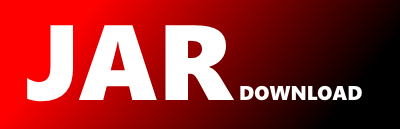
org.apache.juneau.assertions.Assertions Maven / Gradle / Ivy
Show all versions of juneau-assertions Show documentation
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.assertions;
import static org.apache.juneau.common.internal.IOUtils.*;
import java.io.*;
import java.time.*;
import java.util.*;
import java.util.stream.*;
import org.apache.juneau.*;
import org.apache.juneau.common.utils.*;
/**
* Main class for creation of assertions for stand-alone testing.
*
*
* Provides assertions for various common POJO types.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Assert string is greater than 100 characters and contains "foo".
* assertString (myString )
* .length().isGt(100)
* .contains("foo" );
*
*
*
* Provides simple testing that {@link Throwable Throwables} are being thrown correctly.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Assert that calling doBadCall() causes a RuntimeException.
* assertThrown (() -> myPojo .doBadCall())
* .isType(RuntimeException.class )
* .message().contains("Bad thing happened." );
*
*
*
* Provides other assertion convenience methods such as asserting non-null method arguments.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* public String getFoo(String bar ) {
* assertArgNotNull ("bar" , bar );
* ...
* }
*
*
* See Also:
*
*/
public class Assertions {
//-----------------------------------------------------------------------------------------------------------------
// Fluent assertions
//-----------------------------------------------------------------------------------------------------------------
/**
* Performs an assertion on an arbitrary POJO.
*
*
* The distinction between {@link ObjectAssertion} and {@link AnyAssertion} is that the latter supports all
* the operations of the former, but adds various transform methods for conversion to specific assertion types.
*
*
* Various transform methods such as {@link FluentListAssertion#asItem(int)} and {@link FluentBeanAssertion#asProperty(String)}
* return generic any-assertions so that they can be easily transformed into other assertion types.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that the property 'foo' of a bean is 'bar'.
* assertAny (myPojo ) // Start with AnyAssertion.
* .asBean(MyBean.class ) // Transform to BeanAssertion.
* .property("foo" ).is("bar" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link AnyAssertion} for supported operations on this type.
*
* @param The value type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final AnyAssertion assertAny(T value) {
return AnyAssertion.create(value);
}
/**
* Performs an assertion on an array of POJOs.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that an Integer array contains [1,2,3].
* Integer[] array = {...};
* assertArray (array )
* .asJson().is("[1,2,3]" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link ArrayAssertion} for supported operations on this type.
*
* @param The value element type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final ArrayAssertion assertArray(E[] value) {
return ArrayAssertion.create(value);
}
/**
* Performs an assertion on a Java bean.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that the 'foo' and 'bar' properties of a bean are 1 and 2 respectively.
* assertBean (myBean )
* .isType(MyBean.class )
* .extract("foo,bar" )
* .asJson().is("{foo:1,bar:2}" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link BeanAssertion} for supported operations on this type.
*
* @param The value type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final BeanAssertion assertBean(T value) {
return BeanAssertion.create(value);
}
/**
* Performs an assertion on a list of Java beans.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a bean list has 3 entries with 'foo' property values of 'bar','baz','qux'.
* assertBeanList (myListOfBeans )
* .isSize(3)
* .property("foo" )
* .is("bar" ,"baz" ,"qux" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link BeanListAssertion} for supported operations on this type.
*
* @param The element type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final BeanListAssertion assertBeanList(List value) {
return BeanListAssertion.create(value);
}
/**
* Performs an assertion on a Boolean.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a Boolean is not null and TRUE.
* assertBoolean (myBoolean )
* .isTrue();
*
*
*
* See Fluent Assertions for general assertion usage and {@link BooleanAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final BooleanAssertion assertBoolean(Boolean value) {
return BooleanAssertion.create(value);
}
/**
* Performs an assertion on a boolean array.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a Boolean array has size of 3 and all entries are TRUE.
* assertBooleanArray (myBooleanArray )
* .isSize(3)
* .all(x -> x == true );
*
*
*
* See Fluent Assertions for general assertion usage and {@link PrimitiveArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final PrimitiveArrayAssertion assertBooleanArray(boolean[] value) {
return PrimitiveArrayAssertion.create(value);
}
/**
* Performs an assertion on a byte array.
*
*
* The distinction between {@link #assertByteArray} and {@link #assertBytes} is that the former returns an assertion
* more tied to general byte arrays and the latter returns an assertion more tied to dealing with binary streams
* that can be decoded or transformed into a string.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a byte array has size of 3 and all bytes are larger than 10.
* assertByteArray (myByteArray )
* .isSize(3)
* .all(x -> x > 10);
*
*
*
* See Fluent Assertions for general assertion usage and {@link PrimitiveArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final PrimitiveArrayAssertion assertByteArray(byte[] value) {
return PrimitiveArrayAssertion.create(value);
}
/**
* Performs an assertion on a byte array.
*
*
* The distinction between {@link #assertByteArray} and {@link #assertBytes} is that the former returns an assertion
* more tied to general byte arrays and the latter returns an assertion more tied to dealing with binary streams
* that can be decoded or transformed into a string.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that the byte array contains the string "foo".
* assertBytes (myBytes )
* .asHex().is("666F6F" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link ByteArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final ByteArrayAssertion assertBytes(byte[] value) {
return ByteArrayAssertion.create(value);
}
/**
* Performs an assertion on the contents of an input stream.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that the stream contains the string "foo".
* assertBytes (myStream )
* .asHex().is("666F6F" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link ByteArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
*
Stream is automatically closed.
* @return
* A new assertion object.
*
Never null .
* @throws IOException If thrown while reading contents from stream.
*/
public static final ByteArrayAssertion assertBytes(InputStream value) throws IOException {
return assertBytes(value == null ? null : readBytes(value));
}
/**
* Performs an assertion on a char array.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that the char array contains the string "foo".
* assertCharArray (myCharArray )
* .asString().is("foo" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link PrimitiveArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final PrimitiveArrayAssertion assertCharArray(char[] value) {
return PrimitiveArrayAssertion.create(value);
}
/**
* Performs an assertion on a collection of POJOs.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a collection of strings has only one entry of 'foo'.
* assertCollection (myCollectionOfStrings )
* .isSize(1)
* .contains("foo" );
*
*
*
* In general, use {@link #assertList(List)} if you're performing an assertion on a list since {@link ListAssertion}
* provides more functionality than {@link CollectionAssertion}.
*
*
* See Fluent Assertions for general assertion usage and {@link CollectionAssertion} for supported operations on this type.
*
* @param The element type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final CollectionAssertion assertCollection(Collection value) {
return CollectionAssertion.create(value);
}
/**
* Performs an assertion on a Comparable.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts a comparable is less than another comparable.
* assertComparable (myComparable )
* .isLt(anotherComparable );
*
*
*
* See Fluent Assertions for general assertion usage and {@link ComparableAssertion} for supported operations on this type.
*
* @param The value type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final > ComparableAssertion assertComparable(T value) {
return ComparableAssertion.create(value);
}
/**
* Performs an assertion on a Date.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts the specified date is after the current date.
* assertDate (myDate )
* .isAfterNow();
*
*
*
* See Fluent Assertions for general assertion usage and {@link DateAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final DateAssertion assertDate(Date value) {
return DateAssertion.create(value);
}
/**
* Performs an assertion on a double array.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a double array is at least size 100 and all values are greater than 1000.
* assertDoubleArray (myDoubleArray )
* .size().isGte(100f)
* .all(x -> x > 1000f);
*
*
*
* See Fluent Assertions for general assertion usage and {@link PrimitiveArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final PrimitiveArrayAssertion assertDoubleArray(double[] value) {
return PrimitiveArrayAssertion.create(value);
}
/**
* Performs an assertion on a float array.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a float array is at least size 100 and all values are greater than 1000.
* assertFloatArray (myFloatArray )
* .size().isGte(100f)
* .all(x -> x > 1000f);
*
*
*
* See Fluent Assertions for general assertion usage and {@link PrimitiveArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final PrimitiveArrayAssertion assertFloatArray(float[] value) {
return PrimitiveArrayAssertion.create(value);
}
/**
* Performs an assertion on an int array.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a double array is at least size 100 and all values are greater than 1000.
* assertIntArray (myIntArray )
* .size().isGte(100)
* .all(x -> x > 1000);
*
*
*
* See Fluent Assertions for general assertion usage and {@link PrimitiveArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final PrimitiveArrayAssertion assertIntArray(int[] value) {
return PrimitiveArrayAssertion.create(value);
}
/**
* Performs an assertion on an Integer.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Assert that an HTTP response status code is 200 or 404.
* assertInteger (httpReponse )
* .isAny(200,404);
*
*
*
* See Fluent Assertions for general assertion usage and {@link IntegerAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final IntegerAssertion assertInteger(Integer value) {
return IntegerAssertion.create(value);
}
/**
* Performs an assertion on a list of POJOs.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Assert that the first entry in a list is "{foo:'bar'}" when serialized to simplified JSON.
* assertList (myList )
* .item(0)
* .asJson().is("{foo:'bar'}" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link ListAssertion} for supported operations on this type.
*
* @param The element type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final ListAssertion assertList(List value) {
return ListAssertion.create(value);
}
/**
* Performs an assertion on a stream of POJOs.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Assert that the first entry in a list is "{foo:'bar'}" when serialized to simplified JSON.
* assertList (myStream )
* .item(0)
* .asJson().is("{foo:'bar'}" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link ListAssertion} for supported operations on this type.
*
* @param The element type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final ListAssertion assertList(Stream value) {
return ListAssertion.create(value);
}
/**
* Performs an assertion on a Long.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Throw a BadReqest if an HTTP response length is greater than 100k.
* assertLong (responseLength )
* .throwable(BadRequest.class )
* .msg("Request is too large" )
* .isLt(100000);
*
*
*
* See Fluent Assertions for general assertion usage and {@link LongAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final LongAssertion assertLong(Long value) {
return LongAssertion.create(value);
}
/**
* Performs an assertion on a long array.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a long array is at least size 100 and all values are greater than 1000.
* assertLongArray (myLongArray )
* .size().isGte(100)
* .all(x -> x > 1000);
*
*
*
* See Fluent Assertions for general assertion usage and {@link PrimitiveArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final PrimitiveArrayAssertion assertLongArray(long[] value) {
return PrimitiveArrayAssertion.create(value);
}
/**
* Performs an assertion on a map.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Assert the specified map is a HashMap and contains the key "foo".
* assertMap (myMap )
* .isType(HashMap.class )
* .containsKey("foo" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link MapAssertion} for supported operations on this type.
*
* @param The key type.
* @param The value type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final MapAssertion assertMap(Map value) {
return MapAssertion.create(value);
}
/**
* Performs an assertion on a Java Object.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts the specified POJO is of type MyBean and is "{foo:'bar'}"
* // when serialized to Simplified JSON.
* assertObject (myPojo )
* .isType(MyBean.class )
* .asJson().is("{foo:'bar'}" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link ObjectAssertion} for supported operations on this type.
*
* @param The value type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final ObjectAssertion assertObject(T value) {
return ObjectAssertion.create(value);
}
/**
* Performs an assertion on a Java Object wrapped in an Optional.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts the specified POJO is of type MyBean and is "{foo:'bar'}"
* // when serialized to Simplified JSON.
* assertOptional (opt )
* .isType(MyBean.class )
* .asJson().is("{foo:'bar'}" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link AnyAssertion} for supported operations on this type.
*
* @param The value type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final AnyAssertion assertOptional(Optional value) {
return AnyAssertion.create(value.orElse(null));
}
/**
* Performs an assertion on the contents of a Reader.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts the contents of the Reader contains "foo".
* assertReader (myReader )
* .contains("foo" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link StringAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
*
Reader is automatically closed.
* @return
* A new assertion object.
*
Never null .
* @throws IOException If thrown while reading contents from reader.
*/
public static final StringAssertion assertReader(Reader value) throws IOException {
return assertString(read(value));
}
/**
* Performs an assertion on a short array.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that a float array is at least size 10 and all values are greater than 100.
* assertShortArray (myShortArray )
* .size().isGte(10)
* .all(x -> x > 100);
*
*
*
* See Fluent Assertions for general assertion usage and {@link PrimitiveArrayAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final PrimitiveArrayAssertion assertShortArray(short[] value) {
return PrimitiveArrayAssertion.create(value);
}
/**
* Performs an assertion on a String.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts a string is at least 100 characters long and contains "foo".
* assertString (myString )
* .size().isGte(100)
* .contains("foo" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link StringAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final StringAssertion assertString(Object value) {
if (value instanceof Optional)
value = ((Optional>)value).orElse(null);
return StringAssertion.create(value);
}
/**
* Performs an assertion on a list of Strings.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts a list of strings contain "foo,bar,baz" after trimming all and joining.
* assertStringList (myListOfStrings )
* .isSize(3)
* .trim()
* .join("," )
* .is("foo,bar,baz" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link StringListAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final StringListAssertion assertStringList(List value) {
return StringListAssertion.create(value);
}
/**
* Performs an assertion on a Throwable.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts a throwable is a RuntimeException containing 'foobar' in the message.
* assertThrowable (throwable )
* .isExactType(RuntimeException.class )
* .message().contains("foobar" );
*
*
*
* See Fluent Assertions for general assertion usage and {@link ThrowableAssertion} for supported operations on this type.
*
* @param The value type.
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final ThrowableAssertion assertThrowable(T value) {
return ThrowableAssertion.create(value);
}
/**
* Performs an assertion on a Version.
*
* Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts the specified major version is at least 2.
* assertVersion (version )
* .major().isGte(2);
*
*
*
* See Fluent Assertions for general assertion usage and {@link VersionAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final VersionAssertion assertVersion(Version value) {
return VersionAssertion.create(value);
}
/**
* Performs an assertion on a ZonedDateTime.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts the specified date is after the current date.
* assertZonedDateTime (myZonedDateTime )
* .isAfterNow();
*
*
*
* See Fluent Assertions for general assertion usage and {@link ZonedDateTimeAssertion} for supported operations on this type.
*
* @param value
* The object being tested.
*
Can be null .
* @return
* A new assertion object.
*
Never null .
*/
public static final ZonedDateTimeAssertion assertZonedDateTime(ZonedDateTime value) {
return ZonedDateTimeAssertion.create(value);
}
//-----------------------------------------------------------------------------------------------------------------
// Snippet assertions
//-----------------------------------------------------------------------------------------------------------------
/**
* Executes an arbitrary snippet of code and captures anything thrown from it as a Throwable assertion.
*
*
Example:
*
* import static org.apache.juneau.assertions.Assertions.*;
*
* // Asserts that the specified method throws a RuntimeException containing "foobar" in the message.
* assertThrown (()->foo .getBar())
* .isType(RuntimeException.class )
* .message().contains("foobar" );
*
*
* @param snippet The snippet of code to execute.
* @return A new assertion object. Never null .
*/
public static final ThrowableAssertion assertThrown(Snippet snippet) {
try {
snippet.run();
} catch (Throwable e) {
return assertThrowable(e);
}
return assertThrowable(null);
}
}