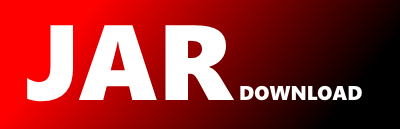
org.apache.maven.plugin.tools.model.Mojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-plugin-tools-model Show documentation
Show all versions of maven-plugin-tools-model Show documentation
The Maven Plugin Metadata Model provides an API to play with the Metadata model.
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello 1.8.1,
// any modifications will be overwritten.
// ==============================================================
package org.apache.maven.plugin.tools.model;
/**
* Mojo descriptor definition.
*
* @version $Revision$ $Date$
*/
@SuppressWarnings( "all" )
public class Mojo
implements java.io.Serializable
{
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* The name of the goal used to invoke this mojo.
*/
private String goal;
/**
* The phase to which this mojo should be bound by default.
*/
private String phase;
/**
* Whether this mojo operates as an aggregator when the reactor
* is run. That is, only runs once.
*
*/
private boolean aggregator = false;
/**
* The scope of dependencies that this mojo requires to have
* resolved.
*/
private String requiresDependencyResolution;
/**
* Whether this mojo requires a project instance in order to
* execute.
*/
private boolean requiresProject = false;
/**
* Whether this mojo requires a reports section in the POM.
*/
private boolean requiresReports = false;
/**
* Whether this mojo requires online mode to operate normally.
*/
private boolean requiresOnline = false;
/**
* Whether this mojo's configuration should propagate down the
* POM inheritance chain by default.
*
*/
private boolean inheritByDefault = false;
/**
* If true, this mojo can only be directly invoked (eg.
* specified directly on the command line).
*
*/
private boolean requiresDirectInvocation = false;
/**
* Information about a sub-execution of the Maven lifecycle
* which should be processed.
*/
private LifecycleExecution execution;
/**
* Field components.
*/
private java.util.List components;
/**
* Field parameters.
*/
private java.util.List parameters;
/**
* The description for this parameter.
*/
private String description;
/**
* A deprecation message for this mojo parameter.
*/
private String deprecation;
/**
* Version when the mojo was added to the API.
*/
private String since;
/**
* The target/method within the script to call when this mojo
* executes.
*/
private String call;
//-----------/
//- Methods -/
//-----------/
/**
* Method addComponent.
*
* @param component
*/
public void addComponent( Component component )
{
getComponents().add( component );
} //-- void addComponent( Component )
/**
* Method addParameter.
*
* @param parameter
*/
public void addParameter( Parameter parameter )
{
getParameters().add( parameter );
} //-- void addParameter( Parameter )
/**
* Get the target/method within the script to call when this
* mojo executes.
*
* @return String
*/
public String getCall()
{
return this.call;
} //-- String getCall()
/**
* Method getComponents.
*
* @return List
*/
public java.util.List getComponents()
{
if ( this.components == null )
{
this.components = new java.util.ArrayList();
}
return this.components;
} //-- java.util.List getComponents()
/**
* Get a deprecation message for this mojo parameter.
*
* @return String
*/
public String getDeprecation()
{
return this.deprecation;
} //-- String getDeprecation()
/**
* Get the description for this parameter.
*
* @return String
*/
public String getDescription()
{
return this.description;
} //-- String getDescription()
/**
* Get information about a sub-execution of the Maven lifecycle
* which should be processed.
*
* @return LifecycleExecution
*/
public LifecycleExecution getExecution()
{
return this.execution;
} //-- LifecycleExecution getExecution()
/**
* Get the name of the goal used to invoke this mojo.
*
* @return String
*/
public String getGoal()
{
return this.goal;
} //-- String getGoal()
/**
* Method getParameters.
*
* @return List
*/
public java.util.List getParameters()
{
if ( this.parameters == null )
{
this.parameters = new java.util.ArrayList();
}
return this.parameters;
} //-- java.util.List getParameters()
/**
* Get the phase to which this mojo should be bound by default.
*
* @return String
*/
public String getPhase()
{
return this.phase;
} //-- String getPhase()
/**
* Get the scope of dependencies that this mojo requires to
* have resolved.
*
* @return String
*/
public String getRequiresDependencyResolution()
{
return this.requiresDependencyResolution;
} //-- String getRequiresDependencyResolution()
/**
* Get version when the mojo was added to the API.
*
* @return String
*/
public String getSince()
{
return this.since;
} //-- String getSince()
/**
* Get whether this mojo operates as an aggregator when the
* reactor is run. That is, only runs once.
*
* @return boolean
*/
public boolean isAggregator()
{
return this.aggregator;
} //-- boolean isAggregator()
/**
* Get whether this mojo's configuration should propagate down
* the POM inheritance chain by default.
