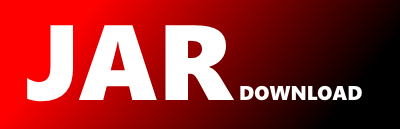
org.apache.hadoop.gateway.topology.Service Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gateway-spi Show documentation
Show all versions of gateway-spi Show documentation
The Service Provider Interface for extending the capabilities of the gateway.
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.hadoop.gateway.topology;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
public class Service {
private String role;
private String name;
private Version version;
private Map params = new LinkedHashMap();
private List urls;
public String getRole() {
return role;
}
public void setRole( String role ) {
this.role = role;
}
public String getName() {
return name;
}
public void setName( String name ) {
this.name = name;
}
public Version getVersion() {
return version;
}
public void setVersion(Version version) {
this.version = version;
}
public List getUrls() {
if ( urls == null ) {
urls = new ArrayList();
}
return urls;
}
public void setUrls( List urls ) {
this.urls = urls;
}
public String getUrl() {
if ( !getUrls().isEmpty() ) {
return getUrls().get( 0 );
}
return null;
}
public void addUrl( String url) {
getUrls().add( url );
}
public Map getParams() {
return params;
}
public Collection getParamsList(){
ArrayList paramList = new ArrayList();
for(Map.Entry entry : params.entrySet()){
Param p = new Param();
p.setName(entry.getKey());
p.setValue(entry.getValue());
paramList.add(p);
}
return paramList;
}
public void setParamsList( Collection params ) {
if( params != null ) {
for( Param param : params ) {
addParam( param );
}
}
}
public void setParams(Map params) {
this.params = params;
}
public void addParam( Param param ) {
params.put(param.getName(), param.getValue());
}
@Override
public boolean equals(Object object) {
if (!(object instanceof Service)) {
return false;
}
Service that = (Service) object;
String thatName = that.getName();
if (thatName != null && !(thatName.equals(name))) {
return false;
}
String thatRole = that.getRole();
if (thatRole != null && !thatRole.equals(role)) {
return false;
}
Version thatVersion = that.getVersion();
if (thatVersion != null && !(thatVersion.equals(version))) {
return false;
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy