
*
*
* When enabled, then a Java class doesn't need to contain at least 1 property to be considered a bean.
* Otherwise, the bean will be serialized as a string using the {@link Object#toString()} method.
*
*
* The {@link Bean @Bean} annotation can be used on a class to override this setting when true .
*
*
Example:
*
* // A bean with no properties.
* public class MyBean {
* }
*
* // Create a serializer that serializes beans even if they have zero properties.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .disableBeansRequireSomeProperties()
* .build();
*
* // Produces: {}
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
* - The {@link Bean @Bean} annotation can be used on the class to force it to be recognized as a bean class
* even if it has no properties.
*
*
* See Also:
* - {@link BeanConfig#disableBeansRequireSomeProperties()}
*
- {@link BeanContext.Builder#disableBeansRequireSomeProperties()}
*
*
* @return This object.
*/
@FluentSetter
public Builder disableBeansRequireSomeProperties() {
bcBuilder.disableBeansRequireSomeProperties();
return this;
}
/**
* Bean property includes.
*
*
* Specifies the set and order of names of properties associated with the bean class.
*
*
* For example, beanProperties(MyBean.class , "foo,bar" ) means only serialize the foo and
* bar properties on the specified bean. Likewise, parsing will ignore any bean properties not specified
* and either throw an exception or silently ignore them depending on whether {@link #ignoreUnknownBeanProperties()}
* has been called.
*
*
* This value is entirely optional if you simply want to expose all the getters and public fields on
* a class as bean properties. However, it's useful if you want certain getters to be ignored or you want the properties to be
* serialized in a particular order. Note that on IBM JREs, the property order is the same as the order in the source code,
* whereas on Oracle JREs, the order is entirely random.
*
*
* Setting applies to specified class and all subclasses.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String
* foo = "foo" ,
* bar = "bar" ,
* baz = "baz" ;
* }
*
* // Create a serializer that includes only the 'foo' and 'bar' properties on the MyBean class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanProperties(MyBean.class , "foo,bar" )
* .build();
*
* // Produces: {"foo":"foo","bar":"bar"}
* String json = serializer .serialize(new MyBean());
*
*
*
* This method is functionally equivalent to the following code:
*
* builder .annotations(BeanAnnotation.create (beanClass ).properties(properties ).build());
*
*
* See Also:
* - {@link Bean#properties()}/{@link Bean#p()} - On an annotation on the bean class itself.
*
*
* @param beanClass The bean class.
* @param properties Comma-delimited list of property names.
* @return This object.
*/
@FluentSetter
public Builder beanProperties(Class> beanClass, String properties) {
bcBuilder.beanProperties(beanClass, properties);
return this;
}
/**
* Bean property includes.
*
*
* Specifies the set and order of names of properties associated with bean classes.
*
*
* For example, beanProperties(AMap.of ("MyBean" , "foo,bar" )) means only serialize the foo and
* bar properties on the specified bean. Likewise, parsing will ignore any bean properties not specified
* and either throw an exception or silently ignore them depending on whether {@link #ignoreUnknownBeanProperties()}
* has been called.
*
*
* This value is entirely optional if you simply want to expose all the getters and public fields on
* a class as bean properties. However, it's useful if you want certain getters to be ignored or you want the properties to be
* serialized in a particular order. Note that on IBM JREs, the property order is the same as the order in the source code,
* whereas on Oracle JREs, the order is entirely random.
*
*
* Setting applies to specified class and all subclasses.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String
* foo = "foo" ,
* bar = "bar" ,
* baz = "baz" ;
* }
*
* // Create a serializer that includes only the 'foo' and 'bar' properties on the MyBean class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanProperties(AMap.of ("MyBean" , "foo,bar" ))
* .build();
*
* // Produces: {"foo":"foo","bar":"bar"}
* String json = serializer .serialize(new MyBean());
*
*
*
* This method is functionally equivalent to the following code for each entry:
*
* builder .annotations(BeanAnnotation.create (key ).properties(value .toString()).build());
*
*
* See Also:
* - {@link Bean#properties()} / {@link Bean#p()}- On an annotation on the bean class itself.
*
*
* @param values
* The values to add to this builder.
*
Keys are bean class names which can be a simple name, fully-qualified name, or "*" for all beans.
*
Values are comma-delimited lists of property names. Non-String objects are first converted to Strings.
* @return This object.
*/
@FluentSetter
public Builder beanProperties(Map values) {
bcBuilder.beanProperties(values);
return this;
}
/**
* Bean property includes.
*
*
* Specifies the set and order of names of properties associated with the bean class.
*
*
* For example, beanProperties("MyBean" , "foo,bar" ) means only serialize the foo and
* bar properties on the specified bean. Likewise, parsing will ignore any bean properties not specified
* and either throw an exception or silently ignore them depending on whether {@link #ignoreUnknownBeanProperties()}
* has been called.
*
*
* This value is entirely optional if you simply want to expose all the getters and public fields on
* a class as bean properties. However, it's useful if you want certain getters to be ignored or you want the properties to be
* serialized in a particular order. Note that on IBM JREs, the property order is the same as the order in the source code,
* whereas on Oracle JREs, the order is entirely random.
*
*
* Setting applies to specified class and all subclasses.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String
* foo = "foo" ,
* bar = "bar" ,
* baz = "baz" ;
* }
*
* // Create a serializer that includes only the 'foo' and 'bar' properties on the MyBean class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanProperties("MyBean" , "foo,bar" )
* .build();
*
* // Produces: {"foo":"foo","bar":"bar"}
* String json = serializer .serialize(new MyBean());
*
*
*
* This method is functionally equivalent to the following code:
*
* builder .annotations(BeanAnnotation.create (beanClassName ).properties(properties ).build());
*
*
* See Also:
* - {@link Bean#properties()} / {@link Bean#p()} - On an annotation on the bean class itself.
*
*
* @param beanClassName
* The bean class name.
*
Can be a simple name, fully-qualified name, or "*" for all beans.
* @param properties Comma-delimited list of property names.
* @return This object.
*/
@FluentSetter
public Builder beanProperties(String beanClassName, String properties) {
bcBuilder.beanProperties(beanClassName, properties);
return this;
}
/**
* Bean property excludes.
*
*
* Specifies to exclude the specified list of properties for the specified bean class.
*
*
* Same as {@link #beanProperties(Class, String)} except you specify a list of bean property names that you want to exclude from
* serialization.
*
*
* Setting applies to specified class and all subclasses.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String
* foo = "foo" ,
* bar = "bar" ,
* baz = "baz" ;
* }
*
* // Create a serializer that excludes the "bar" and "baz" properties on the MyBean class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanPropertiesExcludes(MyBean.class , "bar,baz" )
* .build();
*
* // Produces: {"foo":"foo"}
* String json = serializer .serialize(new MyBean());
*
*
*
* This method is functionally equivalent to the following code:
*
* builder .annotations(BeanAnnotation.create (beanClass ).excludeProperties(properties ).build());
*
*
* See Also:
* - {@link Bean#excludeProperties()} / {@link Bean#xp()}
*
*
* @param beanClass The bean class.
* @param properties Comma-delimited list of property names.
* @return This object.
*/
@FluentSetter
public Builder beanPropertiesExcludes(Class> beanClass, String properties) {
bcBuilder.beanPropertiesExcludes(beanClass, properties);
return this;
}
/**
* Bean property excludes.
*
*
* Specifies to exclude the specified list of properties for the specified bean classes.
*
*
* Same as {@link #beanProperties(Map)} except you specify a list of bean property names that you want to exclude from
* serialization.
*
*
* Setting applies to specified class and all subclasses.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String
* foo = "foo" ,
* bar = "bar" ,
* baz = "baz" ;
* }
*
* // Create a serializer that excludes the "bar" and "baz" properties on the MyBean class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanPropertiesExcludes(AMap.of("MyBean" , "bar,baz" ))
* .build();
*
* // Produces: {"foo":"foo"}
* String json = serializer .serialize(new MyBean());
*
*
*
* This method is functionally equivalent to the following code for each entry:
*
* builder .annotations(BeanAnnotation.create (key ).excludeProperties(value .toString()).build());
*
*
* See Also:
* - {@link Bean#excludeProperties()} / {@link Bean#xp()}
*
*
* @param values
* The values to add to this builder.
*
Keys are bean class names which can be a simple name, fully-qualified name, or "*" for all beans.
*
Values are comma-delimited lists of property names. Non-String objects are first converted to Strings.
* @return This object.
*/
@FluentSetter
public Builder beanPropertiesExcludes(Map values) {
bcBuilder.beanPropertiesExcludes(values);
return this;
}
/**
* Bean property excludes.
*
*
* Specifies to exclude the specified list of properties for the specified bean class.
*
*
* Same as {@link #beanPropertiesExcludes(String, String)} except you specify a list of bean property names that you want to exclude from
* serialization.
*
*
* Setting applies to specified class and all subclasses.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String
* foo = "foo" ,
* bar = "bar" ,
* baz = "baz" ;
* }
*
* // Create a serializer that excludes the "bar" and "baz" properties on the MyBean class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanPropertiesExcludes("MyBean" , "bar,baz" )
* .build();
*
* // Produces: {"foo":"foo"}
* String json = serializer .serialize(new MyBean());
*
*
*
* This method is functionally equivalent to the following code:
*
* builder .annotations(BeanAnnotation.create (beanClassName ).excludeProperties(properties ).build());
*
*
* See Also:
* - {@link Bean#excludeProperties()} / {@link Bean#xp()}
*
*
* @param beanClassName
* The bean class name.
*
Can be a simple name, fully-qualified name, or "*" for all bean classes.
* @param properties Comma-delimited list of property names.
* @return This object.
*/
@FluentSetter
public Builder beanPropertiesExcludes(String beanClassName, String properties) {
bcBuilder.beanPropertiesExcludes(beanClassName, properties);
return this;
}
/**
* Read-only bean properties.
*
*
* Specifies one or more properties on a bean that are read-only despite having valid getters.
* Serializers will serialize such properties as usual, but parsers will silently ignore them.
* Note that this is different from the {@link #beanProperties(Class,String) beanProperties}/{@link #beanPropertiesExcludes(Class,String) beanPropertiesExcludes} settings which include or exclude properties
* for both serializers and parsers.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String foo , bar , baz ;
* }
*
* // Create a serializer with read-only property settings.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanPropertiesReadOnly(MyBean.class , "bar,baz" )
* .build();
*
* // All 3 properties will be serialized.
* String json = serializer .serialize(new MyBean());
*
* // Create a parser with read-only property settings.
* ReaderParser parser = JsonParser
* .create ()
* .beanPropertiesReadOnly(MyBean.class , "bar,baz" )
* .ignoreUnknownBeanProperties()
* .build();
*
* // Parser ignores bar and baz properties.
* MyBean bean = parser .parse("{foo:'foo',bar:'bar',baz:'baz'}" , MyBean.class );
*
*
*
* This method is functionally equivalent to the following code:
*
* builder .annotations(BeanAnnotation.create (beanClass ).readOnlyProperties(properties ).build());
*
*
* See Also:
* - {@link Bean#readOnlyProperties()} / {@link Bean#ro()}
*
*
* @param beanClass The bean class.
* @param properties Comma-delimited list of property names.
* @return This object.
*/
@FluentSetter
public Builder beanPropertiesReadOnly(Class> beanClass, String properties) {
bcBuilder.beanPropertiesReadOnly(beanClass, properties);
return this;
}
/**
* Read-only bean properties.
*
*
* Specifies one or more properties on beans that are read-only despite having valid getters.
* Serializers will serialize such properties as usual, but parsers will silently ignore them.
* Note that this is different from the {@link #beanProperties(Class,String) beanProperties}/{@link #beanPropertiesExcludes(Class,String) beanPropertiesExcludes} settings which include or exclude properties
* for both serializers and parsers.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String foo , bar , baz ;
* }
*
* // Create a serializer with read-only property settings.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanPropertiesReadOnly(AMap.of ("MyBean" , "bar,baz" ))
* .build();
*
* // All 3 properties will be serialized.
* String json = serializer .serialize(new MyBean());
*
* // Create a parser with read-only property settings.
* ReaderParser parser = JsonParser
* .create ()
* .beanPropertiesReadOnly(AMap.of ("MyBean" , "bar,baz" ))
* .ignoreUnknownBeanProperties()
* .build();
*
* // Parser ignores bar and baz properties.
* MyBean bean = parser .parse("{foo:'foo',bar:'bar',baz:'baz'}" , MyBean.class );
*
*
*
* This method is functionally equivalent to the following code for each entry:
*
* builder .annotations(BeanAnnotation.create (key ).readOnlyProperties(value .toString()).build());
*
*
* See Also:
* - {@link Bean#readOnlyProperties()} / {@link Bean#ro()}
*
*
* @param values
* The values to add to this builder.
*
Keys are bean class names which can be a simple name, fully-qualified name, or "*" for all beans.
*
Values are comma-delimited lists of property names. Non-String objects are first converted to Strings.
* @return This object.
*/
@FluentSetter
public Builder beanPropertiesReadOnly(Map values) {
bcBuilder.beanPropertiesReadOnly(values);
return this;
}
/**
* Read-only bean properties.
*
*
* Specifies one or more properties on a bean that are read-only despite having valid getters.
* Serializers will serialize such properties as usual, but parsers will silently ignore them.
* Note that this is different from the {@link #beanProperties(Class,String) beanProperties}/{@link #beanPropertiesExcludes(Class,String) beanPropertiesExcludes} settings which include or exclude properties
* for both serializers and parsers.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String foo , bar , baz ;
* }
*
* // Create a serializer with read-only property settings.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanPropertiesReadOnly("MyBean" , "bar,baz" )
* .build();
*
* // All 3 properties will be serialized.
* String json = serializer .serialize(new MyBean());
*
* // Create a parser with read-only property settings.
* ReaderParser parser = JsonParser
* .create ()
* .beanPropertiesReadOnly("MyBean" , "bar,baz" )
* .ignoreUnknownBeanProperties()
* .build();
*
* // Parser ignores bar and baz properties.
* MyBean bean = parser .parse("{foo:'foo',bar:'bar',baz:'baz'}" , MyBean.class );
*
*
*
* This method is functionally equivalent to the following code:
*
* builder .annotations(BeanAnnotation.create (beanClassName ).readOnlyProperties(properties ).build());
*
*
* See Also:
* - {@link Bean#readOnlyProperties()} / {@link Bean#ro()}
*
*
* @param beanClassName
* The bean class name.
*
Can be a simple name, fully-qualified name, or "*" for all bean classes.
* @param properties Comma-delimited list of property names.
* @return This object.
*/
@FluentSetter
public Builder beanPropertiesReadOnly(String beanClassName, String properties) {
bcBuilder.beanPropertiesReadOnly(beanClassName, properties);
return this;
}
/**
* Write-only bean properties.
*
*
* Specifies one or more properties on a bean that are write-only despite having valid setters.
* Parsers will parse such properties as usual, but serializers will silently ignore them.
* Note that this is different from the {@link #beanProperties(Class,String) beanProperties}/{@link #beanPropertiesExcludes(Class,String) beanPropertiesExcludes} settings which include or exclude properties
* for both serializers and parsers.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String foo , bar , baz ;
* }
*
* // Create a serializer with write-only property settings.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanPropertiesWriteOnly(MyBean.class , "bar,baz" )
* .build();
*
* // Only foo will be serialized.
* String json = serializer .serialize(new MyBean());
*
* // Create a parser with write-only property settings.
* ReaderParser parser = JsonParser
* .create ()
* .beanPropertiesWriteOnly(MyBean.class , "bar,baz" )
* .build();
*
* // Parser parses all 3 properties.
* MyBean bean = parser .parse("{foo:'foo',bar:'bar',baz:'baz'}" , MyBean.class );
*
*
*
* This method is functionally equivalent to the following code:
*
* builder .annotations(BeanAnnotation.create (beanClass ).writeOnlyProperties(properties ).build());
*
*
* See Also:
* - {@link Bean#writeOnlyProperties()} / {@link Bean#wo()}
*
*
* @param beanClass The bean class.
* @param properties Comma-delimited list of property names.
* @return This object.
*/
@FluentSetter
public Builder beanPropertiesWriteOnly(Class> beanClass, String properties) {
bcBuilder.beanPropertiesWriteOnly(beanClass, properties);
return this;
}
/**
* Write-only bean properties.
*
*
* Specifies one or more properties on a bean that are write-only despite having valid setters.
* Parsers will parse such properties as usual, but serializers will silently ignore them.
* Note that this is different from the {@link #beanProperties(Class,String) beanProperties}/{@link #beanPropertiesExcludes(Class,String) beanPropertiesExcludes} settings which include or exclude properties
* for both serializers and parsers.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String foo , bar , baz ;
* }
*
* // Create a serializer with write-only property settings.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanPropertiesWriteOnly(AMap.of ("MyBean" , "bar,baz" ))
* .build();
*
* // Only foo will be serialized.
* String json = serializer .serialize(new MyBean());
*
* // Create a parser with write-only property settings.
* ReaderParser parser = JsonParser
* .create ()
* .beanPropertiesWriteOnly(AMap.of ("MyBean" , "bar,baz" ))
* .build();
*
* // Parser parses all 3 properties.
* MyBean bean = parser .parse("{foo:'foo',bar:'bar',baz:'baz'}" , MyBean.class );
*
*
*
* This method is functionally equivalent to the following code for each entry:
*
* builder .annotations(BeanAnnotation.create (key ).writeOnlyProperties(value .toString()).build());
*
*
* See Also:
* - {@link Bean#writeOnlyProperties()} / {@link Bean#wo()}
*
*
* @param values
* The values to add to this builder.
*
Keys are bean class names which can be a simple name, fully-qualified name, or "*" for all beans.
*
Values are comma-delimited lists of property names. Non-String objects are first converted to Strings.
* @return This object.
*/
@FluentSetter
public Builder beanPropertiesWriteOnly(Map values) {
bcBuilder.beanPropertiesWriteOnly(values);
return this;
}
/**
* Write-only bean properties.
*
*
* Specifies one or more properties on a bean that are write-only despite having valid setters.
* Parsers will parse such properties as usual, but serializers will silently ignore them.
* Note that this is different from the {@link #beanProperties(Class,String) beanProperties}/{@link #beanPropertiesExcludes(Class,String) beanPropertiesExcludes} settings which include or exclude properties
* for both serializers and parsers.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String foo , bar , baz ;
* }
*
* // Create a serializer with write-only property settings.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .beanPropertiesWriteOnly("MyBean" , "bar,baz" )
* .build();
*
* // Only foo will be serialized.
* String json = serializer .serialize(new MyBean());
*
* // Create a parser with write-only property settings.
* ReaderParser parser = JsonParser
* .create ()
* .beanPropertiesWriteOnly("MyBean" , "bar,baz" )
* .build();
*
* // Parser parses all 3 properties.
* MyBean bean = parser .parse("{foo:'foo',bar:'bar',baz:'baz'}" , MyBean.class );
*
*
*
* This method is functionally equivalent to the following code:
*
* builder .annotations(BeanAnnotation.create (beanClassName ).writeOnlyProperties(properties ).build());
*
*
* See Also:
* - {@link Bean#writeOnlyProperties()} / {@link Bean#wo()}
*
*
* @param beanClassName
* The bean class name.
*
Can be a simple name, fully-qualified name, or "*" for all bean classes.
* @param properties Comma-delimited list of property names.
* @return This object.
*/
@FluentSetter
public Builder beanPropertiesWriteOnly(String beanClassName, String properties) {
bcBuilder.beanPropertiesWriteOnly(beanClassName, properties);
return this;
}
/**
* Bean dictionary.
*
*
* The list of classes that make up the bean dictionary in this bean context.
*
*
* Values are prepended to the list so that later calls can override classes of earlier calls.
*
*
* A dictionary is a name/class mapping used to find class types during parsing when they cannot be inferred
* through reflection. The names are defined through the {@link Bean#typeName() @Bean(typeName)} annotation defined
* on the bean class. For example, if a class Foo has a type-name of "myfoo" , then it would end up
* serialized as "{_type:'myfoo',...}" in JSON
* or "<myfoo>...</myfoo>" in XML.
*
*
* This setting tells the parsers which classes to look for when resolving "_type" attributes.
*
*
* Values can consist of any of the following types:
*
* - Any bean class that specifies a value for {@link Bean#typeName() @Bean(typeName)}.
*
- Any subclass of {@link BeanDictionaryList} containing a collection of bean classes with type name annotations.
*
- Any subclass of {@link BeanDictionaryMap} containing a mapping of type names to classes without type name annotations.
*
- Any array or collection of the objects above.
*
*
* Example:
*
* // POJOs with @Bean(name) annotations.
* @Bean (typeName="foo" )
* public class Foo {...}
* @Bean (typeName="bar" )
* public class Bar {...}
*
* // Create a parser and tell it which classes to try to resolve.
* ReaderParser parser = JsonParser
* .create ()
* .dictionary(Foo.class , Bar.class )
* .addBeanTypes()
* .build();
*
* // A bean with a field with an indeterminate type.
* public class MyBean {
* public Object mySimpleField ;
* }
*
* // Parse bean.
* MyBean bean = parser .parse("{mySimpleField:{_type:'foo',...}}" , MyBean.class );
*
*
*
* Another option is to use the {@link Bean#dictionary()} annotation on the POJO class itself:
*
*
* // Instead of by parser, define a bean dictionary on a class through an annotation.
* // This applies to all properties on this class and all subclasses.
* @Bean (dictionary={Foo.class ,Bar.class })
* public class MyBean {
* public Object mySimpleField ; // May contain Foo or Bar object.
* public Map<String,Object> myMapField ; // May contain Foo or Bar objects.
* }
*
*
*
* A typical usage is to allow for HTML documents to be parsed back into HTML beans:
*
* // Use the predefined HTML5 bean dictionary which is a BeanDictionaryList.
* ReaderParser parser = HtmlParser
* .create ()
* .dictionary(HtmlBeanDictionary.class )
* .build();
*
* // Parse an HTML body into HTML beans.
* Body body = parser .parse("<body><ul><li>foo</li><li>bar</li></ul>" , Body.class );
*
*
* See Also:
* - {@link Bean#dictionary()}
*
- {@link Beanp#dictionary()}
*
- {@link BeanConfig#dictionary()}
*
- {@link BeanConfig#dictionary_replace()}
*
*
* @param values
* The values to add to this setting.
* @return This object.
*/
@FluentSetter
public Builder beanDictionary(Class>...values) {
bcBuilder.beanDictionary(values);
return this;
}
/**
* Bean dictionary.
*
*
* This is identical to {@link #beanDictionary(Class...)}, but specifies a dictionary within the context of
* a single class as opposed to globally.
*
*
Example:
*
* // POJOs with @Bean(name) annotations.
* @Bean (typeName="foo" )
* public class Foo {...}
* @Bean (typeName="bar" )
* public class Bar {...}
*
* // A bean with a field with an indeterminate type.
* public class MyBean {
* public Object mySimpleField ;
* }
*
* // Create a parser and tell it which classes to try to resolve.
* ReaderParser parser = JsonParser
* .create ()
* .dictionaryOn(MyBean.class , Foo.class , Bar.class )
* .build();
*
* // Parse bean.
* MyBean bean = parser .parse("{mySimpleField:{_type:'foo',...}}" , MyBean.class );
*
*
*
* This is functionally equivalent to the {@link Bean#dictionary()} annotation.
*
*
See Also:
* - {@link Bean#dictionary()}
*
- {@link BeanContext.Builder#beanDictionary(Class...)}
*
*
* @param on The class that the dictionary values apply to.
* @param values
* The new values for this setting.
* @return This object.
*/
@FluentSetter
public Builder dictionaryOn(Class> on, Class>...values) {
bcBuilder.dictionaryOn(on, values);
return this;
}
/**
* POJO example.
*
*
* Specifies an example of the specified class.
*
*
* Examples are used in cases such as POJO examples in Swagger documents.
*
*
Example:
*
* // Create a serializer that excludes the 'foo' and 'bar' properties on the MyBean class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .example(MyBean.class , new MyBean().setFoo("foo" ).setBar(123))
* .build();
*
*
*
* This is a shorthand method for the following code:
*
* builder .annotations(MarshalledAnnotation.create (pojoClass ).example(Json5.DEFAULT .toString(object )).build())
*
*
* Notes:
* - Using this method assumes the serialized form of the object is the same as that produced
* by the default serializer. This may not be true based on settings or swaps on the constructed serializer.
*
*
*
* POJO examples can also be defined on classes via the following:
*
* - The {@link Marshalled#example()} annotation on the class itself.
*
- A static field annotated with {@link Example @Example}.
*
- A static method annotated with {@link Example @Example} with zero arguments or one {@link BeanSession} argument.
*
- A static method with name
example with no arguments or one {@link BeanSession} argument.
*
*
* @param The POJO class.
* @param pojoClass The POJO class.
* @param o
* An instance of the POJO class used for examples.
* @return This object.
*/
@FluentSetter
public Builder example(Class pojoClass, T o) {
bcBuilder.example(pojoClass, o);
return this;
}
/**
* POJO example.
*
*
* Specifies an example in JSON of the specified class.
*
*
* Examples are used in cases such as POJO examples in Swagger documents.
*
*
* Setting applies to specified class and all subclasses.
*
*
Example:
*
* // Create a serializer that excludes the 'foo' and 'bar' properties on the MyBean class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .example(MyBean.class , "{foo:'bar'}" )
* .build();
*
*
*
* This is a shorthand method for the following code:
*
* builder .annotations(MarshalledAnnotation.create (pojoClass ).example(json ).build())
*
*
*
* POJO examples can also be defined on classes via the following:
*
* - A static field annotated with {@link Example @Example}.
*
- A static method annotated with {@link Example @Example} with zero arguments or one {@link BeanSession} argument.
*
- A static method with name
example with no arguments or one {@link BeanSession} argument.
*
*
* See Also:
* - {@link Marshalled#example()}
*
*
* @param The POJO class type.
* @param pojoClass The POJO class.
* @param json The JSON 5 representation of the example.
* @return This object.
*/
@FluentSetter
public Builder example(Class pojoClass, String json) {
bcBuilder.example(pojoClass, json);
return this;
}
/**
* Find fluent setters.
*
*
* When enabled, fluent setters are detected on beans during parsing.
*
*
* Fluent setters must have the following attributes:
*
* - Public.
*
- Not static.
*
- Take in one parameter.
*
- Return the bean itself.
*
*
* Example:
*
* // A bean with a fluent setter.
* public class MyBean {
* public MyBean foo(String value ) {...}
* }
*
* // Create a parser that finds fluent setters.
* ReaderParser parser = JsonParser
* .create ()
* .findFluentSetters()
* .build();
*
* // Parse into bean using fluent setter.
* MyBean bean = parser .parse("{foo:'bar'}" , MyBean.class );
*
*
* Notes:
* - The {@link Beanp @Beanp} annotation can also be used on methods to individually identify them as fluent setters.
*
- The {@link Bean#findFluentSetters() @Bean.fluentSetters()} annotation can also be used on classes to specify to look for fluent setters.
*
*
* See Also:
* - {@link Bean#findFluentSetters()}
*
- {@link BeanConfig#findFluentSetters()}
*
- {@link BeanContext.Builder#findFluentSetters()}
*
*
* @return This object.
*/
@FluentSetter
public Builder findFluentSetters() {
bcBuilder.findFluentSetters();
return this;
}
/**
* Find fluent setters.
*
*
* Identical to {@link #findFluentSetters()} but enables it on a specific class only.
*
*
Example:
*
* // A bean with a fluent setter.
* public class MyBean {
* public MyBean foo(String value ) {...}
* }
*
* // Create a parser that finds fluent setters.
* ReaderParser parser = JsonParser
* .create ()
* .findFluentSetters(MyBean.class )
* .build();
*
* // Parse into bean using fluent setter.
* MyBean bean = parser .parse("{foo:'bar'}" , MyBean.class );
*
*
* Notes:
* - This method is functionally equivalent to using the {@link Bean#findFluentSetters()} annotation.
*
*
* See Also:
* - {@link Bean#findFluentSetters()}
*
- {@link BeanContext.Builder#findFluentSetters()}
*
*
* @param on The class that this applies to.
* @return This object.
*/
@FluentSetter
public Builder findFluentSetters(Class> on) {
bcBuilder.findFluentSetters(on);
return this;
}
/**
* Ignore invocation errors on getters.
*
*
* When enabled, errors thrown when calling bean getter methods will silently be ignored.
* Otherwise, a {@code BeanRuntimeException} is thrown.
*
*
Example:
*
* // A bean with a property that throws an exception.
* public class MyBean {
* public String getFoo() {
* throw new RuntimeException("foo" );
* }
* }
*
* // Create a serializer that ignores bean getter exceptions.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .ingoreInvocationExceptionsOnGetters()
* .build();
*
* // Exception is ignored.
* String json = serializer .serialize(new MyBean());
*
*
* See Also:
* - {@link BeanConfig#ignoreInvocationExceptionsOnGetters()}
*
- {@link BeanContext.Builder#ignoreInvocationExceptionsOnGetters()}
*
*
* @return This object.
*/
@FluentSetter
public Builder ignoreInvocationExceptionsOnGetters() {
bcBuilder.ignoreInvocationExceptionsOnGetters();
return this;
}
/**
* Ignore invocation errors on setters.
*
*
* When enabled, errors thrown when calling bean setter methods will silently be ignored.
* Otherwise, a {@code BeanRuntimeException} is thrown.
*
*
Example:
*
* // A bean with a property that throws an exception.
* public class MyBean {
* public void setFoo(String foo ) {
* throw new RuntimeException("foo" );
* }
* }
*
* // Create a parser that ignores bean setter exceptions.
* ReaderParser parser = JsonParser
* .create ()
* .ignoreInvocationExceptionsOnSetters()
* .build();
*
* // Exception is ignored.
* MyBean bean = parser .parse("{foo:'bar'}" , MyBean.class );
*
*
* See Also:
* - {@link BeanConfig#ignoreInvocationExceptionsOnSetters()}
*
- {@link BeanContext.Builder#ignoreInvocationExceptionsOnSetters()}
*
*
* @return This object.
*/
@FluentSetter
public Builder ignoreInvocationExceptionsOnSetters() {
bcBuilder.ignoreInvocationExceptionsOnSetters();
return this;
}
/**
* Don't silently ignore missing setters.
*
*
* When enabled, trying to set a value on a bean property without a setter will throw a {@link BeanRuntimeException}.
* Otherwise, it will be silently ignored.
*
*
Example:
*
* // A bean with a property with a getter but not a setter.
* public class MyBean {
* public void getFoo() {
* return "foo" ;
* }
* }
*
* // Create a parser that throws an exception if a setter is not found but a getter is.
