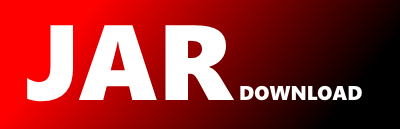
org.apache.kafka.tools.LogDirsCommand Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.kafka.tools;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import joptsimple.OptionSpec;
import joptsimple.OptionSpecBuilder;
import org.apache.kafka.clients.admin.Admin;
import org.apache.kafka.clients.admin.AdminClientConfig;
import org.apache.kafka.clients.admin.DescribeLogDirsResult;
import org.apache.kafka.clients.admin.LogDirDescription;
import org.apache.kafka.clients.admin.ReplicaInfo;
import org.apache.kafka.common.Node;
import org.apache.kafka.common.TopicPartition;
import org.apache.kafka.common.utils.Exit;
import org.apache.kafka.common.utils.Utils;
import org.apache.kafka.server.util.CommandDefaultOptions;
import org.apache.kafka.server.util.CommandLineUtils;
import java.io.IOException;
import java.util.Arrays;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class LogDirsCommand {
public static void main(String... args) {
Exit.exit(mainNoExit(args));
}
static int mainNoExit(String... args) {
try {
execute(args);
return 0;
} catch (TerseException e) {
System.err.println(e.getMessage());
return 1;
} catch (Throwable e) {
System.err.println(e.getMessage());
System.err.println(Utils.stackTrace(e));
return 1;
}
}
private static void execute(String... args) throws Exception {
LogDirsCommandOptions options = new LogDirsCommandOptions(args);
try (Admin adminClient = createAdminClient(options)) {
execute(options, adminClient);
}
}
static void execute(LogDirsCommandOptions options, Admin adminClient) throws Exception {
Set topics = options.topics();
Set clusterBrokers = adminClient.describeCluster().nodes().get().stream().map(Node::id).collect(Collectors.toSet());
Set inputBrokers = options.brokers();
Set existingBrokers = inputBrokers.isEmpty() ? new HashSet<>(clusterBrokers) : new HashSet<>(inputBrokers);
existingBrokers.retainAll(clusterBrokers);
Set nonExistingBrokers = new HashSet<>(inputBrokers);
nonExistingBrokers.removeAll(clusterBrokers);
if (!nonExistingBrokers.isEmpty()) {
throw new TerseException(
String.format(
"ERROR: The given brokers do not exist from --broker-list: %s. Current existent brokers: %s",
commaDelimitedStringFromIntegerSet(nonExistingBrokers),
commaDelimitedStringFromIntegerSet(clusterBrokers)));
} else {
System.out.println("Querying brokers for log directories information");
DescribeLogDirsResult describeLogDirsResult = adminClient.describeLogDirs(existingBrokers);
Map> logDirInfosByBroker = describeLogDirsResult.allDescriptions().get();
System.out.printf(
"Received log directory information from brokers %s%n",
commaDelimitedStringFromIntegerSet(existingBrokers));
System.out.println(formatAsJson(logDirInfosByBroker, topics));
}
}
private static String commaDelimitedStringFromIntegerSet(Set set) {
return set.stream().map(String::valueOf).collect(Collectors.joining(","));
}
private static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy