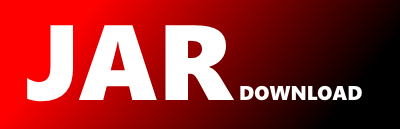
org.apache.juneau.rest.widget.MenuItemWidget Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.rest.widget;
import static org.apache.juneau.common.internal.IOUtils.*;
import static org.apache.juneau.common.internal.StringUtils.*;
import static org.apache.juneau.common.internal.ThrowableUtils.*;
import java.io.*;
import org.apache.juneau.html.*;
import org.apache.juneau.internal.*;
import org.apache.juneau.rest.*;
import org.apache.juneau.serializer.*;
/**
* A subclass of widgets for rendering menu items with drop-down windows.
*
* See Also:
* - Predefined Widgets
*
- Widgets
*
*/
public abstract class MenuItemWidget extends Widget {
/**
* Returns the Javascript needed for the show and hide actions of the menu item.
*/
@Override /* Widget */
public String getScript(RestRequest req, RestResponse res) {
return loadScript(req, "scripts/MenuItemWidget.js");
}
/**
* Optional Javascript to execute immediately before a menu item is shown.
*
*
* For example, the following shows how the method could be used to make an AJAX call back to the REST
* interface to populate a SELECT element in the contents of the popup dialog:
*
*
* @Override
* public String getBeforeShowScript(RestRequest req ) {
* return ""
* + "\n var xhr = new XMLHttpRequest();"
* + "\n xhr.open('GET', '/petstore/pet?s=status=AVAILABLE&v=id,name', true);"
* + "\n xhr.setRequestHeader('Accept', 'application/json');"
* + "\n xhr.onload = function() {"
* + "\n var pets = JSON.parse(xhr.responseText);"
* + "\n var select = document.getElementById('addPet_names');"
* + "\n select.innerHTML = '';"
* + "\n for (var i in pets) {"
* + "\n var pet = pets[i];"
* + "\n var opt = document.createElement('option');"
* + "\n opt.value = pet.id;"
* + "\n opt.innerHTML = pet.name;"
* + "\n select.appendChild(opt);"
* + "\n }"
* + "\n }"
* + "\n xhr.send();"
* ;
* }
*
*
*
* Note that it's often easier (and cleaner) to use the {@link #loadScript(RestRequest,String)} method and read the Javascript from
* your classpath:
*
*
* @Override
* public String getBeforeShowScript(RestRequest req ) throws Exception {
* return loadScript("AddOrderMenuItem_beforeShow.js" );
* }
*
*
* @param req The HTTP request object.
* @param res The HTTP response object.
* @return Javascript code to execute, or null if there isn't any.
*/
public String getBeforeShowScript(RestRequest req, RestResponse res) {
return null;
}
/**
* Optional Javascript to execute immediately after a menu item is shown.
*
*
* Same as {@link #getBeforeShowScript(RestRequest,RestResponse)} except this Javascript gets executed after the popup dialog has become visible.
*
* @param req The HTTP request object.
* @param res The HTTP response object.
* @return Javascript code to execute, or null if there isn't any.
*/
public String getAfterShowScript(RestRequest req, RestResponse res) {
return null;
}
/**
* Defines a "menu-item" class that needs to be used on the outer element of the HTML returned by the
* {@link #getHtml(RestRequest,RestResponse)} method.
*/
@Override /* Widget */
public String getStyle(RestRequest req, RestResponse res) {
return loadStyle(req, "styles/MenuItemWidget.css");
}
@Override /* Widget */
public String getHtml(RestRequest req, RestResponse res) {
StringBuilder sb = new StringBuilder();
// Need a unique number to define unique function names.
Integer id = null;
String pre = nullIfEmpty(getBeforeShowScript(req, res)), post = nullIfEmpty(getAfterShowScript(req, res));
sb.append("\n
"
);
return sb.toString();
}
private Integer getId(RestRequest req) {
Integer id = req.getAttribute("LastMenuItemId").as(Integer.class).orElse(0) + 1;
req.setAttribute("LastMenuItemId", id);
return id;
}
/**
* The label for the menu item as it's rendered in the menu bar.
*
* @param req The HTTP request object.
* @param res The HTTP response object.
* @return The menu item label.
*/
public abstract String getLabel(RestRequest req, RestResponse res);
/**
* The content of the popup.
*
* @param req The HTTP request object.
* @param res The HTTP response object.
* @return
* The content of the popup.
*
Can be any of the following types:
*
* - {@link Reader} - Serialized directly to the output.
*
- {@link CharSequence} - Serialized directly to the output.
*
- Other - Serialized as HTML using {@link HtmlSerializer#DEFAULT}.
*
Note that this includes any of the {@link org.apache.juneau.dto.html5} beans.
*
*/
public abstract Object getContent(RestRequest req, RestResponse res);
}