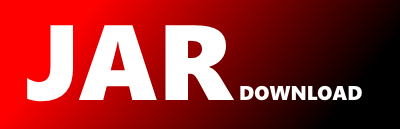
org.apache.juneau.swap.Surrogate Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.swap;
import org.apache.juneau.annotation.*;
/**
* Identifies a class as being a surrogate class.
*
*
* Surrogate classes are used in place of other classes during serialization.
*
For example, you may want to use a surrogate class to change the names or order of bean properties on a bean.
*
*
* This interface has no methods to implement.
*
It's simply used by the framework to identify the class as a surrogate class when specified as a swap.
*
*
* The following is an example of a surrogate class change changes a property name:
*
* public class MySurrogate implements Surrogate {
*
* // Public constructor that wraps the normal object during serialization.
* public MySurrogate(NormalClass object ) {...}
*
* // Public no-arg constructor using during parsing.
* // Not required if only used during serializing.
* public MySurrogate() {...}
*
* // Public method that converts surrogate back into normal object during parsing.
* // The method name can be anything (e.g. "build", "create", etc...).
* // Not required if only used during serializing.
* public NormalClass unswap() {...}
* }
*
*
*
* Surrogate classes are associated with serializers and parsers using the {@link org.apache.juneau.BeanContext.Builder#swaps(Class...)}
* method.
*
* JsonSerializer serializer = JsonSerializer
* .create ()
* .swaps(MySurrogate.class )
* .build();
*
* JsonParser parser = JsonParser
* .create ()
* .swaps(MySurrogate.class )
* .build();
*
*
*
* Surrogates can also be associated using the {@link Swap @Swap} annotation.
*
* @Swap (MySurrogate.class )
* public class NormalClass {...}
*
*
*
* On a side note, a surrogate class is functionally equivalent to the following {@link ObjectSwap}
* implementation:
*
* public class MySurrogate extends ObjectSwap<NormalClass,MySurrogate> {
* public MySurrogate swap(NormalClass object ) throws SerializeException {
* return new MySurrogate(object );
* }
* public NormalClass unswap(MySurrogate surrogate , ClassMeta<?> hint ) throws ParseException {
* return surrogate .unswap();
* }
* }
*
*
* See Also:
* - Surrogate Classes
*
- Swaps
*
*/
public interface Surrogate {}