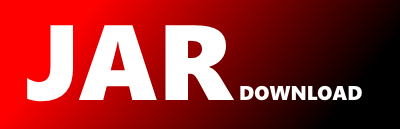
org.apache.juneau.rest.httppart.RequestQueryParams Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.httppart;
import static org.apache.juneau.internal.ClassUtils.*;
import static org.apache.juneau.internal.CollectionUtils.*;
import static org.apache.juneau.common.internal.ArgUtils.*;
import static org.apache.juneau.common.internal.StringUtils.*;
import static org.apache.juneau.httppart.HttpPartType.*;
import static java.util.Optional.*;
import static java.util.stream.Collectors.*;
import java.util.*;
import java.util.stream.*;
import org.apache.http.*;
import org.apache.juneau.httppart.*;
import org.apache.juneau.objecttools.*;
import org.apache.juneau.rest.*;
import org.apache.juneau.svl.*;
import org.apache.juneau.*;
import org.apache.juneau.collections.*;
import org.apache.juneau.common.internal.*;
import org.apache.juneau.http.*;
import org.apache.juneau.http.part.*;
/**
* Represents the query parameters in an HTTP request.
*
*
* The {@link RequestQueryParams} object is the API for accessing the GET query parameters of an HTTP request.
* It can be accessed by passing it as a parameter on your REST Java method:
*
*
* @RestPost (...)
* public Object myMethod(RequestQueryParams query ) {...}
*
*
* Example:
*
* @RestPost (...)
* public Object myMethod(RequestQueryParams query ) {
*
* // Get query parameters converted to various types.
* int p1 = query .get("p1" ).asInteger().orElse(0);
* String p2 = query .get("p2" ).orElse(null );
* UUID p3 = query .get("p3" ).as(UUID.class ).orElse(null );
* }
*
*
*
* An important distinction between the behavior of this object and HttpServletRequest.getParameter(String) is
* that the former will NOT load the content of the request on FORM POSTS and will only look at parameters
* found in the query string.
* This can be useful in cases where you're mixing GET parameters and FORM POSTS and you don't want to
* inadvertently read the content of the request to get a query parameter.
*
*
*
* Some important methods on this class are:
*
*
* - {@link RequestQueryParams}
*
* - Methods for retrieving query parameters:
*
* - {@link RequestQueryParams#contains(String) contains(String)}
*
- {@link RequestQueryParams#containsAny(String...) containsAny(String...)}
*
- {@link RequestQueryParams#get(Class) get(Class)}
*
- {@link RequestQueryParams#get(String) get(String)}
*
- {@link RequestQueryParams#getAll(String) getAll(String)}
*
- {@link RequestQueryParams#getFirst(String) getFirst(String)}
*
- {@link RequestQueryParams#getLast(String) getLast(String)}
*
- {@link RequestQueryParams#getSearchArgs() getSearchArgs()}
*
- {@link RequestQueryParams#getViewArgs() getViewArgs()}
*
- {@link RequestQueryParams#getSortArgs() getSortArgs()}
*
- {@link RequestQueryParams#getPageArgs() getPageArgs()}
*
* - Methods overridding query parameters:
*
* - {@link RequestQueryParams#add(NameValuePair...) add(NameValuePair...)}
*
- {@link RequestQueryParams#add(String,Object) add(String,Object)}
*
- {@link RequestQueryParams#addDefault(List) addDefault(List)}
*
- {@link RequestQueryParams#addDefault(NameValuePair...) addDefault(NameValuePair...)}
*
- {@link RequestQueryParams#addDefault(String,String) addDefault(String,String)}
*
- {@link RequestQueryParams#remove(String) remove(String)}
*
- {@link RequestQueryParams#set(NameValuePair...) set(NameValuePair...)}
*
- {@link RequestQueryParams#set(String,Object) set(String,Object)}
*
* - Other methods:
*
* - {@link RequestQueryParams#asQueryString() asQueryString()}
*
- {@link RequestQueryParams#copy() copy()}
*
- {@link RequestQueryParams#isEmpty() isEmpty()}
*
*
*
*
*
* Entries are stored in a case-sensitive map unless overridden via the constructor.
*
*
See Also:
* - {@link RequestQueryParam}
*
- {@link org.apache.juneau.http.annotation.Query}
*
- {@link org.apache.juneau.http.annotation.HasQuery}
*
- HTTP Parts
*
*/
public class RequestQueryParams extends ArrayList {
private static final long serialVersionUID = 1L;
private final RestRequest req;
private boolean caseSensitive;
private final VarResolverSession vs;
private HttpPartParserSession parser;
/**
* Constructor.
*
* @param req The request creating this bean.
* @param query The raw parsed query parameter values.
* @param caseSensitive Whether case-sensitive name matching is enabled.
*/
public RequestQueryParams(RestRequest req, Map query, boolean caseSensitive) {
this.req = req;
this.caseSensitive = caseSensitive;
this.vs = req.getVarResolverSession();
for (Map.Entry e : query.entrySet()) {
String name = e.getKey();
String[] values = e.getValue();
if (values == null)
values = new String[0];
// Fix for behavior difference between Tomcat and WAS.
// getParameter("foo") on "&foo" in Tomcat returns "".
// getParameter("foo") on "&foo" in WAS returns null.
if (values.length == 1 && values[0] == null)
values[0] = "";
if (values.length == 0)
values = new String[]{null};
for (String value : values)
add(new RequestQueryParam(req, name, value));
}
}
/**
* Copy constructor.
*/
private RequestQueryParams(RequestQueryParams copyFrom) {
req = copyFrom.req;
caseSensitive = copyFrom.caseSensitive;
parser = copyFrom.parser;
addAll(copyFrom);
vs = copyFrom.vs;
}
/**
* Subset constructor.
*/
private RequestQueryParams(RequestQueryParams copyFrom, String...names) {
this.req = copyFrom.req;
caseSensitive = copyFrom.caseSensitive;
parser = copyFrom.parser;
vs = copyFrom.vs;
for (String n : names)
copyFrom.stream().filter(x -> eq(x.getName(), n)).forEach(this::add);
}
/**
* Sets the parser to use for part values.
*
* @param value The new value for this setting.
* @return This object.
*/
public RequestQueryParams parser(HttpPartParserSession value) {
this.parser = value;
forEach(x -> x.parser(parser));
return this;
}
/**
* Sets case sensitivity for names in this list.
*
* @param value The new value for this setting.
* @return This object (for method chaining).
*/
public RequestQueryParams caseSensitive(boolean value) {
this.caseSensitive = value;
return this;
}
//-----------------------------------------------------------------------------------------------------------------
// Basic operations.
//-----------------------------------------------------------------------------------------------------------------
/**
* Adds default entries to these parameters.
*
*
* Similar to {@link #set(String, Object)} but doesn't override existing values.
*
* @param pairs
* The default entries.
*
Can be null .
* @return This object.
*/
public RequestQueryParams addDefault(List extends NameValuePair> pairs) {
for (NameValuePair p : pairs) {
String name = p.getName();
Stream l = stream(name);
boolean hasAllBlanks = l.allMatch(x -> StringUtils.isEmpty(x.getValue()));
if (hasAllBlanks) {
removeAll(getAll(name));
add(new RequestQueryParam(req, name, vs.resolve(p.getValue())));
}
}
return this;
}
/**
* Adds default entries to these parameters.
*
*
* Similar to {@link #set(String, Object)} but doesn't override existing values.
*
* @param pairs
* The default entries.
*
Can be null .
* @return This object.
*/
public RequestQueryParams addDefault(NameValuePair...pairs) {
return addDefault(alist(pairs));
}
/**
* Adds a default entry to the query parameters.
*
* @param name The name.
* @param value The value.
* @return This object.
*/
public RequestQueryParams addDefault(String name, String value) {
return addDefault(BasicStringPart.of(name, value));
}
/**
* Adds a parameter value.
*
*
* Parameter is added to the end.
*
Existing parameter with the same name are not changed.
*
* @param name The parameter name. Must not be null .
* @param value The parameter value.
* @return This object.
*/
public RequestQueryParams add(String name, Object value) {
assertArgNotNull("name", name);
add(new RequestQueryParam(req, name, stringify(value)).parser(parser));
return this;
}
/**
* Adds request parameter values.
*
*
* Parameters are added to the end.
*
Existing parameters with the same name are not changed.
*
* @param parameters The parameter objects. Must not be null .
* @return This object.
*/
public RequestQueryParams add(NameValuePair...parameters) {
assertArgNotNull("parameters", parameters);
for (NameValuePair p : parameters)
if (p != null)
add(p.getName(), p.getValue());
return this;
}
/**
* Sets a parameter value.
*
*
* Parameter is added to the end.
*
Any previous parameters with the same name are removed.
*
* @param name The parameter name. Must not be null .
* @param value
* The parameter value.
*
Converted to a string using {@link Object#toString()}.
*
Can be null .
* @return This object.
*/
public RequestQueryParams set(String name, Object value) {
assertArgNotNull("name", name);
set(new RequestQueryParam(req, name, stringify(value)).parser(parser));
return this;
}
/**
* Sets request header values.
*
*
* Parameters are added to the end of the headers.
*
Any previous parameters with the same name are removed.
*
* @param parameters The parameters to set. Must not be null or contain null .
* @return This object.
*/
public RequestQueryParams set(NameValuePair...parameters) {
assertArgNotNull("headers", parameters);
for (NameValuePair p : parameters)
remove(p);
for (NameValuePair p : parameters)
add(p);
return this;
}
/**
* Remove parameters.
*
* @param name The parameter names. Must not be null .
* @return This object.
*/
public RequestQueryParams remove(String name) {
assertArgNotNull("name", name);
removeIf(x -> eq(x.getName(), name));
return this;
}
/**
* Returns a copy of this object but only with the specified param names copied.
*
* @param names The list to include in the copy.
* @return A new list object.
*/
public RequestQueryParams subset(String...names) {
return new RequestQueryParams(this, names);
}
//-----------------------------------------------------------------------------------------------------------------
// Convenience getters.
//-----------------------------------------------------------------------------------------------------------------
/**
* Returns true if the parameters with the specified name is present.
*
* @param name The parameter name. Must not be null .
* @return true if the parameters with the specified names are present.
*/
public boolean contains(String name) {
return stream(name).findAny().isPresent();
}
/**
* Returns true if the parameter with any of the specified names are present.
*
* @param names The parameter names. Must not be null .
* @return true if the parameter with any of the specified names are present.
*/
public boolean containsAny(String...names) {
assertArgNotNull("names", names);
for (String n : names)
if (stream(n).findAny().isPresent())
return true;
return false;
}
/**
* Returns all the parameters with the specified name.
*
* @param name The parameter name.
* @return The list of all parameters with the specified name, or an empty list if none are found.
*/
public List getAll(String name) {
return stream(name).collect(toList());
}
/**
* Returns all headers with the specified name.
*
* @param name The header name.
* @return The stream of all headers with matching names. Never null .
*/
public Stream stream(String name) {
return stream().filter(x -> eq(x.getName(), name));
}
/**
* Returns all headers in sorted order.
*
* @return The stream of all headers in sorted order.
*/
public Stream getSorted() {
Comparator x;
if (caseSensitive)
x = Comparator.comparing(RequestQueryParam::getName);
else
x = (x1,x2) -> String.CASE_INSENSITIVE_ORDER.compare(x1.getName(), x2.getName());
return stream().sorted(x);
}
/**
* Returns all the unique header names in this list.
* @return The list of all unique header names in this list.
*/
public List getNames() {
return stream().map(RequestQueryParam::getName).map(x -> caseSensitive ? x : x.toLowerCase()).distinct().collect(toList());
}
/**
* Returns the first parameter with the specified name.
*
*
* Note that this method never returns null and that {@link RequestQueryParam#isPresent()} can be used
* to test for the existence of the parameter.
*
* @param name The parameter name.
* @return The parameter. Never null .
*/
public RequestQueryParam getFirst(String name) {
assertArgNotNull("name", name);
return stream(name).findFirst().orElseGet(()->new RequestQueryParam(req, name, null).parser(parser));
}
/**
* Returns the last parameter with the specified name.
*
*
* Note that this method never returns null and that {@link RequestQueryParam#isPresent()} can be used
* to test for the existence of the parameter.
*
* @param name The parameter name.
* @return The parameter. Never null .
*/
public RequestQueryParam getLast(String name) {
assertArgNotNull("name", name);
Value v = Value.empty();
stream(name).forEach(x -> v.set(x));
return v.orElseGet(() -> new RequestQueryParam(req, name, null).parser(parser));
}
/**
* Returns the condensed header with the specified name.
*
*
* If multiple headers are present, they will be combined into a single comma-delimited list.
*
* @param name The header name.
* @return The header, never null .
*/
public RequestQueryParam get(String name) {
List l = getAll(name);
if (l.isEmpty())
return new RequestQueryParam(req, name, null).parser(parser);
if (l.size() == 1)
return l.get(0);
StringBuilder sb = new StringBuilder(128);
for (int i = 0, j = l.size(); i < j; i++) {
if (i > 0)
sb.append(", ");
sb.append(l.get(i).getValue());
}
return new RequestQueryParam(req, name, sb.toString()).parser(parser);
}
/**
* Returns the query parameter as the specified bean type.
*
*
* Type must have a name specified via the {@link org.apache.juneau.http.annotation.Query} annotation
* and a public constructor that takes in either value or name,value as strings.
*
* @param The bean type to create.
* @param type The bean type to create.
* @return The bean, never null .
*/
public Optional get(Class type) {
ClassMeta cm = req.getBeanSession().getClassMeta(type);
String name = HttpParts.getName(QUERY, cm).orElseThrow(()->new BasicRuntimeException("@Query(name) not found on class {0}", className(type)));
return get(name).as(type);
}
//-----------------------------------------------------------------------------------------------------------------
// Other methods
//-----------------------------------------------------------------------------------------------------------------
/**
* Converts this object to a query string.
*
*
* Returned query string does not start with '?' .
*
* @return A new query string, or an empty string if this object is empty.
*/
public String asQueryString() {
StringBuilder sb = new StringBuilder();
for (RequestQueryParam e : this) {
if (sb.length() > 0)
sb.append("&");
sb.append(urlEncode(e.getName())).append('=').append(urlEncode(e.getValue()));
}
return sb.toString();
}
/**
* Makes a copy of these parameters.
*
* @return A new parameters object.
*/
public RequestQueryParams copy() {
return new RequestQueryParams(this);
}
/**
* Locates the search query argument ({@code &s=}) in the query string and returns them as a {@link SearchArgs} object.
*
* @return
* A new {@link SearchArgs} object initialized with the query arguments, or {@link Optional#empty()} if not found.
*/
public Optional getSearchArgs() {
return ofNullable(SearchArgs.create(get("s").asString().orElse(null)));
}
/**
* Locates the view query argument ({@code &v=}) in the query string and returns them as a {@link ViewArgs} object.
*
* @return
* A new {@link ViewArgs} object initialized with the query arguments, or {@link Optional#empty()} if not found.
*/
public Optional getViewArgs() {
return ofNullable(ViewArgs.create(get("v").asString().orElse(null)));
}
/**
* Locates the sort query argument ({@code &o=}) in the query string and returns them as a {@link SortArgs} object.
*
* @return
* A new {@link SortArgs} object initialized with the query arguments, or {@link Optional#empty()} if not found.
*/
public Optional getSortArgs() {
return ofNullable(SortArgs.create(get("o").asString().orElse(null)));
}
/**
* Locates the position/limit query arguments ({@code &p=}, {@code &l=}) in the query string and returns them as a {@link PageArgs} object.
*
* @return
* A new {@link PageArgs} object initialized with the query arguments, or {@link Optional#empty()} if not found.
*/
public Optional getPageArgs() {
return ofNullable(PageArgs.create(get("p").asInteger().orElse(null), get("l").asInteger().orElse(null)));
}
private boolean eq(String s1, String s2) {
if (caseSensitive)
return StringUtils.eq(s1, s2);
return StringUtils.eqic(s1, s2);
}
@Override /* Object */
public String toString() {
JsonMap m = new JsonMap();
for (String n : getNames())
m.put(n, get(n).asString().orElse(null));
return m.asJson();
}
}