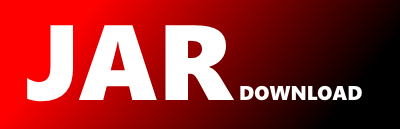
org.apache.juneau.cp.FileFinder Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.cp;
import static org.apache.juneau.common.internal.ArgUtils.*;
import static org.apache.juneau.internal.CollectionUtils.*;
import java.io.*;
import java.nio.file.*;
import java.util.*;
import java.util.regex.*;
import org.apache.juneau.*;
import org.apache.juneau.internal.*;
/**
* Utility class for finding regular or localized files on the classpath and file system.
*
* Example:
*
* // Constructor a file source that looks for files in the "files" working directory, then in the
* // package "foo.bar", then in the package "foo.bar.files", then in the package "files".
* FileFinder finder = FileFinder
* .create ()
* .dir("files" )
* .cp(foo.bar.MyClass.class ,null ,true )
* .cp(foo.bar.MyClass.class ,"files" ,true )
* .cp(foo.bar.MyClass.class ,"/files" ,true )
* .cache(1_000_000l) // Cache files less than 1MB in size.
* .ignore(Pattern.compile ("(?i)(.*\\.(class|properties))|(package.html)" )) // Ignore certain files.
* .build();
*
* // Find a normal file.
* InputStream is1 = finder .getStream("text.txt" );
*
* // Find a localized file called "text_ja_JP.txt".
* InputStream is2 = finder .getStream("text.txt" , Locale.JAPAN );
*
*
*
* If the locale is specified, then we look for resources whose name matches that locale.
* For example, if looking for the resource "MyResource.txt" for the Japanese locale, we will look for
* files in the following order:
*
* "MyResource_ja_JP.txt"
* "MyResource_ja.txt"
* "MyResource.txt"
*
*
*
* The default implementation of this interface is {@link BasicFileFinder}.
* The {@link Builder#type(Class)} method is provided for instantiating other instances.
*
*
Example:
*
* public class MyFileFinder extends BasicFileFinder {
* public MyFileFinder(FileFinder.Builder builder ) {
* super (builder );
* }
* }
*
* // Instantiate subclass.
* FileFinder myFinder = FileFinder.create ().type(MyFileFinder.class ).build();
*
*
*
* Subclasses must provide a public constructor that takes in any of the following arguments:
*
* - {@link Builder} - The builder object.
*
- Any beans present in the bean store passed into the constructor.
*
- Any {@link Optional} beans optionally present in bean store passed into the constructor.
*
*
* See Also:
*
*/
public interface FileFinder {
//-----------------------------------------------------------------------------------------------------------------
// Static
//-----------------------------------------------------------------------------------------------------------------
/** Represents no file finder */
public abstract class Void implements FileFinder {}
/**
* Static creator.
*
* @param beanStore The bean store to use for creating beans.
* @return A new builder for this object.
*/
static Builder create(BeanStore beanStore) {
return new Builder(beanStore);
}
/**
* Static creator.
*
* @return A new builder for this object.
*/
static Builder create() {
return new Builder(BeanStore.INSTANCE);
}
//-----------------------------------------------------------------------------------------------------------------
// Builder
//-----------------------------------------------------------------------------------------------------------------
/**
* Builder class.
*/
@FluentSetters
public static class Builder extends BeanBuilder {
final Set roots;
long cachingLimit;
Pattern[] include, exclude;
/**
* Constructor.
*
* @param beanStore The bean store to use for creating beans.
*/
protected Builder(BeanStore beanStore) {
super(BasicFileFinder.class, beanStore);
roots = set();
cachingLimit = -1;
include = new Pattern[]{Pattern.compile(".*")};
exclude = new Pattern[0];
}
@Override /* BeanBuilder */
protected FileFinder buildDefault() {
return new BasicFileFinder(this);
}
//-------------------------------------------------------------------------------------------------------------
// Properties
//-------------------------------------------------------------------------------------------------------------
/**
* Adds a class subpackage to the lookup paths.
*
* @param c The class whose package will be added to the lookup paths. Must not be null .
* @param path The absolute or relative subpath.
* @param recursive If true , also recursively adds all the paths of the parent classes as well.
* @return This object.
*/
@FluentSetter
public Builder cp(Class> c, String path, boolean recursive) {
assertArgNotNull("c", c);
while (c != null) {
roots.add(new LocalDir(c, path));
c = recursive ? c.getSuperclass() : null;
}
return this;
}
/**
* Adds a file system directory to the lookup paths.
*
* @param path The path relative to the working directory. Must not be null
* @return This object.
*/
@FluentSetter
public Builder dir(String path) {
assertArgNotNull("path", path);
return path(Paths.get(".").resolve(path));
}
/**
* Adds a file system directory to the lookup paths.
*
* @param path The directory path.
* @return This object.
*/
@FluentSetter
public Builder path(Path path) {
roots.add(new LocalDir(path));
return this;
}
/**
* Enables in-memory caching of files for quicker retrieval.
*
* @param cachingLimit The maximum file size in bytes.
* @return This object.
*/
@FluentSetter
public Builder caching(long cachingLimit) {
this.cachingLimit = cachingLimit;
return this;
}
/**
* Specifies the regular expression file name patterns to use to include files being retrieved from the file source.
*
* @param patterns
* The regular expression include patterns.
*
The default is ".*" .
* @return This object.
*/
@FluentSetter
public Builder include(String...patterns) {
this.include = alist(patterns).stream().map(Pattern::compile).toArray(Pattern[]::new);
return this;
}
/**
* Specifies the regular expression file name pattern to use to exclude files from being retrieved from the file source.
*
* @param patterns
* The regular expression exclude patterns.
*
If none are specified, no files will be excluded.
* @return This object.
*/
@FluentSetter
public Builder exclude(String...patterns) {
this.exclude = alist(patterns).stream().map(Pattern::compile).toArray(Pattern[]::new);
return this;
}
//
@Override /* GENERATED - org.apache.juneau.BeanBuilder */
public Builder impl(Object value) {
super.impl(value);
return this;
}
@Override /* GENERATED - org.apache.juneau.BeanBuilder */
public Builder type(Class> value) {
super.type(value);
return this;
}
//
}
//-----------------------------------------------------------------------------------------------------------------
// Instance
//-----------------------------------------------------------------------------------------------------------------
/**
* Returns the contents of the resource with the specified name.
*
* @param name The resource name.
* See {@link Class#getResource(String)} for format.
* @param locale
* The locale of the resource to retrieve.
*
If null , won't look for localized file names.
* @return The resolved resource contents, or null if the resource was not found.
* @throws IOException Thrown by underlying stream.
*/
Optional getStream(String name, Locale locale) throws IOException;
/**
* Returns the file with the specified name as a string.
*
* @param name The file name.
* @param locale
* The locale of the resource to retrieve.
*
If null , won't look for localized file names.
* @return The contents of the file as a string. Assumes UTF-8 encoding.
* @throws IOException If file could not be read.
*/
Optional getString(String name, Locale locale) throws IOException;
}