* ReaderParser parser = JsonParser
* .create ()
* .disableIgnoreMissingSetters()
* .build();
*
* // Throws a ParseException.
* MyBean bean = parser .parse("{foo:'bar'}" , MyBean.class );
*
*
* Notes:
* - The {@link BeanIgnore @BeanIgnore} annotation can also be used on getters and fields to ignore them.
*
*
* See Also:
* - {@link BeanConfig#disableIgnoreMissingSetters()}
*
- {@link BeanContext.Builder#disableIgnoreMissingSetters()}
*
*
* @return This object.
*/
@FluentSetter
public Builder disableIgnoreMissingSetters() {
bcBuilder.disableIgnoreMissingSetters();
return this;
}
/**
* Don't ignore transient fields.
*
*
* When enabled, methods and fields marked as transient will not be ignored as bean properties.
*
*
Example:
*
* // A bean with a transient field.
* public class MyBean {
* public transient String foo = "foo" ;
* }
*
* // Create a serializer that doesn't ignore transient fields.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .disableIgnoreTransientFields()
* .build();
*
* // Produces: {"foo":"foo"}
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
* - The {@link Beanp @Beanp} annotation can also be used on transient fields to keep them from being ignored.
*
*
* See Also:
* - {@link BeanConfig#disableIgnoreTransientFields()}
*
- {@link BeanContext.Builder#disableIgnoreTransientFields()}
*
*
* @return This object.
*/
@FluentSetter
public Builder disableIgnoreTransientFields() {
bcBuilder.disableIgnoreTransientFields();
return this;
}
/**
* Ignore unknown properties.
*
*
* When enabled, trying to set a value on a non-existent bean property will silently be ignored.
* Otherwise, a {@code BeanRuntimeException} is thrown.
*
*
Example:
*
* // A bean with a single property.
* public class MyBean {
* public String foo ;
* }
*
* // Create a parser that ignores missing bean properties.
* ReaderParser parser = JsonParser
* .create ()
* .ignoreUnknownBeanProperties()
* .build();
*
* // Doesn't throw an exception on unknown 'bar' property.
* MyBean bean = parser .parse("{foo:'foo',bar:'bar'}" , MyBean.class );
*
*
* See Also:
* - {@link BeanConfig#ignoreUnknownBeanProperties()}
*
- {@link BeanContext.Builder#ignoreUnknownBeanProperties()}
*
*
* @return This object.
*/
@FluentSetter
public Builder ignoreUnknownBeanProperties() {
bcBuilder.ignoreUnknownBeanProperties();
return this;
}
/**
* Ignore unknown enum values.
*
*
* When enabled, unknown enum values are set to null instead of throwing a parse exception.
*
*
See Also:
* - {@link BeanConfig#ignoreUnknownEnumValues()}
*
- {@link BeanContext.Builder#ignoreUnknownEnumValues()}
*
*
* @return This object.
*/
@FluentSetter
public Builder ignoreUnknownEnumValues() {
bcBuilder.ignoreUnknownEnumValues();
return this;
}
/**
* Don't ignore unknown properties with null values.
*
*
* When enabled, trying to set a null value on a non-existent bean property will throw a {@link BeanRuntimeException}.
* Otherwise it will be silently ignored.
*
*
Example:
*
* // A bean with a single property.
* public class MyBean {
* public String foo ;
* }
*
* // Create a parser that throws an exception on an unknown property even if the value being set is null.
* ReaderParser parser = JsonParser
* .create ()
* .disableIgnoreUnknownNullBeanProperties()
* .build();
*
* // Throws a BeanRuntimeException wrapped in a ParseException on the unknown 'bar' property.
* MyBean bean = parser .parse("{foo:'foo',bar:null}" , MyBean.class );
*
*
* See Also:
* - {@link BeanConfig#disableIgnoreUnknownNullBeanProperties()}
*
- {@link BeanContext.Builder#disableIgnoreUnknownNullBeanProperties()}
*
*
* @return This object.
*/
@FluentSetter
public Builder disableIgnoreUnknownNullBeanProperties() {
bcBuilder.disableIgnoreUnknownNullBeanProperties();
return this;
}
/**
* Implementation classes.
*
*
* For interfaces and abstract classes this method can be used to specify an implementation class for the
* interface/abstract class so that instances of the implementation class are used when instantiated (e.g. during a
* parse).
*
*
Example:
*
* // A bean interface.
* public interface MyBean {
* ...
* }
*
* // A bean implementation.
* public class MyBeanImpl implements MyBean {
* ...
* }
* // Create a parser that instantiates MyBeanImpls when parsing MyBeans.
* ReaderParser parser = JsonParser
* .create ()
* .implClass(MyBean.class , MyBeanImpl.class )
* .build();
*
* // Instantiates a MyBeanImpl,
* MyBean bean = parser .parse("..." , MyBean.class );
*
*
* @param interfaceClass The interface class.
* @param implClass The implementation class.
* @return This object.
*/
@FluentSetter
public Builder implClass(Class> interfaceClass, Class> implClass) {
bcBuilder.implClass(interfaceClass, implClass);
return this;
}
/**
* Implementation classes.
*
*
* For interfaces and abstract classes this method can be used to specify an implementation class for the
* interface/abstract class so that instances of the implementation class are used when instantiated (e.g. during a
* parse).
*
*
Example:
*
* // A bean with a single property.
* public interface MyBean {
* ...
* }
*
* // A bean with a single property.
* public class MyBeanImpl implements MyBean {
* ...
* }
* // Create a parser that instantiates MyBeanImpls when parsing MyBeans.
* ReaderParser parser = JsonParser
* .create ()
* .implClasses(AMap.of (MyBean.class , MyBeanImpl.class ))
* .build();
*
* // Instantiates a MyBeanImpl,
* MyBean bean = parser .parse("..." , MyBean.class );
*
*
* @param values
* The new value for this setting.
* @return This object.
*/
@FluentSetter
public Builder implClasses(Map,Class>> values) {
bcBuilder.implClasses(values);
return this;
}
/**
* Identifies a class to be used as the interface class for the specified class and all subclasses.
*
*
* When specified, only the list of properties defined on the interface class will be used during serialization.
* Additional properties on subclasses will be ignored.
*
*
* // Parent class or interface
* public abstract class A {
* public String foo = "foo" ;
* }
*
* // Sub class
* public class A1 extends A {
* public String bar = "bar" ;
* }
*
* // Create a serializer and define our interface class mapping.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .interfaceClass(A1.class , A.class )
* .build();
*
* // Produces "{"foo":"foo"}"
* String json = serializer .serialize(new A1());
*
*
*
* This annotation can be used on the parent class so that it filters to all child classes, or can be set
* individually on the child classes.
*
*
Notes:
* - The {@link Bean#interfaceClass() @Bean(interfaceClass)} annotation is the equivalent annotation-based solution.
*
*
* @param on The class that the interface class applies to.
* @param value
* The new value for this setting.
* @return This object.
*/
@FluentSetter
public Builder interfaceClass(Class> on, Class> value) {
bcBuilder.interfaceClass(on, value);
return this;
}
/**
* Identifies a set of interfaces.
*
*
* When specified, only the list of properties defined on the interface class will be used during serialization
* of implementation classes. Additional properties on subclasses will be ignored.
*
*
* // Parent class or interface
* public abstract class A {
* public String foo = "foo" ;
* }
*
* // Sub class
* public class A1 extends A {
* public String bar = "bar" ;
* }
*
* // Create a serializer and define our interface class mapping.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .interfaces(A.class )
* .build();
*
* // Produces "{"foo":"foo"}"
* String json = serializer .serialize(new A1());
*
*
*
* This annotation can be used on the parent class so that it filters to all child classes, or can be set
* individually on the child classes.
*
*
Notes:
* - The {@link Bean#interfaceClass() @Bean(interfaceClass)} annotation is the equivalent annotation-based solution.
*
*
* @param value
* The new value for this setting.
* @return This object.
*/
@FluentSetter
public Builder interfaces(Class>...value) {
bcBuilder.interfaces(value);
return this;
}
/**
* Context configuration property: Locale.
*
*
* Specifies the default locale for serializer and parser sessions when not specified via {@link BeanSession.Builder#locale(Locale)}.
* Typically used for POJO swaps that need to deal with locales such as swaps that convert Date and Calendar
* objects to strings by accessing it via the session passed into the {@link ObjectSwap#swap(BeanSession, Object)} and
* {@link ObjectSwap#unswap(BeanSession, Object, ClassMeta, String)} methods.
*
*
Example:
*
* // Define a POJO swap that skips serializing beans if we're in the UK.
* public class MyBeanSwap extends StringSwap<MyBean> {
* @Override
* public String swap(BeanSession session , MyBean bean ) throws Exception {
* if (session .getLocale().equals(Locale.UK ))
* return null ;
* return bean .toString();
* }
* }
*
* // Create a serializer that uses the specified locale if it's not passed in through session args.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .locale(Locale.UK )
* .swaps(MyBeanSwap.class )
* .build();
*
*
* See Also:
* - {@link BeanConfig#locale()}
*
- {@link BeanContext.Builder#locale(Locale)}
*
- {@link BeanSession.Builder#locale(Locale)}
*
*
* @param value The new value for this property.
* @return This object.
*/
@FluentSetter
public Builder locale(Locale value) {
bcBuilder.locale(value);
return this;
}
/**
* Context configuration property: Media type.
*
*
* Specifies the default media type for serializer and parser sessions when not specified via {@link BeanSession.Builder#mediaType(MediaType)}.
* Typically used for POJO swaps that need to serialize the same POJO classes differently depending on
* the specific requested media type. For example, a swap could handle a request for media types "application/json"
* and "application/json+foo" slightly differently even though they're both being handled by the same JSON
* serializer or parser.
*
*
Example:
*
* // Define a POJO swap that skips serializing beans if the media type is application/json.
* public class MyBeanSwap extends StringSwap<MyBean> {
* @Override
* public String swap(BeanSession session , MyBean bean ) throws Exception {
* if (session .getMediaType().equals("application/json" ))
* return null ;
* return bean .toString();
* }
* }
*
* // Create a serializer that uses the specified media type if it's not passed in through session args.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .mediaType(MediaType.JSON )
* .build();
*
*
* See Also:
* - {@link BeanConfig#mediaType()}
*
- {@link BeanContext.Builder#mediaType(MediaType)}
*
- {@link BeanSession.Builder#mediaType(MediaType)}
*
*
* @param value The new value for this property.
* @return This object.
*/
@FluentSetter
public Builder mediaType(MediaType value) {
bcBuilder.mediaType(value);
return this;
}
/**
* Bean class exclusions.
*
*
* List of classes that should not be treated as beans even if they appear to be bean-like.
* Not-bean classes are converted to Strings during serialization.
*
*
* Values can consist of any of the following types:
*
* - Classes.
*
- Arrays and collections of classes.
*
*
* Example:
*
* // A bean with a single property.
* public class MyBean {
* public String foo = "bar" ;
*
* public String toString() {
* return "baz" ;
* }
* }
*
* // Create a serializer that doesn't treat MyBean as a bean class.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .notBeanClasses(MyBean.class )
* .build();
*
* // Produces "baz" instead of {"foo":"bar"}
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
* - The {@link BeanIgnore @BeanIgnore} annotation can also be used on classes to prevent them from being recognized as beans.
*
*
* See Also:
* - {@link BeanIgnore}
*
- {@link BeanConfig#notBeanClasses()}
*
- {@link BeanContext.Builder#notBeanClasses()}
*
*
* @param values
* The values to add to this setting.
*
Values can consist of any of the following types:
*
* - Classes.
*
- Arrays and collections of classes.
*
* @return This object.
*/
@FluentSetter
public Builder notBeanClasses(Class>...values) {
bcBuilder.notBeanClasses(values);
return this;
}
/**
* Bean package exclusions.
*
*
* Used as a convenient way of defining the {@link BeanContext.Builder#notBeanClasses(Class...)} property for entire packages.
* Any classes within these packages will be serialized to strings using {@link Object#toString()}.
*
*
* Note that you can specify suffix patterns to include all subpackages.
*
*
* Values can consist of any of the following types:
*
* - Strings.
*
- Arrays and collections of strings.
*
*
* Example:
*
* // Create a serializer that ignores beans in the specified packages.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .notBeanPackages("org.apache.foo" , "org.apache.bar.*" )
* .build();
*
*
* See Also:
* - {@link BeanContext.Builder#notBeanPackages(String...)}
*
*
* @param values
* The values to add to this setting.
*
Values can consist of any of the following types:
*
* - {@link Package} objects.
*
- Strings.
*
- Arrays and collections of anything in this list.
*
* @return This object.
*/
@FluentSetter
public Builder notBeanPackages(String...values) {
bcBuilder.notBeanPackages(values);
return this;
}
/**
* Bean property namer
*
*
* The class to use for calculating bean property names.
*
*
* Predefined classes:
*
* - {@link BasicPropertyNamer} - Default.
*
- {@link PropertyNamerDLC} - Dashed-lower-case names.
*
- {@link PropertyNamerULC} - Dashed-upper-case names.
*
*
* Example:
*
* // A bean with a single property.
* public class MyBean {
* public String fooBarBaz = "fooBarBaz" ;
* }
*
* // Create a serializer that uses Dashed-Lower-Case property names.
* // (e.g. "foo-bar-baz" instead of "fooBarBaz")
* WriterSerializer serializer = JsonSerializer
* .create ()
* .propertyNamer(PropertyNamerDLC.class )
* .build();
*
* // Produces: {"foo-bar-baz":"fooBarBaz"}
* String json = serializer .serialize(new MyBean());
*
*
* See Also:
* - {@link BeanContext.Builder#propertyNamer(Class)}
*
*
* @param value
* The new value for this setting.
*
The default is {@link BasicPropertyNamer}.
* @return This object.
*/
@FluentSetter
public Builder propertyNamer(Class extends PropertyNamer> value) {
bcBuilder.propertyNamer(value);
return this;
}
/**
* Bean property namer
*
*
* Same as {@link #propertyNamer(Class)} but allows you to specify a namer for a specific class.
*
*
Example:
*
* // A bean with a single property.
* public class MyBean {
* public String fooBarBaz = "fooBarBaz" ;
* }
*
* // Create a serializer that uses Dashed-Lower-Case property names for the MyBean class only.
* // (e.g. "foo-bar-baz" instead of "fooBarBaz")
* WriterSerializer serializer = JsonSerializer
* .create ()
* .propertyNamer(MyBean.class , PropertyNamerDLC.class )
* .build();
*
* // Produces: {"foo-bar-baz":"fooBarBaz"}
* String json = serializer .serialize(new MyBean());
*
*
* See Also:
* - {@link Bean#propertyNamer() Bean(propertyNamer)}
*
- {@link BeanContext.Builder#propertyNamer(Class)}
*
*
* @param on The class that the namer applies to.
* @param value
* The new value for this setting.
*
The default is {@link BasicPropertyNamer}.
* @return This object.
*/
@FluentSetter
public Builder propertyNamer(Class> on, Class extends PropertyNamer> value) {
bcBuilder.propertyNamer(on, value);
return this;
}
/**
* Sort bean properties.
*
*
* When enabled, all bean properties will be serialized and access in alphabetical order.
* Otherwise, the natural order of the bean properties is used which is dependent on the JVM vendor.
* On IBM JVMs, the bean properties are ordered based on their ordering in the Java file.
* On Oracle JVMs, the bean properties are not ordered (which follows the official JVM specs).
