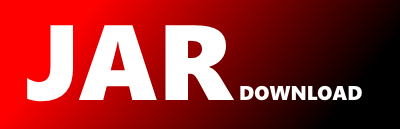
org.apache.juneau.serializer.annotation.SerializerConfig Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.serializer.annotation;
import static java.lang.annotation.ElementType.*;
import static java.lang.annotation.RetentionPolicy.*;
import java.lang.annotation.*;
import java.nio.charset.*;
import org.apache.juneau.*;
import org.apache.juneau.annotation.*;
import org.apache.juneau.parser.*;
import org.apache.juneau.serializer.*;
/**
* Annotation for specifying config properties defined in {@link Serializer}, {@link OutputStreamSerializer}, and {@link WriterSerializer}.
*
*
* Used primarily for specifying bean configuration properties on REST classes and methods.
*
*
See Also:
*/
@Target({TYPE,METHOD})
@Retention(RUNTIME)
@Inherited
@ContextApply({SerializerConfigAnnotation.SerializerApply.class,SerializerConfigAnnotation.OutputStreamSerializerApply.class,SerializerConfigAnnotation.WriterSerializerApply.class})
public @interface SerializerConfig {
/**
* Optional rank for this config.
*
*
* Can be used to override default ordering and application of config annotations.
*
* @return The annotation value.
*/
int rank() default 0;
//-------------------------------------------------------------------------------------------------------------------
// OutputStreamSerializer
//-------------------------------------------------------------------------------------------------------------------
/**
* Binary output format.
*
*
* When using the {@link OutputStreamSerializer#serializeToString(Object)} method on stream-based serializers, this defines the format to use
* when converting the resulting byte array to a string.
*
*
* "SPACED_HEX"
* "HEX" (default)
* "BASE64"
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.OutputStreamSerializer.Builder#binaryFormat(BinaryFormat)}
*
*
* @return The annotation value.
*/
String binaryFormat() default "";
//-------------------------------------------------------------------------------------------------------------------
// Serializer
//-------------------------------------------------------------------------------------------------------------------
/**
* Add "_type" properties when needed.
*
*
* If "true" , then "_type" properties will be added to beans if their type cannot be inferred
* through reflection.
*
*
* This is used to recreate the correct objects during parsing if the object types cannot be inferred.
*
For example, when serializing a Map<String,Object> field where the bean class cannot be determined from
* the type of the values.
*
*
* Note the differences between the following settings:
*
* - {@link org.apache.juneau.serializer.Serializer.Builder#addRootType()} - Affects whether
'_type' is added to root node.
* - {@link org.apache.juneau.serializer.Serializer.Builder#addBeanTypes()} - Affects whether
'_type' is added to any nodes.
*
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#addBeanTypes()}
*
*
* @return The annotation value.
*/
String addBeanTypes() default "";
/**
* Add type attribute to root nodes.
*
*
* When disabled, it is assumed that the parser knows the exact Java POJO type being parsed, and therefore top-level
* type information that might normally be included to determine the data type will not be serialized.
*
*
* For example, when serializing a top-level POJO with a {@link Bean#typeName() @Bean(typeName)} value, a
* '_type' attribute will only be added when this setting is enabled.
*
*
* Note the differences between the following settings:
*
* - {@link org.apache.juneau.serializer.Serializer.Builder#addRootType()} - Affects whether
'_type' is added to root node.
* - {@link org.apache.juneau.serializer.Serializer.Builder#addBeanTypes()} - Affects whether
'_type' is added to any nodes.
*
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#addRootType()}
*
*
* @return The annotation value.
*/
String addRootType() default "";
/**
* Don't trim null bean property values.
*
*
* If "true" , null bean values will be serialized to the output.
*
*
* Note that not enabling this setting has the following effects on parsing:
*
* -
* Map entries with
null values will be lost.
*
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#keepNullProperties()}
*
*
* @return The annotation value.
*/
String keepNullProperties() default "";
/**
* Serializer listener.
*
*
* Class used to listen for errors and warnings that occur during serialization.
*
*
See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#listener(Class)}
*
*
* @return The annotation value.
*/
Class extends SerializerListener> listener() default SerializerListener.Void.class;
/**
* Sort arrays and collections alphabetically.
*
*
* Copies and sorts the contents of arrays and collections before serializing them.
*
*
* Note that this introduces a performance penalty.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#sortCollections()}
*
*
* @return The annotation value.
*/
String sortCollections() default "";
/**
* Sort maps alphabetically.
*
*
* Copies and sorts the contents of maps by their keys before serializing them.
*
*
* Note that this introduces a performance penalty.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#sortMaps()}
*
*
* @return The annotation value.
*/
String sortMaps() default "";
/**
* Trim empty lists and arrays.
*
*
* If "true" , empty lists and arrays will not be serialized.
*
*
* Note that enabling this setting has the following effects on parsing:
*
* -
* Map entries with empty list values will be lost.
*
-
* Bean properties with empty list values will not be set.
*
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#trimEmptyCollections()}
*
*
* @return The annotation value.
*/
String trimEmptyCollections() default "";
/**
* Trim empty maps.
*
*
* If "true" , empty map values will not be serialized to the output.
*
*
* Note that enabling this setting has the following effects on parsing:
*
* -
* Bean properties with empty map values will not be set.
*
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#trimEmptyMaps()}
*
*
* @return The annotation value.
*/
String trimEmptyMaps() default "";
/**
* Trim strings.
*
*
* If "true" , string values will be trimmed of whitespace using {@link String#trim()} before being serialized.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#trimStrings()}
*
*
* @return The annotation value.
*/
String trimStrings() default "";
/**
* URI context bean.
*
* Description:
*
* Bean used for resolution of URIs to absolute or root-relative form.
*
*
Notes:
* -
* Format: JSON object representing a {@link UriContext}
*
-
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#uriContext(UriContext)}
*
*
* @return The annotation value.
*/
String uriContext() default "";
/**
* URI relativity.
*
*
* Defines what relative URIs are relative to when serializing any of the following:
*
* - {@link java.net.URI}
*
- {@link java.net.URL}
*
- Properties and classes annotated with {@link Uri @Uri}
*
*
*
* "RESOURCE" (default) - Relative URIs should be considered relative to the servlet URI.
* "PATH_INFO" - Relative URIs should be considered relative to the request URI.
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#uriRelativity(UriRelativity)}
*
- URIs
*
*
* @return The annotation value.
*/
String uriRelativity() default "";
/**
* URI resolution.
*
*
* Defines the resolution level for URIs when serializing any of the following:
*
* - {@link java.net.URI}
*
- {@link java.net.URL}
*
- Properties and classes annotated with {@link Uri @Uri}
*
*
*
* "ABSOLUTE" - Resolve to an absolute URL (e.g. "http://host:port/context-root/servlet-path/path-info" ).
* "ROOT_RELATIVE" - Resolve to a root-relative URL (e.g. "/context-root/servlet-path/path-info" ).
* "NONE" (default) - Don't do any URL resolution.
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.Serializer.Builder#uriResolution(UriResolution)}
*
- URIs
*
*
* @return The annotation value.
*/
String uriResolution() default "";
//-------------------------------------------------------------------------------------------------------------------
// WriterSerializer
//-------------------------------------------------------------------------------------------------------------------
/**
* File charset.
*
*
* The character set to use for writing Files to the file system.
*
*
* Used when passing in files to {@link Serializer#serialize(Object, Object)}.
*
*
Notes:
* -
* Format: string
*
-
* "DEFAULT" can be used to indicate the JVM default file system charset.
*
-
* Default: JVM system default.
*
-
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
* -
* This setting does not apply to the RDF serializers.
*
*
* See Also:
* - {@link org.apache.juneau.serializer.WriterSerializer.Builder#fileCharset(java.nio.charset.Charset)}
*
*
* @return The annotation value.
*/
String fileCharset() default "";
/**
* Maximum indentation.
*
*
* Specifies the maximum indentation level in the serialized document.
*
*
Notes:
* -
* Format: integer
*
-
* Default: 100
*
-
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
* -
* This setting does not apply to the RDF serializers.
*
*
* See Also:
* - {@link org.apache.juneau.serializer.WriterSerializer.Builder#maxIndent(int)}
*
*
* @return The annotation value.
*/
String maxIndent() default "";
/**
* Quote character.
*
*
* This is the character used for quoting attributes and values.
*
*
Notes:
* -
* Default:
"
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
* -
* This setting does not apply to the RDF serializers.
*
*
* See Also:
* - {@link org.apache.juneau.serializer.WriterSerializer.Builder#quoteChar(char)}
*
*
* @return The annotation value.
*/
String quoteChar() default "";
/**
* Output stream charset.
*
*
* The character set to use when writing to OutputStreams.
*
*
* Used when passing in output streams and byte arrays to {@link WriterSerializer#serialize(Object, Object)}.
*
*
Notes:
* -
* Format: string
*
-
* Default:
"utf-8" .
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
* -
* This setting does not apply to the RDF serializers.
*
*
* See Also:
* - {@link org.apache.juneau.serializer.WriterSerializer.Builder#streamCharset(Charset)}
*
*
* @return The annotation value.
*/
String streamCharset() default "";
/**
* Use whitespace.
*
*
* If "true" , whitespace is added to the output to improve readability.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.serializer.WriterSerializer.Builder#useWhitespace()}
*
*
* @return The annotation value.
*/
String useWhitespace() default "";
//-----------------------------------------------------------------------------------------------------------------
// BeanTraverseContext
//-----------------------------------------------------------------------------------------------------------------
/**
* Automatically detect POJO recursions.
*
*
* Specifies that recursions should be checked for during traversal.
*
*
* Recursions can occur when traversing models that aren't true trees but rather contain loops.
*
In general, unchecked recursions cause stack-overflow-errors.
*
These show up as {@link ParseException ParseExceptions} with the message "Depth too deep. Stack overflow occurred." .
*
*
* The behavior when recursions are detected depends on the value for {@link org.apache.juneau.BeanTraverseContext.Builder#ignoreRecursions()}.
*
*
* For example, if a model contains the links A->B->C->A, then the JSON generated will look like
* the following when BEANTRAVERSE_ignoreRecursions is true ...
*
*
* {A:{B:{C:null }}}
*
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Checking for recursion can cause a small performance penalty.
*
-
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanTraverseContext.Builder#detectRecursions()}
*
*
* @return The annotation value.
*/
String detectRecursions() default "";
/**
* Ignore recursion errors.
*
*
* Used in conjunction with {@link org.apache.juneau.BeanTraverseContext.Builder#detectRecursions()}.
*
Setting is ignored if BEANTRAVERSE_detectRecursions is "false" .
*
*
* If "true" , when we encounter the same object when traversing a tree, we set the value to null .
*
Otherwise, a {@link BeanRecursionException} is thrown with the message "Recursion occurred, stack=..." .
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanTraverseContext.Builder#ignoreRecursions()}
*
*
* @return The annotation value.
*/
String ignoreRecursions() default "";
/**
* Initial depth.
*
*
* The initial indentation level at the root.
*
Useful when constructing document fragments that need to be indented at a certain level.
*
*
Notes:
* -
* Format: integer
*
-
* Default value:
"0"
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanTraverseContext.Builder#initialDepth(int)}
*
*
* @return The annotation value.
*/
String initialDepth() default "";
/**
* Max traversal depth.
*
*
* Abort traversal if specified depth is reached in the POJO tree.
*
If this depth is exceeded, an exception is thrown.
*
*
Notes:
* -
* Format: integer
*
-
* Default value:
"100"
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.BeanTraverseContext.Builder#maxDepth(int)}
*
*
* @return The annotation value.
*/
String maxDepth() default "";
}