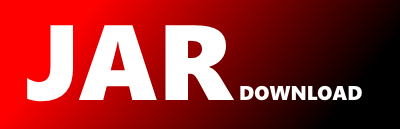
org.apache.juneau.xml.annotation.XmlConfig Maven / Gradle / Ivy
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.xml.annotation;
import static java.lang.annotation.ElementType.*;
import static java.lang.annotation.RetentionPolicy.*;
import java.lang.annotation.*;
import javax.xml.stream.*;
import javax.xml.stream.util.*;
import org.apache.juneau.annotation.*;
import org.apache.juneau.collections.*;
import org.apache.juneau.serializer.*;
import org.apache.juneau.xml.*;
/**
* Annotation for specifying config properties defined in {@link XmlSerializer}, {@link XmlDocSerializer}, and {@link XmlParser}.
*
*
* Used primarily for specifying bean configuration properties on REST classes and methods.
*
*
See Also:
* - XML Details
*
*/
@Target({TYPE,METHOD})
@Retention(RUNTIME)
@Inherited
@ContextApply({XmlConfigAnnotation.SerializerApply.class,XmlConfigAnnotation.ParserApply.class})
public @interface XmlConfig {
/**
* Optional rank for this config.
*
*
* Can be used to override default ordering and application of config annotations.
*
* @return The annotation value.
*/
int rank() default 0;
//-------------------------------------------------------------------------------------------------------------------
// XmlCommon
//-------------------------------------------------------------------------------------------------------------------
//-------------------------------------------------------------------------------------------------------------------
// XmlParser
//-------------------------------------------------------------------------------------------------------------------
/**
* XML event allocator.
*
*
* Associates an {@link XMLEventAllocator} with this parser.
*
*
See Also:
* - {@link org.apache.juneau.xml.XmlParser.Builder#eventAllocator(Class)}
*
*
* @return The annotation value.
*/
Class extends XMLEventAllocator> eventAllocator() default XmlEventAllocator.Void.class;
/**
* Preserve root element during generalized parsing.
*
*
* If "true" , when parsing into a generic {@link JsonMap}, the map will contain a single entry whose key
* is the root element name.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.xml.XmlParser.Builder#preserveRootElement()}
*
*
* @return The annotation value.
*/
String preserveRootElement() default "";
/**
* XML reporter.
*
*
* Associates an {@link XMLReporter} with this parser.
*
*
Notes:
* -
* Reporters are not copied to new parsers during a clone.
*
*
* See Also:
* - {@link org.apache.juneau.xml.XmlParser.Builder#reporter(Class)}
*
*
* @return The annotation value.
*/
Class extends XMLReporter> reporter() default XmlReporter.Void.class;
/**
* XML resolver.
*
*
* Associates an {@link XMLResolver} with this parser.
*
*
See Also:
* - {@link org.apache.juneau.xml.XmlParser.Builder#resolver(Class)}
*
*
* @return The annotation value.
*/
Class extends XMLResolver> resolver() default XmlResolver.Void.class;
/**
* Enable validation.
*
*
* If "true" , XML document will be validated.
*
*
* See {@link XMLInputFactory#IS_VALIDATING} for more info.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.xml.XmlParser.Builder#validating()}
*
*
* @return The annotation value.
*/
String validating() default "";
//-------------------------------------------------------------------------------------------------------------------
// XmlSerializer
//-------------------------------------------------------------------------------------------------------------------
/**
* Add "_type" properties when needed.
*
*
* If "true" , then "_type" properties will be added to beans if their type cannot be inferred
* through reflection.
*
*
* When present, this value overrides the {@link org.apache.juneau.serializer.Serializer.Builder#addBeanTypes()} setting and is
* provided to customize the behavior of specific serializers in a {@link SerializerSet}.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.xml.XmlSerializer.Builder#addBeanTypesXml()}
*
*
* @return The annotation value.
*/
String addBeanTypes() default "";
/**
* Add namespace URLs to the root element.
*
*
* Use this setting to add {@code xmlns:x} attributes to the root element for the default and all mapped namespaces.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* This setting is ignored if {@link org.apache.juneau.xml.XmlSerializer.Builder#enableNamespaces()} is not enabled.
*
-
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.xml.XmlSerializer.Builder#addNamespaceUrisToRoot()}
*
- Namespaces
*
*
* @return The annotation value.
*/
String addNamespaceUrisToRoot() default "";
/**
* Don't auto-detect namespace usage.
*
*
* Don't detect namespace usage before serialization.
*
*
* Used in conjunction with {@link org.apache.juneau.xml.XmlSerializer.Builder#addNamespaceUrisToRoot()} to reduce the list of namespace URLs appended to the
* root element to only those that will be used in the resulting document.
*
*
* If disabled, then the data structure will first be crawled looking for namespaces that will be encountered before
* the root element is serialized.
*
*
* This setting is ignored if {@link org.apache.juneau.xml.XmlSerializer.Builder#enableNamespaces()} is not enabled.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Auto-detection of namespaces can be costly performance-wise.
*
In high-performance environments, it's recommended that namespace detection be
* disabled, and that namespaces be manually defined through the {@link org.apache.juneau.xml.XmlSerializer.Builder#namespaces(Namespace...)} property.
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.xml.XmlSerializer.Builder#disableAutoDetectNamespaces()}
*
- Namespaces
*
*
* @return The annotation value.
*/
String disableAutoDetectNamespaces() default "";
/**
* Default namespace.
*
*
* Specifies the default namespace URI for this document.
*
*
Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.xml.XmlSerializer.Builder#defaultNamespace(Namespace)}
*
- Namespaces
*
*
* @return The annotation value.
*/
String defaultNamespace() default "";
/**
* Enable support for XML namespaces.
*
*
* If not enabled, XML output will not contain any namespaces regardless of any other settings.
*
*
* "true"
* "false" (default)
*
*
* Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.xml.XmlSerializer.Builder#enableNamespaces()}
*
- Namespaces
*
*
* @return The annotation value.
*/
String enableNamespaces() default "";
/**
* Default namespaces.
*
*
* The default list of namespaces associated with this serializer.
*
*
Notes:
* -
* Supports VarResolver.DEFAULT (e.g.
"$C{myConfigVar}" ).
*
*
* See Also:
* - {@link org.apache.juneau.xml.XmlSerializer.Builder#namespaces(Namespace...)}
*
- Namespaces
*
*
* @return The annotation value.
*/
String[] namespaces() default {};
}