*
*
* this setting is disabled by default so that IBM JVM users don't have to use {@link Bean @Bean} annotations
* to force bean properties to be in a particular order and can just alter the order of the fields/methods
* in the Java file.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String c = "1" ;
* public String b = "2" ;
* public String a = "3" ;
* }
*
* // Create a serializer that sorts bean properties.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .sortProperties()
* .build();
*
* // Produces: {"a":"3","b":"2","c":"1"}
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
* - The {@link Bean#sort() @Bean.sort()} annotation can also be used to sort properties on just a single class.
*
*
* See Also:
* - {@link BeanContext.Builder#sortProperties()}
*
*
* @return This object.
*/
@FluentSetter
public Builder sortProperties() {
bcBuilder.sortProperties();
return this;
}
/**
* Sort bean properties.
*
*
* Same as {@link #sortProperties()} but allows you to specify individual bean classes instead of globally.
*
*
Example:
*
* // A bean with 3 properties.
* public class MyBean {
* public String c = "1" ;
* public String b = "2" ;
* public String a = "3" ;
* }
*
* // Create a serializer that sorts properties on MyBean.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .sortProperties(MyBean.class )
* .build();
*
* // Produces: {"a":"3","b":"2","c":"1"}
* String json = serializer .serialize(new MyBean());
*
*
* See Also:
* - {@link Bean#sort() Bean(sort)}
*
- {@link BeanContext.Builder#sortProperties()}
*
*
* @param on The bean classes to sort properties on.
* @return This object.
*/
@FluentSetter
public Builder sortProperties(Class>...on) {
bcBuilder.sortProperties(on);
return this;
}
/**
* Identifies a stop class for the annotated class.
*
*
* Identical in purpose to the stop class specified by {@link Introspector#getBeanInfo(Class, Class)}.
* Any properties in the stop class or in its base classes will be ignored during analysis.
*
*
* For example, in the following class hierarchy, instances of C3 will include property p3 ,
* but not p1 or p2 .
*
*
Example:
*
* public class C1 {
* public int getP1();
* }
*
* public class C2 extends C1 {
* public int getP2();
* }
*
* public class C3 extends C2 {
* public int getP3();
* }
*
* // Create a serializer specifies a stop class for C3.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .stopClass(C3.class , C2.class )
* .build();
*
* // Produces: {"p3":"..."}
* String json = serializer .serialize(new C3());
*
*
* @param on The class on which the stop class is being applied.
* @param value
* The new value for this setting.
* @return This object.
*/
@FluentSetter
public Builder stopClass(Class> on, Class> value) {
bcBuilder.stopClass(on, value);
return this;
}
/**
* Java object swaps.
*
*
* Swaps are used to "swap out" non-serializable classes with serializable equivalents during serialization,
* and "swap in" the non-serializable class during parsing.
*
*
* An example of a swap would be a Calendar object that gets swapped out for an ISO8601 string.
*
*
* Multiple swaps can be associated with a single class.
* When multiple swaps are applicable to the same class, the media type pattern defined by
* {@link ObjectSwap#forMediaTypes()} or {@link Swap#mediaTypes() @Swap(mediaTypes)} are used to come up with the best match.
*
*
* Values can consist of any of the following types:
*
* - Any subclass of {@link ObjectSwap}.
*
- Any instance of {@link ObjectSwap}.
*
- Any surrogate class. A shortcut for defining a {@link SurrogateSwap}.
*
- Any array or collection of the objects above.
*
*
* Example:
*
* // Sample swap for converting Dates to ISO8601 strings.
* public class MyDateSwap extends StringSwap<Date> {
* // ISO8601 formatter.
* private DateFormat format = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ssZ" );
*
* @Override
* public String swap(BeanSession session , Date date ) {
* return format .format(date );
* }
*
* @Override
* public Date unswap(BeanSession session , String string , ClassMeta hint ) throws Exception {
* return format .parse(string );
* }
* }
*
* // Sample bean with a Date field.
* public class MyBean {
* public Date date = new Date(112, 2, 3, 4, 5, 6);
* }
*
* // Create a serializer that uses our date swap.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .swaps(MyDateSwap.class )
* .build();
*
* // Produces: {"date":"2012-03-03T04:05:06-0500"}
* String json = serializer .serialize(new MyBean());
*
* // Create a serializer that uses our date swap.
* ReaderParser parser = JsonParser
* .create ()
* .swaps(MyDateSwap.class )
* .build();
*
* // Use our parser to parse a bean.
* MyBean bean = parser .parse(json , MyBean.class );
*
*
* Notes:
* - The {@link Swap @Swap} annotation can also be used on classes to identify swaps for the class.
*
- The {@link Swap @Swap} annotation can also be used on bean methods and fields to identify swaps for values of those bean properties.
*
*
* See Also:
* - {@link BeanContext.Builder#swaps(Class...)}
*
*
* @param values
* The values to add to this setting.
*
Values can consist of any of the following types:
*
* - Any subclass of {@link ObjectSwap}.
*
- Any surrogate class. A shortcut for defining a {@link SurrogateSwap}.
*
- Any array or collection of the objects above.
*
* @return This object.
*/
@FluentSetter
public Builder swaps(Class>...values) {
bcBuilder.swaps(values);
return this;
}
/**
* A shortcut for defining a {@link FunctionalSwap}.
*
* Example:
*
* // Create a serializer that performs a custom format for Date objects.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .swap(Date.class , String.class , x -> format (x ))
* .build();
*
*
* @param The object type being swapped out.
* @param The object type being swapped in.
* @param normalClass The object type being swapped out.
* @param swappedClass The object type being swapped in.
* @param swapFunction The function to convert the object.
* @return This object.
*/
@FluentSetter
public Builder swap(Class normalClass, Class swappedClass, ThrowingFunction swapFunction) {
bcBuilder.swap(normalClass, swappedClass, swapFunction);
return this;
}
/**
* A shortcut for defining a {@link FunctionalSwap}.
*
* Example:
*
* // Create a serializer that performs a custom format for Date objects.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .swap(Date.class , String.class , x -> format (x ), x -> parse (x ))
* .build();
*
*
* @param The object type being swapped out.
* @param The object type being swapped in.
* @param normalClass The object type being swapped out.
* @param swappedClass The object type being swapped in.
* @param swapFunction The function to convert the object during serialization.
* @param unswapFunction The function to convert the object during parsing.
* @return This object.
*/
@FluentSetter
public Builder swap(Class normalClass, Class swappedClass, ThrowingFunction swapFunction, ThrowingFunction unswapFunction) {
bcBuilder.swap(normalClass, swappedClass, swapFunction, unswapFunction);
return this;
}
/**
* Context configuration property: TimeZone.
*
*
* Specifies the default time zone for serializer and parser sessions when not specified via {@link BeanSession.Builder#timeZone(TimeZone)}.
* Typically used for POJO swaps that need to deal with timezones such as swaps that convert Date and Calendar
* objects to strings by accessing it via the session passed into the {@link ObjectSwap#swap(BeanSession, Object)} and
* {@link ObjectSwap#unswap(BeanSession, Object, ClassMeta, String)} methods.
*
*
Example:
*
* // Define a POJO swap that skips serializing beans if the time zone is GMT.
* public class MyBeanSwap extends StringSwap<MyBean> {
* @Override
* public String swap(BeanSession session , MyBean bean ) throws Exception {
* if (session .getTimeZone().equals(TimeZone.GMT ))
* return null ;
* return bean .toString();
* }
* }
*
* // Create a serializer that uses GMT if the timezone is not specified in the session args.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .timeZone(TimeZone.GMT )
* .build();
*
*
* See Also:
* - {@link BeanConfig#timeZone()}
*
- {@link BeanContext.Builder#timeZone(TimeZone)}
*
- {@link BeanSession.Builder#timeZone(TimeZone)}
*
*
* @param value The new value for this property.
* @return This object.
