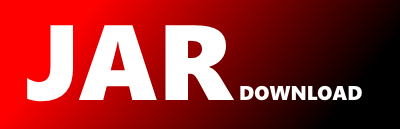
org.apache.juneau.rest.annotation.RestInject Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.annotation;
import static java.lang.annotation.ElementType.*;
import static java.lang.annotation.RetentionPolicy.*;
import java.lang.annotation.*;
import java.util.logging.*;
import org.apache.juneau.*;
import org.apache.juneau.config.*;
import org.apache.juneau.cp.*;
import org.apache.juneau.encoders.*;
import org.apache.juneau.http.header.*;
import org.apache.juneau.http.part.*;
import org.apache.juneau.httppart.*;
import org.apache.juneau.jsonschema.*;
import org.apache.juneau.parser.*;
import org.apache.juneau.rest.*;
import org.apache.juneau.rest.arg.*;
import org.apache.juneau.rest.converter.*;
import org.apache.juneau.rest.debug.*;
import org.apache.juneau.rest.guard.*;
import org.apache.juneau.rest.httppart.*;
import org.apache.juneau.rest.logger.*;
import org.apache.juneau.rest.matcher.*;
import org.apache.juneau.rest.processor.*;
import org.apache.juneau.rest.staticfile.*;
import org.apache.juneau.rest.stats.*;
import org.apache.juneau.rest.swagger.*;
import org.apache.juneau.rest.util.*;
import org.apache.juneau.serializer.*;
import org.apache.juneau.svl.*;
/**
* Rest bean injection annotation.
*
*
* Used on methods of {@link Rest}-annotated classes to denote methods and fields that override and customize beans
* used by the REST framework.
*
*
Example
*
* // Rest resource that uses a customized call logger.
* @Rest
* public class MyRest extends BasicRestServlet {
*
* // Option #1: As a field.
* @RestInject
* CallLogger myCallLogger = CallLogger.create ().logger("mylogger" ).build();
*
* // Option #2: As a method.
* @RestInject
* public CallLogger myCallLogger() {
* return CallLogger.create ().logger("mylogger" ).build();
* }
* }
*
*
*
* The {@link RestInject#name()}/{@link RestInject#value()} attributes are used to differentiate between named beans.
*
* Example
*
* // Customized default request headers.
* @RestInject ("defaultRequestHeaders" )
* HeaderList defaultRequestHeaders = HeaderList.create ().set(ContentType.TEXT_PLAIN ).build();
*
* // Customized default response headers.
* @RestInject ("defaultResponseHeaders" )
* HeaderList defaultResponseHeaders = HeaderList.create ().set(ContentType.TEXT_PLAIN ).build();
*
*
*
* The {@link RestInject#methodScope()} attribute is used to define beans in the scope of specific {@link RestOp}-annotated methods.
*
* Example
*
* // Set a default header on a specific REST method.
* // Input parameter is the default header list builder with all annotations applied.
* @RestInject (name="defaultRequestHeaders" , methodScope="myRestMethod" )
* public HeaderList.Builder myRequestHeaders(HeaderList.Builder builder ) {
* return builder .set(ContentType.TEXT_PLAIN );
* }
*
* // Method that picks up default header defined above.
* @RestGet
* public Object myRestMethod(ContentType contentType ) { ... }
*
*
*
* This annotation can also be used to inject arbitrary beans into the bean store which allows them to be
* passed as resolved parameters on {@link RestOp}-annotated methods.
*
* Example
*
* // Custom beans injected into the bean store.
* @RestInject MyBean myBean1 = new MyBean();
* @RestInject ("myBean2" ) MyBean myBean2 = new MyBean();
*
* // Method that uses injected beans.
* @RestGet
* public Object doGet(MyBean myBean1 , @Named ("myBean2" ) MyBean myBean2 ) { ... }
*
*
*
* This annotation can also be used on uninitialized fields. When fields are uninitialized, they will
* be set during initialization based on beans found in the bean store.
*
* Example
*
* // Fields that get set during initialization based on beans found in the bean store.
* @RestInject CallLogger callLogger ;
* @RestInject BeanStore beanStore ; // Note that BeanStore itself can be accessed this way.
*
*
* Notes:
* - Methods and fields can be static or non-static.
*
- Any injectable beans (including spring beans) can be passed as arguments into methods.
*
- Bean names are required when multiple beans of the same type exist in the bean store.
*
- By default, the injected bean scope is class-level (applies to the entire class). The
* {@link RestInject#methodScope()} annotation can be used to apply to method-level only (when applicable).
*
*
*
* Any of the following types can be customized via injection:
*
* Bean class Bean qualifying names Scope
* {@link BeanContext}
{@link org.apache.juneau.BeanContext.Builder} class
method
* {@link BeanStore}
{@link org.apache.juneau.cp.BeanStore.Builder} class
* {@link CallLogger}
{@link org.apache.juneau.rest.logger.CallLogger.Builder} class
* {@link Config} class
* {@link DebugEnablement}
{@link org.apache.juneau.rest.debug.DebugEnablement.Builder} class
* {@link EncoderSet}
{@link org.apache.juneau.encoders.EncoderSet.Builder} class
method
* {@link FileFinder}
{@link org.apache.juneau.cp.FileFinder.Builder} class
* {@link HeaderList}
{@link org.apache.juneau.http.header.HeaderList} "defaultRequestHeaders"
"defaultResponseHeaders" class
method
* {@link HttpPartParser}
{@link org.apache.juneau.httppart.HttpPartParser.Creator} class
method
* {@link HttpPartSerializer}
{@link org.apache.juneau.httppart.HttpPartSerializer.Creator} class
method
* {@link JsonSchemaGenerator}
{@link org.apache.juneau.jsonschema.JsonSchemaGenerator.Builder} class
method
* {@link Logger} class
* {@link Messages}
{@link org.apache.juneau.cp.Messages.Builder} class
* {@link MethodExecStore}
{@link org.apache.juneau.rest.stats.MethodExecStore.Builder} class
* {@link MethodList} "destroyMethods"
"endCallMethods"
"postCallMethods"
"postInitChildFirstMethods"
"postInitMethods"
"preCallMethods"
"startCallMethods" class
* {@link NamedAttributeMap}
{@link org.apache.juneau.rest.httppart.NamedAttributeMap} "defaultRequestAttributes" class
method
* {@link ParserSet}
{@link org.apache.juneau.parser.ParserSet.Builder} class
method
* {@link PartList}
{@link org.apache.juneau.http.part.PartList} "defaultRequestQueryData"
"defaultRequestFormData" method
* {@link ResponseProcessorList}
{@link org.apache.juneau.rest.processor.ResponseProcessorList.Builder} class
* {@link RestChildren}
{@link org.apache.juneau.rest.RestChildren.Builder} class
* {@link RestConverterList}
{@link org.apache.juneau.rest.converter.RestConverterList.Builder} method
* {@link RestGuardList}
{@link org.apache.juneau.rest.guard.RestGuardList.Builder} method
* {@link RestMatcherList}
{@link org.apache.juneau.rest.matcher.RestMatcherList.Builder} method
* {@link RestOpArgList}
{@link org.apache.juneau.rest.arg.RestOpArgList.Builder} class
* {@link RestOperations}
{@link org.apache.juneau.rest.RestOperations.Builder} class
* {@link SerializerSet}
{@link org.apache.juneau.serializer.SerializerSet.Builder} class
method
* {@link StaticFiles}
{@link org.apache.juneau.rest.staticfile.StaticFiles.Builder} class
* {@link SwaggerProvider}
{@link org.apache.juneau.rest.swagger.SwaggerProvider.Builder} class
* {@link ThrownStore}
{@link org.apache.juneau.rest.stats.ThrownStore.Builder} class
* {@link UrlPathMatcherList} method
* {@link VarList} class
* {@link VarResolver}
{@link org.apache.juneau.svl.VarResolver.Builder} class
*
*/
@Target({METHOD,FIELD})
@Retention(RUNTIME)
@Inherited
public @interface RestInject {
/**
* The bean name to use to distinguish beans of the same type for different purposes.
*
*
* For example, there are two {@link HeaderList} beans: "defaultRequestHeaders" and "defaultResponseHeaders" . This annotation
* would be used to differentiate between them.
*
* @return The bean name to use to distinguish beans of the same type for different purposes, or blank if bean type is unique.
*/
String name() default "";
/**
* Same as {@link #name()}.
*
* @return The bean name to use to distinguish beans of the same type for different purposes, or blank if bean type is unique.
*/
String value() default "";
/**
* The short names of the methods that this annotation applies to.
*
*
* Can use "*" to apply to all methods.
*
*
* Ignored for class-level scope.
*
* @return The short names of the methods that this annotation applies to, or empty if class-scope.
*/
String[] methodScope() default {};
}