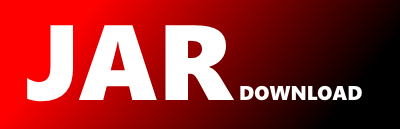
org.apache.maven.continuum.model.project.BuildDefinition Maven / Gradle / Ivy
The newest version!
/*
* $Id$
*/
package org.apache.maven.continuum.model.project;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import java.util.Date;
import org.apache.maven.continuum.model.scm.ChangeFile;
import org.apache.maven.continuum.model.scm.ChangeSet;
import org.apache.maven.continuum.model.scm.ScmResult;
import org.apache.maven.continuum.model.system.Installation;
import org.apache.maven.continuum.model.system.NotificationAddress;
import org.apache.maven.continuum.model.system.Profile;
import org.apache.maven.continuum.model.system.SystemConfiguration;
import org.apache.maven.continuum.project.ContinuumProjectState;
/**
* null
*
* @version $Revision$ $Date$
*/
public class BuildDefinition implements java.io.Serializable {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field id
*/
private int id = 0;
/**
* Field defaultForProject
*/
private boolean defaultForProject = false;
/**
* Field goals
*/
private String goals;
/**
* Field arguments
*/
private String arguments;
/**
* Field buildFile
*/
private String buildFile;
/**
* Field buildFresh
*/
private boolean buildFresh = false;
/**
* Field description
*/
private String description;
/**
* Field type
*/
private String type;
/**
* Field schedule
*/
private Schedule schedule;
/**
* Field profile
*/
private Profile profile;
/**
* Field alwaysBuild
*/
private boolean alwaysBuild = false;
/**
* Field template
*/
private boolean template = false;
//-----------/
//- Methods -/
//-----------/
/**
* Method equals
*
* @param other
*/
public boolean equals(Object other)
{
if ( this == other)
{
return true;
}
if ( !(other instanceof BuildDefinition) )
{
return false;
}
BuildDefinition that = (BuildDefinition) other;
boolean result = true;
result = result && id== that.id;
return result;
} //-- boolean equals(Object)
/**
* Get null
*/
public String getArguments()
{
return this.arguments;
} //-- String getArguments()
/**
* Get null
*/
public String getBuildFile()
{
return this.buildFile;
} //-- String getBuildFile()
/**
* Get description of the buid defintion
*/
public String getDescription()
{
return this.description;
} //-- String getDescription()
/**
* Get null
*/
public String getGoals()
{
return this.goals;
} //-- String getGoals()
/**
* Get null
*/
public int getId()
{
return this.id;
} //-- int getId()
/**
* Get null
*/
public Profile getProfile()
{
return this.profile;
} //-- Profile getProfile()
/**
* Get null
*/
public Schedule getSchedule()
{
return this.schedule;
} //-- Schedule getSchedule()
/**
* Get type of the buid defintion
*/
public String getType()
{
return this.type;
} //-- String getType()
/**
* Method hashCode
*/
public int hashCode()
{
int result = 17;
long tmp;
result = 37 * result + (int) id;
return result;
} //-- int hashCode()
/**
* Get
* true if the build had to be forced even if there
* is no scm change
*
*/
public boolean isAlwaysBuild()
{
return this.alwaysBuild;
} //-- boolean isAlwaysBuild()
/**
* Get
* true if the build is to be smoked and checked
* back out from the scm each build
*
*/
public boolean isBuildFresh()
{
return this.buildFresh;
} //-- boolean isBuildFresh()
/**
* Get null
*/
public boolean isDefaultForProject()
{
return this.defaultForProject;
} //-- boolean isDefaultForProject()
/**
* Get
* true if this buildDefinition is a template
*
*/
public boolean isTemplate()
{
return this.template;
} //-- boolean isTemplate()
/**
* Set
* true if the build had to be forced even if there
* is no scm change
*
*
* @param alwaysBuild
*/
public void setAlwaysBuild(boolean alwaysBuild)
{
this.alwaysBuild = alwaysBuild;
} //-- void setAlwaysBuild(boolean)
/**
* Set null
*
* @param arguments
*/
public void setArguments(String arguments)
{
this.arguments = arguments;
} //-- void setArguments(String)
/**
* Set null
*
* @param buildFile
*/
public void setBuildFile(String buildFile)
{
this.buildFile = buildFile;
} //-- void setBuildFile(String)
/**
* Set
* true if the build is to be smoked and checked
* back out from the scm each build
*
*
* @param buildFresh
*/
public void setBuildFresh(boolean buildFresh)
{
this.buildFresh = buildFresh;
} //-- void setBuildFresh(boolean)
/**
* Set null
*
* @param defaultForProject
*/
public void setDefaultForProject(boolean defaultForProject)
{
this.defaultForProject = defaultForProject;
} //-- void setDefaultForProject(boolean)
/**
* Set description of the buid defintion
*
* @param description
*/
public void setDescription(String description)
{
this.description = description;
} //-- void setDescription(String)
/**
* Set null
*
* @param goals
*/
public void setGoals(String goals)
{
this.goals = goals;
} //-- void setGoals(String)
/**
* Set null
*
* @param id
*/
public void setId(int id)
{
this.id = id;
} //-- void setId(int)
/**
* Set null
*
* @param profile
*/
public void setProfile(Profile profile)
{
this.profile = profile;
} //-- void setProfile(Profile)
/**
* Set null
*
* @param schedule
*/
public void setSchedule(Schedule schedule)
{
this.schedule = schedule;
} //-- void setSchedule(Schedule)
/**
* Set
* true if this buildDefinition is a template
*
*
* @param template
*/
public void setTemplate(boolean template)
{
this.template = template;
} //-- void setTemplate(boolean)
/**
* Set type of the buid defintion
*
* @param type
*/
public void setType(String type)
{
this.type = type;
} //-- void setType(String)
/**
* Method toString
*/
public java.lang.String toString()
{
StringBuffer buf = new StringBuffer();
buf.append( "id = '" );
buf.append( getId() + "'" );
return buf.toString();
} //-- java.lang.String toString()
private String modelEncoding = "UTF-8";
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
public String getModelEncoding()
{
return modelEncoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy