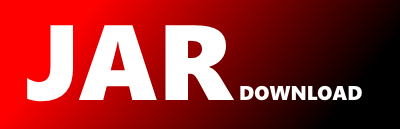
org.apache.maven.doxia.siterenderer.SiteRenderingContext Maven / Gradle / Ivy
package org.apache.maven.doxia.siterenderer;
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import java.io.File;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import org.apache.maven.doxia.site.decoration.DecorationModel;
import org.apache.maven.doxia.site.skin.SkinModel;
import org.codehaus.plexus.util.ReaderFactory;
import org.codehaus.plexus.util.WriterFactory;
/**
* Context for a site rendering.
*
* @author Brett Porter
* @version $Id: SiteRenderingContext.java 1729055 2016-02-08 00:02:32Z hboutemy $
*/
public class SiteRenderingContext
{
private String inputEncoding = ReaderFactory.FILE_ENCODING;
private String outputEncoding = WriterFactory.UTF_8;
private String templateName;
private ClassLoader templateClassLoader;
private Map templateProperties;
private Locale locale = Locale.getDefault();
private List siteLocales = new ArrayList();
private DecorationModel decoration;
private String defaultWindowTitle;
private File skinJarFile;
private SkinModel skinModel;
private boolean usingDefaultTemplate;
private List siteDirectories = new ArrayList();
private Map moduleExcludes;
private List modules = new ArrayList();
private boolean validate;
private Date publishDate;
private File processedContentOutput;
/**
* If input documents should be validated before parsing.
* By default no validation is performed.
*
* @return true if validation is switched on.
* @since 1.1.3
*/
public boolean isValidate()
{
return validate;
}
/**
* Switch on/off validation.
*
* @param validate true to switch on validation.
* @since 1.1.3
*/
public void setValidate( boolean validate )
{
this.validate = validate;
}
/**
* Getter for the field templateName
.
*
* @return a {@link java.lang.String} object.
*/
public String getTemplateName()
{
return templateName;
}
/**
* Getter for the field templateClassLoader
.
*
* @return a {@link java.lang.ClassLoader} object.
*/
public ClassLoader getTemplateClassLoader()
{
return templateClassLoader;
}
/**
* Setter for the field templateClassLoader
.
*
* @param templateClassLoader a {@link java.lang.ClassLoader} object.
*/
public void setTemplateClassLoader( ClassLoader templateClassLoader )
{
this.templateClassLoader = templateClassLoader;
}
/**
* Getter for the field templateProperties
.
*
* @return a {@link java.util.Map} object.
*/
public Map getTemplateProperties()
{
return templateProperties;
}
/**
* Setter for the field templateProperties
.
*
* @param templateProperties a {@link java.util.Map} object.
*/
public void setTemplateProperties( Map templateProperties )
{
this.templateProperties = Collections.unmodifiableMap( templateProperties );
}
/**
* Getter for the field locale
.
*
* @return a {@link java.util.Locale} object.
*/
public Locale getLocale()
{
return locale;
}
/**
* Setter for the field locale
.
*
* @param locale a {@link java.util.Locale} object.
*/
public void setLocale( Locale locale )
{
this.locale = locale;
}
/**
* Getter for the field siteLocales
-
* a list of locales available for this site context.
*
* @return a {@link java.util.List} object with {@link java.util.Locale} objects.
*/
public List getSiteLocales()
{
return siteLocales;
}
/**
* Adds passed locales to the list of site locales.
*
* @param locales List of {@link java.util.Locale} objects to add to the site locales list.
*/
public void addSiteLocales( List locales )
{
siteLocales.addAll( locales );
}
/**
* Getter for the field decoration
.
*
* @return a {@link org.apache.maven.doxia.site.decoration.DecorationModel} object.
*/
public DecorationModel getDecoration()
{
return decoration;
}
/**
* Setter for the field decoration
.
*
* @param decoration a {@link org.apache.maven.doxia.site.decoration.DecorationModel} object.
*/
public void setDecoration( DecorationModel decoration )
{
this.decoration = decoration;
}
/**
* Setter for the field defaultWindowTitle
.
*
* @param defaultWindowTitle a {@link java.lang.String} object.
*/
public void setDefaultWindowTitle( String defaultWindowTitle )
{
this.defaultWindowTitle = defaultWindowTitle;
}
/**
* Getter for the field defaultWindowTitle
.
*
* @return a {@link java.lang.String} object.
*/
public String getDefaultWindowTitle()
{
return defaultWindowTitle;
}
/**
* Getter for the field skinJarFile
.
*
* @return a {@link java.io.File} object.
*/
public File getSkinJarFile()
{
return skinJarFile;
}
/**
* Setter for the field skinJarFile
.
*
* @param skinJarFile a {@link java.io.File} object.
*/
public void setSkinJarFile( File skinJarFile )
{
this.skinJarFile = skinJarFile;
}
/**
* Getter for the field skinModel
.
*
* @return a {@link SkinModel} object.
*/
public SkinModel getSkinModel()
{
return skinModel;
}
/**
* Setter for the field skinModel
.
*
* @param skinModel a {@link SkinModel} object.
*/
public void setSkinModel( SkinModel skinModel )
{
this.skinModel = skinModel;
}
/**
* Setter for the field templateName
.
*
* @param templateName a {@link java.lang.String} object.
*/
public void setTemplateName( String templateName )
{
this.templateName = templateName;
}
/**
* Setter for the field usingDefaultTemplate
.
*
* @param usingDefaultTemplate a boolean.
*/
public void setUsingDefaultTemplate( boolean usingDefaultTemplate )
{
this.usingDefaultTemplate = usingDefaultTemplate;
}
/**
* isUsingDefaultTemplate.
*
* @return a boolean.
*/
public boolean isUsingDefaultTemplate()
{
return usingDefaultTemplate;
}
/**
* Add a site directory, expected to have a Doxia Site layout, ie one directory per Doxia parser module containing
* files with parser extension. Typical values are src/site
or target/generated-site
.
*
* @param siteDirectory a {@link java.io.File} object.
*/
public void addSiteDirectory( File siteDirectory )
{
this.siteDirectories.add( siteDirectory );
}
/**
* Add a extra-module source directory: used for Maven 1.x ${basedir}/xdocs
layout, which contains
* xdoc
and fml
.
*
* @param moduleBasedir The base directory for module's source files.
* @param moduleParserId module's Doxia parser id.
*/
public void addModuleDirectory( File moduleBasedir, String moduleParserId )
{
this.modules.add( new ExtraDoxiaModuleReference( moduleParserId, moduleBasedir ) );
}
/**
* Getter for the field siteDirectories
.
*
* @return List of site directories files.
*/
public List getSiteDirectories()
{
return siteDirectories;
}
/**
* Getter for the field modules
.
*
* @return a {@link java.util.List} object.
*/
public List getModules()
{
return modules;
}
/**
* Getter for the field moduleExcludes
.
*
* @return a map defining exclude patterns (comma separated) by parser id.
*/
public Map getModuleExcludes()
{
return moduleExcludes;
}
/**
* Setter for the field moduleExcludes
.
*
* @param moduleExcludes a {@link java.util.Map} object.
*/
public void setModuleExcludes( Map moduleExcludes )
{
this.moduleExcludes = moduleExcludes;
}
/**
* Getter for the field inputEncoding
.
*
* @return a {@link java.lang.String} object.
*/
public String getInputEncoding()
{
return inputEncoding;
}
/**
* Setter for the field inputEncoding
.
*
* @param inputEncoding a {@link java.lang.String} object.
*/
public void setInputEncoding( String inputEncoding )
{
this.inputEncoding = inputEncoding;
}
/**
* Getter for the field outputEncoding
.
*
* @return a {@link java.lang.String} object.
*/
public String getOutputEncoding()
{
return outputEncoding;
}
/**
* Setter for the field outputEncoding
.
*
* @param outputEncoding a {@link java.lang.String} object.
*/
public void setOutputEncoding( String outputEncoding )
{
this.outputEncoding = outputEncoding;
}
/**
* If you want to specify a specific publish date instead of the current date.
*
* @return the publish date, can be {@code null}
*/
public Date getPublishDate()
{
return publishDate;
}
/**
* Specify a specific publish date instead of the current date.
*
* @param publishDate the publish date
*/
public void setPublishDate( Date publishDate )
{
this.publishDate = publishDate;
}
/**
* Directory where to save content after Velocity processing (*.vm
), but before parsing it with Doxia.
*
* @return not null if the documents are to be saved
*/
public File getProcessedContentOutput()
{
return processedContentOutput;
}
/**
* Where to (eventually) save content after Velocity processing (*.vm
), but before parsing it with
* Doxia?
*
* @param processedContentOutput not null if the documents are to be saved
*/
public void setProcessedContentOutput( File processedContentOutput )
{
this.processedContentOutput = processedContentOutput;
}
}