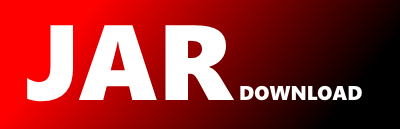
org.apache.juneau.parser.annotation.ParserConfig Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.parser.annotation;
import static java.lang.annotation.ElementType.*;
import static java.lang.annotation.RetentionPolicy.*;
import java.lang.annotation.*;
import java.nio.charset.*;
import org.apache.juneau.*;
import org.apache.juneau.annotation.*;
import org.apache.juneau.html.*;
import org.apache.juneau.json.*;
import org.apache.juneau.msgpack.*;
import org.apache.juneau.parser.*;
import org.apache.juneau.uon.*;
import org.apache.juneau.xml.*;
/**
* Annotation for specifying config properties defined in {@link Parser}, {@link InputStreamParser}, and {@link ReaderParser}.
*
*
* Used primarily for specifying bean configuration properties on REST classes and methods.
*
*
See Also:
*/
@Target({TYPE,METHOD})
@Retention(RUNTIME)
@Inherited
@ContextApply({ParserConfigAnnotation.ParserApply.class,ParserConfigAnnotation.InputStreamParserApply.class,ParserConfigAnnotation.ReaderParserApply.class})
public @interface ParserConfig {
/**
* Optional rank for this config.
*
*
* Can be used to override default ordering and application of config annotations.
*
* @return The annotation value.
*/
int rank() default 0;
//-------------------------------------------------------------------------------------------------------------------
// InputStreamParser
//-------------------------------------------------------------------------------------------------------------------
/**
* Binary input format.
*
*
* When using the {@link Parser#parse(Object,Class)} method on stream-based parsers and the input is a string, this defines the format to use
* when converting the string into a byte array.
*
*
* "SPACED_HEX"
* "HEX" (default)
* "BASE64"
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.parser.InputStreamParser.Builder#binaryFormat(BinaryFormat)}
*
*
* @return The annotation value.
*/
String binaryFormat() default "";
//-------------------------------------------------------------------------------------------------------------------
// Parser
//-------------------------------------------------------------------------------------------------------------------
/**
* Auto-close streams.
*
*
* If "true" , InputStreams and Readers passed into parsers will be closed
* after parsing is complete.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.parser.Parser.Builder#autoCloseStreams()}
*
*
* @return The annotation value.
*/
String autoCloseStreams() default "";
/**
* Debug output lines.
*
*
* When parse errors occur, this specifies the number of lines of input before and after the
* error location to be printed as part of the exception message.
*
*
Notes:
* -
* Format: integer
*
-
* Default: 5
*
-
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.parser.Parser.Builder#debugOutputLines(int)}
*
*
* @return The annotation value.
*/
String debugOutputLines() default "";
/**
* Parser listener.
*
*
* Class used to listen for errors and warnings that occur during parsing.
*
*
See Also:
* - {@link org.apache.juneau.parser.Parser.Builder#listener(Class)}
*
*
* @return The annotation value.
*/
Class extends ParserListener> listener() default ParserListener.Void.class;
/**
* Strict mode.
*
*
* If "true" , strict mode for the parser is enabled.
*
*
* Strict mode can mean different things for different parsers.
*
*
* Parser class Strict behavior
*
* All reader-based parsers
*
* When enabled, throws {@link ParseException ParseExceptions} on malformed charset input.
* Otherwise, malformed input is ignored.
*
*
*
* {@link JsonParser}
*
* When enabled, throws exceptions on the following invalid JSON syntax:
*
* - Unquoted attributes.
*
- Missing attribute values.
*
- Concatenated strings.
*
- Javascript comments.
*
- Numbers and booleans when Strings are expected.
*
- Numbers valid in Java but not JSON (e.g. octal notation, etc...)
*
*
*
*
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.parser.Parser.Builder#strict()}
*
*
* @return The annotation value.
*/
String strict() default "";
/**
* Trim parsed strings.
*
*
* If "true" , string values will be trimmed of whitespace using {@link String#trim()} before being added to
* the POJO.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.parser.Parser.Builder#trimStrings()}
*
*
* @return The annotation value.
*/
String trimStrings() default "";
/**
* Unbuffered.
*
*
* If "true" , don't use internal buffering during parsing.
*
*
* This is useful in cases when you want to parse the same input stream or reader multiple times
* because it may contain multiple independent POJOs to parse.
*
Buffering would cause the parser to read past the current POJO in the stream.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* This only allows for multi-input streams for the following parsers:
*
* - {@link JsonParser}
*
- {@link UonParser}
*
* It has no effect on the following parsers:
*
* - {@link MsgPackParser} - It already doesn't use buffering.
*
- {@link XmlParser}, {@link HtmlParser} - These use StAX which doesn't allow for more than one root element anyway.
*
- RDF parsers - These read everything into an internal model before any parsing begins.
*
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.parser.Parser.Builder#unbuffered()}
*
*
* @return The annotation value.
*/
String unbuffered() default "";
//-------------------------------------------------------------------------------------------------------------------
// ReaderParser
//-------------------------------------------------------------------------------------------------------------------
/**
* File charset.
*
*
* The character set to use for reading Files from the file system.
*
*
* Used when passing in files to {@link Parser#parse(Object, Class)}.
*
*
Notes:
* -
*
"DEFAULT" can be used to indicate the JVM default file system charset.
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.parser.ReaderParser.Builder#fileCharset(Charset)}
*
*
* @return The annotation value.
*/
String fileCharset() default "";
/**
* Input stream charset.
*
*
* The character set to use for converting InputStreams and byte arrays to readers.
*
*
* Used when passing in input streams and byte arrays to {@link Parser#parse(Object, Class)}.
*
*
Notes:
* -
*
"DEFAULT" can be used to indicate the JVM default file system charset.
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.parser.ReaderParser.Builder#streamCharset(Charset)}
*
*
* @return The annotation value.
*/
String streamCharset() default "";
}