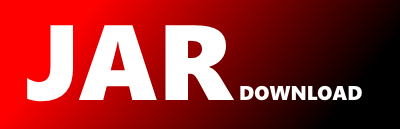
org.apache.juneau.dto.openapi3.SecuritySchemeInfo Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.dto.openapi3;
import static org.apache.juneau.internal.ConverterUtils.*;
import org.apache.juneau.*;
import org.apache.juneau.annotation.Bean;
import org.apache.juneau.internal.*;
import java.util.*;
import static org.apache.juneau.common.internal.StringUtils.*;
import static org.apache.juneau.internal.ArrayUtils.contains;
import static org.apache.juneau.internal.CollectionUtils.*;
/**
* Describes a single operation parameter.
*
*
* A unique parameter is defined by a combination of a name and location.
*
*
* There are five possible parameter types.
*
* "path" - Used together with Path Templating, where the parameter value is actually part of the
* operation's URL.
* This does not include the host or base path of the API.
* For example, in /items/{itemId}
, the path parameter is itemId
.
* "query" - Parameters that are appended to the URL.
* For example, in /items?id=###
, the query parameter is id
.
* "header" - Custom headers that are expected as part of the request.
* "body" - The payload that's appended to the HTTP request.
* Since there can only be one payload, there can only be one body parameter.
* The name of the body parameter has no effect on the parameter itself and is used for documentation purposes
* only.
* Since Form parameters are also in the payload, body and form parameters cannot exist together for the same
* operation.
* "formData" - Used to describe the payload of an HTTP request when either
* application/x-www-form-urlencoded
, multipart/form-data
or both are used as the
* content type of the request (in Swagger's definition, the consumes property of an operation).
* This is the only parameter type that can be used to send files, thus supporting the file type.
* Since form parameters are sent in the payload, they cannot be declared together with a body parameter for the
* same operation.
* Form parameters have a different format based on the content-type used (for further details, consult
* http://www.w3.org/TR/html401/interact/forms.html#h-17.13.4
):
*
* "application/x-www-form-urlencoded" - Similar to the format of Query parameters but as a
* payload.
* For example, foo=1&bar=swagger
- both foo
and bar
are form
* parameters.
* This is normally used for simple parameters that are being transferred.
* "multipart/form-data" - each parameter takes a section in the payload with an internal header.
* For example, for the header Content-Disposition: form-data; name="submit-name"
the name of
* the parameter is submit-name
.
* This type of form parameters is more commonly used for file transfers.
*
*
*
*
* Example:
*
* // Construct using SwaggerBuilder.
* ParameterInfo x = parameterInfo ("query" , "foo" );
*
* // Serialize using JsonSerializer.
* String json = JsonSerializer.DEFAULT .toString(x);
*
* // Or just use toString() which does the same as above.
* String json = x.toString();
*
*
* // Output
* {
* "in" : "query" ,
* "name" : "foo"
* }
*
*/
@Bean(properties="in,name,type,description,scheme,bearerFormat,flows,*")
@FluentSetters
public class SecuritySchemeInfo extends OpenApiElement {
private static final String[] VALID_IN = {"query", "header", "cookie"};
private static final String[] VALID_TYPES = {"apiKey", "http", "oauth2", "openIdConnect"};
private String
type,
description,
name,
in,
scheme,
bearerFormat,
openIdConnectUrl;
private OAuthFlow flows;
/**
* Default constructor.
*/
public SecuritySchemeInfo() {}
/**
* Copy constructor.
*
* @param copyFrom The object to copy.
*/
public SecuritySchemeInfo(SecuritySchemeInfo copyFrom) {
super(copyFrom);
this.name = copyFrom.name;
this.in = copyFrom.in;
this.description = copyFrom.description;
this.type = copyFrom.type;
this.scheme = copyFrom.scheme;
this.bearerFormat = copyFrom.bearerFormat;
this.openIdConnectUrl = copyFrom.openIdConnectUrl;
this.flows = copyFrom.flows;
}
/**
* Make a deep copy of this object.
*
* @return A deep copy of this object.
*/
public SecuritySchemeInfo copy() {
return new SecuritySchemeInfo(this);
}
@Override /* SwaggerElement */
protected SecuritySchemeInfo strict() {
super.strict();
return this;
}
/**
* Bean property getter: name .
*
*
* The name of the parameter.
*
*
Notes:
*
* -
* Parameter names are case sensitive.
*
-
* If
in
is "path" , the name
field MUST correspond to the associated path segment
* from the path
field in the paths object.
* -
* For all other cases, the name corresponds to the parameter name used based on the
in
property.
*
*
* @return The property value, or null if it is not set.
*/
public String getName() {
return name;
}
/**
* Bean property setter: name .
*
*
* The name of the parameter.
*
*
Notes:
*
* -
* Parameter names are case sensitive.
*
-
* If
in
is "path" , the name
field MUST correspond to the associated path segment
* from the path
field in the paths object.
* -
* For all other cases, the name corresponds to the parameter name used based on the
in
property.
*
*
* @param value
* The new value for this property.
*
Property value is required.
* @return This object
*/
public SecuritySchemeInfo setName(String value) {
name = value;
return this;
}
/**
* Bean property getter: in .
*
*
* The location of the parameter.
*
* @return The property value, or null if it is not set.
*/
public String getIn() {
return in;
}
/**
* Bean property setter: in .
*
*
* The location of the parameter.
*
* @param value
* The new value for this property.
*
Valid values:
*
* "query"
* "header"
* "path"
* "formData"
* "body"
*
*
Property value is required.
* @return This object
*/
public SecuritySchemeInfo setIn(String value) {
if (isStrict() && ! contains(value, VALID_IN))
throw new BasicRuntimeException(
"Invalid value passed in to setIn(String). Value=''{0}'', valid values={1}",
value, VALID_IN
);
in = value;
return this;
}
/**
* Bean property getter: description .
*
*
* A brief description of the parameter.
*
This could contain examples of use.
*
* @return The property value, or null if it is not set.
*/
public String getDescription() {
return description;
}
/**
* Bean property setter: description .
*
*
* A brief description of the parameter.
*
This could contain examples of use.
*
* @param value
* The new value for this property.
*
Can be null to unset the property.
* @return This object
*/
public SecuritySchemeInfo setDescription(String value) {
description = value;
return this;
}
/**
* Bean property getter: schema .
*
*
* The schema defining the type used for the body parameter.
*
* @return The property value, or null if it is not set.
*/
public String getScheme() {
return scheme;
}
/**
* Bean property setter: schema .
*
*
* The schema defining the type used for the body parameter.
*
* @param value
* The new value for this property.
*
Property value is required.
* @return This object
*/
public SecuritySchemeInfo setScheme(String value) {
scheme = value;
return this;
}
/**
* Bean property getter: type .
*
*
* The type of the parameter.
*
* @return The property value, or null if it is not set.
*/
public String getType() {
return type;
}
/**
* Bean property setter: type .
*
*
* The type of the parameter.
*
* @param value
* The new value for this property.
*
Valid values:
*
* "string"
* "number"
* "integer"
* "boolean"
* "array"
* "file"
*
*
If type is "file" , the consumes
MUST be either "multipart/form-data" , "application/x-www-form-urlencoded"
* or both and the parameter MUST be in
"formData" .
*
Property value is required.
* @return This object
*/
public SecuritySchemeInfo setType(String value) {
if (isStrict() && ! contains(value, VALID_TYPES))
throw new BasicRuntimeException(
"Invalid value passed in to setType(String). Value=''{0}'', valid values={1}",
value, VALID_TYPES
);
type = value;
return this;
}
/**
* Bean property getter: format .
*
*
* The extending format for the previously mentioned type.
*
* @return The property value, or null if it is not set.
*/
public String getBearerFormat() {
return bearerFormat;
}
/**
* Bean property setter: format .
*
*
* The extending format for the previously mentioned type.
*
* @param value The new value for this property.
* @return This object
*/
public SecuritySchemeInfo setBearerFormat(String value) {
bearerFormat = value;
return this;
}
/**
* Bean property getter: items .
*
*
* Describes the type of items in the array.
*
* @return The property value, or null if it is not set.
*/
public OAuthFlow getFlows() {
return flows;
}
/**
* Bean property setter: items .
*
*
* Describes the type of items in the array.
*
* @param value
* The new value for this property.
*
Property value is required if type
is "array" .
*
Can be null to unset the property.
* @return This object
*/
public SecuritySchemeInfo setFlows(OAuthFlow value) {
flows = value;
return this;
}
/**
* Bean property getter: collectionFormat .
*
*
* Determines the format of the array if type array is used.
*
* @return The property value, or null if it is not set.
*/
public String getOpenIdConnectUrl() {
return openIdConnectUrl;
}
/**
* Bean property setter: collectionFormat .
*
*
* Determines the format of the array if type array is used.
*
* @param value The new value for this property.
* @return This object
*/
public SecuritySchemeInfo setOpenIdConnectUrl(String value) {
openIdConnectUrl = value;
return this;
}
//
//
@Override /* SwaggerElement */
public T get(String property, Class type) {
if (property == null)
return null;
switch (property) {
case "name": return toType(getName(), type);
case "in": return toType(getIn(), type);
case "description": return toType(getDescription(), type);
case "scheme": return toType(getScheme(), type);
case "flows": return toType(getFlows(), type);
case "bearerFormat": return toType(getBearerFormat(), type);
case "openIdConnectUrl": return toType(getOpenIdConnectUrl(), type);
case "type": return toType(getType(), type);
default: return super.get(property, type);
}
}
@Override /* SwaggerElement */
public SecuritySchemeInfo set(String property, Object value) {
if (property == null)
return this;
switch (property) {
case "name": return setName(stringify(value));
case "in": return setIn(stringify(value));
case "description": return setDescription(stringify(value));
case "scheme": return setScheme(stringify(value));
case "bearerFormat": return setBearerFormat(stringify(value));
case "type": return setType(stringify(value));
case "flows": return setFlows(toType(value, OAuthFlow.class));
case "openIdConnectUrl": return setOpenIdConnectUrl(stringify(value));
default:
super.set(property, value);
return this;
}
}
@Override /* SwaggerElement */
public Set keySet() {
Set s = setBuilder(String.class)
.addIf(name != null, "name")
.addIf(in != null, "in")
.addIf(description != null, "description")
.addIf(scheme != null, "scheme")
.addIf(bearerFormat != null, "bearerFormat")
.addIf(type != null, "type")
.addIf(flows != null, "flows")
.addIf(openIdConnectUrl != null, "openIdConnectUrl")
.build();
return new MultiSet<>(s, super.keySet());
}
}