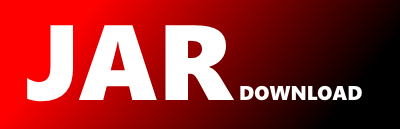
org.apache.juneau.internal.ArrayUtils Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.internal;
import static org.apache.juneau.common.internal.ArgUtils.*;
import static org.apache.juneau.common.internal.StringUtils.*;
import java.lang.reflect.*;
import java.util.*;
import org.apache.juneau.common.internal.*;
/**
* Quick and dirty utilities for working with arrays.
*
* See Also:
*
*/
public final class ArrayUtils {
/**
* Appends one or more elements to an array.
*
* @param The element type.
* @param array The array to append to.
* @param newElements The new elements to append to the array.
* @return A new array with the specified elements appended.
*/
@SuppressWarnings("unchecked")
public static T[] append(T[] array, T...newElements) {
if (array == null)
return newElements;
if (newElements.length == 0)
return array;
T[] a = (T[])Array.newInstance(array.getClass().getComponentType(), array.length + newElements.length);
for (int i = 0; i < array.length; i++)
a[i] = array[i];
for (int i = 0; i < newElements.length; i++)
a[i+array.length] = newElements[i];
return a;
}
/**
* Combine an arbitrary number of arrays into a single array.
*
* @param The element type.
* @param arrays Collection of arrays to combine.
* @return A new combined array, or null if all arrays are null .
*/
@SuppressWarnings("unchecked")
public static E[] combine(E[]...arrays) {
assertArgNotNull("arrays", arrays);
int l = 0;
E[] a1 = null;
for (E[] a : arrays) {
if (a1 == null && a != null)
a1 = a;
l += (a == null ? 0 : a.length);
}
if (a1 == null)
return null;
E[] a = (E[])Array.newInstance(a1.getClass().getComponentType(), l);
int i = 0;
for (E[] aa : arrays)
if (aa != null)
for (E t : aa)
a[i++] = t;
return a;
}
/**
* Converts the specified array to a Set .
*
*
* The order of the entries in the set are the same as the array.
*
* @param The entry type of the array.
* @param array The array being wrapped in a Set interface.
* @return The new set.
*/
public static Set asSet(final T[] array) {
assertArgNotNull("array", array);
return new AbstractSet<>() {
@Override /* Set */
public Iterator iterator() {
return new Iterator<>() {
int i = 0;
@Override /* Iterator */
public boolean hasNext() {
return i < array.length;
}
@Override /* Iterator */
public T next() {
if (i >= array.length)
throw new NoSuchElementException();
T t = array[i];
i++;
return t;
}
@Override /* Iterator */
public void remove() {
throw new UnsupportedOperationException("Not supported.");
}
};
}
@Override /* Set */
public int size() {
return array.length;
}
};
}
/**
* Converts the specified collection to an array.
*
*
* Works on both object and primitive arrays.
*
* @param The element type.
* @param c The collection to convert to an array.
* @param elementType The component type of the collection.
* @return A new array.
*/
public static Object toArray(Collection> c, Class elementType) {
Object a = Array.newInstance(elementType, c.size());
Iterator> it = c.iterator();
int i = 0;
while (it.hasNext())
Array.set(a, i++, it.next());
return a;
}
/**
* Returns true if the specified object is an array.
*
* @param array The array to test.
* @return true if the specified object is an array.
*/
public static boolean isArray(Object array) {
return array != null && array.getClass().isArray();
}
/**
* Converts the specified array to an ArrayList
*
* @param The element type.
* @param array The array to convert.
* @param elementType
* The type of objects in the array.
* It must match the actual component type in the array.
* @return A new {@link ArrayList}
*/
@SuppressWarnings("unchecked")
public static List toList(Object array, Class elementType) {
List l = new ArrayList<>(Array.getLength(array));
for (int i = 0; i < Array.getLength(array); i++)
l.add((E)Array.get(array, i));
return l;
}
/**
* Recursively converts the specified array into a list of objects.
*
* @param array The array to convert.
* @return A new {@link ArrayList}
*/
public static List