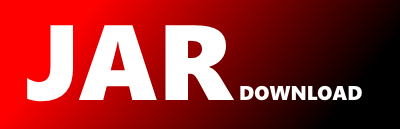
org.apache.juneau.rest.httppart.RequestPathParams Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.httppart;
import static org.apache.juneau.internal.ClassUtils.*;
import static org.apache.juneau.internal.CollectionUtils.*;
import static java.util.stream.Collectors.*;
import static org.apache.juneau.common.internal.ArgUtils.*;
import static org.apache.juneau.common.internal.StringUtils.*;
import static org.apache.juneau.httppart.HttpPartType.*;
import java.util.*;
import java.util.stream.*;
import org.apache.http.*;
import org.apache.juneau.httppart.*;
import org.apache.juneau.rest.*;
import org.apache.juneau.rest.util.*;
import org.apache.juneau.svl.*;
import org.apache.juneau.*;
import org.apache.juneau.collections.*;
import org.apache.juneau.common.internal.*;
import org.apache.juneau.http.*;
import org.apache.juneau.http.part.*;
/**
* Represents the path parameters in an HTTP request.
*
*
* The {@link RequestPathParams} object is the API for accessing the matched variables
* and remainder on the URL path.
*
*
* @RestPost (...)
* public Object myMethod(RequestPathParams path ) {...}
*
*
* Example:
*
* @RestPost (..., path="/{foo}/{bar}/{baz}/*" )
* public void doGet(RequestPathParams path ) {
* // Example URL: /123/qux/true/quux
*
* int foo = path .get("foo" ).asInteger().orElse(0); // =123
* String bar = path .get("bar" ).orElse(null ); // =qux
* boolean baz = path .get("baz" ).asBoolean().orElse(false ); // =true
* String remainder = path .getRemainder(); // =quux
* }
*
*
*
* Some important methods on this class are:
*
*
* - {@link RequestPathParams}
*
* - Methods for retrieving path parameters:
*
* - {@link RequestPathParams#contains(String) contains(String)}
*
- {@link RequestPathParams#containsAny(String...) containsAny(String...)}
*
- {@link RequestPathParams#get(Class) get(Class)}
*
- {@link RequestPathParams#get(String) get(String)}
*
- {@link RequestPathParams#getAll(String) getAll(String)}
*
- {@link RequestPathParams#getFirst(String) getFirst(String)}
*
- {@link RequestPathParams#getLast(String) getLast(String)}
*
- {@link RequestPathParams#getRemainder() getRemainder()}
*
- {@link RequestPathParams#getRemainderUndecoded() getRemainderUndecoded()}
*
* - Methods overridding path parameters:
*
* - {@link RequestPathParams#add(NameValuePair...) add(NameValuePair...)}
*
- {@link RequestPathParams#add(String,Object) add(String,Object)}
*
- {@link RequestPathParams#addDefault(List) addDefault(List)}
*
- {@link RequestPathParams#addDefault(NameValuePair...) addDefault(NameValuePair...)}
*
- {@link RequestPathParams#remove(String) remove(String)}
*
- {@link RequestPathParams#set(NameValuePair...) set(NameValuePair...)}
*
- {@link RequestPathParams#set(String,Object) set(String,Object)}
*
* - Other methods:
*
* - {@link RequestPathParams#copy() copy()}
*
- {@link RequestPathParams#isEmpty() isEmpty()}
*
*
*
*
* See Also:
* - {@link RequestPathParam}
*
- {@link org.apache.juneau.http.annotation.Path}
*
- HTTP Parts
*
*/
public class RequestPathParams extends ArrayList {
private static final long serialVersionUID = 1L;
private final RestRequest req;
private boolean caseSensitive;
private HttpPartParserSession parser;
private final VarResolverSession vs;
/**
* Constructor.
*
* @param session The current HTTP request session.
* @param req The current HTTP request.
* @param caseSensitive Whether case-sensitive name matching is enabled.
*/
public RequestPathParams(RestSession session, RestRequest req, boolean caseSensitive) {
this.req = req;
this.caseSensitive = caseSensitive;
this.vs = req.getVarResolverSession();
// Add parameters from parent context if any.
@SuppressWarnings("unchecked")
Map parentVars = (Map)req.getAttribute("juneau.pathVars").orElse(Collections.emptyMap());
for (Map.Entry e : parentVars.entrySet())
add(e.getKey(), e.getValue());
UrlPathMatch pm = session.getUrlPathMatch();
if (pm != null) {
for (Map.Entry e : pm.getVars().entrySet())
add(e.getKey(), e.getValue());
String r = pm.getRemainder();
if (r != null) {
add("/**", r);
add("/*", urlDecode(r));
}
}
}
/**
* Copy constructor.
*/
private RequestPathParams(RequestPathParams copyFrom) {
req = copyFrom.req;
caseSensitive = copyFrom.caseSensitive;
parser = copyFrom.parser;
addAll(copyFrom);
vs = copyFrom.vs;
}
/**
* Subset constructor.
*/
private RequestPathParams(RequestPathParams copyFrom, String...names) {
this.req = copyFrom.req;
caseSensitive = copyFrom.caseSensitive;
parser = copyFrom.parser;
vs = copyFrom.vs;
for (String n : names)
copyFrom.stream().filter(x -> eq(x.getName(), n)).forEach(this::add);
}
/**
* Sets the parser to use for part values.
*
* @param value The new value for this setting.
* @return This object.
*/
public RequestPathParams parser(HttpPartParserSession value) {
this.parser = value;
forEach(x -> x.parser(parser));
return this;
}
/**
* Sets case sensitivity for names in this list.
*
* @param value The new value for this setting.
* @return This object (for method chaining).
*/
public RequestPathParams caseSensitive(boolean value) {
this.caseSensitive = value;
return this;
}
//-----------------------------------------------------------------------------------------------------------------
// Basic operations.
//-----------------------------------------------------------------------------------------------------------------
/**
* Adds default entries to these parameters.
*
*
* Similar to {@link #set(String, Object)} but doesn't override existing values.
*
* @param pairs
* The default entries.
*
Can be null .
* @return This object.
*/
public RequestPathParams addDefault(List pairs) {
for (NameValuePair p : pairs) {
String name = p.getName();
Stream l = stream(name);
boolean hasAllBlanks = l.allMatch(x -> StringUtils.isEmpty(x.getValue()));
if (hasAllBlanks) {
removeAll(getAll(name));
add(new RequestPathParam(req, name, vs.resolve(p.getValue())));
}
}
return this;
}
/**
* Adds default entries to these parameters.
*
*
* Similar to {@link #set(String, Object)} but doesn't override existing values.
*
* @param pairs
* The default entries.
*
Can be null .
* @return This object.
*/
public RequestPathParams addDefault(NameValuePair...pairs) {
return addDefault(alist(pairs));
}
/**
* Adds a default entry to the query parameters.
*
* @param name The name.
* @param value The value.
* @return This object.
*/
public RequestPathParams addDefault(String name, String value) {
return addDefault(BasicStringPart.of(name, value));
}
/**
* Adds a parameter value.
*
*
* Parameter is added to the end.
*
Existing parameter with the same name are not changed.
*
* @param name The parameter name. Must not be null .
* @param value The parameter value.
* @return This object.
*/
public RequestPathParams add(String name, Object value) {
assertArgNotNull("name", name);
add(new RequestPathParam(req, name, stringify(value)).parser(parser));
return this;
}
/**
* Adds request parameter values.
*
*
* Parameters are added to the end.
*
Existing parameters with the same name are not changed.
*
* @param parameters The parameter objects. Must not be null .
* @return This object.
*/
public RequestPathParams add(NameValuePair...parameters) {
assertArgNotNull("parameters", parameters);
for (NameValuePair p : parameters)
if (p != null)
add(p.getName(), p.getValue());
return this;
}
/**
* Sets a parameter value.
*
*
* Parameter is added to the end.
*
Any previous parameters with the same name are removed.
*
* @param name The parameter name. Must not be null .
* @param value
* The parameter value.
*
Converted to a string using {@link Object#toString()}.
*
Can be null .
* @return This object.
*/
public RequestPathParams set(String name, Object value) {
assertArgNotNull("name", name);
set(new RequestPathParam(req, name, stringify(value)).parser(parser));
return this;
}
/**
* Sets request header values.
*
*
* Parameters are added to the end of the headers.
*
Any previous parameters with the same name are removed.
*
* @param parameters The parameters to set. Must not be null or contain null .
* @return This object.
*/
public RequestPathParams set(NameValuePair...parameters) {
assertArgNotNull("headers", parameters);
for (NameValuePair p : parameters)
remove(p);
for (NameValuePair p : parameters)
add(p);
return this;
}
/**
* Remove parameters.
*
* @param name The parameter name. Must not be null .
* @return This object.
*/
public RequestPathParams remove(String name) {
assertArgNotNull("name", name);
removeIf(x -> eq(x.getName(), name));
return this;
}
/**
* Returns a copy of this object but only with the specified param names copied.
*
* @param names The list to include in the copy.
* @return A new list object.
*/
public RequestPathParams subset(String...names) {
return new RequestPathParams(this, names);
}
//-----------------------------------------------------------------------------------------------------------------
// Convenience getters.
//-----------------------------------------------------------------------------------------------------------------
/**
* Returns true if the parameters with the specified name is present.
*
* @param name The parameter name. Must not be null .
* @return true if the parameters with the specified name is present.
*/
public boolean contains(String name) {
assertArgNotNull("names", name);
return stream(name).findAny().isPresent();
}
/**
* Returns true if the parameter with any of the specified names are present.
*
* @param names The parameter names. Must not be null .
* @return true if the parameter with any of the specified names are present.
*/
public boolean containsAny(String...names) {
assertArgNotNull("names", names);
for (String n : names)
if (stream(n).findAny().isPresent())
return true;
return false;
}
/**
* Returns all the parameters with the specified name.
*
* @param name The parameter name.
* @return The list of all parameters with the specified name, or an empty list if none are found.
*/
public List getAll(String name) {
assertArgNotNull("name", name);
return stream(name).collect(toList());
}
/**
* Returns all headers with the specified name.
*
* @param name The header name.
* @return The stream of all headers with matching names. Never null .
*/
public Stream stream(String name) {
return stream().filter(x -> eq(x.getName(), name));
}
/**
* Returns all headers in sorted order.
*
* @return The stream of all headers in sorted order.
*/
public Stream getSorted() {
Comparator x;
if (caseSensitive)
x = Comparator.comparing(RequestPathParam::getName);
else
x = (x1,x2) -> String.CASE_INSENSITIVE_ORDER.compare(x1.getName(), x2.getName());
return stream().sorted(x);
}
/**
* Returns all the unique header names in this list.
* @return The list of all unique header names in this list.
*/
public List getNames() {
return stream().map(RequestPathParam::getName).map(x -> caseSensitive ? x : x.toLowerCase()).distinct().collect(toList());
}
/**
* Returns the first parameter with the specified name.
*
*
* Note that this method never returns null and that {@link RequestPathParam#isPresent()} can be used
* to test for the existence of the parameter.
*
* @param name The parameter name.
* @return The parameter. Never null .
*/
public RequestPathParam getFirst(String name) {
assertArgNotNull("name", name);
return stream(name).findFirst().orElseGet(()->new RequestPathParam(req, name, null).parser(parser));
}
/**
* Returns the last parameter with the specified name.
*
*
* Note that this method never returns null and that {@link RequestPathParam#isPresent()} can be used
* to test for the existence of the parameter.
*
* @param name The parameter name.
* @return The parameter. Never null .
*/
public RequestPathParam getLast(String name) {
assertArgNotNull("name", name);
Value v = Value.empty();
stream(name).forEach(x -> v.set(x));
return v.orElseGet(() -> new RequestPathParam(req, name, null).parser(parser));
}
/**
* Returns the last parameter with the specified name.
*
*
* This is equivalent to {@link #getLast(String)}.
*
* @param name The parameter name.
* @return The parameter value, or {@link Optional#empty()} if it doesn't exist.
*/
public RequestPathParam get(String name) {
List l = getAll(name);
if (l.isEmpty())
return new RequestPathParam(req, name, null).parser(parser);
if (l.size() == 1)
return l.get(0);
StringBuilder sb = new StringBuilder(128);
for (int i = 0, j = l.size(); i < j; i++) {
if (i > 0)
sb.append(", ");
sb.append(l.get(i).getValue());
}
return new RequestPathParam(req, name, sb.toString()).parser(parser);
}
/**
* Returns the path parameter as the specified bean type.
*
*
* Type must have a name specified via the {@link org.apache.juneau.http.annotation.Path} annotation
* and a public constructor that takes in either value or name,value as strings.
*
* @param The bean type to create.
* @param type The bean type to create.
* @return The bean, never null .
*/
public Optional get(Class type) {
ClassMeta cm = req.getBeanSession().getClassMeta(type);
String name = HttpParts.getName(PATH, cm).orElseThrow(()->new BasicRuntimeException("@Path(name) not found on class {0}", className(type)));
return get(name).as(type);
}
//-----------------------------------------------------------------------------------------------------------------
// Other methods
//-----------------------------------------------------------------------------------------------------------------
/**
* Makes a copy of these parameters.
*
* @return A new parameters object.
*/
public RequestPathParams copy() {
return new RequestPathParams(this);
}
/**
* Returns the decoded remainder of the URL following any path pattern matches.
*
*
* The behavior of path remainder is shown below given the path pattern "/foo/*":
*
*
* URL
* Path Remainder
*
*
* /foo
* null
*
*
* /foo/
* ""
*
*
* /foo//
* "/"
*
*
* /foo///
* "//"
*
*
* /foo/a/b
* "a/b"
*
*
* /foo//a/b/
* "/a/b/"
*
*
* /foo/a%2Fb
* "a/b"
*
*
*
* Example:
*
* // REST method
* @RestGet ("/foo/{bar}/*" )
* public String doGetById(RequestPathParams path , int bar ) {
* return path .remainder().orElse(null );
* }
*
*
*
* The remainder can also be retrieved by calling get("/**" )
.
*
* @return The path remainder string.
*/
public RequestPathParam getRemainder() {
return get("/*");
}
/**
* Same as {@link #getRemainder()} but doesn't decode characters.
*
*
* The undecoded remainder can also be retrieved by calling get("/*" )
.
*
* @return The un-decoded path remainder.
*/
public RequestPathParam getRemainderUndecoded() {
return get("/**");
}
private boolean eq(String s1, String s2) {
if (caseSensitive)
return StringUtils.eq(s1, s2);
return StringUtils.eqic(s1, s2);
}
@Override /* Object */
public String toString() {
JsonMap m = new JsonMap();
for (String n : getNames())
m.put(n, get(n).asString().orElse(null));
return m.asJson();
}
}