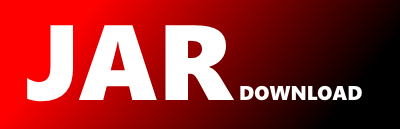
org.apache.juneau.rest.annotation.Attr Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.annotation;
import static java.lang.annotation.ElementType.*;
import static java.lang.annotation.RetentionPolicy.*;
import java.lang.annotation.*;
import org.apache.juneau.httppart.*;
import org.apache.juneau.oapi.*;
/**
* REST request attribute annotation.
*
*
* Identifies a POJO retrieved from the request attributes map.
*
* Annotation that can be applied to a parameter of a @RestOp -annotated method to identify it as a value
* retrieved from the request attributes.
*
*
Example:
*
* @RestGet
* public Person getPerson(@Attr ("ETag" ) UUID etag ) {...}
*
*
*
* This is functionally equivalent to the following code...
*
* @RestPost
* public void postPerson(RestRequest req , RestResponse res ) {
* UUID etag = req .getAttributes().get(UUID.class , "ETag" );
* ...
* }
*
*
* See Also:
*/
@Target({PARAMETER,TYPE})
@Retention(RUNTIME)
@Inherited
public @interface Attr {
/**
* Specifies the {@link HttpPartParser} class used for parsing strings to values.
*
*
* Overrides for this part the part parser defined on the REST resource which by default is {@link OpenApiParser}.
*
* @return The annotation value.
*/
Class extends HttpPartParser> parser() default HttpPartParser.Void.class;
//=================================================================================================================
// Attributes common to all Swagger Parameter objects
//=================================================================================================================
/**
* Request attribute name.
*
*
* The value should be either a valid attribute name, or "*" to represent multiple name/value pairs
*
*
* A blank value (the default) has the following behavior:
*
* -
* If the data type is
NameValuePairs , Map , or a bean,
* then it's the equivalent to "*" which will cause the value to be serialized as name/value pairs.
*
* Examples:
*
* @RestPost ("/addPet" )
* public void addPet(@Attr JsonMap allAttributes ) {...}
*
*
*
*
* @return The annotation value.
*/
String name() default "";
/**
* A synonym for {@link #name()}.
*
*
* Allows you to use shortened notation if you're only specifying the name.
*
*
* The following are completely equivalent ways of defining a header entry:
*
* public Order placeOrder(@Attr (name="api_key" ) String apiKey ) {...}
*
*
* public Order placeOrder(@Attr ("api_key" ) String apiKey ) {...}
*
*
* @return The annotation value.
*/
String value() default "";
}