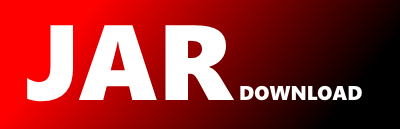
org.apache.juneau.marshaller.CharMarshaller Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.marshaller;
import java.lang.reflect.*;
import org.apache.juneau.*;
import org.apache.juneau.parser.*;
import org.apache.juneau.serializer.*;
/**
* A subclass of {@link Marshaller} for character-based serializers and parsers.
*
* See Also:
* - Marshallers
*
*/
public class CharMarshaller extends Marshaller {
//-----------------------------------------------------------------------------------------------------------------
// Instance
//-----------------------------------------------------------------------------------------------------------------
private final ReaderParser p;
private final WriterSerializer s;
/**
* Constructor.
*
* @param s
* The serializer to use for serializing output.
*
Must not be null .
* @param p
* The parser to use for parsing input.
*
Must not be null .
*/
public CharMarshaller(WriterSerializer s, ReaderParser p) {
super(s, p);
this.s = s;
this.p = p;
}
/**
* Same as {@link #read(Object,Class)} but reads from a string and thus doesn't throw an IOException .
*
*
* This is the preferred parse method for simple types since you don't need to cast the results.
*
*
Examples:
*
* Marshaller marshaller = Json.DEFAULT ;
*
* // Parse into a string.
* String string = marshaller .read(json , String.class );
*
* // Parse into a bean.
* MyBean bean = marshaller .read(json , MyBean.class );
*
* // Parse into a bean array.
* MyBean[] beanArray = marshaller .read(json , MyBean[].class );
*
* // Parse into a linked-list of objects.
* List list = marshaller .read(json , LinkedList.class );
*
* // Parse into a map of object keys/values.
* Map map = marshaller .read(json , TreeMap.class );
*
*
* @param The class type of the object being created.
* @param input The input.
* @param type The object type to create.
* @return The parsed object.
* @throws ParseException Malformed input encountered.
*/
public final T read(String input, Class type) throws ParseException {
return p.parse(input, type);
}
/**
* Same as {@link #read(Object,Type,Type...)} but reads from a string and thus doesn't throw an IOException .
*
* @param The class type of the object to create.
* @param input The input.
* @param type
* The object type to create.
*
Can be any of the following: {@link ClassMeta}, {@link Class}, {@link ParameterizedType}, {@link GenericArrayType}
* @param args
* The type arguments of the class if it's a collection or map.
*
Can be any of the following: {@link ClassMeta}, {@link Class}, {@link ParameterizedType}, {@link GenericArrayType}
*
Ignored if the main type is not a map or collection.
* @return The parsed object.
* @throws ParseException Malformed input encountered.
* @see BeanSession#getClassMeta(Type,Type...) for argument syntax for maps and collections.
*/
public final T read(String input, Type type, Type...args) throws ParseException {
return p.parse(input, type, args);
}
/**
* Serializes a POJO directly to a String .
*
* @param object The object to serialize.
* @return
* The serialized object.
* @throws SerializeException If a problem occurred trying to convert the output.
*/
public final String write(Object object) throws SerializeException {
return s.serializeToString(object);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy