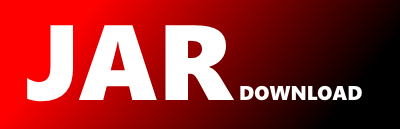
org.apache.juneau.dto.openapi3.OAuthFlows Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.dto.openapi3;
import static org.apache.juneau.internal.ConverterUtils.*;
import org.apache.juneau.annotation.Bean;
import org.apache.juneau.internal.*;
import java.util.Set;
import static org.apache.juneau.internal.CollectionUtils.*;
/**
* Describes a single operation parameter.
*
*
* A unique parameter is defined by a combination of a name and location.
*
*
* There are five possible parameter types.
*
* "path" - Used together with Path Templating, where the parameter value is actually part of the
* operation's URL.
* This does not include the host or base path of the API.
* For example, in /items/{itemId}
, the path parameter is itemId
.
* "query" - Parameters that are appended to the URL.
* For example, in /items?id=###
, the query parameter is id
.
* "header" - Custom headers that are expected as part of the request.
* "body" - The payload that's appended to the HTTP request.
* Since there can only be one payload, there can only be one body parameter.
* The name of the body parameter has no effect on the parameter itself and is used for documentation purposes
* only.
* Since Form parameters are also in the payload, body and form parameters cannot exist together for the same
* operation.
* "formData" - Used to describe the payload of an HTTP request when either
* application/x-www-form-urlencoded
, multipart/form-data
or both are used as the
* content type of the request (in Swagger's definition, the consumes property of an operation).
* This is the only parameter type that can be used to send files, thus supporting the file type.
* Since form parameters are sent in the payload, they cannot be declared together with a body parameter for the
* same operation.
* Form parameters have a different format based on the content-type used (for further details, consult
* http://www.w3.org/TR/html401/interact/forms.html#h-17.13.4
):
*
* "application/x-www-form-urlencoded" - Similar to the format of Query parameters but as a
* payload.
* For example, foo=1&bar=swagger
- both foo
and bar
are form
* parameters.
* This is normally used for simple parameters that are being transferred.
* "multipart/form-data" - each parameter takes a section in the payload with an internal header.
* For example, for the header Content-Disposition: form-data; name="submit-name"
the name of
* the parameter is submit-name
.
* This type of form parameters is more commonly used for file transfers.
*
*
*
*
* Example:
*
* // Construct using SwaggerBuilder.
* ParameterInfo x = parameterInfo ("query" , "foo" );
*
* // Serialize using JsonSerializer.
* String json = JsonSerializer.DEFAULT .toString(x);
*
* // Or just use toString() which does the same as above.
* String json = x.toString();
*
*
* // Output
* {
* "in" : "query" ,
* "name" : "foo"
* }
*
*/
@Bean(properties="implicit,password,clientCredentials,authorizationCode,*")
@FluentSetters
public class OAuthFlows extends OpenApiElement {
private OAuthFlow implicit,
password,
clientCredentials,
authorizationCode;
/**
* Default constructor.
*/
public OAuthFlows() {}
/**
* Copy constructor.
*
* @param copyFrom The object to copy.
*/
public OAuthFlows(OAuthFlows copyFrom) {
super(copyFrom);
this.implicit = copyFrom.implicit;
this.password = copyFrom.password;
this.clientCredentials = copyFrom.clientCredentials;
this.authorizationCode = copyFrom.authorizationCode;
}
/**
* Make a deep copy of this object.
*
* @return A deep copy of this object.
*/
public OAuthFlows copy() {
return new OAuthFlows(this);
}
@Override /* SwaggerElement */
protected OAuthFlows strict() {
super.strict();
return this;
}
/**
* Bean property getter: implicit .
*
*
* Describes the type of items in the array.
*
* @return The property value, or null if it is not set.
*/
public OAuthFlow getImplicit() {
return implicit;
}
/**
* Bean property setter: items .
*
*
* Describes the type of items in the array.
*
* @param value
* The new value for this property.
*
Property value is required if type
is "array" .
*
Can be null to unset the property.
* @return This object
*/
public OAuthFlows setImplicit(OAuthFlow value) {
implicit = value;
return this;
}
/**
* Bean property getter: password .
*
*
* Describes the type of items in the array.
*
* @return The property value, or null if it is not set.
*/
public OAuthFlow getPassword() {
return password;
}
/**
* Bean property setter: items .
*
*
* Describes the type of items in the array.
*
* @param value
* The new value for this property.
*
Property value is required if type
is "array" .
*
Can be null to unset the property.
* @return This object
*/
public OAuthFlows setPassword(OAuthFlow value) {
password = value;
return this;
}
/**
* Bean property getter: clientCredentials .
*
*
* Describes the type of items in the array.
*
* @return The property value, or null if it is not set.
*/
public OAuthFlow getClientCredentials() {
return clientCredentials;
}
/**
* Bean property setter: items .
*
*
* Describes the type of items in the array.
*
* @param value
* The new value for this property.
*
Property value is required if type
is "array" .
*
Can be null to unset the property.
* @return This object
*/
public OAuthFlows setClientCredentials(OAuthFlow value) {
clientCredentials = value;
return this;
}
/**
* Bean property getter: authorizationCode .
*
*
* Describes the type of items in the array.
*
* @return The property value, or null if it is not set.
*/
public OAuthFlow getAuthorizationCode() {
return authorizationCode;
}
/**
* Bean property setter: authorizationCode .
*
*
* Describes the type of items in the array.
*
* @param value
* The new value for this property.
*
Property value is required if type
is "array" .
*
Can be null to unset the property.
* @return This object
*/
public OAuthFlows setAuthorizationCode(OAuthFlow value) {
authorizationCode = value;
return this;
}
//
//
@Override /* SwaggerElement */
public T get(String property, Class type) {
if (property == null)
return null;
switch (property) {
case "implicit": return toType(getImplicit(), type);
case "password": return toType(getPassword(), type);
case "clientCredentials": return toType(getClientCredentials(), type);
case "authorizationCode": return toType(getAuthorizationCode(), type);
default: return super.get(property, type);
}
}
@Override /* SwaggerElement */
public OAuthFlows set(String property, Object value) {
if (property == null)
return this;
switch (property) {
case "implicit": return setImplicit(toType(value, OAuthFlow.class));
case "password": return setPassword(toType(value, OAuthFlow.class));
case "clientCredentials": return setClientCredentials(toType(value, OAuthFlow.class));
case "authorizationCode": return setAuthorizationCode(toType(value, OAuthFlow.class));
default:
super.set(property, value);
return this;
}
}
@Override /* SwaggerElement */
public Set keySet() {
Set s = setBuilder(String.class)
.addIf(implicit != null, "implicit")
.addIf(password != null, "password")
.addIf(clientCredentials != null, "clientCredentials")
.addIf(authorizationCode != null, "authorizationCode")
.build();
return new MultiSet<>(s, super.keySet());
}
}