*
* @return boolean
*/
public boolean isInheritByDefault()
{
return this.inheritByDefault;
} //-- boolean isInheritByDefault()
/**
* Get if true, this mojo can only be directly invoked (eg.
* specified directly on the command line).
*
* @return boolean
*/
public boolean isRequiresDirectInvocation()
{
return this.requiresDirectInvocation;
} //-- boolean isRequiresDirectInvocation()
/**
* Get whether this mojo requires online mode to operate
* normally.
*
* @return boolean
*/
public boolean isRequiresOnline()
{
return this.requiresOnline;
} //-- boolean isRequiresOnline()
/**
* Get whether this mojo requires a project instance in order
* to execute.
*
* @return boolean
*/
public boolean isRequiresProject()
{
return this.requiresProject;
} //-- boolean isRequiresProject()
/**
* Get whether this mojo requires a reports section in the POM.
*
* @return boolean
*/
public boolean isRequiresReports()
{
return this.requiresReports;
} //-- boolean isRequiresReports()
/**
* Method removeComponent.
*
* @param component
*/
public void removeComponent( Component component )
{
getComponents().remove( component );
} //-- void removeComponent( Component )
/**
* Method removeParameter.
*
* @param parameter
*/
public void removeParameter( Parameter parameter )
{
getParameters().remove( parameter );
} //-- void removeParameter( Parameter )
/**
* Set whether this mojo operates as an aggregator when the
* reactor is run. That is, only runs once.
*
* @param aggregator
*/
public void setAggregator( boolean aggregator )
{
this.aggregator = aggregator;
} //-- void setAggregator( boolean )
/**
* Set the target/method within the script to call when this
* mojo executes.
*
* @param call
*/
public void setCall( String call )
{
this.call = call;
} //-- void setCall( String )
/**
* Set list of plexus components required by this mojo.
*
* @param components
*/
public void setComponents( java.util.List components )
{
this.components = components;
} //-- void setComponents( java.util.List )
/**
* Set a deprecation message for this mojo parameter.
*
* @param deprecation
*/
public void setDeprecation( String deprecation )
{
this.deprecation = deprecation;
} //-- void setDeprecation( String )
/**
* Set the description for this parameter.
*
* @param description
*/
public void setDescription( String description )
{
this.description = description;
} //-- void setDescription( String )
/**
* Set information about a sub-execution of the Maven lifecycle
* which should be processed.
*
* @param execution
*/
public void setExecution( LifecycleExecution execution )
{
this.execution = execution;
} //-- void setExecution( LifecycleExecution )
/**
* Set the name of the goal used to invoke this mojo.
*
* @param goal
*/
public void setGoal( String goal )
{
this.goal = goal;
} //-- void setGoal( String )
/**
* Set whether this mojo's configuration should propagate down
* the POM inheritance chain by default.
*
* @param inheritByDefault
*/
public void setInheritByDefault( boolean inheritByDefault )
{
this.inheritByDefault = inheritByDefault;
} //-- void setInheritByDefault( boolean )
/**
* Set list of parameters used by this mojo.
*
* @param parameters
*/
public void setParameters( java.util.List parameters )
{
this.parameters = parameters;
} //-- void setParameters( java.util.List )
/**
* Set the phase to which this mojo should be bound by default.
*
* @param phase
*/
public void setPhase( String phase )
{
this.phase = phase;
} //-- void setPhase( String )
/**
* Set the scope of dependencies that this mojo requires to
* have resolved.
*
* @param requiresDependencyResolution
*/
public void setRequiresDependencyResolution( String requiresDependencyResolution )
{
this.requiresDependencyResolution = requiresDependencyResolution;
} //-- void setRequiresDependencyResolution( String )
/**
* Set if true, this mojo can only be directly invoked (eg.
* specified directly on the command line).
*
* @param requiresDirectInvocation
*/
public void setRequiresDirectInvocation( boolean requiresDirectInvocation )
{
this.requiresDirectInvocation = requiresDirectInvocation;
} //-- void setRequiresDirectInvocation( boolean )
/**
* Set whether this mojo requires online mode to operate
* normally.
*
* @param requiresOnline
*/
public void setRequiresOnline( boolean requiresOnline )
{
this.requiresOnline = requiresOnline;
} //-- void setRequiresOnline( boolean )
/**
* Set whether this mojo requires a project instance in order
* to execute.
*
* @param requiresProject
*/
public void setRequiresProject( boolean requiresProject )
{
this.requiresProject = requiresProject;
} //-- void setRequiresProject( boolean )
/**
* Set whether this mojo requires a reports section in the POM.
*
* @param requiresReports
*/
public void setRequiresReports( boolean requiresReports )
{
this.requiresReports = requiresReports;
} //-- void setRequiresReports( boolean )
/**
* Set version when the mojo was added to the API.
*
* @param since
*/
public void setSince( String since )
{
this.since = since;
} //-- void setSince( String )
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy