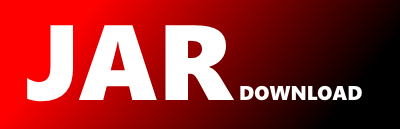
org.apache.juneau.objecttools.ObjectIntrospector Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.objecttools;
import java.io.*;
import java.lang.reflect.*;
import org.apache.juneau.json.*;
import org.apache.juneau.parser.*;
import org.apache.juneau.reflect.*;
/**
* POJO method introspector.
*
*
* This class is used to invoke methods on {@code Objects} using arguments in serialized form.
*
*
* Example:
*
* String string1 = "foobar" ;
* String string2 = ObjectIntrospector
* .create(string )
* .invoke(String.class , "substring(int,int)" , "[3,6]" ); // "bar"
*
*
* The arguments passed to the identified method are POJOs serialized in JSON format. Arbitrarily complex arguments can be passed
* in as arguments.
*
*
* - This is an extremely powerful but potentially dangerous tool. Use wisely.
*
*
* See Also:
*
*/
public final class ObjectIntrospector {
//-----------------------------------------------------------------------------------------------------------------
// Static
//-----------------------------------------------------------------------------------------------------------------
/**
* Static creator.
* @param o The object on which Java methods will be invoked.
* @return A new {@link ObjectIntrospector} object.
*/
public static ObjectIntrospector create(Object o) {
return new ObjectIntrospector(o);
}
/**
* Static creator.
* @param o The object on which Java methods will be invoked.
* @param parser The parser to use to parse the method arguments.
* @return A new {@link ObjectIntrospector} object.
*/
public static ObjectIntrospector create(Object o, ReaderParser parser) {
return new ObjectIntrospector(o, parser);
}
//-----------------------------------------------------------------------------------------------------------------
// Instance
//-----------------------------------------------------------------------------------------------------------------
private final Object object;
private final ReaderParser parser;
/**
* Constructor.
*
* @param object The object on which Java methods will be invoked.
* @param parser The parser to use to parse the method arguments.
* If null , {@link JsonParser#DEFAULT} is used.
*/
public ObjectIntrospector(Object object, ReaderParser parser) {
if (parser == null)
parser = JsonParser.DEFAULT;
this.object = object;
this.parser = parser;
}
/**
* Shortcut for calling new ObjectIntrospector(o, null );
*
* @param o The object on which Java methods will be invoked.
*/
public ObjectIntrospector(Object o) {
this(o, null);
}
/**
* Primary method.
*
*
* Invokes the specified method on this bean.
*
* @param method The method being invoked.
* @param args
* The arguments to pass as parameters to the method.
* These will automatically be converted to the appropriate object type if possible.
* Can be null if method has no arguments.
* @return The object returned by the call to the method, or null if target object is null .
* @throws IllegalAccessException
* If the Constructor object enforces Java language access control and the underlying constructor is
* inaccessible.
* @throws IllegalArgumentException
* If one of the following occurs:
*
* -
* The number of actual and formal parameters differ.
*
-
* An unwrapping conversion for primitive arguments fails.
*
-
* A parameter value cannot be converted to the corresponding formal parameter type by a method invocation
* conversion.
*
-
* The constructor pertains to an enum type.
*
* @throws InvocationTargetException If the underlying constructor throws an exception.
* @throws ParseException Malformed input encountered.
* @throws IOException Thrown by underlying stream.
*/
public Object invokeMethod(Method method, Reader args) throws InvocationTargetException, IllegalArgumentException, IllegalAccessException, ParseException, IOException {
if (object == null)
return null;
Object[] params = args == null ? null : parser.parseArgs(args, method.getGenericParameterTypes());
return method.invoke(object, params);
}
/**
* Primary method.
*
*
* Invokes the specified method on this bean.
*
* @param The return type of the method call.
* @param returnType The return type of the method call.
* @param method The method being invoked.
* @param args
* The arguments to pass as parameters to the method.
* These will automatically be converted to the appropriate object type if possible.
* Can be null if method has no arguments.
* @return The object returned by the call to the method, or null if target object is null .
* @throws IllegalAccessException
* If the Constructor object enforces Java language access control and the underlying constructor is
* inaccessible.
* @throws IllegalArgumentException
* If one of the following occurs:
*
* -
* The number of actual and formal parameters differ.
*
-
* An unwrapping conversion for primitive arguments fails.
*
-
* A parameter value cannot be converted to the corresponding formal parameter type by a method invocation
* conversion.
*
-
* The constructor pertains to an enum type.
*
* @throws InvocationTargetException If the underlying constructor throws an exception.
* @throws ParseException Malformed input encountered.
* @throws IOException Thrown by underlying stream.
*/
public T invokeMethod(Class returnType, Method method, Reader args) throws InvocationTargetException, IllegalArgumentException, IllegalAccessException, ParseException, IOException {
return returnType.cast(invokeMethod(method, args));
}
/**
* Convenience method for invoking argument from method signature (@see {@link MethodInfo#getSignature()}.
*
* @param method The method being invoked.
* @param args
* The arguments to pass as parameters to the method.
* These will automatically be converted to the appropriate object type if possible.
* Can be null if method has no arguments.
* @return The object returned by the call to the method, or null if target object is null .
* @throws NoSuchMethodException If method does not exist.
* @throws IllegalAccessException
* If the Constructor object enforces Java language access control and
* the underlying constructor is inaccessible.
* @throws IllegalArgumentException
* If one of the following occurs:
*
* -
* The number of actual and formal parameters differ.
*
-
* An unwrapping conversion for primitive arguments fails.
*
-
* A parameter value cannot be converted to the corresponding formal parameter type by a method invocation
* conversion.
*
-
* The constructor pertains to an enum type.
*
* @throws InvocationTargetException If the underlying constructor throws an exception.
* @throws ParseException Malformed input encountered.
* @throws IOException Thrown by underlying stream.
*/
public Object invokeMethod(String method, String args) throws NoSuchMethodException, IllegalArgumentException, InvocationTargetException, IllegalAccessException, ParseException, IOException {
if (object == null)
return null;
Method m = parser.getBeanContext().getClassMeta(object.getClass()).getPublicMethods().get(method);
if (m == null)
throw new NoSuchMethodException(method);
return invokeMethod(m, args == null ? null : new StringReader(args));
}
/**
* Convenience method for invoking argument from method signature (@see {@link MethodInfo#getSignature()}.
*
* @param The return type of the method call.
* @param returnType The return type of the method call.
* @param method The method being invoked.
* @param args
* The arguments to pass as parameters to the method.
* These will automatically be converted to the appropriate object type if possible.
* Can be null if method has no arguments.
* @return The object returned by the call to the method, or null if target object is null .
* @throws NoSuchMethodException If method does not exist.
* @throws IllegalAccessException
* If the Constructor object enforces Java language access control and
* the underlying constructor is inaccessible.
* @throws IllegalArgumentException
* If one of the following occurs:
*
* -
* The number of actual and formal parameters differ.
*
-
* An unwrapping conversion for primitive arguments fails.
*
-
* A parameter value cannot be converted to the corresponding formal parameter type by a method invocation
* conversion.
*
-
* The constructor pertains to an enum type.
*
* @throws InvocationTargetException If the underlying constructor throws an exception.
* @throws ParseException Malformed input encountered.
* @throws IOException Thrown by underlying stream.
*/
public T invokeMethod(Class returnType, String method, String args) throws NoSuchMethodException, IllegalArgumentException, InvocationTargetException, IllegalAccessException, ParseException, IOException {
return returnType.cast(invokeMethod(method, args));
}
}