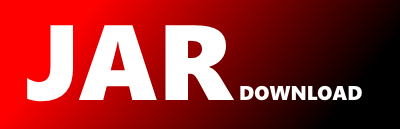
org.apache.juneau.internal.CollectionUtils Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.internal;
import java.lang.reflect.*;
import java.util.*;
import java.util.function.*;
/**
* Utility methods for collections.
*
* See Also:
*
*/
public final class CollectionUtils {
/**
* Creates a new set from the specified collection.
*
* @param The element type.
* @param val The value to copy from.
* @return A new {@link LinkedHashSet}, or null if the input was null.
*/
public static Set setFrom(Collection val) {
return val == null ? null : new LinkedHashSet<>(val);
}
/**
* Creates a new set from the specified collection.
*
* @param The element type.
* @param val The value to copy from.
* @return A new {@link LinkedHashSet}, or null if the input was null.
*/
public static Set copyOf(Set val) {
return val == null ? null : new LinkedHashSet<>(val);
}
/**
* Creates a new collection from the specified collection.
*
* @param The element type.
* @param val The value to copy from.
* @return A new {@link LinkedHashSet}, or null if the input was null.
*/
public static Collection copyOf(Collection val) {
return val == null ? null : new LinkedHashSet<>(val);
}
/**
* Creates a new map from the specified map.
*
* @param The key type.
* @param The value type.
* @param val The value to copy from.
* @return A new {@link LinkedHashMap}, or null if the input was null.
*/
public static Map copyOf(Map val) {
return val == null ? null : new LinkedHashMap<>(val);
}
/**
* Instantiates a new builder on top of the specified map.
*
* @param The key type.
* @param The value type.
* @param addTo The map to add to.
* @return A new builder on top of the specified map.
*/
public static MapBuilder mapBuilder(Map addTo) {
return new MapBuilder<>(addTo);
}
/**
* Instantiates a new builder of the specified map type.
*
* @param The key type.
* @param The value type.
* @param keyType The key type.
* @param valueType The value type.
* @param valueTypeArgs The value type args.
* @return A new builder on top of the specified map.
*/
public static MapBuilder mapBuilder(Class keyType, Class valueType, Type...valueTypeArgs) {
return new MapBuilder<>(keyType, valueType, valueTypeArgs);
}
/**
* Instantiates a new builder on top of the specified list.
*
* @param The element type.
* @param addTo The list to add to.
* @return A new builder on top of the specified list.
*/
public static ListBuilder listBuilder(List addTo) {
return new ListBuilder<>(addTo);
}
/**
* Instantiates a new builder of the specified list type.
*
* @param The element type.
* @param elementType The element type.
* @param elementTypeArgs The element type args.
* @return A new builder on top of the specified list.
*/
public static ListBuilder listBuilder(Class elementType, Type...elementTypeArgs) {
return new ListBuilder<>(elementType, elementTypeArgs);
}
/**
* Instantiates a new builder on top of the specified set.
*
* @param The element type.
* @param addTo The set to add to.
* @return A new builder on top of the specified set.
*/
public static SetBuilder setBuilder(Set addTo) {
return new SetBuilder<>(addTo);
}
/**
* Instantiates a new builder of the specified set.
*
* @param The element type.
* @param elementType The element type.
* @param elementTypeArgs The element type args.
* @return A new builder on top of the specified set.
*/
public static SetBuilder setBuilder(Class elementType, Type...elementTypeArgs) {
return new SetBuilder<>(elementType, elementTypeArgs);
}
/**
* Simple passthrough to {@link Collections#emptyList()}
*
* @param The element type.
* @return A new unmodifiable empty list.
*/
public static List emptyList() {
return Collections.emptyList();
}
/**
* Convenience method for creating an {@link ArrayList}.
*
* @param The element type.
* @param values The values to initialize the list with.
* @return A new modifiable list.
*/
@SafeVarargs
public static ArrayList list(E...values) {
ArrayList l = new ArrayList<>(values.length);
for (E v : values)
l.add(v);
return l;
}
/**
* Convenience method for creating an {@link ArrayList} of the specified size.
*
* @param The element type.
* @param size The initial size of the list.
* @return A new modifiable list.
*/
public static ArrayList list(int size) {
return new ArrayList<>(size);
}
/**
* Convenience method for creating a {@link LinkedList}.
*
* @param The element type.
* @param values The values to initialize the list with.
* @return A new modifiable list.
*/
@SafeVarargs
public static LinkedList linkedList(E...values) {
LinkedList l = new LinkedList<>();
for (E v : values)
l.add(v);
return l;
}
/**
* Convenience method for creating an array-backed list by calling {@link Arrays#asList(Object...)}.
*
* @param The element type.
* @param values The values to initialize the list with.
* @return A new modifiable list, or null if the array was null .
*/
@SafeVarargs
public static List alist(E...values) {
if (values == null)
return null;
return Arrays.asList(values);
}
/**
* Creates an {@link ArrayList} copy from a collection.
*
* @param The element type.
* @param value The collection to copy from.
* @return A new modifiable list.
*/
public static ArrayList listFrom(Collection value) {
return listFrom(value, false);
}
/**
* Creates an {@link ArrayList} copy from a collection.
*
* @param The key type.
* @param The value type.
* @param value The collection to copy from.
* @return A new modifiable list.
*/
public static LinkedHashMap mapFrom(Map value) {
if (value == null)
return null;
return new LinkedHashMap<>(value);
}
/**
* Creates an {@link ArrayList} copy from a collection.
*
* @param The element type.
* @param value The collection to copy from.
* @param nullIfEmpty If true will return null if the collection is empty.
* @return A new modifiable list.
*/
public static ArrayList listFrom(Collection value, boolean nullIfEmpty) {
if (value == null || (nullIfEmpty && value.isEmpty()))
return null;
ArrayList l = new ArrayList<>();
value.forEach(x -> l.add(x));
return l;
}
/**
* Convenience method for creating a {@link LinkedHashSet}.
*
* @param The element type.
* @param values The values to initialize the set with.
* @return A new modifiable set.
*/
@SafeVarargs
public static LinkedHashSet set(E...values) {
LinkedHashSet l = new LinkedHashSet<>();
for (E v : values)
l.add(v);
return l;
}
/**
* Convenience method for creating an unmodifiable {@link LinkedHashSet}.
*
* @param The element type.
* @param values The values to initialize the set with.
* @return A new unmodifiable set.
*/
@SafeVarargs
public static Set uset(E...values) {
return unmodifiable(set(values));
}
/**
* Convenience method for creating an unmodifiable list.
*
* @param The element type.
* @param values The values to initialize the list with.
* @return A new unmodifiable list, or null if the array was null .
*/
@SafeVarargs
public static List ulist(E...values) {
if (values == null)
return null;
return unmodifiable(alist(values));
}
/**
* Convenience method for creating a {@link TreeSet}.
*
* @param The element type.
* @param values The values to initialize the set with.
* @return A new modifiable set.
*/
@SafeVarargs
public static TreeSet sortedSet(E...values) {
TreeSet l = new TreeSet<>();
for (E v : values)
l.add(v);
return l;
}
/**
* Creates a new {@link TreeSet} from the specified collection.
*
* @param The element type.
* @param value The value to copy from.
* @return A new {@link TreeSet}, or null if the input was null.
*/
public static TreeSet sortedSetFrom(Collection value) {
if (value == null)
return null;
TreeSet l = new TreeSet<>();
value.forEach(x -> l.add(x));
return l;
}
/**
* Creates a new {@link TreeSet} from the specified collection.
*
* @param The element type.
* @param value The value to copy from.
* @param nullIfEmpty If true returns null if the collection is empty.
* @return A new {@link TreeSet}, or null if the input was null.
*/
public static TreeSet sortedSetFrom(Collection value, boolean nullIfEmpty) {
if (value == null || (nullIfEmpty && value.isEmpty()))
return null;
TreeSet l = new TreeSet<>();
value.forEach(x -> l.add(x));
return l;
}
/**
* Convenience method for creating a {@link LinkedHashMap}.
*
* @param The key type.
* @param The value type.
* @return A new modifiable map.
*/
public static LinkedHashMap map() {
LinkedHashMap m = new LinkedHashMap<>();
return m;
}
/**
* Convenience method for creating a {@link LinkedHashMap}.
*
* @param The key type.
* @param The value type.
* @param k1 Key 1.
* @param v1 Value 1.
* @return A new modifiable map.
*/
public static LinkedHashMap map(K k1, V v1) {
LinkedHashMap m = new LinkedHashMap<>();
m.put(k1, v1);
return m;
}
/**
* Convenience method for creating a {@link LinkedHashMap}.
*
* @param The key type.
* @param The value type.
* @param k1 Key 1.
* @param v1 Value 1.
* @param k2 Key 2.
* @param v2 Value 2.
* @return A new modifiable map.
*/
public static LinkedHashMap map(K k1, V v1, K k2, V v2) {
LinkedHashMap m = new LinkedHashMap<>();
m.put(k1, v1);
m.put(k2, v2);
return m;
}
/**
* Convenience method for creating a {@link LinkedHashMap}.
*
* @param The key type.
* @param The value type.
* @param k1 Key 1.
* @param v1 Value 1.
* @param k2 Key 2.
* @param v2 Value 2.
* @param k3 Key 3.
* @param v3 Value 3.
* @return A new modifiable map.
*/
public static LinkedHashMap map(K k1, V v1, K k2, V v2, K k3, V v3) {
LinkedHashMap m = new LinkedHashMap<>();
m.put(k1, v1);
m.put(k2, v2);
m.put(k3, v3);
return m;
}
/**
* Convenience method for creating a {@link TreeMap}.
*
* @param The key type.
* @param The value type.
* @return A new modifiable set.
*/
public static TreeMap sortedMap() {
return new TreeMap<>();
}
/**
* Convenience method for copying a list.
*
* @param The element type.
* @param value The list to copy.
* @return A new modifiable list.
*/
public static ArrayList copyOf(List value) {
return value == null ? null : new ArrayList<>(value);
}
/**
* Convenience method for creating an {@link ArrayList} and sorting it.
*
* @param The element type.
* @param values The values to initialize the list with.
* @return A new modifiable list.
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
@SafeVarargs
public static ArrayList sortedList(E...values) {
ArrayList l = list(values);
Collections.sort((List) l);
return l;
}
/**
* Convenience method for creating an {@link ArrayList} and sorting it.
*
* @param The element type.
* @param comparator The comparator to use to sort the list.
* @param values The values to initialize the list with.
* @return A new modifiable list.
*/
public static ArrayList sortedList(Comparator comparator, E[] values) {
ArrayList l = list(values);
Collections.sort(l, comparator);
return l;
}
/**
* Convenience method for creating an {@link ArrayList} and sorting it.
*
* @param The element type.
* @param comparator The comparator to use to sort the list.
* @param value The values to initialize the list with.
* @return A new modifiable list.
*/
public static ArrayList sortedList(Comparator comparator, Collection value) {
ArrayList l = listFrom(value);
Collections.sort(l, comparator);
return l;
}
/**
* Wraps the specified list in {@link Collections#unmodifiableList(List)}.
*
* @param The element type.
* @param value The list to wrap.
* @return The wrapped list.
*/
public static List unmodifiable(List value) {
return value == null ? null: Collections.unmodifiableList(value);
}
/**
* Wraps the specified set in {@link Collections#unmodifiableSet(Set)}.
*
* @param The element type.
* @param value The set to wrap.
* @return The wrapped set.
*/
public static Set unmodifiable(Set value) {
return value == null ? null: Collections.unmodifiableSet(value);
}
/**
* Wraps the specified map in {@link Collections#unmodifiableMap(Map)}.
*
* @param The key type.
* @param The value type.
* @param value The map to wrap.
* @return The wrapped map.
*/
public static Map unmodifiable(Map value) {
return value == null ? null: Collections.unmodifiableMap(value);
}
/**
* Wraps the specified list in {@link Collections#unmodifiableList(List)}.
*
* @param The element type.
* @param value The list to wrap.
* @return The wrapped list.
*/
public static List synced(List value) {
return value == null ? null: Collections.synchronizedList(value);
}
/**
* Wraps the specified set in {@link Collections#unmodifiableSet(Set)}.
*
* @param The element type.
* @param value The set to wrap.
* @return The wrapped set.
*/
public static Set synced(Set value) {
return value == null ? null: Collections.synchronizedSet(value);
}
/**
* Wraps the specified map in {@link Collections#unmodifiableMap(Map)}.
