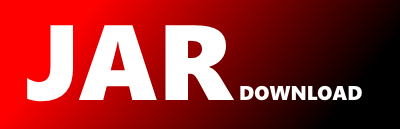
org.apache.juneau.rest.logger.BasicTestCaptureCallLogger Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.logger;
import static java.util.logging.Level.*;
import static org.apache.juneau.rest.logger.CallLoggingDetail.*;
import java.util.concurrent.atomic.*;
import java.util.logging.*;
import org.apache.juneau.assertions.*;
import org.apache.juneau.cp.*;
/**
*
* Implementation of a {@link CallLogger} that captures log entries for testing logging itself.
*
*
* Instead of logging messages to a log file, messages are simply kept in an internal atomic string reference.
* Once a message has been logged, you can use the {@link #getMessage()} or {@link #assertMessage()} methods
* to access and evaluate it.
*
*
* The following example shows how the capture logger can be associated with a REST class so that logged messages can
* be examined.
*
*
* public class MyTests {
*
* private static final CaptureLogger LOGGER = new CaptureLogger();
*
* public static class CaptureLogger extends BasicTestCaptureCallLogger {
* // How our REST class will get the logger.
* public static CaptureLogger getInstance () {
* return LOGGER ;
* }
* }
*
* @Rest (callLogger=CaptureLogger.class )
* public static class TestRest implements BasicRestServlet {
* @RestGet
* public boolean bad() throws InternalServerError {
* throw new InternalServerError("foo" );
* }
* }
*
* @Test
* public void testBadRequestLogging() throws Exception {
* // Create client that won't throw exceptions.
* RestClient client = MockRestClient.create (TestRest.class ).ignoreErrors().build();
* // Make the REST call.
* client .get("/bad" ).run().assertStatusCode(500).assertContent().contains("foo" );
* // Make sure the message was logged in our expected format.
* LOGGER .assertMessageAndReset().contains("[500] HTTP GET /bad" );
* }
* }
*
*
* See Also:
*/
public class BasicTestCaptureCallLogger extends CallLogger {
private AtomicReference lastRecord = new AtomicReference<>();
/**
* Constructor using specific settings.
*
* @param beanStore The bean store containing injectable beans for this logger.
*/
public BasicTestCaptureCallLogger(BeanStore beanStore) {
super(beanStore);
}
/**
* Constructor using default settings.
*
* Uses the same settings as {@link CallLogger}.
*/
public BasicTestCaptureCallLogger() {
super(BeanStore.INSTANCE);
}
@Override
protected Builder init(BeanStore beanStore) {
return super.init(beanStore)
.normalRules( // Rules when debugging is not enabled.
CallLoggerRule.create(beanStore) // Log 500+ errors with status-line and header information.
.statusFilter(x -> x >= 500)
.level(SEVERE)
.requestDetail(HEADER)
.responseDetail(HEADER)
.build(),
CallLoggerRule.create(beanStore) // Log 400-500 errors with just status-line information.
.statusFilter(x -> x >= 400)
.level(WARNING)
.requestDetail(STATUS_LINE)
.responseDetail(STATUS_LINE)
.build()
)
.debugRules( // Rules when debugging is enabled.
CallLoggerRule.create(beanStore) // Log everything with full details.
.level(SEVERE)
.requestDetail(ENTITY)
.responseDetail(ENTITY)
.build()
)
;
}
@Override
protected void log(Level level, String msg, Throwable e) {
LogRecord r = new LogRecord(level, msg);
r.setThrown(e);
this.lastRecord.set(r);
}
/**
* Returns the last logged message.
*
* @return The last logged message, or null if nothing was logged.
*/
public String getMessage() {
LogRecord r = lastRecord.get();
return r == null ? null : r.getMessage();
}
/**
* Returns the last logged message and then deletes it internally.
*
* @return The last logged message.
*/
public String getMessageAndReset() {
String msg = getMessage();
reset();
return msg;
}
/**
* Returns an assertion of the last logged message.
*
* @return The last logged message as an assertion object. Never null .
*/
public StringAssertion assertMessage() {
return new StringAssertion(getMessage());
}
/**
* Returns an assertion of the last logged message and then deletes it internally.
*
* @return The last logged message as an assertion object. Never null .
*/
public StringAssertion assertMessageAndReset() {
return new StringAssertion(getMessageAndReset());
}
/**
* Returns the last logged message level.
*
* @return The last logged message level, or null if nothing was logged.
*/
public Level getLevel() {
LogRecord r = lastRecord.get();
return r == null ? null : r.getLevel();
}
/**
* Returns the last logged message level.
*
* @return The last logged message level, or null if nothing was logged.
*/
public Throwable getThrown() {
LogRecord r = lastRecord.get();
return r == null ? null : r.getThrown();
}
/**
* Returns the last logged message level.
*
* @return The last logged message level, or null if nothing was logged.
*/
public ThrowableAssertion assertThrown() {
return new ThrowableAssertion<>(getThrown());
}
/**
* Resets the internal message buffer.
*
* @return This object.
*/
public BasicTestCaptureCallLogger reset() {
this.lastRecord.set(null);
return this;
}
}