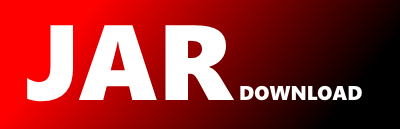
org.apache.maven.continuum.model.project.BuildResult Maven / Gradle / Ivy
The newest version!
/*
* $Id$
*/
package org.apache.maven.continuum.model.project;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import java.util.Date;
import org.apache.maven.continuum.model.scm.ChangeFile;
import org.apache.maven.continuum.model.scm.ChangeSet;
import org.apache.maven.continuum.model.scm.ScmResult;
import org.apache.maven.continuum.model.system.Installation;
import org.apache.maven.continuum.model.system.NotificationAddress;
import org.apache.maven.continuum.model.system.Profile;
import org.apache.maven.continuum.model.system.SystemConfiguration;
import org.apache.maven.continuum.project.ContinuumProjectState;
/**
*
* This class is a single continuum build.
*
*
* @version $Revision$ $Date$
*/
public class BuildResult implements java.io.Serializable {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field project
*/
private Project project;
/**
* Field buildDefinition
*/
private BuildDefinition buildDefinition = null;
/**
* Field id
*/
private int id = 0;
/**
* Field buildNumber
*/
private int buildNumber = 0;
/**
* Field state
*/
private int state = 0;
/**
* Field trigger
*/
private int trigger = 0;
/**
* Field startTime
*/
private long startTime = 0;
/**
* Field endTime
*/
private long endTime = 0;
/**
* Field error
*/
private String error;
/**
* Field success
*/
private boolean success = false;
/**
* Field exitCode
*/
private int exitCode = 0;
/**
* Field scmResult
*/
private ScmResult scmResult;
/**
* Field modifiedDependencies
*/
private java.util.List modifiedDependencies;
//-----------/
//- Methods -/
//-----------/
/**
* Method addModifiedDependency
*
* @param projectDependency
*/
public void addModifiedDependency(ProjectDependency projectDependency)
{
if ( !(projectDependency instanceof ProjectDependency) )
{
throw new ClassCastException( "BuildResult.addModifiedDependencies(projectDependency) parameter must be instanceof " + ProjectDependency.class.getName() );
}
getModifiedDependencies().add( projectDependency );
} //-- void addModifiedDependency(ProjectDependency)
/**
* Method breakProjectAssociation
*
* @param project
*/
public void breakProjectAssociation(Project project)
{
if ( this.project != project )
{
throw new IllegalStateException( "project isn't associated." );
}
this.project = null;
} //-- void breakProjectAssociation(Project)
/**
* Method createProjectAssociation
*
* @param project
*/
public void createProjectAssociation(Project project)
{
if ( this.project != null )
{
breakProjectAssociation( this.project );
}
this.project = project;
} //-- void createProjectAssociation(Project)
/**
* Method equals
*
* @param other
*/
public boolean equals(Object other)
{
if ( this == other)
{
return true;
}
if ( !(other instanceof BuildResult) )
{
return false;
}
BuildResult that = (BuildResult) other;
boolean result = true;
result = result && id== that.id;
return result;
} //-- boolean equals(Object)
/**
* Get null
*/
public BuildDefinition getBuildDefinition()
{
return this.buildDefinition;
} //-- BuildDefinition getBuildDefinition()
/**
* Get null
*/
public int getBuildNumber()
{
return this.buildNumber;
} //-- int getBuildNumber()
/**
* Get null
*/
public long getEndTime()
{
return this.endTime;
} //-- long getEndTime()
/**
* Get null
*/
public String getError()
{
return this.error;
} //-- String getError()
/**
* Get null
*/
public int getExitCode()
{
return this.exitCode;
} //-- int getExitCode()
/**
* Get null
*/
public int getId()
{
return this.id;
} //-- int getId()
/**
* Method getModifiedDependencies
*/
public java.util.List getModifiedDependencies()
{
if ( this.modifiedDependencies == null )
{
this.modifiedDependencies = new java.util.ArrayList();
}
return this.modifiedDependencies;
} //-- java.util.List getModifiedDependencies()
/**
* Get null
*/
public Project getProject()
{
return this.project;
} //-- Project getProject()
/**
* Get null
*/
public ScmResult getScmResult()
{
return this.scmResult;
} //-- ScmResult getScmResult()
/**
* Get null
*/
public long getStartTime()
{
return this.startTime;
} //-- long getStartTime()
/**
* Get null
*/
public int getState()
{
return this.state;
} //-- int getState()
/**
* Get null
*/
public int getTrigger()
{
return this.trigger;
} //-- int getTrigger()
/**
* Method hashCode
*/
public int hashCode()
