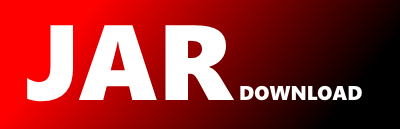
org.apache.juneau.annotation.Schema Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.annotation;
import static java.lang.annotation.ElementType.*;
import static java.lang.annotation.RetentionPolicy.*;
import java.lang.annotation.*;
import java.util.*;
import org.apache.juneau.collections.*;
import org.apache.juneau.http.annotation.*;
import org.apache.juneau.httppart.*;
import org.apache.juneau.oapi.*;
/**
* Swagger schema annotation.
*
*
* The Schema Object allows the definition of input and output data types.
* These types can be objects, but also primitives and arrays.
* This object is based on the JSON Schema Specification Draft 4 and uses a predefined subset of it.
* On top of this subset, there are extensions provided by this specification to allow for more complete documentation.
*
*
* Used to populate the auto-generated Swagger documentation and UI for server-side @Rest -annotated classes.
*
Also used to define OpenAPI schema information for POJOs serialized through {@link OpenApiSerializer} and parsed through {@link OpenApiParser}.
*
*
Examples:
*
* // A response object thats a hex-encoded string
* @Response (
* schema=@Schema (
* type="string" ,
* format="binary"
* )
* )
*
*
* // A request body consisting of an array of arrays, the internal
* // array being of type integer, numbers must be between 0 and 63 (inclusive)
* @Content (
* schema=@Schema (
* items=@Items (
* type="array" ,
* items=@SubItems (
* type="integer" ,
* minimum="0" ,
* maximum="63"
* )
* )
* )
* )
*
*
* See Also:
* - Swagger
*
- Swagger Schema Object
*
*/
@Documented
@Target({PARAMETER,METHOD,TYPE,FIELD})
@Retention(RUNTIME)
@Repeatable(SchemaAnnotation.Array.class)
@ContextApply(SchemaAnnotation.Apply.class)
public @interface Schema {
/**
* default field of the Swagger Schema Object.
*
*
* Declares the value of the parameter that the server will use if none is provided, for example a "count" to control the number of results per page might default to 100 if not supplied by the client in the request.
*
(Note: "default" has no meaning for required parameters.)
*
*
* Additionally, this value is used to create instances of POJOs that are then serialized as language-specific examples in the generated Swagger documentation
* if the examples are not defined in some other way.
*
*
* The format of this value is a string.
*
Multiple lines are concatenated with newlines.
*
*
Examples:
*
* public Order placeOrder(
* @Header ("X-PetId" )
* @Schema (_default="100" )
* long petId ,
*
* @Header ("X-AdditionalInfo" )
* @Schema (format="uon" , _default="(rushOrder=false)" )
* AdditionalInfo additionalInfo ,
*
* @Header ("X-Flags" )
* @Schema (collectionFormat="uon" , _default="@(new-customer)" )
* String[] flags
* ) {...}
*
*
* Used for:
*
* -
* Server-side schema-based parsing.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing.
*
*
* @return The annotation value.
*/
String[] _default() default {};
/**
* enum field of the Swagger Schema Object.
*
*
* If specified, the input validates successfully if it is equal to one of the elements in this array.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* The format is either individual values or a comma-delimited list.
*
Multiple lines are concatenated with newlines.
*
*
Examples:
*
* // Comma-delimited list
* public Collection<Pet> findPetsByStatus(
* @Header ("X-Status" )
* @Schema (_enum="AVAILABLE,PENDING,SOLD" )
* PetStatus status
* ) {...}
*
*
* Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
String[] _enum() default {};
/**
* $ref field of the Swagger Schema Object.
*
*
* A JSON reference to the schema definition.
*
*
Notes:
* -
* The format is a JSON Reference.
*
*
* @return The annotation value.
*/
String $ref() default "";
/**
* additionalProperties field of the Swagger Schema Object.
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
String[] additionalProperties() default {};
/**
* allOf field of the Swagger Schema Object.
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
String[] allOf() default {};
/**
* Synonym for {@link #allowEmptyValue()}.
*
* @return The annotation value.
*/
boolean aev() default false;
/**
* allowEmptyValue field of the Swagger Parameter Object.
*
*
* Sets the ability to pass empty-valued heaver values.
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
*
* Note: This is technically only valid for either query or formData parameters, but support is provided anyway for backwards compatability.
*
* @return The annotation value.
*/
boolean allowEmptyValue() default false;
/**
* Synonym for {@link #collectionFormat()}.
*
* @return The annotation value.
*/
String cf() default "";
/**
* collectionFormat field.
*
*
* Note that this field isn't part of the Swagger 2.0 specification, but the specification does not specify how
* items are supposed to be represented.
*
*
* Determines the format of the array if type "array" is used.
*
Can only be used if type is "array" .
*
*
* Static strings are defined in {@link CollectionFormatType}.
*
*
* Note that for collections/arrays parameters with POJO element types, the input is broken into a string array before being converted into POJO elements.
*
*
Used for:
*
* -
* Server-side schema-based parsing.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing.
*
*
*
* Note that for collections/arrays parameters with POJO element types, the input is broken into a string array before being converted into POJO elements.
*
*
* "csv" (default) - Comma-separated values (e.g. "foo,bar" ).
* "ssv" - Space-separated values (e.g. "foo bar" ).
* "tsv" - Tab-separated values (e.g. "foo\tbar" ).
* "pipes - Pipe-separated values (e.g. "foo|bar" ).
* "multi" - Corresponds to multiple parameter instances instead of multiple values for a single instance (e.g. "foo=bar&foo=baz" ).
* "uon" - UON notation (e.g. "@(foo,bar)" ).
*
*
* @return The annotation value.
*/
String collectionFormat() default "";
/**
* Synonym for {@link #description()}.
*
* @return The annotation value.
*/
String[] d() default {};
/**
* description field of the Swagger Schema Object.
*
*
* A brief description of the body. This could contain examples of use.
*
*
Examples:
*
* // Used on parameter
* @RestPost
* public void addPet(
* @Content @Schema (description="Pet object to add to the store" ) Pet input
* ) {...}
*
*
* // Used on class
* @RestPost
* public void addPet(Pet input ) {...}
*
* @Content @Schema (description="Pet object to add to the store" )
* public class Pet {...}
*
*
* Notes:
* -
* The format is plain text.
*
Multiple lines are concatenated with newlines.
* -
* Supports SVL Variables (e.g.
"$L{my.localized.variable}" ) for the swagger generator.
*
*
* @return The annotation value.
*/
String[] description() default {};
/**
* Synonym for {@link #_default()}.
*
* @return The annotation value.
*/
String[] df() default {};
/**
* discriminator field of the Swagger Schema Object.
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
String discriminator() default "";
/**
* Synonym for {@link #_enum()}.
*
* @return The annotation value.
*/
String[] e() default {};
/**
* Synonym for {@link #exclusiveMaximum()}.
*
* @return The annotation value.
*/
boolean emax() default false;
/**
* Synonym for {@link #exclusiveMinimum()}.
*
* @return The annotation value.
*/
boolean emin() default false;
/**
* exclusiveMaximum field of the Swagger Schema Object.
*
*
* Defines whether the maximum is matched exclusively.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* Only allowed for the following types: "integer" , "number" .
*
If true , must be accompanied with maximum .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
boolean exclusiveMaximum() default false;
/**
* exclusiveMinimum field of the Swagger Schema Object.
*
*
* Defines whether the minimum is matched exclusively.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* Only allowed for the following types: "integer" , "number" .
*
If true , must be accompanied with minimum .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
boolean exclusiveMinimum() default false;
/**
* externalDocs field of the Swagger Schema Object.
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
ExternalDocs externalDocs() default @ExternalDocs;
/**
* Synonym for {@link #format()}.
*
* @return The annotation value.
*/
String f() default "";
/**
* format field of the Swagger Schema Object.
*
*
* The extending format for the previously mentioned parameter type.
*
*
* Static strings are defined in {@link FormatType}.
*
*
Examples:
*
* // Used on parameter
* @RestPut
* public void setAge(
* @Content @Schema (type="integer" , format="int32" ) String input
* ) {...}
*
*
* Used for:
*
* -
* Server-side schema-based parsing.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing.
*
*
*
* -
*
"int32" - Signed 32 bits.
*
Only valid with type "integer" .
* -
*
"int64" - Signed 64 bits.
*
Only valid with type "integer" .
* -
*
"float" - 32-bit floating point number.
*
Only valid with type "number" .
* -
*
"double" - 64-bit floating point number.
*
Only valid with type "number" .
* -
*
"byte" - BASE-64 encoded characters.
*
Only valid with type "string" .
*
Parameters of type POJO convertible from string are converted after the string has been decoded.
* -
*
"binary" - Hexadecimal encoded octets (e.g. "00FF" ).
*
Only valid with type "string" .
*
Parameters of type POJO convertible from string are converted after the string has been decoded.
* -
*
"date" - An RFC3339 full-date.
*
Only valid with type "string" .
* -
*
"date-time" - An RFC3339 date-time.
*
Only valid with type "string" .
* -
*
"password" - Used to hint UIs the input needs to be obscured.
*
This format does not affect the serialization or parsing of the parameter.
* -
*
"uon" - UON notation (e.g. "(foo=bar,baz=@(qux,123))" ).
*
Only valid with type "object" .
*
If not specified, then the input is interpreted as plain-text and is converted to a POJO directly.
*
*
* Notes:
* -
* The format is plain text.
*
*
* See Also:
*
* @return The annotation value.
*/
String format() default "";
/**
* Specifies that schema information for this part should not be shown in the generated Swagger documentation.
*
* @return The annotation value.
*/
boolean ignore() default false;
/**
* items field of the Swagger Schema Object.
*
*
* Describes the type of items in the array.
*
*
* Required if type is "array" .
*
Can only be used if type is "array" .
*
*
Used for:
*
* -
* Server-side schema-based parsing and parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing and serializing validation.
*
*
* @return The annotation value.
*/
Items items() default @Items;
/**
* Synonym for {@link #maximum()}.
*
* @return The annotation value.
*/
String max() default "";
/**
* Synonym for {@link #maxItems()}.
*
* @return The annotation value.
*/
long maxi() default -1;
/**
* maximum field of the Swagger Schema Object.
*
*
* Defines the maximum value for a parameter of numeric types.
*
The value must be a valid JSON number.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* Only allowed for the following types: "integer" , "number" .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
String maximum() default "";
/**
* maxItems field of the Swagger Schema Object.
*
*
* An array or collection is valid if its size is less than, or equal to, the value of this keyword.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* Only allowed for the following types: "array" .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
long maxItems() default -1;
/**
* Synonym for {@link #maxLength()}.
*
* @return The annotation value.
*/
long maxl() default -1;
/**
* maxLength field of the Swagger Schema Object.
*
*
* A string instance is valid against this keyword if its length is less than, or equal to, the value of this keyword.
*
The length of a string instance is defined as the number of its characters as defined by RFC 4627.
*
The value -1 is always ignored.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* Only allowed for the following types: "string" .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
long maxLength() default -1;
/**
* Synonym for {@link #maxProperties()}.
*
* @return The annotation value.
*/
long maxp() default -1;
/**
* maxProperties field of the Swagger Schema Object.
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
long maxProperties() default -1;
/**
* Synonym for {@link #minimum()}.
*
* @return The annotation value.
*/
String min() default "";
/**
* Synonym for {@link #minItems()}.
*
* @return The annotation value.
*/
long mini() default -1;
/**
* minimum field of the Swagger Schema Object.
*
*
* Defines the minimum value for a parameter of numeric types.
*
The value must be a valid JSON number.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* Only allowed for the following types: "integer" , "number" .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
String minimum() default "";
/**
* minItems field of the Swagger Schema Object.
*
*
* An array or collection is valid if its size is greater than, or equal to, the value of this keyword.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* Only allowed for the following types: "array" .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
long minItems() default -1;
/**
* Synonym for {@link #minLength()}.
*
* @return The annotation value.
*/
long minl() default -1;
/**
* minLength field of the Swagger Schema Object.
*
*
* A string instance is valid against this keyword if its length is greater than, or equal to, the value of this keyword.
*
The length of a string instance is defined as the number of its characters as defined by RFC 4627.
*
The value -1 is always ignored.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* Only allowed for the following types: "string" .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
long minLength() default -1;
/**
* Synonym for {@link #minProperties()}.
*
* @return The annotation value.
*/
long minp() default -1;
/**
* minProperties field of the Swagger Schema Object.
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
long minProperties() default -1;
/**
* Synonym for {@link #multipleOf()}.
*
* @return The annotation value.
*/
String mo() default "";
/**
* multipleOf field of the Swagger Schema Object.
*
*
* A numeric instance is valid if the result of the division of the instance by this keyword's value is an integer.
*
The value must be a valid JSON number.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* Only allowed for the following types: "integer" , "number" .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
String multipleOf() default "";
/**
* Dynamically apply this annotation to the specified classes/methods/fields.
*
*
* Used in conjunction with {@link org.apache.juneau.BeanContext.Builder#applyAnnotations(Class...)} to dynamically apply an annotation to an existing class/method/field.
* It is ignored when the annotation is applied directly to classes/methods/fields.
*
*
Valid patterns:
*
* - Classes:
*
* - Fully qualified:
*
* "com.foo.MyClass"
*
* - Fully qualified inner class:
*
* "com.foo.MyClass$Inner1$Inner2"
*
* - Simple:
*
* "MyClass"
*
* - Simple inner:
*
* "MyClass$Inner1$Inner2"
* "Inner1$Inner2"
* "Inner2"
*
*
* - Methods:
*
* - Fully qualified with args:
*
* "com.foo.MyClass.myMethod(String,int)"
* "com.foo.MyClass.myMethod(java.lang.String,int)"
* "com.foo.MyClass.myMethod()"
*
* - Fully qualified:
*
* "com.foo.MyClass.myMethod"
*
* - Simple with args:
*
* "MyClass.myMethod(String,int)"
* "MyClass.myMethod(java.lang.String,int)"
* "MyClass.myMethod()"
*
* - Simple:
*
* "MyClass.myMethod"
*
* - Simple inner class:
*
* "MyClass$Inner1$Inner2.myMethod"
* "Inner1$Inner2.myMethod"
* "Inner2.myMethod"
*
*
* - Fields:
*
* - Fully qualified:
*
* "com.foo.MyClass.myField"
*
* - Simple:
*
* "MyClass.myField"
*
* - Simple inner class:
*
* "MyClass$Inner1$Inner2.myField"
* "Inner1$Inner2.myField"
* "Inner2.myField"
*
*
* - A comma-delimited list of anything on this list.
*
*
* See Also:
*
* @return The annotation value.
*/
String[] on() default {};
/**
* Dynamically apply this annotation to the specified classes.
*
*
* Identical to {@link #on()} except allows you to specify class objects instead of a strings.
*
*
See Also:
*
* @return The annotation value.
*/
Class>[] onClass() default {};
/**
* Synonym for {@link #pattern()}.
*
* @return The annotation value.
*/
String p() default "";
/**
* pattern field of the Swagger Schema Object.
*
*
* A string input is valid if it matches the specified regular expression pattern.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
Example:
*
* @RestPut
* public void doPut(@Content @Schema (pattern="/\\w+\\.\\d+/" ) String input ) {...}
*
*
*
* Only allowed for the following types: "string" .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
String pattern() default "";
/**
* properties field of the Swagger Schema Object.
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
String[] properties() default {};
/**
* Synonym for {@link #required()}.
*
* @return The annotation value.
*/
boolean r() default false;
/**
* readOnly field of the Swagger Schema Object.
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
boolean readOnly() default false;
/**
* required field of the Swagger Schema Object.
*
*
* Determines whether the parameter is mandatory.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
Examples:
*
* // Used on parameter
* @RestPost
* public void addPet(
* @Content @Schema (required=true ) Pet input
* ) {...}
*
*
* // Used on class
* @RestPost
* public void addPet(Pet input ) {...}
*
* @Content (required=true )
* public class Pet {...}
*
*
* Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
boolean required() default false;
/**
* Synonym for {@link #readOnly()}.
*
* @return The annotation value.
*/
boolean ro() default false;
/**
* Synonym for {@link #skipIfEmpty()}.
*
* @return The annotation value.
*/
boolean sie() default false;
/**
* Skips this value during serialization if it's an empty string or empty collection/array.
*
*
* Note that null values are already ignored.
*
*
Used for:
*
* -
* Client-side schema-based serializing.
*
*
* @return The annotation value.
*/
boolean skipIfEmpty() default false;
/**
* Synonym for {@link #type()}.
*
* @return The annotation value.
*/
String t() default "";
/**
* title field of the Swagger Schema Object.
*
* Notes:
* -
* The format is plain text.
*
*
* @return The annotation value.
*/
String title() default "";
/**
* type field of the Swagger Schema Object.
*
*
* The type of the parameter.
*
*
Examples:
*
* // Used on parameter
* @RestPost
* public void addPet(
* @Content @Schema (type="object" ) Pet input
* ) {...}
*
*
* // Used on class
* @RestPost
* public void addPet(Pet input ) {...}
*
* @Content @Schema (type="object" )
* public class Pet {...}
*
*
* Used for:
*
* -
* Server-side schema-based parsing.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing.
*
*
*
* -
*
"string"
*
Parameter must be a string or a POJO convertible from a string.
* -
*
"number"
*
Parameter must be a number primitive or number object.
*
If parameter is Object , creates either a Float or Double depending on the size of the number.
* -
*
"integer"
*
Parameter must be a integer/long primitive or integer/long object.
*
If parameter is Object , creates either a Short , Integer , or Long depending on the size of the number.
* -
*
"boolean"
*
Parameter must be a boolean primitive or object.
* -
*
"array"
*
Parameter must be an array or collection.
*
Elements must be strings or POJOs convertible from strings.
*
If parameter is Object , creates an {@link JsonList}.
* -
*
"object"
*
Parameter must be a map or bean.
*
If parameter is Object , creates an {@link JsonMap}.
*
Note that this is an extension of the OpenAPI schema as Juneau allows for arbitrarily-complex POJOs to be serialized as HTTP parts.
* -
*
"file"
*
This type is currently not supported.
*
*
* Notes:
* - Static strings are defined in {@link ParameterType}.
*
*
* See Also:
*
* @return The annotation value.
*/
String type() default "";
/**
* Synonym for {@link #uniqueItems()}.
*
* @return The annotation value.
*/
boolean ui() default false;
/**
* uniqueItems field of the Swagger Schema Object.
*
*
* If true the input validates successfully if all of its elements are unique.
*
*
* If validation fails during serialization or parsing, the part serializer/parser will throw a {@link SchemaValidationException}.
*
On the client-side, this gets converted to a RestCallException which is thrown before the connection is made.
*
On the server-side, this gets converted to a BadRequest (400).
*
*
* If the parameter type is a subclass of {@link Set}, this validation is skipped (since a set can only contain unique items anyway).
*
Otherwise, the collection or array is checked for duplicate items.
*
*
* Only allowed for the following types: "array" .
*
*
Used for:
*
* -
* Server-side schema-based parsing validation.
*
-
* Server-side generated Swagger documentation.
*
-
* Client-side schema-based serializing validation.
*
*
* @return The annotation value.
*/
boolean uniqueItems() default false;
/**
* xml field of the Swagger Schema Object.
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
String[] xml() default {};
}