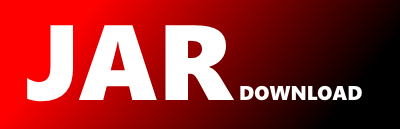
org.apache.juneau.collections.Args Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.collections;
import static org.apache.juneau.common.internal.StringUtils.*;
import static org.apache.juneau.internal.CollectionUtils.*;
import java.util.*;
import org.apache.juneau.*;
/**
* Utility class to make it easier to work with command-line arguments pass in through a
* main(String[] args) method.
*
*
* Used to parse command-line arguments of the form
* "[zero or more main arguments] [zero or more optional arguments]" .
*
*
* The format of a main argument is a token that does not start with '-' .
*
*
* The format of an optional argument is "-argName [zero or more tokens]" .
*
*
Command-line examples
*
* java com.sample.MyClass mainArg1
* java com.sample.MyClass mainArg1 mainArg2
* java com.sample.MyClass mainArg1 -optArg1
* java com.sample.MyClass -optArg1
* java com.sample.MyClass mainArg1 -optArg1 optArg1Val
* java com.sample.MyClass mainArg1 -optArg1 optArg1Val1 optArg1Val2
* java com.sample.MyClass mainArg1 -optArg1 optArg1Val1 -optArg1 optArg1Val2
*
*
* Example:
*
*
* // Main method with arguments
* public static void main (String[] _args ) {
*
* // Wrap in Args
* Args args = new Args(_args );
*
* // One main argument
* // a1
* String a1 = args .getArg(0); // "a1"
* String a2 = args .getArg(1); // null
*
* // Two main arguments
* // a1 a2
* String a1 = args .getArg(0); // "a1"
* String a2 = args .getArg(1); // "a2"
*
* // One main argument and one optional argument with no value
* // a1 -a2
* String a1 = args .getArg(0);
* boolean hasA2 = args .hasArg("a2" ); // true
* boolean hasA3 = args .hasArg("a3" ); // false
*
* // One main argument and one optional argument with one value
* // a1 -a2 v2
* String a1 = args .getArg(0);
* String a2 = args .getArg("a2" ); // "v2"
* String a3 = args .getArg("a3" ); // null
*
* // One main argument and one optional argument with two values
* // a1 -a2 v2a v2b
* String a1 = a.getArg(0);
* List<String> a2 = args .getArgs("a2" ); // Contains ["v2a","v2b"]
* List<String> a3 = args .getArgs("a3" ); // Empty list
*
* // Same as previous, except specify optional argument name multiple times
* // a1 -a2 v2a -a2 v2b
* String a1 = args .getArg(0);
* List<String> a2 = args .getArgs("a2" ); // Contains ["v2a","v2b"]
* }
*
*
*
* Main arguments are available through numeric string keys (e.g. "0" , "1" , ...).
* So you could use the {@link JsonMap} API to convert main arguments directly to POJOs, such as an Enum
*
* // Get 1st main argument as an Enum
* MyEnum _enum = args .get(MyEnum.class , "0" );
*
* // Get 1st main argument as an integer
* int _int = args .get(int .class , "0" );
*
*
*
* Equivalent operations are available on optional arguments through the {@link #getArg(Class, String)} method.
*
*
Notes:
* - This class is not thread safe.
*
*
* See Also:
*
*
* @serial exclude
*/
public final class Args extends JsonMap {
private static final long serialVersionUID = 1L;
/**
* Constructor.
*
* @param args Arguments passed in through a main(String[] args) method.
*/
public Args(String[] args) {
List argList = linkedList(args);
// Capture the main arguments.
int i = 0;
while (! argList.isEmpty()) {
String s = argList.get(0);
if (startsWith(s,'-'))
break;
put(Integer.toString(i), argList.remove(0));
i++;
}
// Capture the mapped arguments.
String key = null;
while (! argList.isEmpty()) {
String s = argList.remove(0);
if (startsWith(s, '-')) {
key = s.substring(1);
if (key.matches("\\d*"))
throw new BasicRuntimeException("Invalid optional key name ''{0}''", key);
if (! containsKey(key))
put(key, new JsonList());
} else {
((JsonList)get(key)).add(s);
}
}
}
/**
* Constructor.
*
* @param args Arguments passed in as a raw command line.
*/
public Args(String args) {
this(splitQuoted(args));
}
/**
* Returns main argument at the specified index, or null if the index is out of range.
*
*
* Can be used in conjunction with {@link #hasArg(int)} to check for existence of arg.
*
* // Check for no arguments
* if (! args .hasArg(0))
* printUsageAndExit ();
*
* // Get the first argument
* String firstArg = args .getArg(0);
*
*
*
* Since main arguments are stored as numeric keys, this method is essentially equivalent to...
*
* // Check for no arguments
* if (! args .containsKey("0" ))
* printUsageAndExit ();
*
* // Get the first argument
* String firstArg = args .getString("0" );
*
*
* @param i The index position of the main argument (zero-indexed).
* @return The main argument value, or "" if argument doesn't exist at that position.
*/
public String getArg(int i) {
return getString(Integer.toString(i));
}
/**
* Returns true if argument exists at specified index.
*
* @param i The zero-indexed position of the argument.
* @return true if argument exists at specified index.
*/
public boolean hasArg(int i) {
return containsKey(Integer.toString(i));
}
/**
* Returns true if the named argument exists.
*
* @param name The argument name.
* @return true if the named argument exists.
*/
public boolean hasArg(String name) {
JsonList l = (JsonList)get(name);
return l != null;
}
/**
* Returns the optional argument value, or blank if the optional argument was not specified.
*
*
* If the optional arg has multiple values, returns values as a comma-delimited list.
*
* @param name The optional argument name.
* @return The optional argument value, or blank if the optional argument was not specified.
*/
public String getArg(String name) {
JsonList l = (JsonList)get(name);
if (l == null || l.size() == 0)
return null;
if (l.size() == 1)
return l.get(0).toString();
return Arrays.toString(l.toArray()).replaceAll("[\\[\\]]", "");
}
/**
* Returns the optional argument value converted to the specified object type.
*
*
* If the optional arg has multiple values, returns only the first converted value.
*
*
Example:
*
* // Command: java com.sample.MyClass -verbose true -debug 5
* boolean bool = args .getArg(boolean .class , "verbose" );
* int _int = args .getArg(int .class , "debug" );
*
*
* @param c The class type to convert the value to.
* @param The class type to convert the value to.
* @param name The optional argument name.
* @return The optional argument value, or blank if the optional argument was not specified.
*/
public T getArg(Class c, String name) {
JsonList l = (JsonList)get(name);
if (l == null || l.size() == 0)
return null;
return l.get(0, c);
}
/**
* Returns the optional argument values as a list of strings.
*
* Example:
*
* // Command: java com.sample.MyClass -extraArgs foo bar baz
* List<String> list1 = args .getArgs("extraArgs" ); // ['foo','bar','baz']
* List<String> list2 = args .getArgs("nonExistentArgs" ); // An empty list
*
*
* @param name The optional argument name.
* @return The optional argument values, or an empty list if the optional argument was not specified.
*/
@SuppressWarnings({"rawtypes", "unchecked"})
public List getArgs(String name) {
List l = (JsonList)get(name);
if (l == null)
return Collections.emptyList();
return l;
}
}