{
int result = 17;
long tmp;
result = 37 * result + (int) id;
return result;
} //-- int hashCode()
/**
* Get null
*/
public boolean isSuccess()
{
return this.success;
} //-- boolean isSuccess()
/**
* Method removeModifiedDependency
*
* @param projectDependency
*/
public void removeModifiedDependency(ProjectDependency projectDependency)
{
if ( !(projectDependency instanceof ProjectDependency) )
{
throw new ClassCastException( "BuildResult.removeModifiedDependencies(projectDependency) parameter must be instanceof " + ProjectDependency.class.getName() );
}
getModifiedDependencies().remove( projectDependency );
} //-- void removeModifiedDependency(ProjectDependency)
/**
* Set null
*
* @param buildDefinition
*/
public void setBuildDefinition(BuildDefinition buildDefinition)
{
this.buildDefinition = buildDefinition;
} //-- void setBuildDefinition(BuildDefinition)
/**
* Set null
*
* @param buildNumber
*/
public void setBuildNumber(int buildNumber)
{
this.buildNumber = buildNumber;
} //-- void setBuildNumber(int)
/**
* Set null
*
* @param endTime
*/
public void setEndTime(long endTime)
{
this.endTime = endTime;
} //-- void setEndTime(long)
/**
* Set null
*
* @param error
*/
public void setError(String error)
{
this.error = error;
} //-- void setError(String)
/**
* Set null
*
* @param exitCode
*/
public void setExitCode(int exitCode)
{
this.exitCode = exitCode;
} //-- void setExitCode(int)
/**
* Set null
*
* @param id
*/
public void setId(int id)
{
this.id = id;
} //-- void setId(int)
/**
* Set null
*
* @param modifiedDependencies
*/
public void setModifiedDependencies(java.util.List modifiedDependencies)
{
this.modifiedDependencies = modifiedDependencies;
} //-- void setModifiedDependencies(java.util.List)
/**
* Set null
*
* @param project
*/
public void setProject(Project project)
{
if ( this.project != null )
{
this.project.breakBuildResultAssociation( this );
}
this.project = project;
if ( project != null )
{
this.project.createBuildResultAssociation( this );
}
} //-- void setProject(Project)
/**
* Set null
*
* @param scmResult
*/
public void setScmResult(ScmResult scmResult)
{
this.scmResult = scmResult;
} //-- void setScmResult(ScmResult)
/**
* Set null
*
* @param startTime
*/
public void setStartTime(long startTime)
{
this.startTime = startTime;
} //-- void setStartTime(long)
/**
* Set null
*
* @param state
*/
public void setState(int state)
{
this.state = state;
} //-- void setState(int)
/**
* Set null
*
* @param success
*/
public void setSuccess(boolean success)
{
this.success = success;
} //-- void setSuccess(boolean)
/**
* Set null
*
* @param trigger
*/
public void setTrigger(int trigger)
{
this.trigger = trigger;
} //-- void setTrigger(int)
/**
* Method toString
*/
public java.lang.String toString()
{
StringBuffer buf = new StringBuffer();
buf.append( "id = '" );
buf.append( getId() + "'" );
return buf.toString();
} //-- java.lang.String toString()
public String getElapsedTime()
{
return getTimeDifference( startTime, getSystemTime() );
}
public String getDurationTime()
{
return getTimeDifference( startTime, endTime );
}
private long getSystemTime()
{
return java.util.Calendar.getInstance().getTime().getTime();
}
private String getTimeDifference( long startTime, long endTime )
{
long start = startTime;
long end = endTime;
if ( start == 0 )
{
return "";
}
if ( end == 0 )
{
end = getSystemTime();
}
int timeInSeconds = (int) ( ( end - start ) / 1000 );
int days, hours, minutes, seconds;
days = timeInSeconds / 86400;
timeInSeconds = timeInSeconds - ( days * 86400 );
hours = timeInSeconds / 3600;
timeInSeconds = timeInSeconds - ( hours * 3600 );
minutes = timeInSeconds / 60;
timeInSeconds = timeInSeconds - ( minutes * 60 );
seconds = timeInSeconds;
String elapsedTime = "";
if ( days > 0 )
{
elapsedTime = days + " d ";
elapsedTime += hours + " h ";
elapsedTime += minutes + " min ";
elapsedTime += seconds + " sec";
}
else
{
if ( hours > 0 )
{
elapsedTime = hours + " h ";
elapsedTime += minutes + " min ";
elapsedTime += seconds + " sec";
}
else
{
if ( minutes > 0 )
{
elapsedTime = minutes + " min ";
elapsedTime += seconds + " sec";
}
else
{
elapsedTime = seconds + " sec";
}
}
}
return elapsedTime;
}
private String modelEncoding = "UTF-8";
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
public String getModelEncoding()
{
return modelEncoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy