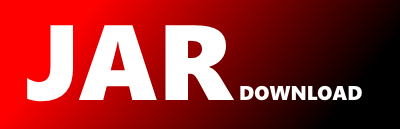
org.apache.juneau.rest.vars.FileVar Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.vars;
import org.apache.juneau.http.response.*;
import org.apache.juneau.internal.*;
import org.apache.juneau.rest.*;
import org.apache.juneau.svl.*;
/**
* File resource variable resolver
*
*
* The format for this var is "$F{path[,defaultValue]}" .
*
*
* Contents of files are retrieved from the request using {@link RestRequest#getStaticFiles()}.
*
* Localized resources (based on the locale of the HTTP request) are supported.
* For example, if looking for the resource "MyResource.txt" for the Japanese locale, we will look for
* files in the following order:
*
* "MyResource_ja_JP.txt"
* "MyResource_ja.txt"
* "MyResource.txt"
*
*
*
* Example:
*
* @HtmlDocConfig (
* aside="$F{resources/MyAsideMessage.html, Oops not found!}"
* )
*
*
*
* Files of type HTML, XHTML, XML, JSON, Javascript, and CSS will be stripped of comments.
* This allows you to place license headers in files without them being serialized to the output.
*
*
* This variable resolver requires that a {@link RestRequest} bean be available in the session bean store.
*
*
See Also:
* - SVL Variables
*
*/
public class FileVar extends DefaultingVar {
/**
* The name of this variable.
*/
public static final String NAME = "F";
/**
* Constructor.
*/
public FileVar() {
super(NAME);
}
@Override /* Var */
public String resolve(VarResolverSession session, String key) throws Exception {
RestRequest req = session.getBean(RestRequest.class).orElseThrow(InternalServerError::new);
String s = req.getStaticFiles().getString(key, null).orElse(null);
if (s == null)
return null;
String subType = FileUtils.getExtension(key);
if ("html".equals(subType) || "xhtml".equals(subType) || "xml".equals(subType))
s = s.replaceAll("(?s)\\s*", "");
else if ("json".equals(subType) || "javascript".equals(subType) || "css".equals(subType))
s = s.replaceAll("(?s)\\/\\*(.*?)\\*\\/\\s*", "");
return s;
}
@Override /* Var */
public boolean canResolve(VarResolverSession session) {
return session.getBean(RestRequest.class).isPresent();
}
}