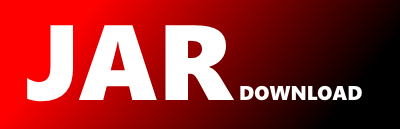
org.apache.juneau.assertions.FluentVersionAssertion Maven / Gradle / Ivy
Show all versions of juneau-assertions Show documentation
// ***************************************************************************************************************************
// * Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements. See the NOTICE file *
// * distributed with this work for additional information regarding copyright ownership. The ASF licenses this file *
// * to you under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance *
// * with the License. You may obtain a copy of the License at *
// * *
// * http://www.apache.org/licenses/LICENSE-2.0 *
// * *
// * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an *
// * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the *
// * specific language governing permissions and limitations under the License. *
// ***************************************************************************************************************************
package org.apache.juneau.assertions;
import java.io.*;
import java.util.function.*;
import org.apache.juneau.*;
import org.apache.juneau.internal.*;
import org.apache.juneau.serializer.*;
/**
* Used for fluent assertion calls against {@link Version} objects.
*
* Example:
*
* // Validates the response expiration is after the current date.
* client
* .get(URL )
* .run()
* .getHeader(ClientVersion.class ).assertVersion().asMajor().isGreaterThanOrEqual(2);
*
*
*
* Test Methods:
*
*
* - {@link FluentComparableAssertion}
*
* - {@link FluentComparableAssertion#isGt(Comparable) isGt(Comparable)}
*
- {@link FluentComparableAssertion#isGte(Comparable) isGte(Comparable)}
*
- {@link FluentComparableAssertion#isLt(Comparable) isLt(Comparable)}
*
- {@link FluentComparableAssertion#isLte(Comparable) isLte(Comparable)}
*
- {@link FluentComparableAssertion#isBetween(Comparable,Comparable) isBetween(Comparable,Comparable)}
*
* - {@link FluentObjectAssertion}
*
* - {@link FluentObjectAssertion#isExists() isExists()}
*
- {@link FluentObjectAssertion#is(Object) is(Object)}
*
- {@link FluentObjectAssertion#is(Predicate) is(Predicate)}
*
- {@link FluentObjectAssertion#isNot(Object) isNot(Object)}
*
- {@link FluentObjectAssertion#isAny(Object...) isAny(Object...)}
*
- {@link FluentObjectAssertion#isNotAny(Object...) isNotAny(Object...)}
*
- {@link FluentObjectAssertion#isNull() isNull()}
*
- {@link FluentObjectAssertion#isNotNull() isNotNull()}
*
- {@link FluentObjectAssertion#isString(String) isString(String)}
*
- {@link FluentObjectAssertion#isJson(String) isJson(String)}
*
- {@link FluentObjectAssertion#isSame(Object) isSame(Object)}
*
- {@link FluentObjectAssertion#isSameJsonAs(Object) isSameJsonAs(Object)}
*
- {@link FluentObjectAssertion#isSameSortedJsonAs(Object) isSameSortedJsonAs(Object)}
*
- {@link FluentObjectAssertion#isSameSerializedAs(Object, WriterSerializer) isSameSerializedAs(Object, WriterSerializer)}
*
- {@link FluentObjectAssertion#isType(Class) isType(Class)}
*
- {@link FluentObjectAssertion#isExactType(Class) isExactType(Class)}
*
*
*
* Transform Methods:
*
*
* - {@link FluentVersionAssertion}
*
* - {@link FluentVersionAssertion#asPart(int) asPart(int)}
*
- {@link FluentVersionAssertion#asMajor() asMajor()}
*
- {@link FluentVersionAssertion#asMinor() asMinor()}
*
- {@link FluentVersionAssertion#asMaintenance() asMaintenance()}
*
* - {@link FluentObjectAssertion}
*
* - {@link FluentObjectAssertion#asString() asString()}
*
- {@link FluentObjectAssertion#asString(WriterSerializer) asString(WriterSerializer)}
*
- {@link FluentObjectAssertion#asString(Function) asString(Function)}
*
- {@link FluentObjectAssertion#asJson() asJson()}
*
- {@link FluentObjectAssertion#asJsonSorted() asJsonSorted()}
*
- {@link FluentObjectAssertion#asTransformed(Function) asApplied(Function)}
*
- {@link FluentObjectAssertion#asAny() asAny()}
*
*
*
* Configuration Methods:
*
*
* - {@link Assertion}
*
* - {@link Assertion#setMsg(String, Object...) setMsg(String, Object...)}
*
- {@link Assertion#setOut(PrintStream) setOut(PrintStream)}
*
- {@link Assertion#setSilent() setSilent()}
*
- {@link Assertion#setStdOut() setStdOut()}
*
- {@link Assertion#setThrowable(Class) setThrowable(Class)}
*
*
*
* See Also:
*
* @param The return type.
*/
@FluentSetters(returns="FluentVersionAssertion")
public class FluentVersionAssertion extends FluentComparableAssertion {
//-----------------------------------------------------------------------------------------------------------------
// Instance
//-----------------------------------------------------------------------------------------------------------------
/**
* Constructor.
*
* @param value
* The object being tested.
*
Can be null .
* @param returns
* The object to return after a test method is called.
*
If null , the test method returns this object allowing multiple test method calls to be
* used on the same assertion.
*/
public FluentVersionAssertion(Version value, R returns) {
this(null, value, returns);
}
/**
* Chained constructor.
*
*
* Used when transforming one assertion into another so that the assertion config can be used by the new assertion.
*
* @param creator
* The assertion that created this assertion.
*
Should be null if this is the top-level assertion.
* @param value
* The object being tested.
*
Can be null .
* @param returns
* The object to return after a test method is called.
*
If null , the test method returns this object allowing multiple test method calls to be
* used on the same assertion.
*/
public FluentVersionAssertion(Assertion creator, Version value, R returns) {
super(creator, value, returns);
}
//-----------------------------------------------------------------------------------------------------------------
// Transform methods
//-----------------------------------------------------------------------------------------------------------------
/**
* Extracts the specified version part (zero-indexed position).
*
* @param index The index of the version part to extract.
* @return This object.
*/
public FluentIntegerAssertion asPart(int index) {
return new FluentIntegerAssertion<>(this, valueIsNull() ? null : value().getPart(index).orElse(null), returns());
}
/**
* Extracts the major part of the version string (index position 0).
*
* @return This object.
*/
public FluentIntegerAssertion asMajor() {
return asPart(0);
}
/**
* Extracts the minor part of the version string (index position 1).
*
* @return This object.
*/
public FluentIntegerAssertion asMinor() {
return asPart(1);
}
/**
* Extracts the maintenance part of the version string (index position 2).
*
* @return This object.
*/
public FluentIntegerAssertion asMaintenance() {
return asPart(2);
}
//-----------------------------------------------------------------------------------------------------------------
// Fluent setters
//-----------------------------------------------------------------------------------------------------------------
//
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentVersionAssertion setMsg(String msg, Object...args) {
super.setMsg(msg, args);
return this;
}
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentVersionAssertion setOut(PrintStream value) {
super.setOut(value);
return this;
}
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentVersionAssertion setSilent() {
super.setSilent();
return this;
}
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentVersionAssertion setStdOut() {
super.setStdOut();
return this;
}
@Override /* GENERATED - org.apache.juneau.assertions.Assertion */
public FluentVersionAssertion setThrowable(Class extends java.lang.RuntimeException> value) {
super.setThrowable(value);
return this;
}
//
}