*
* @param The key type.
* @param The value type.
* @param value The map to wrap.
* @return The wrapped map.
*/
public static Map synced(Map value) {
return value == null ? null: Collections.synchronizedMap(value);
}
/**
* Converts the specified collection to an array.
*
* @param The element type.
* @param value The collection to convert.
* @param componentType The component type of the array.
* @return A new array.
*/
@SuppressWarnings("unchecked")
public static E[] array(Collection value, Class componentType) {
if (value == null)
return null;
E[] array = (E[])Array.newInstance(componentType, value.size());
return value.toArray(array);
}
/**
* Iterates the specified list in reverse order.
*
* @param The element type.
* @param value The list to iterate.
* @param action The action to perform.
*/
public static void forEachReverse(List value, Consumer action) {
if (value instanceof ArrayList) {
for (int i = value.size()-1; i >= 0; i--)
action.accept(value.get(i));
} else {
ListIterator i = value.listIterator(value.size());
while (i.hasPrevious())
action.accept(i.previous());
}
}
/**
* Iterates the specified array in reverse order.
*
* @param The element type.
* @param value The array to iterate.
* @param action The action to perform.
*/
public static void forEachReverse(E[] value, Consumer action) {
for (int i = value.length-1; i >= 0; i--)
action.accept(value[i]);
}
/**
* Adds all the specified values to the specified collection.
* Creates a new set if the value is null .
*
* @param The element type.
* @param value The collection to add to.
* @param entries The entries to add.
* @return The set.
*/
@SafeVarargs
public static Set addAll(Set value, E...entries) {
if (entries != null) {
if (value == null)
value = set(entries);
else
Collections.addAll(value, entries);
}
return value;
}
/**
* Adds all the specified values to the specified collection.
* Creates a new set if the value is null .
*
* @param The element type.
* @param value The collection to add to.
* @param entries The entries to add.
* @return The set.
*/
@SafeVarargs
public static SortedSet addAll(SortedSet value, E...entries) {
if (entries != null) {
if (value == null)
value = sortedSet(entries);
else
Collections.addAll(value, entries);
}
return value;
}
/**
* Adds all the specified values to the specified collection.
* Creates a new set if the value is null .
*
* @param The element type.
* @param value The collection to add to.
* @param entries The entries to add.
* @return The set.
*/
@SafeVarargs
public static List addAll(List value, E...entries) {
if (entries != null) {
if (value == null)
value = list(entries);
else
Collections.addAll(value, entries);
}
return value;
}
/**
* Adds all the specified values to the specified collection.
* Creates a new set if the value is null .
*
* @param The element type.
* @param value The collection to add to.
* @param entries The entries to add.
* @return The set.
*/
@SafeVarargs
public static List prependAll(List value, E...entries) {
if (entries != null) {
if (value == null)
value = list(entries);
else
value.addAll(0, alist(entries));
}
return value;
}
/**
* Returns the last entry in a list.
*
* @param The element type.
* @param l The list.
* @return The last element, or null if the list is null or empty.
*/
public static E last(List l) {
if (l == null || l.isEmpty())
return null;
return l.get(l.size()-1);
}
/**
* Returns the last entry in an array.
*
* @param The element type.
* @param l The array.
* @return The last element, or null if the array is null or empty.
*/
public static E last(E[] l) {
if (l == null || l.length == 0)
return null;
return l[l.length-1];
}
/**
* Returns an optional of the specified value.
*
* @param The component type.
* @param value The value.
* @return A new Optional.
*/
public static Optional optional(T value) {
return Optional.ofNullable(value);
}
/**
* Returns an empty {@link Optional}.
*
* @param The component type.
* @return An empty {@link Optional}.
*/
public static Optional empty() {
return Optional.empty();
}
/**
* Returns true if the specified collection is not null and not empty.
*
* @param The element type.
* @param value The value being checked.
* @return true if the specified collection is not null and not empty.
*/
public static boolean isNotEmpty(Collection value) {
return value != null && ! value.isEmpty();
}
/**
* Returns true if the specified map is not null and not empty.
*
* @param The key type.
* @param The value type.
* @param value The value being checked.
* @return true if the specified map is not null and not empty.
*/
public static boolean isNotEmpty(Map value) {
return value != null && ! value.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy