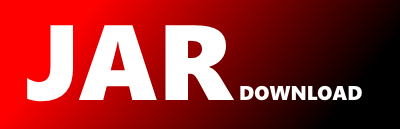
org.apache.juneau.rest.annotation.Rest Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.annotation;
import static java.lang.annotation.ElementType.*;
import static java.lang.annotation.RetentionPolicy.*;
import java.lang.annotation.*;
import java.nio.charset.*;
import java.util.*;
import org.apache.juneau.*;
import org.apache.juneau.annotation.*;
import org.apache.juneau.config.*;
import org.apache.juneau.cp.*;
import org.apache.juneau.encoders.*;
import org.apache.juneau.httppart.*;
import org.apache.juneau.parser.*;
import org.apache.juneau.rest.*;
import org.apache.juneau.rest.arg.*;
import org.apache.juneau.rest.converter.*;
import org.apache.juneau.rest.debug.*;
import org.apache.juneau.rest.guard.*;
import org.apache.juneau.rest.httppart.*;
import org.apache.juneau.rest.logger.*;
import org.apache.juneau.rest.processor.*;
import org.apache.juneau.rest.servlet.*;
import org.apache.juneau.rest.staticfile.*;
import org.apache.juneau.rest.swagger.*;
import org.apache.juneau.serializer.*;
/**
* Used to denote that a class is a REST resource and to associate metadata on it.
*
*
* Usually used on a subclass of {@link RestServlet}, but can be used to annotate any class that you want to expose as
* a REST resource.
*
*
See Also:
*/
@Target(TYPE)
@Retention(RUNTIME)
@Inherited
@ContextApply({RestAnnotation.RestContextApply.class,RestAnnotation.RestOpContextApply.class})
@AnnotationGroup(Rest.class)
public @interface Rest {
/**
* Disable content URL parameter.
*
*
* When enabled, the HTTP content content on PUT and POST requests can be passed in as text using the "content"
* URL parameter.
*
* For example:
*
* ?content=(name='John%20Smith',age=45)
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#disableContentParam()}
*
*
* @return The annotation value.
*/
String disableContentParam() default "";
/**
* Allowed header URL parameters.
*
*
* When specified, allows headers such as "Accept" and "Content-Type" to be passed in as URL query
* parameters.
*
* For example:
*
* ?Accept=text/json&Content-Type=text/json
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
* -
* Use
"*" to represent all methods.
* -
* Use
"NONE" (case insensitive) to suppress inheriting a value from a parent class.
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#allowedHeaderParams(String)}
*
*
* @return The annotation value.
*/
String allowedHeaderParams() default "";
/**
* Allowed method headers.
*
*
* A comma-delimited list of HTTP method names that are allowed to be passed as values in an X-Method HTTP header
* to override the real HTTP method name.
*
* Allows you to override the actual HTTP method with a simulated method.
*
For example, if an HTTP Client API doesn't support PATCH but does support POST (because
* PATCH is not part of the original HTTP spec), you can add a X-Method: PATCH header on a normal
* HTTP POST /foo request call which will make the HTTP call look like a PATCH request in any of the REST APIs.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
* -
* Method names are case-insensitive.
*
-
* Use
"*" to represent all methods.
* -
* Use
"NONE" (case insensitive) to suppress inheriting a value from a parent class.
*
*
* @return The annotation value.
*/
String allowedMethodHeaders() default "";
/**
* Allowed method parameters.
*
*
* When specified, the HTTP method can be overridden by passing in a "method" URL parameter on a regular
* GET request.
*
* For example:
*
* ?method=OPTIONS
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
* -
* Use
"*" to represent all methods.
* -
* Use
"NONE" (case insensitive) to suppress inheriting a value from a parent class.
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#allowedMethodParams(String)}
*
*
* @return The annotation value.
*/
String allowedMethodParams() default "";
/**
* Specifies the logger to use for logging of HTTP requests and responses.
*
* Notes:
* -
* The default call logger if not specified is {@link CallLogger}.
*
-
* The resource class itself will be used if it implements the {@link CallLogger} interface and not
* explicitly overridden via this annotation.
*
-
* The implementation must have one of the following constructors:
*
* public T(RestContext)
* public T()
* public static T create (RestContext)
* public static T create ()
*
* -
* Inner classes of the REST resource class are allowed.
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#callLogger()}
*
- Logging / Debugging
*
*
* @return The annotation value.
*/
Class extends CallLogger> callLogger() default CallLogger.Void.class;
/**
* The resolver used for resolving instances of child resources and various other beans including:
*
* - {@link CallLogger}
*
- {@link SwaggerProvider}
*
- {@link FileFinder}
*
- {@link StaticFiles}
*
*
*
* Note that the SpringRestServlet classes uses the SpringBeanStore class to allow for any
* Spring beans to be injected into your REST resources.
*
* @return The annotation value.
*/
Class extends BeanStore> beanStore() default BeanStore.Void.class;
/**
* REST children.
*
*
* Defines children of this resource.
*
*
Inheritance Rules
*
* - Children on child are combined with those on parent class.
*
- Children are list parent-to-child in the order they appear in the annotation.
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#children(Object...)}
*
*
* @return The annotation value.
*/
Class>[] children() default {};
/**
* Client version header.
*
*
* Specifies the name of the header used to denote the client version on HTTP requests.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#clientVersionHeader(String)}
*
*
* @return The annotation value.
*/
String clientVersionHeader() default "";
/**
* Optional location of configuration file for this servlet.
*
*
* The configuration file .
*
*
Inheritance Rules
*
* - Config file is searched for in child-to-parent order.
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
* -
* Use the keyword
SYSTEM_DEFAULT to refer to the system default configuration
* returned by the {@link Config#getSystemDefault()}.
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#config(Config)}
*
*
* @return The annotation value.
*/
String config() default "";
/**
* Supported content media types.
*
*
* Overrides the media types inferred from the parsers that identify what media types can be consumed by the resource.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#consumes(MediaType...)}
*
*
* @return The annotation value.
*/
String[] consumes() default {};
/**
* Class-level response converters.
*
*
* Associates one or more {@link RestConverter converters} with a resource class.
*
*
Inheritance Rules
*
* - Converters on child are combined with those on parent class.
*
- Converters are executed child-to-parent in the order they appear in the annotation.
*
- Converters on methods are executed before those on classes.
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestOpContext.Builder#converters()} - Registering converters with REST resources.
*
*
* @return The annotation value.
*/
Class extends RestConverter>[] converters() default {};
/**
* Enable debug mode.
*
*
* Enables the following:
*
* -
* HTTP request/response bodies are cached in memory for logging purposes.
*
-
* HTTP requests/responses are logged to the registered {@link CallLogger}.
*
*
*
* "true" - Debug is enabled for all requests.
* "false" - Debug is disabled for all requests.
* "conditional" - Debug is enabled only for requests that have a Debug: true header.
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
* -
* These debug settings can be overridden by the {@link Rest#debugOn()} annotation or at runtime by directly
* calling {@link RestRequest#setDebug()}.
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#debugEnablement()}
*
*
* @return The annotation value.
*/
String debug() default "";
/**
* Debug enablement bean.
*
* TODO
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#debugEnablement()}
*
*
* @return The annotation value.
*/
Class extends DebugEnablement> debugEnablement() default DebugEnablement.Void.class;
/**
* Enable debug mode on specified classes/methods.
*
*
* Enables the following:
*
* -
* HTTP request/response bodies are cached in memory for logging purposes on matching classes and methods.
*
-
* HTTP requests/responses are logged to the registered {@link CallLogger}.
*
*
*
* Consists of a comma-delimited list of strings of the following forms:
*
* "class-identifier" - Enable debug on the specified class.
* "class-identifier=[true|false|conditional]" - Explicitly enable debug on the specified class.
* "method-identifier" - Enable debug on the specified class.
* "method-identifier=[true|false|conditional]" - Explicitly enable debug on the specified class.
*
*
*
* Class identifiers can be any of the following forms:
*
* - Fully qualified:
*
* "com.foo.MyClass"
*
* - Fully qualified inner class:
*
* "com.foo.MyClass$Inner1$Inner2"
*
* - Simple:
*
* "MyClass"
*
* - Simple inner:
*
* "MyClass$Inner1$Inner2"
* "Inner1$Inner2"
* "Inner2"
*
*
*
*
* Method identifiers can be any of the following forms:
*
* - Fully qualified with args:
*
* "com.foo.MyClass.myMethod(String,int)"
* "com.foo.MyClass.myMethod(java.lang.String,int)"
* "com.foo.MyClass.myMethod()"
*
* - Fully qualified:
*
* "com.foo.MyClass.myMethod"
*
* - Simple with args:
*
* "MyClass.myMethod(String,int)"
* "MyClass.myMethod(java.lang.String,int)"
* "MyClass.myMethod()"
*
* - Simple:
*
* "MyClass.myMethod"
*
* - Simple inner class:
*
* "MyClass$Inner1$Inner2.myMethod"
* "Inner1$Inner2.myMethod"
* "Inner2.myMethod"
*
*
*
* Example:
*
* // Turn on debug per-request on the class and always on the doX() method .
* @Rest (
* debugOn="MyResource=conditional,MyResource.doX=true"
* )
* public class MyResource {
*
* @RestGet
* public void String getX() {
* ...
* }
*
*
*
* A more-typical scenario is to pull this setting from an external source such as system property or environment
* variable:
*
*
Example:
*
* @Rest (
* debugOn="$E{DEBUG_ON_SETTINGS}"
* )
* public class MyResource {...}
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
* -
* These debug settings override the settings define via {@link Rest#debug()} and {@link RestOp#debug()}.
*
-
* These debug settings can be overridden at runtime by directly calling {@link RestRequest#setDebug()}.
*
*
* @return The annotation value.
*/
String debugOn() default "";
/**
* Default Accept header.
*
*
* The default value for the Accept header if not specified on a request.
*
*
* This is a shortcut for using {@link #defaultRequestHeaders()} for just this specific header.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
String defaultAccept() default "";
/**
* Default character encoding.
*
*
* The default character encoding for the request and response if not specified on the request.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#defaultCharset(Charset)}
*
- {@link org.apache.juneau.rest.RestOpContext.Builder#defaultCharset(Charset)}
*
- {@link RestOp#defaultCharset}
*
- {@link RestGet#defaultCharset}
*
- {@link RestPut#defaultCharset}
*
- {@link RestPost#defaultCharset}
*
- {@link RestDelete#defaultCharset}
*
*
* @return The annotation value.
*/
String defaultCharset() default "";
/**
* Default Content-Type header.
*
*
* The default value for the Content-Type header if not specified on a request.
*
*
* This is a shortcut for using {@link #defaultRequestHeaders()} for just this specific header.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
String defaultContentType() default "";
/**
* Default request attributes.
*
*
* Specifies default values for request attributes if they're not already set on the request.
*
*
* Affects values returned by the following methods:
*
* - {@link RestRequest#getAttribute(String)}.
*
- {@link RestRequest#getAttributes()}.
*
*
* Example:
*
* // Defined via annotation resolving to a config file setting with default value.
* @Rest (defaultRequestAttributes={"Foo=bar" , "Baz: $C{REST/myAttributeValue}" })
* public class MyResource {
*
* // Override at the method level.
* @RestGet (defaultRequestAttributes={"Foo: bar" })
* public Object myMethod() {...}
* }
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#defaultRequestAttributes(NamedAttribute...)}
*
- {@link RestOp#defaultRequestAttributes()}
*
- {@link RestGet#defaultRequestAttributes()}
*
- {@link RestPut#defaultRequestAttributes()}
*
- {@link RestPost#defaultRequestAttributes()}
*
- {@link RestDelete#defaultRequestAttributes()}
*
*
* @return The annotation value.
*/
String[] defaultRequestAttributes() default {};
/**
* Default request headers.
*
*
* Specifies default values for request headers if they're not passed in through the request.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#defaultRequestHeaders(org.apache.http.Header...)}
*
*
* @return The annotation value.
*/
String[] defaultRequestHeaders() default {};
/**
* Default response headers.
*
*
* Specifies default values for response headers if they're not set after the Java REST method is called.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#defaultResponseHeaders(org.apache.http.Header...)}
*
*
* @return The annotation value.
*/
String[] defaultResponseHeaders() default {};
/**
* Optional servlet description.
*
*
* It is used to populate the Swagger description field.
*
*
Inheritance Rules
*
* - Description is searched for in child-to-parent order.
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
* -
* The format is plain-text.
*
Multiple lines are concatenated with newlines.
*
*
* @return The annotation value.
*/
String[] description() default {};
/**
* Specifies the compression encoders for this resource.
*
*
* Encoders are used to enable various kinds of compression (e.g. "gzip" ) on requests and responses.
*
*
* Encoders are automatically inherited from {@link Rest#encoders()} annotations on parent classes with the encoders on child classes
* prepended to the encoder group.
* The {@link org.apache.juneau.encoders.EncoderSet.NoInherit} class can be used to prevent inheriting from the parent class.
*
*
Example:
*
* // Define a REST resource that handles GZIP compression.
* @Rest (
* encoders={
* GzipEncoder.class
* }
* )
* public class MyResource {
* ...
* }
*
*
*
* The encoders can also be tailored at the method level using {@link RestOp#encoders()} (and related annotations).
*
*
* The programmatic equivalent to this annotation is:
*
* RestContext.Builder builder = RestContext.create (resource );
* builder .getEncoders().add(classes );
*
*
* Inheritance Rules
*
* - Encoders on child are combined with those on parent class.
*
*
* See Also:
* - Encoders
*
*
* @return The annotation value.
*/
Class extends Encoder>[] encoders() default {};
/**
* Class-level guards.
*
*
* Associates one or more {@link RestGuard RestGuards} with all REST methods defined in this class.
*
*
Inheritance Rules
*
* - Guards on child are combined with those on parent class.
*
- Guards are executed child-to-parent in the order they appear in the annotation.
*
- Guards on methods are executed before those on classes.
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestOpContext.Builder#guards()}
*
*
* @return The annotation value.
*/
Class extends RestGuard>[] guards() default {};
/**
* The maximum allowed input size (in bytes) on HTTP requests.
*
*
* Useful for alleviating DoS attacks by throwing an exception when too much input is received instead of resulting
* in out-of-memory errors which could affect system stability.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#maxInput(String)}
*
- {@link org.apache.juneau.rest.RestOpContext.Builder#maxInput(String)}
*
- {@link RestOp#maxInput}
*
- {@link RestPost#maxInput}
*
- {@link RestPut#maxInput}
*
*
* @return The annotation value.
*/
String maxInput() default "";
/**
* Messages.
*
* Identifies the location of the resource bundle for this class.
*
*
* There are two possible formats:
*
* - A simple string - Represents the {@link org.apache.juneau.cp.Messages.Builder#name(String) name} of the resource bundle.
*
Example:
*
* // Bundle name is Messages.properties.
* @Rest (messages="Messages" )
*
* - Simplified JSON - Represents parameters for the {@link org.apache.juneau.cp.Messages.Builder} class.
*
Example:
*
* // Bundles can be found in two packages.
* @Rest (messages="{name:'Messages',baseNames:['{package}.{name}','{package}.i18n.{name}']" )
*
*
*
*
* If the bundle name is not specified, the class name of the resource object is used.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
String messages() default "";
/**
* Dynamically apply this annotation to the specified classes.
*
* See Also:
*
* @return The annotation value.
*/
String[] on() default {};
/**
* Dynamically apply this annotation to the specified classes.
*
*
* Identical to {@link #on()} except allows you to specify class objects instead of a strings.
*
*
See Also:
*
* @return The annotation value.
*/
Class>[] onClass() default {};
/**
* Specifies the parsers for converting HTTP request bodies into POJOs.
*
*
* Parsers are used to convert the content of HTTP requests into POJOs.
*
Any of the Juneau framework parsers can be used in this setting.
*
The parser selected is based on the request Content-Type header matched against the values returned by the following method
* using a best-match algorithm:
*
* - {@link Parser#getMediaTypes()}
*
*
*
* Parsers are automatically inherited from {@link Rest#parsers()} annotations on parent classes with the parsers on child classes
* prepended to the parser group.
* The {@link org.apache.juneau.parser.ParserSet.NoInherit} class can be used to prevent inheriting from the parent class.
*
*
Example:
*
* // Define a REST resource that can consume JSON and XML.
* @Rest (
* parsers={
* JsonParser.class ,
* XmlParser.class
* }
* )
* public class MyResource {
* ...
* }
*
*
*
* The parsers can also be tailored at the method level using {@link RestOp#parsers()} (and related annotations).
*
*
* The programmatic equivalent to this annotation is:
*
* RestContext.Builder builder = RestContext.create (resource );
* builder .getParsers().add(classes );
*
*
* Inheritance Rules
*
* - Parsers on child override those on parent class.
*
- {@link org.apache.juneau.parser.ParserSet.Inherit} class can be used to inherit and augment values from parent.
*
- {@link org.apache.juneau.parser.ParserSet.NoInherit} class can be used to suppress inheriting from parent.
*
- Parsers on methods take precedence over those on classes.
*
*
* See Also:
* - Marshalling
*
*
* @return The annotation value.
*/
Class>[] parsers() default {};
/**
* HTTP part parser.
*
*
* Specifies the {@link HttpPartParser} to use for parsing headers, query/form parameters, and URI parts.
*
* @return The annotation value.
*/
Class extends HttpPartParser> partParser() default HttpPartParser.Void.class;
/**
* HTTP part serializer.
*
*
* Specifies the {@link HttpPartSerializer} to use for serializing headers, query/form parameters, and URI parts.
*
* @return The annotation value.
*/
Class extends HttpPartSerializer> partSerializer() default HttpPartSerializer.Void.class;
/**
* Resource path.
*
*
* Used in the following situations:
*
* -
* On child resources (resource classes attached to parents via the {@link #children()} annotation) to identify
* the subpath used to access the child resource relative to the parent.
*
-
* On top-level {@link RestServlet} classes deployed as Spring beans when
JuneauRestInitializer is being used.
*
*
* On child resources
*
* The typical usage is to define a path to a child resource relative to the parent resource.
*
*
Example:
*
* @Rest (
* children={ChildResource.class }
* )
* public class TopLevelResource extends BasicRestServlet {...}
*
* @Rest (
* path="/child" ,
* children={GrandchildResource.class }
* )
* public class ChildResource {...}
*
* @Rest (
* path="/grandchild"
* )
* public class GrandchildResource {
* @RestGet ("/" )
* public String sayHello() {
* return "Hello!" ;
* }
* }
*
*
* In the example above, assuming the TopLevelResource servlet is deployed to path /myContext/myServlet ,
* then the sayHello method is accessible through the URI /myContext/myServlet/child/grandchild .
*
*
* Note that in this scenario, the path attribute is not defined on the top-level resource.
* Specifying the path on the top-level resource has no effect, but can be used for readability purposes.
*
*
Path variables
*
* The path can contain variables that get resolved to {@link org.apache.juneau.http.annotation.Path @Path} parameters
* or access through the {@link RestRequest#getPathParams()} method.
*
*
Example:
*
* @Rest (
* path="/myResource/{foo}/{bar}"
* )
* public class MyResource extends BasicRestServlet {
*
* @RestGet ("/{baz}" )
* public void String doX(@Path String foo , @Path int bar , @Path MyPojo baz ) {
* ...
* }
* }
*
*
*
* Variables can be used on either top-level or child resources and can be defined on multiple levels.
*
*
* All variables in the path must be specified or else the target will not resolve and a 404 will result.
*
*
* When variables are used on a path of a top-level resource deployed as a Spring bean in a Spring Boot application,
* the first part of the URL must be a literal which will be used as the servlet path of the registered servlet.
*
*
Notes:
* -
* The leading slash is optional.
"/myResource" and "myResource" is equivalent.
* -
* The paths
"/myResource" and "/myResource/*" are equivalent.
* -
* Paths must not end with
"/" (per the servlet spec).
*
*
* Inheritance Rules
*
* - Path is searched for in child-to-parent order.
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#path(String)}
*
*
* @return The annotation value.
*/
String path() default "";
/**
* Supported accept media types.
*
*
* Overrides the media types inferred from the serializers that identify what media types can be produced by the resource.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#produces(MediaType...)}
*
*
* @return The annotation value.
*/
String[] produces() default {};
/**
* Render response stack traces in responses.
*
*
* Render stack traces in HTTP response bodies when errors occur.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
String renderResponseStackTraces() default "";
/**
* Response processors.
*
*
* Specifies a list of {@link ResponseProcessor} classes that know how to convert POJOs returned by REST methods or
* set via {@link RestResponse#setContent(Object)} into appropriate HTTP responses.
*
*
See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#responseProcessors()}
*
*
* @return The annotation value.
*/
Class extends ResponseProcessor>[] responseProcessors() default {};
/**
* REST children class.
*
*
* Allows you to extend the {@link RestChildren} class to modify how any of the methods are implemented.
*
*
See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#restChildrenClass(Class)}
*
*
* @return The annotation value.
*/
Class extends RestChildren> restChildrenClass() default RestChildren.Void.class;
/**
* REST methods class.
*
*
* Allows you to extend the {@link RestOperations} class to modify how any of the methods are implemented.
*
*
See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#restOperationsClass(Class)}
*
*
* @return The annotation value.
*/
Class extends RestOperations> restOperationsClass() default RestOperations.Void.class;
/**
* Java REST operation method parameter resolvers.
*
*
* By default, the Juneau framework will automatically Java method parameters of various types (e.g.
* RestRequest , Accept , Reader ).
*
This setting allows you to provide your own resolvers for your own class types that you want resolved.
*
*
See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#restOpArgs(Class...)}
*
*
* @return The annotation value.
*/
Class extends RestOpArg>[] restOpArgs() default {};
/**
* Role guard.
*
*
* An expression defining if a user with the specified roles are allowed to access methods on this class.
*
*
* This is a shortcut for specifying {@link RestOp#roleGuard()} on all the REST operations on a class.
*
*
Example:
*
* @Rest (
* path="/foo" ,
* roleGuard="ROLE_ADMIN || (ROLE_READ_WRITE && ROLE_SPECIAL)"
* )
* public class MyResource extends BasicRestServlet {
* ...
* }
*
*
* Notes:
* -
* Supports any of the following expression constructs:
*
* "foo" - Single arguments.
* "foo,bar,baz" - Multiple OR'ed arguments.
* "foo | bar | baz" - Multiple OR'ed arguments, pipe syntax.
* "foo || bar || baz" - Multiple OR'ed arguments, Java-OR syntax.
* "fo*" - Patterns including '*' and '?' .
* "fo* & *oo" - Multiple AND'ed arguments, ampersand syntax.
* "fo* && *oo" - Multiple AND'ed arguments, Java-AND syntax.
* "fo* || (*oo || bar)" - Parenthesis.
*
* -
* AND operations take precedence over OR operations (as expected).
*
-
* Whitespace is ignored.
*
-
*
null or empty expressions always match as false .
* -
* If patterns are used, you must specify the list of declared roles using {@link #rolesDeclared()} or {@link org.apache.juneau.rest.RestOpContext.Builder#rolesDeclared(String...)}.
*
-
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestOpContext.Builder#roleGuard(String)}
*
*
* @return The annotation value.
*/
String roleGuard() default "";
/**
* Declared roles.
*
*
* A comma-delimited list of all possible user roles.
*
*
* Used in conjunction with {@link #roleGuard()} is used with patterns.
*
*
* This is a shortcut for specifying {@link RestOp#rolesDeclared()} on all the REST operations on a class.
*
*
Example:
*
* @Rest (
* rolesDeclared="ROLE_ADMIN,ROLE_READ_WRITE,ROLE_READ_ONLY,ROLE_SPECIAL" ,
* roleGuard="ROLE_ADMIN || (ROLE_READ_WRITE && ROLE_SPECIAL)"
* )
* public class MyResource extends BasicRestServlet {
* ...
* }
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestOpContext.Builder#rolesDeclared(String...)}
*
*
* @return The annotation value.
*/
String rolesDeclared() default "";
/**
* Specifies the serializers for POJOs into HTTP response bodies.
*
*
* Serializer are used to convert POJOs to HTTP response bodies.
*
Any of the Juneau framework serializers can be used in this setting.
*
The serializer selected is based on the request Accept header matched against the values returned by the following method
* using a best-match algorithm:
*
* - {@link Serializer#getMediaTypeRanges()}
*
*
*
* Serializers are automatically inherited from {@link Rest#serializers()} annotations on parent classes with the serializers on child classes
* prepended to the serializer group.
* The {@link org.apache.juneau.serializer.SerializerSet.NoInherit} class can be used to prevent inheriting from the parent class.
*
*
Example:
*
* // Define a REST resource that can produce JSON and XML.
* @Rest (
* serializers={
* JsonParser.class ,
* XmlParser.class
* }
* )
* public class MyResource {
* ...
* }
*
*
*
* The serializers can also be tailored at the method level using {@link RestOp#serializers()} (and related annotations).
