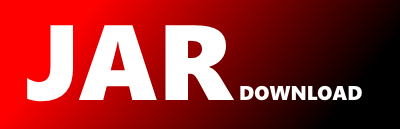
org.apache.juneau.microservice.console.ConsoleCommand Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.microservice.console;
import java.io.*;
import java.util.*;
import org.apache.juneau.collections.*;
/**
* Implements a command that can be invoked from the console of the microservice.
*
*
* Console commands allow you to interact with your microservice through the system console.
*
*
* Console commands are associated with the microservice through the following:
*
* - The
"Console/commands" configuration value.
*
This is a comma-delimited list of fully-qualified names of classes implementing this interface.
*
When associated this way, the implementation class must have a no-arg constructor.
* - Specifying commands via the {@link org.apache.juneau.microservice.Microservice.Builder#consoleCommands(Class...)} method.
*
This allows you to override the default implementation above and augment or replace the list
* with your own implementation objects.
*
*
*
* For example, the {@link HelpCommand} is used to provide help on other commands.
*
*
* Running class 'JettyMicroservice' using config file 'examples.cfg'.
* Server started on port 10000
*
* List of available commands:
* exit -- Shut down service
* restart -- Restarts service
* help -- Commands help
* echo -- Echo command
*
* > help help
* NAME
* help -- Commands help
*
* SYNOPSIS
* help [command]
*
* DESCRIPTION
* When called without arguments, prints the descriptions of all available commands.
* Can also be called with one or more arguments to get detailed information on a command.
*
* EXAMPLES
* List all commands:
* > help
*
* List help on the help command:
* > help help
*
* >
*
*
*
* The arguments are available as an {@link Args} object which allows for easy accessed to parsed command lines.
* Some simple examples of valid command lines:
*
*
* // mycommand
* args .get("0" ); // "mycommand"
*
* // mycommand arg1 arg2
* args .get("0" ); // "mycommand"
* args .get("1" ); // "arg1"
* args .get("2" ); // "arg2"
*
* // mycommand -optArg1 foo bar -optArg2 baz qux
* args .get("0" ); // "mycommand"
* args .get("optArg1" , String[].class ); // ["foo","bar"]
* args .get("optArg2" , String[].class ); // ["baz","qux"]
*
* // mycommand -optArg1 "foo bar" -optArg2 'baz qux'
* args .get("0" ); // "mycommand"
* args .get("optArg1" , String[].class ); // ["foo bar"]
* args .get("optArg2" , String[].class ); // ["baz qux"]
*
*
* See Also:
*/
public abstract class ConsoleCommand {
/**
* Returns the name of the command.
*
*
* Example: "help" for the help command.
*
* @return
* The name of the command.
*
Must not be null or contain spaces.
*/
abstract public String getName();
/**
* Returns the usage synopsis of the command.
*
*
* Example: "help [command ...]"
*
*
* The default implementation just returns the name, which implies the command takes no additional arguments.
*
* @return The synopsis of the command.
*/
public String getSynopsis() {
return getName();
}
/**
* Returns a one-line localized description of the command.
*
*
* The locale should be the system locale.
*
* @return
* The localized description of the command.
*
Can be null if there is no information.
*/
public String getInfo() {
return null;
}
/**
* Returns localized details of the command.
*
*
* The locale should be the system locale.
*
* @return
* The localized details of the command.
*
Can be null if there is no additional description.
*/
public String getDescription() {
return null;
}
/**
* Returns localized examples of the command.
*
*
* The locale should be the system locale.
*
* @return
* The localized examples of the command.
*
Can be null if there is no examples.
*/
public String getExamples() {
return null;
}
/**
* Executes a command.
* @param in The console reader.
* @param out The console writer.
* @param args The command arguments. The first argument is always the command itself.
*
* @return
* true if the console read thread should exit.
*
Normally you want to return true if your action is causing the microservice to exit or restart.
* @throws Exception
* Any thrown exception will simply be sent to STDERR.
*/
abstract public boolean execute(Scanner in, PrintWriter out, Args args) throws Exception;
}