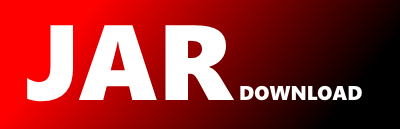
org.apache.juneau.rest.httppart.RequestAttributes Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.httppart;
import static org.apache.juneau.common.internal.ArgUtils.*;
import static org.apache.juneau.internal.CollectionUtils.*;
import java.util.*;
import jakarta.servlet.http.*;
import org.apache.juneau.collections.*;
import org.apache.juneau.rest.*;
import org.apache.juneau.svl.*;
/**
* Represents the attributes in an HTTP request.
*
*
* The {@link RequestAttributes} object is the API for accessing the standard servlet attributes on an HTTP request
* (i.e. {@link jakarta.servlet.ServletRequest#getAttribute(String)}).
*
*
*
* @RestPost (...)
* public Object myMethod(RequestAttributes attributes ) {...}
*
*
* Example:
*
* @RestPost (...)
* public Object myMethod(RequestAttributes attributes ) {
*
* // Add a default value.
* attributes .addDefault("Foo" , 123);
*
* // Get an attribute value as a POJO.
* UUID etag = attributes .get("ETag" ).as(UUID.class ).orElse(null );
* }
*
*
*
* Some important methods on this class are:
*
*
* - {@link RequestHeaders}
*
* - Methods for retrieving request attributes:
*
* - {@link RequestAttributes#contains(String...) contains(String...)}
*
- {@link RequestAttributes#containsAny(String...) containsAny(String...)}
*
- {@link RequestAttributes#get(String) get(String)}
*
- {@link RequestAttributes#getAll() getAll()}
*
* - Methods for overriding request attributes:
*
* - {@link RequestAttributes#addDefault(List) addDefault(List)}
*
- {@link RequestAttributes#addDefault(NamedAttribute...) addDefault(NamedAttribute...)}
*
- {@link RequestAttributes#addDefault(NamedAttributeMap) addDefault(NamedAttributeMap)}
*
- {@link RequestAttributes#addDefault(String,Object) addDefault(String,Object)}
*
- {@link RequestAttributes#remove(NamedAttribute...) remove(NamedAttribute...)}
*
- {@link RequestAttributes#remove(String...) remove(String...)}
*
- {@link RequestAttributes#set(NamedAttribute...) set(NamedAttribute...)}
*
- {@link RequestAttributes#set(String,Object) set(String,Object)}
*
* - Other methods:
*
* - {@link RequestAttributes#asMap() asMap()}
*
*
*
*
*
* Modifications made to request attributes through the RequestAttributes bean are automatically reflected in
* the underlying servlet request attributes making it possible to mix the usage of both APIs.
*
*
* See Also:
* - HTTP Parts
*
*/
public class RequestAttributes {
final RestRequest req;
final HttpServletRequest sreq;
final VarResolverSession vs;
/**
* Constructor.
*
* @param req The request creating this bean.
*/
public RequestAttributes(RestRequest req) {
this.req = req;
this.sreq = req.getHttpServletRequest();
this.vs = req.getVarResolverSession();
}
/**
* Adds default entries to the request attributes.
*
* @param pairs
* The default entries.
*
Can be null .
* @return This object.
*/
public RequestAttributes addDefault(List pairs) {
for (NamedAttribute p : pairs)
if (sreq.getAttribute(p.getName()) == null) {
Object o = p.getValue();
sreq.setAttribute(p.getName(), o instanceof String ? vs.resolve(o) : o);
}
return this;
}
/**
* Adds default entries to the request attributes.
*
* @param pairs
* The default entries.
*
Can be null .
* @return This object.
*/
public RequestAttributes addDefault(NamedAttributeMap pairs) {
for (NamedAttribute p : pairs.values())
if (sreq.getAttribute(p.getName()) == null) {
Object o = p.getValue();
sreq.setAttribute(p.getName(), o instanceof String ? vs.resolve(o) : o);
}
return this;
}
/**
* Adds default entries to the request attributes.
*
* @param pairs
* The default entries.
*
Can be null .
* @return This object.
*/
public RequestAttributes addDefault(NamedAttribute...pairs) {
return addDefault(alist(pairs));
}
/**
* Adds a default entry to the request attributes.
*
* @param name The name.
* @param value The value.
* @return This object.
*/
public RequestAttributes addDefault(String name, Object value) {
return addDefault(BasicNamedAttribute.of(name, value));
}
/**
* Returns the request attribute with the specified name.
*
* @param name The attribute name.
* @return The parameter value, or {@link Optional#empty()} if it doesn't exist.
*/
public RequestAttribute get(String name) {
return new RequestAttribute(req, name, sreq.getAttribute(name));
}
/**
* Returns all the attribute on this request.
*
* @return All the attribute on this request.
*/
public List getAll() {
List l = list();
Enumeration e = sreq.getAttributeNames();
while (e.hasMoreElements()) {
String n = e.nextElement();
l.add(new RequestAttribute(req, n, sreq.getAttribute(n)));
}
return l;
}
/**
* Returns true if the attributes with the specified names are present.
*
* @param names The attribute names. Must not be null .
* @return true if the parameters with the specified names are present.
*/
public boolean contains(String...names) {
assertArgNotNull("names", names);
for (String n : names)
if (sreq.getAttribute(n) == null)
return false;
return true;
}
/**
* Returns true if the attribute with any of the specified names are present.
*
* @param names The attribute names. Must not be null .
* @return true if the attribute with any of the specified names are present.
*/
public boolean containsAny(String...names) {
assertArgNotNull("names", names);
for (String n : names)
if (sreq.getAttribute(n) != null)
return true;
return false;
}
/**
* Sets a request attribute.
*
* @param name The attribute name. Must not be null .
* @param value
* The attribute value.
*
Can be null .
* @return This object.
*/
public RequestAttributes set(String name, Object value) {
assertArgNotNull("name", name);
sreq.setAttribute(name, value);
return this;
}
/**
* Sets request attributes.
*
* @param attributes The parameters to set. Must not be null or contain null .
* @return This object.
*/
public RequestAttributes set(NamedAttribute...attributes) {
assertArgNotNull("attributes", attributes);
for (NamedAttribute p : attributes)
set(p);
return this;
}
/**
* Remove request attributes.
*
* @param name The attribute names. Must not be null .
* @return This object.
*/
public RequestAttributes remove(String...name) {
assertArgNotNull("name", name);
for (String n : name) {
sreq.removeAttribute(n);
}
return this;
}
/**
* Remove request attributes.
*
* @param attributes The attributes to remove. Must not be null .
* @return This object.
*/
public RequestAttributes remove(NamedAttribute...attributes) {
for (NamedAttribute p : attributes)
remove(p.getName());
return this;
}
/**
* Returns the request attributes as a map.
*
* @return The request attributes as a map. Never null .
*/
public Map asMap() {
JsonMap m = new JsonMap();
Enumeration e = sreq.getAttributeNames();
while (e.hasMoreElements()) {
String n = e.nextElement();
m.put(n, sreq.getAttribute(n));
}
return m;
}
@Override /* Object */
public String toString() {
return asMap().toString();
}
}