*
*
* The programmatic equivalent to this annotation is:
*
* RestContext.Builder builder = RestContext.create (resource );
* builder .getSerializers().add(classes );
*
*
* Inheritance Rules
*
* - Serializers on child override those on parent class.
*
- {@link org.apache.juneau.serializer.SerializerSet.Inherit} class can be used to inherit and augment values from parent.
*
- {@link org.apache.juneau.serializer.SerializerSet.NoInherit} class can be used to suppress inheriting from parent.
*
- Serializers on methods take precedence over those on classes.
*
*
* See Also:
* - Marshalling
*
*
* @return The annotation value.
*/
Class extends Serializer>[] serializers() default {};
/**
* Optional site name.
*
*
* The site name is intended to be a title that can be applied to the entire site.
*
*
* One possible use is if you want to add the same title to the top of all pages by defining a header on a
* common parent class like so:
*
* @HtmlDocConfig (
* header={
* "<h1>$RS{siteName}</h1>" ,
* "<h2>$RS{title}</h2>"
* }
* )
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
String siteName() default "";
/**
* Static files.
*
*
* Used to retrieve localized files to be served up as static files through the REST API via the following
* predefined methods:
*
* - {@link BasicRestObject#getHtdoc(String, Locale)}.
*
- {@link BasicRestServlet#getHtdoc(String, Locale)}.
*
*
*
* The static file finder can be accessed through the following methods:
*
* - {@link RestContext#getStaticFiles()}
*
- {@link RestRequest#getStaticFiles()}
*
*
* Inheritance Rules
*
* - Static files on child are combined with those on parent class.
*
- Static files are are executed child-to-parent in the order they appear in the annotation.
*
*
* @return The annotation value.
*/
Class extends StaticFiles> staticFiles() default StaticFiles.Void.class;
/**
* Provides swagger-specific metadata on this resource.
*
*
* Used to populate the auto-generated OPTIONS swagger documentation.
*
*
Example:
*
* @Rest (
* path="/addressBook" ,
*
* // Swagger info.
* swagger=@Swagger({
* "contact:{name:'John Smith',email:'[email protected]'}," ,
* "license:{name:'Apache 2.0',url:'http://www.apache.org/licenses/LICENSE-2.0.html'}," ,
* "version:'2.0', ,
* "termsOfService:'You are on your own.'," ,
* "tags:[{name:'Java',description:'Java utility',externalDocs:{description:'Home page',url:'http://juneau.apache.org'}}]," ,
* "externalDocs:{description:'Home page',url:'http://juneau.apache.org'}"
* })
* )
*
*
* See Also:
* - {@link Swagger}
*
*
* @return The annotation value.
*/
Swagger swagger() default @Swagger;
/**
* Swagger provider.
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#swaggerProvider(Class)}
*
*
* @return The annotation value.
*/
Class extends SwaggerProvider> swaggerProvider() default SwaggerProvider.Void.class;
/**
* Optional servlet title.
*
*
* It is used to populate the Swagger title field.
*
*
Inheritance Rules
*
* - Label is searched for in child-to-parent order.
*
*
* Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
* -
* Corresponds to the swagger field
/info/title .
*
*
* @return The annotation value.
*/
String[] title() default {};
/**
* Resource authority path.
*
*
* Overrides the authority path value for this resource and any child resources.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#uriAuthority(String)}
*
*
* @return The annotation value.
*/
String uriAuthority() default "";
/**
* Resource context path.
*
*
* Overrides the context path value for this resource and any child resources.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#uriContext(String)}
*
*
* @return The annotation value.
*/
String uriContext() default "";
/**
* URI-resolution relativity.
*
*
* Specifies how relative URIs should be interpreted by serializers.
*
*
* See {@link UriResolution} for possible values.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#uriRelativity(UriRelativity)}
*
*
* @return The annotation value.
*/
String uriRelativity() default "";
/**
* URI-resolution.
*
*
* Specifies how relative URIs should be interpreted by serializers.
*
*
* See {@link UriResolution} for possible values.
*
*
Notes:
* -
* Supports SVL Variables
* (e.g.
"$L{my.localized.variable}" ).
*
*
* See Also:
* - {@link org.apache.juneau.rest.RestContext.Builder#uriResolution(UriResolution)}
*
*
* @return The annotation value.
*/
String uriResolution() default "";
}