*/
@FluentSetter
public Builder timeZone(TimeZone value) {
bcBuilder.timeZone(value);
return this;
}
/**
* An identifying name for this class.
*
*
* The name is used to identify the class type during parsing when it cannot be inferred through reflection.
* For example, if a bean property is of type Object , then the serializer will add the name to the
* output so that the class can be determined during parsing.
*
*
* It is also used to specify element names in XML.
*
*
Example:
*
* // Use _type='mybean' to identify this bean.
* public class MyBean {...}
*
* // Create a serializer and specify the type name..
* WriterSerializer serializer = JsonSerializer
* .create ()
* .typeName(MyBean.class , "mybean" )
* .build();
*
* // Produces: {"_type":"mybean",...}
* String json = serializer .serialize(new MyBean());
*
*
* Notes:
* - Equivalent to the {@link Bean#typeName() Bean(typeName)} annotation.
*
*
* See Also:
* - {@link Bean#typeName() Bean(typeName)}
*
- {@link BeanContext.Builder#beanDictionary(Class...)}
*
*
* @param on
* The class the type name is being defined on.
* @param value
* The new value for this setting.
* @return This object.
*/
@FluentSetter
public Builder typeName(Class> on, String value) {
bcBuilder.typeName(on, value);
return this;
}
/**
* Bean type property name.
*
*
* This specifies the name of the bean property used to store the dictionary name of a bean type so that the
* parser knows the data type to reconstruct.
*
*
Example:
*
* // POJOs with @Bean(name) annotations.
* @Bean (typeName="foo" )
* public class Foo {...}
* @Bean (typeName="bar" )
* public class Bar {...}
*
* // Create a serializer that uses 't' instead of '_type' for dictionary names.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .typePropertyName("t" )
* .dictionary(Foo.class , Bar.class )
* .build();
*
* // Create a serializer that uses 't' instead of '_type' for dictionary names.
* ReaderParser parser = JsonParser
* .create ()
* .typePropertyName("t" )
* .dictionary(Foo.class , Bar.class )
* .build();
*
* // A bean with a field with an indeterminate type.
* public class MyBean {
* public Object mySimpleField ;
* }
*
* // Produces "{mySimpleField:{t:'foo',...}}".
* String json = serializer .serialize(new MyBean());
*
* // Parse bean.
* MyBean bean = parser .parse(json , MyBean.class );
*
*
* See Also:
* - {@link Bean#typePropertyName()}
*
- {@link BeanConfig#typePropertyName()}
*
- {@link BeanContext.Builder#typePropertyName(String)}
*
*
* @param value
* The new value for this setting.
*
The default is "_type" .
* @return This object.
*/
@FluentSetter
public Builder typePropertyName(String value) {
bcBuilder.typePropertyName(value);
return this;
}
/**
* Bean type property name.
*
*
* Same as {@link #typePropertyName(String)} except targets a specific bean class instead of globally.
*
*
Example:
*
* // POJOs with @Bean(name) annotations.
* @Bean (typeName="foo" )
* public class Foo {...}
* @Bean (typeName="bar" )
* public class Bar {...}
*
* // A bean with a field with an indeterminate type.
* public class MyBean {
* public Object mySimpleField ;
* }
*
* // Create a serializer that uses 't' instead of '_type' for dictionary names.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .typePropertyName(MyBean.class , "t" )
* .dictionary(Foo.class , Bar.class )
* .build();
*
* // Produces "{mySimpleField:{t:'foo',...}}".
* String json = serializer .serialize(new MyBean());
*
*
* See Also:
* - {@link Bean#typePropertyName() Bean(typePropertyName)}
*
- {@link BeanContext.Builder#typePropertyName(String)}
*
*
* @param on The class the type property name applies to.
* @param value
* The new value for this setting.
*
The default is "_type" .
* @return This object.
*/
@FluentSetter
public Builder typePropertyName(Class> on, String value) {
bcBuilder.typePropertyName(on, value);
return this;
}
/**
* Use enum names.
*
*
* When enabled, enums are always serialized by name, not using {@link Object#toString()}.
*
*
Example:
*
* // Create a serializer with debug enabled.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .useEnumNames()
* .build();
*
* // Enum with overridden toString().
* // Will be serialized as ONE/TWO/THREE even though there's a toString() method.
* public enum Option {
* ONE (1),
* TWO (2),
* THREE (3);
*
* private int value ;
*
* Option(int value ) {
* this .value = value ;
* }
*
* @Override
* public String toString() {
* return String.valueOf (value );
* }
* }
*
*
* See Also:
* - {@link BeanContext.Builder#useEnumNames()}
*
*
* @return This object.
*/
@FluentSetter
public Builder useEnumNames() {
bcBuilder.useEnumNames();
return this;
}
/**
* Don't use interface proxies.
*
*
* When enabled, interfaces will be instantiated as proxy classes through the use of an
* {@link InvocationHandler} if there is no other way of instantiating them.
* Otherwise, throws a {@link BeanRuntimeException}.
*
*
See Also:
* - {@link BeanConfig#disableInterfaceProxies()}
*
- {@link BeanContext.Builder#disableInterfaceProxies()}
*
*
* @return This object.
*/
@FluentSetter
public Builder disableInterfaceProxies() {
bcBuilder.disableInterfaceProxies();
return this;
}
/**
* Use Java Introspector.
*
*
* Using the built-in Java bean introspector will not pick up fields or non-standard getters/setters.
*
Most {@link Bean @Bean} annotations will be ignored.
*
*
Example:
*
* // Create a serializer that only uses the built-in java bean introspector for finding properties.
* WriterSerializer serializer = JsonSerializer
* .create ()
* .useJavaBeanIntrospector()
* .build();
*
*
* See Also:
* - {@link BeanContext.Builder#useJavaBeanIntrospector()}
*
*
* @return This object.
*/
@FluentSetter
public Builder useJavaBeanIntrospector() {
bcBuilder.useJavaBeanIntrospector();
return this;
}
@Override /* Context.Builder */
public Builder annotations(Annotation...value) {
bcBuilder.annotations(value);
super.annotations(value);
return this;
}
@Override /* Context.Builder */
public Builder debug() {
bcBuilder.debug();
super.debug();
return this;
}
//
@Override /* GENERATED - org.apache.juneau.Context.Builder */
public Builder apply(AnnotationWorkList work) {
super.apply(work);
return this;
}
@Override /* GENERATED - org.apache.juneau.Context.Builder */
public Builder applyAnnotations(java.lang.Class>...fromClasses) {
super.applyAnnotations(fromClasses);
return this;
}
@Override /* GENERATED - org.apache.juneau.Context.Builder */
public Builder applyAnnotations(Method...fromMethods) {
super.applyAnnotations(fromMethods);
return this;
}
@Override /* GENERATED - org.apache.juneau.Context.Builder */
public Builder cache(Cache value) {
super.cache(value);
return this;
}
@Override /* GENERATED - org.apache.juneau.Context.Builder */
public Builder impl(Context value) {
super.impl(value);
return this;
}
@Override /* GENERATED - org.apache.juneau.Context.Builder */
public Builder type(Class extends org.apache.juneau.Context> value) {
super.type(value);
return this;
}
//
}
//-----------------------------------------------------------------------------------------------------------------
// Instance
//-----------------------------------------------------------------------------------------------------------------
final BeanContext beanContext;
/**
* Constructor.
*
* @param b The builder for this object.
*/
protected BeanContextable(Builder b) {
super(b);
beanContext = b.bc != null ? b.bc : b.bcBuilder.build();
}
/**
* Returns the bean context for this object.
*
* @return The bean context for this object.
*/
public BeanContext getBeanContext() {
return beanContext;
}
//-----------------------------------------------------------------------------------------------------------------
// Other methods
//-----------------------------------------------------------------------------------------------------------------
@Override /* Context */
protected JsonMap properties() {
return filteredMap("beanContext", beanContext.properties());
}
}