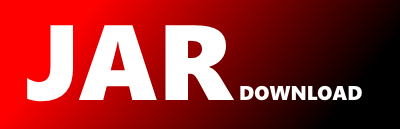
org.apache.juneau.rest.annotation.Swagger Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.annotation;
import static java.lang.annotation.RetentionPolicy.*;
import java.lang.annotation.*;
import org.apache.juneau.annotation.*;
import org.apache.juneau.http.annotation.*;
/**
* Extended annotation for {@link Rest#swagger() @Rest(swagger)}.
*
* See Also:
* - Swagger
*
*/
@Retention(RUNTIME)
public @interface Swagger {
/**
* Defines the swagger field /info/contact .
*
*
* A Swagger string with the following fields:
*
* {
* name: string,
* url: string,
* email: string
* }
*
*
*
* The default value pulls the description from the contact entry in the servlet resource bundle.
* (e.g. "contact = {name:'John Smith',email:'[email protected]'}" or
* "MyServlet.contact = {name:'John Smith',email:'[email protected]'}" ).
*
*
Example:
*
* @Rest (
* swagger=@Swagger (
* contact="{name:'John Smith',email:'[email protected]'}"
* )
* )
*
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
* -
* Supports SVL Variables (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
Contact contact() default @Contact;
/**
* Defines the swagger field /info/description .
*
* Example:
*
* @Rest (
* swagger=@Swagger (
* description={
* "This is a sample server Petstore server based on the Petstore sample at Swagger.io." ,
* "You can find out more about Swagger at <a class='link' href='http://swagger.io'>http://swagger.io</a>."
* }
* )
* )
*
*
* Notes:
* -
* The format is plain text.
*
Multiple lines are concatenated with newlines.
* -
* The precedence of lookup for this field is:
*
* {resource-class}.description property in resource bundle.
* - {@link Swagger#description()} on this class, then any parent classes.
*
- Value defined in Swagger JSON file.
*
- {@link Rest#description()} on this class, then any parent classes.
*
* -
* Supports SVL Variables (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
String[] description() default {};
/**
* Defines the swagger field /externalDocs .
*
*
* It is used to populate the Swagger external documentation field and to display on HTML pages.
* *
*
* The default value pulls the description from the externalDocs entry in the servlet resource bundle.
* (e.g. "externalDocs = {url:'http://juneau.apache.org'}" or
* "MyServlet.externalDocs = {url:'http://juneau.apache.org'}" ).
*
*
Example:
*
* @Rest (
* swagger=@Swagger (
* externalDocs=@ExternalDocs (url="http://juneau.apache.org" )
* )
* )
*
*
* @return The annotation value.
*/
ExternalDocs externalDocs() default @ExternalDocs;
/**
* Defines the swagger field /info/license .
*
*
* It is used to populate the Swagger license field and to display on HTML pages.
*
*
* A Swagger string with the following fields:
*
* {
* name: string,
* url: string
* }
*
*
*
* The default value pulls the description from the license entry in the servlet resource bundle.
* (e.g. "license = {name:'Apache 2.0',url:'http://www.apache.org/licenses/LICENSE-2.0.html'}" or
* "MyServlet.license = {name:'Apache 2.0',url:'http://www.apache.org/licenses/LICENSE-2.0.html'}" ).
*
*
Example:
*
* @Rest (
* swagger=@Swagger (
* license="{name:'Apache 2.0',url:'http://www.apache.org/licenses/LICENSE-2.0.html'}"
* )
* )
*
*
* Notes:
* -
* The format is a Swagger object.
*
Multiple lines are concatenated with newlines.
* -
* Supports SVL Variables (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
License license() default @License;
/**
* Defines the swagger field /tags .
*
*
* Optional tagging information for the exposed API.
*
*
* It is used to populate the Swagger tags field and to display on HTML pages.
*
*
* A Swagger string with the following fields:
*
* [
* {
* name: string,
* description: string,
* externalDocs: {
* description: string,
* url: string
* }
* }
* ]
*
*
*
* The default value pulls the description from the tags entry in the servlet resource bundle.
* (e.g. "tags = [{name:'Foo',description:'Foobar'}]" or
* "MyServlet.tags = [{name:'Foo',description:'Foobar'}]" ).
*
*
Example:
*
* @Rest (
* swagger=@Swagger (
* tags="[{name:'Foo',description:'Foobar'}]"
* )
* )
*
*
* Notes:
* -
* The format is a Swagger array.
*
Multiple lines are concatenated with newlines.
* -
* Supports SVL Variables (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
Tag[] tags() default {};
/**
* Defines the swagger field /info/termsOfService .
*
*
* Optional servlet terms-of-service for this API.
*
*
* It is used to populate the Swagger terms-of-service field.
*
*
* The default value pulls the description from the termsOfService entry in the servlet resource bundle.
* (e.g. "termsOfService = foo" or "MyServlet.termsOfService = foo" ).
*
*
Notes:
* -
* The format is plain text.
*
Multiple lines are concatenated with newlines.
* -
* Supports SVL Variables (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
String[] termsOfService() default {};
/**
* Defines the swagger field /info/title .
*
* Example:
*
* @Rest (
* swagger=@Swagger (
* title="Petstore application"
* )
* )
*
*
* Notes:
* -
* The format is plain-text.
*
Multiple lines are concatenated with newlines.
* -
* The precedence of lookup for this field is:
*
* {resource-class}.title property in resource bundle.
* - {@link Swagger#title()} on this class, then any parent classes.
*
- Value defined in Swagger JSON file.
*
- {@link Rest#title()} on this class, then any parent classes.
*
* -
* Supports SVL Variables (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
String[] title() default {};
/**
* Free-form value for the swagger of a resource.
*
*
* This is a Swagger object that makes up the swagger information for this resource.
*
*
* The following are completely equivalent ways of defining the swagger description of a resource:
*
* // Normal
* @Rest (
* swagger=@Swagger (
* title="Petstore application" ,
* description={
* "This is a sample server Petstore server based on the Petstore sample at Swagger.io." ,
* "You can find out more about Swagger at <a class='link' href='http://swagger.io'>http://swagger.io</a>."
* },
* contact=@Contact (
* name="John Smith" ,
* email="[email protected]"
* ),
* license=@License (
* name="Apache 2.0" ,
* url="http://www.apache.org/licenses/LICENSE-2.0.html"
* ),
* version="2.0" ,
* termsOfService="You are on your own." ,
* tags={
* @Tag (
* name="Java" ,
* description="Java utility" ,
* externalDocs=@ExternalDocs (
* description="Home page" ,
* url="http://juneau.apache.org"
* )
* }
* },
* externalDocs=@ExternalDocs (
* description="Home page" ,
* url="http://juneau.apache.org"
* )
* )
* )
*
*
* // Free-form
* @Rest (
* swagger=@Swagger({
* "title: 'Petstore application'," ,
* "description: 'This is a sample server Petstore server based on the Petstore sample at Swagger.io.\nYou can find out more about Swagger at <a class='link' href='http://swagger.io'>http://swagger.io</a>.'," ,
* "contact:{" ,
* "name: 'John Smith'," ,
* "email: '[email protected]'" ,
* "}," ,
* "license:{" ,
* "name: 'Apache 2.0'," ,
* "url: 'http://www.apache.org/licenses/LICENSE-2.0.html'" ,
* "}," ,
* "version: '2.0'," ,
* "termsOfService: 'You are on your own.'," ,
* "tags:[" ,
* "{" ,
* "name: 'Java'," ,
* "description: 'Java utility'," ,
* "externalDocs:{" ,
* "description: 'Home page'," ,
* "url: 'http://juneau.apache.org'" ,
* "}" ,
* "}" ,
* "]," ,
* "externalDocs:{" ,
* "description: 'Home page'," ,
* "url: 'http://juneau.apache.org'" ,
* "}"
* })
* )
*
*
* // Free-form with variables
* @Rest (
* swagger=@Swagger("$F{MyResourceSwagger.json}" )
* )
*
*
*
* The reasons why you may want to use this field include:
*
* - You want to pull in the entire Swagger JSON definition for this content from an external source such as a properties file.
*
- You want to add extra fields to the Swagger documentation that are not officially part of the Swagger specification.
*
*
* Notes:
* -
* The format is a Swagger object.
*
-
* The leading/trailing
{ } characters are optional.
*
The following two example are considered equivalent:
*
* @Swagger ("{title:'Petstore application'}" )
*
*
* @Swagger ("title:'Petstore application'" )
*
* -
* Multiple lines are concatenated with newlines so that you can format the value to be readable.
*
-
* Supports SVL Variables (e.g.
"$L{my.localized.variable}" ).
* -
* Values defined in this field supersede values pulled from the Swagger JSON file and are superseded by individual values defined on this annotation.
*
*
* @return The annotation value.
*/
String[] value() default {};
/**
* Defines the swagger field /info/version .
*
*
*
* Provides the version of the application API (not to be confused with the specification version).
*
*
* It is used to populate the Swagger version field and to display on HTML pages.
*
*
* The default value pulls the description from the version entry in the servlet resource bundle.
* (e.g. "version = 2.0" or "MyServlet.version = 2.0" ).
*
*
Notes:
* -
* The format is plain text.
*
-
* Supports SVL Variables (e.g.
"$L{my.localized.variable}" ).
*
*
* @return The annotation value.
*/
String version() default "";
}