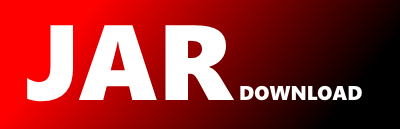
org.apache.maven.continuum.model.system.Profile Maven / Gradle / Ivy
The newest version!
/*
* $Id$
*/
package org.apache.maven.continuum.model.system;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import java.util.Date;
import org.apache.maven.continuum.model.project.BuildDefinition;
import org.apache.maven.continuum.model.project.BuildDefinitionTemplate;
import org.apache.maven.continuum.model.project.BuildResult;
import org.apache.maven.continuum.model.project.ContinuumDatabase;
import org.apache.maven.continuum.model.project.Project;
import org.apache.maven.continuum.model.project.ProjectDependency;
import org.apache.maven.continuum.model.project.ProjectDeveloper;
import org.apache.maven.continuum.model.project.ProjectGroup;
import org.apache.maven.continuum.model.project.ProjectNotifier;
import org.apache.maven.continuum.model.project.Schedule;
import org.apache.maven.continuum.model.scm.ChangeFile;
import org.apache.maven.continuum.model.scm.ChangeSet;
import org.apache.maven.continuum.model.scm.ScmResult;
import org.apache.maven.continuum.project.ContinuumProjectState;
/**
* null
*
* @version $Revision$ $Date$
*/
public class Profile implements java.io.Serializable {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field id
*/
private int id = 0;
/**
* Field active
*/
private boolean active = false;
/**
* Field name
*/
private String name;
/**
* Field description
*/
private String description;
/**
* Field scmMode
*/
private int scmMode = 0;
/**
* Field buildWithoutChanges
*/
private boolean buildWithoutChanges = false;
/**
* Field jdk
*/
private Installation jdk;
/**
* Field builder
*/
private Installation builder;
/**
* Field environmentVariables
*/
private java.util.List environmentVariables;
//-----------/
//- Methods -/
//-----------/
/**
* Method addEnvironmentVariable
*
* @param installation
*/
public void addEnvironmentVariable(Installation installation)
{
if ( !(installation instanceof Installation) )
{
throw new ClassCastException( "Profile.addEnvironmentVariables(installation) parameter must be instanceof " + Installation.class.getName() );
}
getEnvironmentVariables().add( installation );
} //-- void addEnvironmentVariable(Installation)
/**
* Method equals
*
* @param other
*/
public boolean equals(Object other)
{
if ( this == other)
{
return true;
}
if ( !(other instanceof Profile) )
{
return false;
}
Profile that = (Profile) other;
boolean result = true;
result = result && id== that.id;
return result;
} //-- boolean equals(Object)
/**
* Get null
*/
public Installation getBuilder()
{
return this.builder;
} //-- Installation getBuilder()
/**
* Get null
*/
public String getDescription()
{
return this.description;
} //-- String getDescription()
/**
* Method getEnvironmentVariables
*/
public java.util.List getEnvironmentVariables()
{
if ( this.environmentVariables == null )
{
this.environmentVariables = new java.util.ArrayList();
}
return this.environmentVariables;
} //-- java.util.List getEnvironmentVariables()
/**
* Get null
*/
public int getId()
{
return this.id;
} //-- int getId()
/**
* Get null
*/
public Installation getJdk()
{
return this.jdk;
} //-- Installation getJdk()
/**
* Get null
*/
public String getName()
{
return this.name;
} //-- String getName()
/**
* Get null
*/
public int getScmMode()
{
return this.scmMode;
} //-- int getScmMode()
/**
* Method hashCode
*/
public int hashCode()
{
int result = 17;
long tmp;
result = 37 * result + (int) id;
return result;
} //-- int hashCode()
/**
* Get null
*/
public boolean isActive()
{
return this.active;
} //-- boolean isActive()
/**
* Get null
*/
public boolean isBuildWithoutChanges()
{
return this.buildWithoutChanges;
} //-- boolean isBuildWithoutChanges()
/**
* Method removeEnvironmentVariable
*
* @param installation
*/
public void removeEnvironmentVariable(Installation installation)
{
if ( !(installation instanceof Installation) )
{
throw new ClassCastException( "Profile.removeEnvironmentVariables(installation) parameter must be instanceof " + Installation.class.getName() );
}
getEnvironmentVariables().remove( installation );
} //-- void removeEnvironmentVariable(Installation)
/**
* Set null
*
* @param active
*/
public void setActive(boolean active)
{
this.active = active;
} //-- void setActive(boolean)
/**
* Set null
*
* @param buildWithoutChanges
*/
public void setBuildWithoutChanges(boolean buildWithoutChanges)
{
this.buildWithoutChanges = buildWithoutChanges;
} //-- void setBuildWithoutChanges(boolean)
/**
* Set null
*
* @param builder
*/
public void setBuilder(Installation builder)
{
this.builder = builder;
} //-- void setBuilder(Installation)
/**
* Set null
*
* @param description
*/
public void setDescription(String description)
{
this.description = description;
} //-- void setDescription(String)
/**
* Set null
*
* @param environmentVariables
*/
public void setEnvironmentVariables(java.util.List environmentVariables)
{
this.environmentVariables = environmentVariables;
} //-- void setEnvironmentVariables(java.util.List)
/**
* Set null
*
* @param id
*/
public void setId(int id)
{
this.id = id;
} //-- void setId(int)
/**
* Set null
*
* @param jdk
*/
public void setJdk(Installation jdk)
{
this.jdk = jdk;
} //-- void setJdk(Installation)
/**
* Set null
*
* @param name
*/
public void setName(String name)
{
this.name = name;
} //-- void setName(String)
/**
* Set null
*
* @param scmMode
*/
public void setScmMode(int scmMode)
{
this.scmMode = scmMode;
} //-- void setScmMode(int)
/**
* Method toString
*/
public java.lang.String toString()
{
StringBuffer buf = new StringBuffer();
buf.append( "id = '" );
buf.append( getId() + "'" );
return buf.toString();
} //-- java.lang.String toString()
private String modelEncoding = "UTF-8";
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
public String getModelEncoding()
{
return modelEncoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy