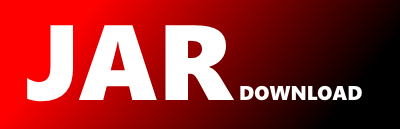
org.apache.juneau.rest.mock.MockConsole Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.mock;
import java.io.*;
import org.apache.juneau.assertions.*;
/**
* A capturing {@link PrintStream} that allows you to easily capture console output.
*
*
* Stores output into an internal {@link ByteArrayOutputStream}. Note that this means you could run into memory
* constraints if you heavily use this class.
*
*
* Typically used in conjunction with the {@link org.apache.juneau.rest.client.RestClient.Builder#console(PrintStream)} to capture console output for
* testing purposes.
*
*
Example:
*
* // A simple REST API that echos a posted bean.
* @Rest
* public class MyRest extends BasicRestObject {
* @RestPost ("/bean" )
* public Bean postBean(@Content Bean bean ) {
* return bean ;
* }
* }
*
* // Our mock console.
* MockConsole console = MockConsole.create ();
*
* // Make a call against our REST API and log the call.
* MockRestClient
* .create (MyRest.class )
* .json5()
* .logRequests(DetailLevel.FULL , Level.SEVERE )
* .logToConsole()
* .console(console )
* .build()
* .post("/bean" , bean )
* .run();
*
* console .assertContents().is(
* "" ,
* "=== HTTP Call (outgoing) ======================================================" ,
* "=== REQUEST ===" ,
* "POST http://localhost/bean" ,
* "---request headers---" ,
* " Accept: application/json5" ,
* "---request entity---" ,
* " Content-Type: application/json5" ,
* "---request content---" ,
* "{f:1}" ,
* "=== RESPONSE ===" ,
* "HTTP/1.1 200 " ,
* "---response headers---" ,
* " Content-Type: application/json" ,
* "---response content---" ,
* "{f:1}" ,
* "=== END =======================================================================" ,
* ""
* );
*
*
* See Also:
* - juneau-rest-mock
*
*/
public class MockConsole extends PrintStream {
private static final ByteArrayOutputStream baos = new ByteArrayOutputStream();
/**
* Constructor.
*/
public MockConsole() {
super(baos);
}
/**
* Creator.
*
* @return A new {@link MockConsole} object.
*/
public static MockConsole create() {
return new MockConsole();
}
/**
* Resets the contents of this buffer.
*
* @return This object.
*/
public synchronized MockConsole reset() {
baos.reset();
return this;
}
/**
* Allows you to perform fluent-style assertions on the contents of this buffer.
*
* Example:
*
* MockConsole console = MockConsole.create ();
*
* MockRestClient
* .create (MyRest.class )
* .console(console )
* .debug()
* .json5()
* .build()
* .get("/url" )
* .run();
*
* console .assertContents().isContains("HTTP GET /url" );
*
*
* @return A new fluent-style assertion object.
*/
public synchronized FluentStringAssertion assertContents() {
return new FluentStringAssertion<>(toString(), this);
}
/**
* Allows you to perform fluent-style assertions on the size of this buffer.
*
* Example:
*
* MockConsole console = MockConsole.create ();
*
* MockRestClient
* .create (MyRest.class )
* .console(console )
* .debug()
* .json5()
* .build()
* .get("/url" )
* .run();
*
* console .assertSize().isGreaterThan(0);
*
*
* @return A new fluent-style assertion object.
*/
public synchronized FluentIntegerAssertion assertSize() {
return new FluentIntegerAssertion<>(baos.size(), this);
}
/**
* Returns the contents of this buffer as a string.
*/
@Override
public String toString() {
return baos.toString();
}
}