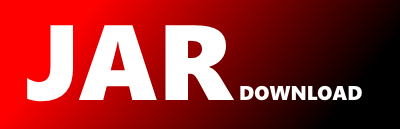
org.apache.juneau.marshaller.PlainText Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.marshaller;
import java.io.*;
import java.lang.reflect.*;
import java.nio.charset.*;
import org.apache.juneau.*;
import org.apache.juneau.parser.*;
import org.apache.juneau.plaintext.*;
import org.apache.juneau.serializer.*;
/**
* A pairing of a {@link PlainTextSerializer} and {@link PlainTextParser} into a single class with convenience read/write methods.
*
*
* The general idea is to combine a single serializer and parser inside a simplified API for reading and writing POJOs.
*
*
Examples:
*
* // Using instance.
* PlainText plainText = new PlainText();
* MyPojo myPojo = plainText .read(string , MyPojo.class );
* String string = plainText .write(myPojo );
*
*
* // Using DEFAULT instance.
* MyPojo myPojo = PlainText.DEFAULT .read(string , MyPojo.class );
* String string = PlainText.DEFAULT .write(myPojo );
*
*
* See Also:
* - Marshallers
*
*/
public class PlainText extends CharMarshaller {
//-----------------------------------------------------------------------------------------------------------------
// Static
//-----------------------------------------------------------------------------------------------------------------
/**
* Default reusable instance.
*/
public static final PlainText DEFAULT = new PlainText();
//-----------------------------------------------------------------------------------------------------------------
// Instance
//-----------------------------------------------------------------------------------------------------------------
/**
* Constructor.
*
* @param s
* The serializer to use for serializing output.
*
Must not be null .
* @param p
* The parser to use for parsing input.
*
Must not be null .
*/
public PlainText(PlainTextSerializer s, PlainTextParser p) {
super(s, p);
}
/**
* Constructor.
*
*
* Uses {@link PlainTextSerializer#DEFAULT} and {@link PlainTextParser#DEFAULT}.
*/
public PlainText() {
this(PlainTextSerializer.DEFAULT, PlainTextParser.DEFAULT);
}
/**
* Parses a Plain Text input string to the specified type.
*
*
* A shortcut for calling DEFAULT .read(input , type ) .
*
* @param The class type of the object being created.
* @param input The input.
* @param type The object type to create.
* @return The parsed object.
* @throws ParseException Malformed input encountered.
*/
public static T to(String input, Class type) throws ParseException {
return DEFAULT.read(input, type);
}
/**
* Parses a Plain Text input object to the specified Java type.
*
*
* A shortcut for calling DEFAULT .read(input , type ) .
*
* @param The class type of the object being created.
* @param input
* The input.
*
Can be any of the following types:
*
* null
* - {@link Reader}
*
- {@link CharSequence}
*
- {@link InputStream} containing UTF-8 encoded text (or charset defined by
* {@link org.apache.juneau.parser.ReaderParser.Builder#streamCharset(Charset)} property value).
*
byte []
containing UTF-8 encoded text (or charset defined by
* {@link org.apache.juneau.parser.ReaderParser.Builder#streamCharset(Charset)} property value).
* - {@link File} containing system encoded text (or charset defined by
* {@link org.apache.juneau.parser.ReaderParser.Builder#fileCharset(Charset)} property value).
*
* @param type The object type to create.
* @return The parsed object.
* @throws ParseException Malformed input encountered.
* @throws IOException Thrown by underlying stream.
*/
public static T to(Object input, Class type) throws ParseException, IOException {
return DEFAULT.read(input, type);
}
/**
* Parses a Plain Text input string to the specified Java type.
*
*
* A shortcut for calling DEFAULT .read(input , type , args ) .
*
* @param The class type of the object to create.
* @param input The input.
* @param type
* The object type to create.
*
Can be any of the following: {@link ClassMeta}, {@link Class}, {@link ParameterizedType}, {@link GenericArrayType}
* @param args
* The type arguments of the class if it's a collection or map.
*
Can be any of the following: {@link ClassMeta}, {@link Class}, {@link ParameterizedType}, {@link GenericArrayType}
*
Ignored if the main type is not a map or collection.
* @return The parsed object.
* @throws ParseException Malformed input encountered.
* @see BeanSession#getClassMeta(Type,Type...) for argument syntax for maps and collections.
*/
public static T to(String input, Type type, Type...args) throws ParseException {
return DEFAULT.read(input, type, args);
}
/**
* Parses a Plain Text input object to the specified Java type.
*
*
* A shortcut for calling DEFAULT .read(input , type , args ) .
*
* @param The class type of the object to create.
* @param input
* The input.
*
Can be any of the following types:
*
* null
* - {@link Reader}
*
- {@link CharSequence}
*
- {@link InputStream} containing UTF-8 encoded text (or charset defined by
* {@link org.apache.juneau.parser.ReaderParser.Builder#streamCharset(Charset)} property value).
*
byte []
containing UTF-8 encoded text (or charset defined by
* {@link org.apache.juneau.parser.ReaderParser.Builder#streamCharset(Charset)} property value).
* - {@link File} containing system encoded text (or charset defined by
* {@link org.apache.juneau.parser.ReaderParser.Builder#fileCharset(Charset)} property value).
*
* @param type
* The object type to create.
*
Can be any of the following: {@link ClassMeta}, {@link Class}, {@link ParameterizedType}, {@link GenericArrayType}
* @param args
* The type arguments of the class if it's a collection or map.
*
Can be any of the following: {@link ClassMeta}, {@link Class}, {@link ParameterizedType}, {@link GenericArrayType}
*
Ignored if the main type is not a map or collection.
* @return The parsed object.
* @throws ParseException Malformed input encountered.
* @throws IOException Thrown by underlying stream.
* @see BeanSession#getClassMeta(Type,Type...) for argument syntax for maps and collections.
*/
public static T to(Object input, Type type, Type...args) throws ParseException, IOException {
return DEFAULT.read(input, type, args);
}
/**
* Serializes a Java object to a Plain Text string.
*
*
* A shortcut for calling DEFAULT .write(object ) .
*
* @param object The object to serialize.
* @return
* The serialized object.
* @throws SerializeException If a problem occurred trying to convert the output.
*/
public static String of(Object object) throws SerializeException {
return DEFAULT.write(object);
}
/**
* Serializes a Java object to a Plain Text output.
*
*
* A shortcut for calling DEFAULT .write(output ) .
*
* @param object The object to serialize.
* @param output
* The output object.
*
Can be any of the following types:
*
* - {@link Writer}
*
- {@link OutputStream} - Output will be written as UTF-8 encoded stream.
*
- {@link File} - Output will be written as system-default encoded stream.
*
- {@link StringBuilder} - Output will be written to the specified string builder.
*
* @return The output object.
* @throws SerializeException If a problem occurred trying to convert the output.
* @throws IOException Thrown by underlying stream.
*/
public static Object of(Object object, Object output) throws SerializeException, IOException {
DEFAULT.write(object, output);
return output;
}
}