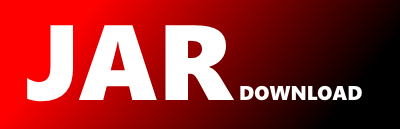
org.apache.juneau.annotation.BeanConfig Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.annotation;
import static java.lang.annotation.ElementType.*;
import static java.lang.annotation.RetentionPolicy.*;
import java.io.*;
import java.lang.annotation.*;
import java.lang.reflect.*;
import java.util.*;
import org.apache.juneau.*;
import org.apache.juneau.swap.*;
/**
* Annotation for specifying config properties defined in {@link BeanContext} and {@link BeanTraverseContext}.
*
*
* Used primarily for specifying bean configuration properties on REST classes and methods.
*
*
See Also:
*
*/
@Target({TYPE,METHOD})
@Retention(RUNTIME)
@Inherited
@ContextApply(BeanConfigAnnotation.Applier.class)
public @interface BeanConfig {
/**
* Optional rank for this config.
*
*
* Can be used to override default ordering and application of config annotations.
*
* @return The annotation value.
*/
int rank() default 0;
//-----------------------------------------------------------------------------------------------------------------
// BeanContext
//-----------------------------------------------------------------------------------------------------------------
/**
* Minimum bean class visibility.
*
*
* Classes are not considered beans unless they meet the minimum visibility requirements.
*
*
* For example, if the visibility is PUBLIC and the bean class is protected , then the class
* will not be interpreted as a bean class and be serialized as a string.
*
Use this setting to reduce the visibility requirement.
*
*
* "PUBLIC" (default)
* "PROTECTED"
* "DEFAULT"
* "PRIVATE"
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#beanClassVisibility(Visibility)}
*
*
* @return The annotation value.
*/
String beanClassVisibility() default "";
/**
* Minimum bean constructor visibility.
*
*
* Only look for constructors with the specified minimum visibility.
*
*
* This setting affects the logic for finding no-arg constructors for bean.
*
Normally, only public no-arg constructors are used.
*
Use this setting if you want to reduce the visibility requirement.
*
*
* "PUBLIC" (default)
* "PROTECTED"
* "DEFAULT"
* "PRIVATE"
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#beanConstructorVisibility(Visibility)}
*
*
* @return The annotation value.
*/
String beanConstructorVisibility() default "";
/**
* Minimum bean field visibility.
*
*
* Only look for bean fields with the specified minimum visibility.
*
*
* This affects which fields on a bean class are considered bean properties.
*
Normally only public fields are considered.
*
Use this setting if you want to reduce the visibility requirement.
*
*
* "PUBLIC" (default)
* "PROTECTED"
* "DEFAULT"
* "PRIVATE"
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#beanFieldVisibility(Visibility)}
*
*
* @return The annotation value.
*/
String beanFieldVisibility() default "";
/**
* BeanMap.put() returns old property value.
*
*
* If "true" , then the {@link BeanMap#put(String,Object) BeanMap.put()} method will return old property
* values.
*
Otherwise, it returns null .
*
*
* "true"
* "false" (default because it introduces a slight performance penalty during serialization)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#beanMapPutReturnsOldValue()}
*
*
* @return The annotation value.
*/
String beanMapPutReturnsOldValue() default "";
/**
* Minimum bean method visibility.
*
*
* Only look for bean methods with the specified minimum visibility.
*
*
* This affects which methods are detected as getters and setters on a bean class.
*
Normally only public getters and setters are considered.
*
Use this setting if you want to reduce the visibility requirement.
*
*
* "PUBLIC" (default)
* "PROTECTED"
* "DEFAULT"
* "PRIVATE"
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#beanMethodVisibility(Visibility)}
*
*
* @return The annotation value.
*/
String beanMethodVisibility() default "";
/**
* Beans require no-arg constructors.
*
*
* If "true" , a Java class must implement a default no-arg constructor to be considered a bean.
*
Otherwise, the bean will be serialized as a string using the {@link Object#toString()} method.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
* -
* The {@link Bean @Bean} annotation can be used on a class to override this setting when
"true" .
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#beansRequireDefaultConstructor()}
*
*
* @return The annotation value.
*/
String beansRequireDefaultConstructor() default "";
/**
* Beans require Serializable interface.
*
*
* If "true" , a Java class must implement the {@link Serializable} interface to be considered a bean.
*
Otherwise, the bean will be serialized as a string using the {@link Object#toString()} method.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
* -
* The {@link Bean @Bean} annotation can be used on a class to override this setting when
"true" .
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#beansRequireSerializable()}
*
*
* @return The annotation value.
*/
String beansRequireSerializable() default "";
/**
* Beans require setters for getters.
*
*
* If "true" , only getters that have equivalent setters will be considered as properties on a bean.
*
Otherwise, they will be ignored.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#beansRequireSettersForGetters()}
*
*
* @return The annotation value.
*/
String beansRequireSettersForGetters() default "";
/**
* Beans don't require at least one property.
*
*
* If "true" , then a Java class doesn't need to contain at least 1 property to be considered a bean.
*
Otherwise, the bean will be serialized as a string using the {@link Object#toString()} method.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#disableBeansRequireSomeProperties()}
*
*
* @return The annotation value.
*/
String disableBeansRequireSomeProperties() default "";
/**
* Bean type property name.
*
*
* This specifies the name of the bean property used to store the dictionary name of a bean type so that the
* parser knows the data type to reconstruct.
*
*
Notes:
* -
* Default value:
"_type" .
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
* See Also:
* - {@link Bean#typePropertyName()}
*
- {@link org.apache.juneau.BeanContext.Builder#typePropertyName(String)}
*
*
* @return The annotation value.
*/
String typePropertyName() default "";
/**
* Debug mode.
*
*
* Enables the following additional information during serialization:
*
* -
* When bean getters throws exceptions, the exception includes the object stack information
* in order to determine how that method was invoked.
*
-
* Enables {@link org.apache.juneau.BeanTraverseContext.Builder#detectRecursions()}.
*
*
*
* Enables the following additional information during parsing:
*
* -
* When bean setters throws exceptions, the exception includes the object stack information
* in order to determine how that method was invoked.
*
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.Context.Builder#debug()}
*
*
* @return The annotation value.
*/
String debug() default "";
/**
* Bean dictionary.
*
*
* The list of classes that make up the bean dictionary in this bean context.
*
*
* A dictionary is a name/class mapping used to find class types during parsing when they cannot be inferred
* through reflection.
*
The names are defined through the {@link Bean#typeName() @Bean(typeName)} annotation defined on the bean class.
*
For example, if a class Foo has a type-name of "myfoo" , then it would end up serialized
* as "{_type:'myfoo',...}" .
*
*
* This setting tells the parsers which classes to look for when resolving "_type" attributes.
*
*
See Also:
* - {@link Bean#dictionary()}
*
- {@link Beanp#dictionary()}
*
- {@link BeanConfig#dictionary_replace()}
*
- {@link org.apache.juneau.BeanContext.Builder#beanDictionary(Class...)}
*
- Bean Names and Dictionaries
*
*
* @return The annotation value.
*/
Class>[] dictionary() default {};
/**
* Replace bean dictionary.
*
*
* Same as {@link #dictionary()} but replaces any existing value.
*
*
See Also:
* - {@link Bean#dictionary()}
*
- {@link Beanp#dictionary()}
*
- {@link BeanConfig#dictionary()}
*
- {@link org.apache.juneau.BeanContext.Builder#beanDictionary(Class...)}
*
*
* @return The annotation value.
*/
Class>[] dictionary_replace() default {};
/**
* Find fluent setters.
*
*
* When enabled, fluent setters are detected on beans.
*
*
* Fluent setters must have the following attributes:
*
* - Public.
*
- Not static.
*
- Take in one parameter.
*
- Return the bean itself.
*
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link Bean#findFluentSetters()}
*
- {@link org.apache.juneau.BeanContext.Builder#findFluentSetters()}
*
*
* @return The annotation value.
*/
String findFluentSetters() default "";
/**
* Ignore invocation errors on getters.
*
*
* If "true" , errors thrown when calling bean getter methods will silently be ignored.
*
Otherwise, a {@code BeanRuntimeException} is thrown.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#ignoreInvocationExceptionsOnGetters()}
*
*
* @return The annotation value.
*/
String ignoreInvocationExceptionsOnGetters() default "";
/**
* Ignore invocation errors on setters.
*
*
* If "true" , errors thrown when calling bean setter methods will silently be ignored.
*
Otherwise, a {@code BeanRuntimeException} is thrown.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#ignoreInvocationExceptionsOnSetters()}
*
*
* @return The annotation value.
*/
String ignoreInvocationExceptionsOnSetters() default "";
/**
* Don't silently ignore missing setters.
*
*
* If "true" , trying to set a value on a bean property without a setter will throw a {@code BeanRuntimeException}.
*
Otherwise it will be sliently ignored.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#disableIgnoreMissingSetters()}
*
*
* @return The annotation value.
*/
String disableIgnoreMissingSetters() default "";
/**
* Don't ignore transient fields.
*
*
* If true , methods and fields marked as transient will not be ignored as bean properties.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#disableIgnoreTransientFields()}
*
*
* @return The annotation value.
*/
String disableIgnoreTransientFields() default "";
/**
* Ignore unknown properties.
*
*
* If "true" , trying to set a value on a non-existent bean property will silently be ignored.
*
Otherwise, a {@code RuntimeException} is thrown.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#ignoreUnknownBeanProperties()}
*
*
* @return The annotation value.
*/
String ignoreUnknownBeanProperties() default "";
/**
* Ignore unknown enum values.
*
*
* If "true" , unknown enum values are set to null instead of throwing an exception.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#ignoreUnknownEnumValues()}
*
*
* @return The annotation value.
*/
String ignoreUnknownEnumValues() default "";
/**
* Don't ignore unknown properties with null values.
*
*
* If "true" , trying to set a null value on a non-existent bean property will throw a {@code BeanRuntimeException}.
* Otherwise it will be silently ignored.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#disableIgnoreUnknownNullBeanProperties()}
*
*
* @return The annotation value.
*/
String disableIgnoreUnknownNullBeanProperties() default "";
/**
* Identifies a set of interfaces.
*
*
* When specified, only the list of properties defined on the interface class will be used during serialization
* of implementation classes. Additional properties on subclasses will be ignored.
*
*
* // Parent class or interface
* public abstract class A {
* public String foo = "foo" ;
* }
*
* // Sub class
* public class A1 extends A {
* public String bar = "bar" ;
* }
*
* // Apply it to a config
* @BeanConfig (
* interfaces={
* A.class
* }
* )
*
*
*
* This annotation can be used on the parent class so that it filters to all child classes, or can be set
* individually on the child classes.
*
*
Notes:
* - The {@link Bean#interfaceClass() @Bean(interfaceClass)} annotation is the equivalent annotation-based solution.
*
*
* @return The annotation value.
*/
Class>[] interfaces() default {};
/**
* Locale.
*
*
* Specifies the default locale for serializer and parser sessions.
*
*
Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanSession.Builder#locale(Locale)}
*
- {@link org.apache.juneau.BeanContext.Builder#locale(Locale)}
*
*
* @return The annotation value.
*/
String locale() default "";
/**
* Media type.
*
*
* Specifies the default media type value for serializer and parser sessions.
*
*
Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanSession.Builder#mediaType(MediaType)}
*
- {@link org.apache.juneau.BeanContext.Builder#mediaType(MediaType)}
*
*
* @return The annotation value.
*/
String mediaType() default "";
/**
* Bean class exclusions.
*
*
* List of classes that should not be treated as beans even if they appear to be bean-like.
*
Not-bean classes are converted to Strings during serialization.
*
*
Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link BeanIgnore}
*
- {@link org.apache.juneau.BeanContext.Builder#notBeanClasses(Class...)}
*
*
* @return The annotation value.
*/
Class>[] notBeanClasses() default {};
/**
* Replace classes that should not be considered beans.
*
*
* Same as {@link #notBeanClasses()} but replaces any existing value.
*
*
See Also:
* - {@link org.apache.juneau.BeanContext.Builder#notBeanClasses(Class...)}
*
*
* @return The annotation value.
*/
Class>[] notBeanClasses_replace() default {};
/**
* Bean package exclusions.
*
*
* When specified, the current list of ignore packages are appended to.
*
*
* Any classes within these packages will be serialized to strings using {@link Object#toString()}.
*
*
* Note that you can specify suffix patterns to include all subpackages.
*
*
Notes:
* -
* The default value excludes the following packages:
*
* java.lang
* java.lang.annotation
* java.lang.ref
* java.lang.reflect
* java.io
* java.net
* java.nio.*
* java.util.*
*
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#notBeanPackages(String...)}
*
*
* @return The annotation value.
*/
String[] notBeanPackages() default {};
/**
* Replace packages whose classes should not be considered beans.
*
*
* Same as {@link #notBeanPackages()} but replaces any existing value.
*
*
See Also:
* - {@link org.apache.juneau.BeanContext.Builder#notBeanPackages(String...)}
*
*
* @return The annotation value.
*/
String[] notBeanPackages_replace() default {};
/**
* Bean property namer.
*
*
* The class to use for calculating bean property names.
*
*
* Predefined classes:
*
* - {@link BasicPropertyNamer} (default)
*
- {@link PropertyNamerDLC} - Dashed-lower-case names.
*
- {@link PropertyNamerULC} - Dashed-upper-case names.
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#propertyNamer(Class)}
*
*
* @return The annotation value.
*/
Class extends PropertyNamer> propertyNamer() default PropertyNamer.Void.class;
/**
* Sort bean properties.
*
*
* When true , all bean properties will be serialized and access in alphabetical order.
*
Otherwise, the natural order of the bean properties is used which is dependent on the JVM vendor.
*
On IBM JVMs, the bean properties are ordered based on their ordering in the Java file.
*
On Oracle JVMs, the bean properties are not ordered (which follows the official JVM specs).
*
*
* This property is disabled by default so that IBM JVM users don't have to use {@link Bean @Bean} annotations
* to force bean properties to be in a particular order and can just alter the order of the fields/methods
* in the Java file.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#sortProperties()}
*
*
* @return The annotation value.
*/
String sortProperties() default "";
/**
* Java object swaps.
*
*
* Swaps are used to "swap out" non-serializable classes with serializable equivalents during serialization,
* and "swap in" the non-serializable class during parsing.
*
*
* An example of a swap would be a Calendar object that gets swapped out for an ISO8601 string.
*
*
* Multiple swaps can be associated with a single class.
*
When multiple swaps are applicable to the same class, the media type pattern defined by
* {@link ObjectSwap#forMediaTypes()} or {@link Swap#mediaTypes() @Swap(mediaTypes)} are used to come up with the best match.
*
*
See Also:
* - {@link org.apache.juneau.BeanContext.Builder#swaps(Class...)}
*
- Swaps
*
- Per-media-type Swaps
*
- One-way Swaps
*
- @Swap Annotation
*
- Auto-detected swaps
*
- Surrogate Classes
*
*
* @return The annotation value.
*/
Class>[] swaps() default {};
/**
* Replace Java object swap classes.
*
*
* Same as {@link #swaps()} but replaces any existing value.
*
*
See Also:
* - {@link org.apache.juneau.BeanContext.Builder#swaps(Class...)}
*
*
* @return The annotation value.
*/
Class>[] swaps_replace() default {};
/**
* Time zone.
*
*
* Specifies the default timezone for serializer and parser sessions.
*
*
Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanSession.Builder#timeZone(TimeZone)}
*
- {@link org.apache.juneau.BeanContext.Builder#timeZone(TimeZone)}
*
*
* @return The annotation value.
*/
String timeZone() default "";
/**
* Use enum names.
*
*
* When enabled, enums are always serialized by name, not using {@link Object#toString()}.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#useEnumNames()}
*
*
* @return The annotation value.
*/
String useEnumNames() default "";
/**
* Don't use interface proxies.
*
*
* Disables the feature where interfaces will be instantiated as proxy classes through the use of an
* {@link InvocationHandler} if there is no other way of instantiating them.
*
Setting this to "true" causes this to be a {@link BeanRuntimeException}.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#disableInterfaceProxies()}
*
*
* @return The annotation value.
*/
String disableInterfaceProxies() default "";
/**
* Use Java Introspector.
*
*
* Using the built-in Java bean introspector will not pick up fields or non-standard getters/setters.
*
Most {@link Bean @Bean} annotations will be ignored.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanContext.Builder#useJavaBeanIntrospector()}
*
*
* @return The annotation value.
*/
String useJavaBeanIntrospector() default "";
}