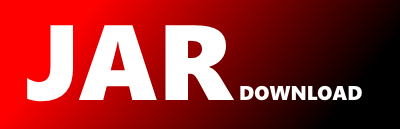
org.apache.juneau.assertions.FluentDateAssertion Maven / Gradle / Ivy
Show all versions of juneau-assertions Show documentation
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.assertions;
import static org.apache.juneau.common.internal.ArgUtils.*;
import static org.apache.juneau.internal.ObjectUtils.*;
import java.io.*;
import java.time.temporal.*;
import java.util.*;
import java.util.function.*;
import org.apache.juneau.cp.*;
import org.apache.juneau.internal.*;
import org.apache.juneau.serializer.*;
/**
* Used for fluent assertion calls against dates.
*
* Example:
*
* // Validates the response expiration is after the current date.
* client
* .get(URL )
* .run()
* .assertDateHeader("Expires" ).isAfterNow();
*
*
*
* Test Methods:
*
*
* - {@link FluentDateAssertion}
*
* - {@link FluentDateAssertion#is(Date,ChronoUnit) is(Date,ChronoUnit)}
*
- {@link FluentDateAssertion#isAfter(Date) isAfter(Date)}
*
- {@link FluentDateAssertion#isAfterNow() isAfterNow()}
*
- {@link FluentDateAssertion#isBefore(Date) isBefore(Date)}
*
- {@link FluentDateAssertion#isBeforeNow() isBeforeNow()}
*
- {@link FluentDateAssertion#isBetween(Date,Date) isBetween(Date,Date)}
*
* - {@link FluentComparableAssertion}
*
* - {@link FluentComparableAssertion#isGt(Comparable) isGt(Comparable)}
*
- {@link FluentComparableAssertion#isGte(Comparable) isGte(Comparable)}
*
- {@link FluentComparableAssertion#isLt(Comparable) isLt(Comparable)}
*
- {@link FluentComparableAssertion#isLte(Comparable) isLte(Comparable)}
*
- {@link FluentComparableAssertion#isBetween(Comparable,Comparable) isBetween(Comparable,Comparable)}
*
* - {@link FluentObjectAssertion}
*
* - {@link FluentObjectAssertion#isExists() isExists()}
*
- {@link FluentObjectAssertion#is(Object) is(Object)}
*
- {@link FluentObjectAssertion#is(Predicate) is(Predicate)}
*
- {@link FluentObjectAssertion#isNot(Object) isNot(Object)}
*
- {@link FluentObjectAssertion#isAny(Object...) isAny(Object...)}
*
- {@link FluentObjectAssertion#isNotAny(Object...) isNotAny(Object...)}
*
- {@link FluentObjectAssertion#isNull() isNull()}
*
- {@link FluentObjectAssertion#isNotNull() isNotNull()}
*
- {@link FluentObjectAssertion#isString(String) isString(String)}
*
- {@link FluentObjectAssertion#isJson(String) isJson(String)}
*
- {@link FluentObjectAssertion#isSame(Object) isSame(Object)}
*
- {@link FluentObjectAssertion#isSameJsonAs(Object) isSameJsonAs(Object)}
*
- {@link FluentObjectAssertion#isSameSortedJsonAs(Object) isSameSortedJsonAs(Object)}
*
- {@link FluentObjectAssertion#isSameSerializedAs(Object, WriterSerializer) isSameSerializedAs(Object, WriterSerializer)}
*
- {@link FluentObjectAssertion#isType(Class) isType(Class)}
*
- {@link FluentObjectAssertion#isExactType(Class) isExactType(Class)}
*
*
*
* Transform Methods:
*
*
* - {@link FluentDateAssertion}
*
* - {@link FluentDateAssertion#asEpochMillis() asEpochMillis()}
*
- {@link FluentDateAssertion#asEpochSeconds() asEpochSeconds()}
*
* - {@link FluentObjectAssertion}
*
* - {@link FluentObjectAssertion#asString() asString()}
*
- {@link FluentObjectAssertion#asString(WriterSerializer) asString(WriterSerializer)}
*
- {@link FluentObjectAssertion#asString(Function) asString(Function)}
*
- {@link FluentObjectAssertion#asJson() asJson()}
*
- {@link FluentObjectAssertion#asJsonSorted() asJsonSorted()}
*
- {@link FluentObjectAssertion#asTransformed(Function) asApplied(Function)}
*
- {@link FluentObjectAssertion#asAny() asAny()}
*
*
*
* Configuration Methods:
*
*
* - {@link Assertion}
*
* - {@link Assertion#setMsg(String, Object...) setMsg(String, Object...)}
*
- {@link Assertion#setOut(PrintStream) setOut(PrintStream)}
*
- {@link Assertion#setSilent() setSilent()}
*
- {@link Assertion#setStdOut() setStdOut()}
*
- {@link Assertion#setThrowable(Class) setThrowable(Class)}
*
*
*
* See Also:
*
* @param The return type.
*/
@FluentSetters(returns="FluentDateAssertion")
public class FluentDateAssertion extends FluentComparableAssertion {
//-----------------------------------------------------------------------------------------------------------------
// Static
//-----------------------------------------------------------------------------------------------------------------
private static final Messages MESSAGES = Messages.of(FluentDateAssertion.class, "Messages");
private static final String
MSG_unexpectedValue = MESSAGES.getString("unexpectedValue"),
MSG_valueWasNotAfterExpected = MESSAGES.getString("valueWasNotAfterExpected"),
MSG_valueWasNotBeforeExpected = MESSAGES.getString("valueWasNotBeforeExpected");
//-----------------------------------------------------------------------------------------------------------------
// Instance
//-----------------------------------------------------------------------------------------------------------------
/**
* Constructor.
*
* @param value
* The object being tested.
*
Can be null .
* @param returns
* The object to return after a test method is called.
*
If null , the test method returns this object allowing multiple test method calls to be
* used on the same assertion.
*/
public FluentDateAssertion(Date value, R returns) {
this(null, value, returns);
}
/**
* Chained constructor.
*
*
* Used when transforming one assertion into another so that the assertion config can be used by the new assertion.
*
* @param creator
* The assertion that created this assertion.
*
Should be null if this is the top-level assertion.
* @param value
* The object being tested.
*
Can be null .
* @param returns
* The object to return after a test method is called.
*
If null , the test method returns this object allowing multiple test method calls to be
* used on the same assertion.
*/
public FluentDateAssertion(Assertion creator, Date value, R returns) {
super(creator, value, returns);
}
//-----------------------------------------------------------------------------------------------------------------
// Transform methods
//-----------------------------------------------------------------------------------------------------------------
/**
* Returns an long assertion on the epoch milliseconds of this date.
*
*
* If the date is null , the returned assertion is a null assertion
* (meaning {@link FluentLongAssertion#isExists()} returns false ).
*
* @return A new assertion.
*/
public FluentLongAssertion asEpochMillis() {
return new FluentLongAssertion<>(this, valueIsNull() ? null : value().getTime(), returns());
}
/**
* Returns an long assertion on the epoch seconds of this date.
*
*
* If the date is null , the returned assertion is a null assertion
* (meaning {@link FluentLongAssertion#isExists()} returns false ).
*
* @return A new assertion.
*/
public FluentLongAssertion asEpochSeconds() {
return new FluentLongAssertion<>(this, valueIsNull() ? null : value().getTime() / 1000, returns());
}
//-----------------------------------------------------------------------------------------------------------------
// Test methods
//-----------------------------------------------------------------------------------------------------------------
/**
* Asserts that the value equals the specified value at the specified precision.
*
* @param value The value to check against.
* @param precision The precision (e.g. {@link ChronoUnit#SECONDS}).
* @return The fluent return object.
* @throws AssertionError If assertion failed.
*/
public R is(Date value, ChronoUnit precision) throws AssertionError {
if (ne(value(), value, (x,y)->x.toInstant().truncatedTo(precision).equals(y.toInstant().truncatedTo(precision))))
throw error(MSG_unexpectedValue, value, value());
return returns();
}
/**
* Asserts that the value is after the specified value.
*
* @param value The values to check against.
* @return The fluent return object.
* @throws AssertionError If assertion failed.
*/
public R isAfter(Date value) throws AssertionError {
assertArgNotNull("value", value);
if (! (value().after(value)))
throw error(MSG_valueWasNotAfterExpected, value, value());
return returns();
}
/**
* Asserts that the value is after the current date.
*
* @return The fluent return object.
* @throws AssertionError If assertion failed.
*/
public R isAfterNow() throws AssertionError {
return isAfter(new Date());
}
/**
* Asserts that the value is before the specified value.
*
* @param value The values to check against.
* @return The fluent return object.
* @throws AssertionError If assertion failed.
*/
public R isBefore(Date value) throws AssertionError {
assertArgNotNull("value", value);
if (! (value().before(value)))
throw error(MSG_valueWasNotBeforeExpected, value, value());
return returns();
}
/**
* Asserts that the value is before the current date.
*
* @return The fluent return object.
* @throws AssertionError If assertion failed.
*/
public R isBeforeNow() throws AssertionError {
return isBefore(new Date());
}
/**
* Asserts that the value is between (inclusive) the specified upper and lower values.
*
* @param lower The lower value to check against.
* @param upper The upper value to check against.
* @return The fluent return object.
* @throws AssertionError If assertion failed.
*/
public R isBetween(Date lower, Date upper) throws AssertionError {
isExists();
assertArgNotNull("lower", lower);
assertArgNotNull("upper", upper);
isLte(upper);
isGte(lower);
return returns();
}
//-----------------------------------------------------------------------------------------------------------------
// Fluent setters
//-----------------------------------------------------------------------------------------------------------------
//
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentDateAssertion setMsg(String msg, Object...args) {
super.setMsg(msg, args);
return this;
}
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentDateAssertion setOut(PrintStream value) {
super.setOut(value);
return this;
}
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentDateAssertion setSilent() {
super.setSilent();
return this;
}
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentDateAssertion setStdOut() {
super.setStdOut();
return this;
}
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentDateAssertion setThrowable(Class extends java.lang.RuntimeException> value) {
super.setThrowable(value);
return this;
}
//
}