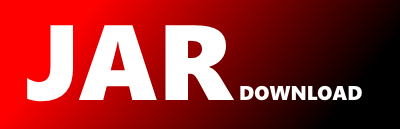
org.apache.maven.plugins.changes.model.Action Maven / Gradle / Ivy
Show all versions of maven-changes-plugin Show documentation
/*
=================== DO NOT EDIT THIS FILE ====================
Generated by Modello 1.0.1 on 2014-04-10 21:00:55,
any modifications will be overwritten.
==============================================================
*/
package org.apache.maven.plugins.changes.model;
/**
*
* A single action done on the project, during this
* release.
*
*
* @version $Revision$ $Date$
*/
public class Action
implements java.io.Serializable
{
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
*
* A short description of the action taken.
*
*/
private String action;
/**
*
*
* Name of developer who committed the
* change.
* This can be either the id of the developer,
* as specified in the developers section of the pom.xml file,
* or the name of the developer. If you generate a changes
* report and specify the id of the developer, a link is
* created to that developer in the team-list.html page.
*
*
*/
private String dev;
/**
*
* Name of the person to be credited for this
* change. This can be used when a patch is submitted by a
* non-committer.
*
*/
private String dueTo;
/**
*
* Email of the person to be credited for this
* change.
*
*/
private String dueToEmail;
/**
*
*
* Id of the issue related to this change.
* This is the id in your issue tracking system.
* The Changes plugin will generate a URL out
* of this id. The URL is constructed using the value of the
* issueLinkTemplate parameter.
* See the changes-report mojo for more
* details.
*
*
*/
private String issue;
/**
*
*
* Supported action types are the following:
*
* - add : added functionnality to the
* project.
* - fix : bug fix for the project.
* - update : updated some part of the
* project.
* - remove : removed some functionnality from
* the project.
*
*
*
*/
private String type;
/**
*
*
* Id of issue tracking system. If empty
* 'default' value will be use.
* The Changes plugin will generate a URL out
* of this id. The URL is constructed using the value of the
* issueLinkTemplatePerSystem parameter.
* See the changes-report mojo for more
* details.
*
*
*/
private String system;
/**
* fix date.
*/
private String date;
/**
* Field fixedIssues.
*/
private java.util.List fixedIssues;
/**
* Field dueTos.
*/
private java.util.List dueTos;
//-----------/
//- Methods -/
//-----------/
/**
* Method addDueTo.
*
* @param dueTo
*/
public void addDueTo( DueTo dueTo )
{
if ( !(dueTo instanceof DueTo) )
{
throw new ClassCastException( "Action.addDueTos(dueTo) parameter must be instanceof " + DueTo.class.getName() );
}
getDueTos().add( dueTo );
} //-- void addDueTo( DueTo )
/**
* Method addFixedIssue.
*
* @param fixedIssue
*/
public void addFixedIssue( FixedIssue fixedIssue )
{
if ( !(fixedIssue instanceof FixedIssue) )
{
throw new ClassCastException( "Action.addFixedIssues(fixedIssue) parameter must be instanceof " + FixedIssue.class.getName() );
}
getFixedIssues().add( fixedIssue );
} //-- void addFixedIssue( FixedIssue )
/**
* Get a short description of the action taken.
*
* @return String
*/
public String getAction()
{
return this.action;
} //-- String getAction()
/**
* Get fix date.
*
* @return String
*/
public String getDate()
{
return this.date;
} //-- String getDate()
/**
* Get Name of developer who committed the change.
* This can be either the id of the developer,
* as specified in the developers section of the pom.xml file,
* or the name of the developer. If you generate a changes
* report and specify the id of the developer, a link is
* created to that developer in the team-list.html page.
*
* @return String
*/
public String getDev()
{
return this.dev;
} //-- String getDev()
/**
* Get name of the person to be credited for this change. This
* can be used when a patch is submitted by a non-committer.
*
* @return String
*/
public String getDueTo()
{
return this.dueTo;
} //-- String getDueTo()
/**
* Get email of the person to be credited for this change.
*
* @return String
*/
public String getDueToEmail()
{
return this.dueToEmail;
} //-- String getDueToEmail()
/**
* Method getDueTos.
*
* @return List
*/
public java.util.List getDueTos()
{
if ( this.dueTos == null )
{
this.dueTos = new java.util.ArrayList();
}
return this.dueTos;
} //-- java.util.List getDueTos()
/**
* Method getFixedIssues.
*
* @return List
*/
public java.util.List getFixedIssues()
{
if ( this.fixedIssues == null )
{
this.fixedIssues = new java.util.ArrayList();
}
return this.fixedIssues;
} //-- java.util.List getFixedIssues()
/**
* Get Id of the issue related to this change. This is the
* id in your issue tracking system.
* The Changes plugin will generate a URL out
* of this id. The URL is constructed using the value of the
* issueLinkTemplate parameter.
* See the changes-report mojo for more
* details.
*
* @return String
*/
public String getIssue()
{
return this.issue;
} //-- String getIssue()
/**
* Get Id of issue tracking system. If empty 'default' value
* will be use.
* The Changes plugin will generate a URL out
* of this id. The URL is constructed using the value of the
* issueLinkTemplatePerSystem parameter.
* See the changes-report mojo for more
* details.
*
* @return String
*/
public String getSystem()
{
return this.system;
} //-- String getSystem()
/**
* Get supported action types are the following:
*
* - add : added functionnality to the
* project.
* - fix : bug fix for the project.
* - update : updated some part of the
* project.
* - remove : removed some functionnality from
* the project.
*
*
* @return String
*/
public String getType()
{
return this.type;
} //-- String getType()
/**
* Method removeDueTo.
*
* @param dueTo
*/
public void removeDueTo( DueTo dueTo )
{
if ( !(dueTo instanceof DueTo) )
{
throw new ClassCastException( "Action.removeDueTos(dueTo) parameter must be instanceof " + DueTo.class.getName() );
}
getDueTos().remove( dueTo );
} //-- void removeDueTo( DueTo )
/**
* Method removeFixedIssue.
*
* @param fixedIssue
*/
public void removeFixedIssue( FixedIssue fixedIssue )
{
if ( !(fixedIssue instanceof FixedIssue) )
{
throw new ClassCastException( "Action.removeFixedIssues(fixedIssue) parameter must be instanceof " + FixedIssue.class.getName() );
}
getFixedIssues().remove( fixedIssue );
} //-- void removeFixedIssue( FixedIssue )
/**
* Set a short description of the action taken.
*
* @param action
*/
public void setAction( String action )
{
this.action = action;
} //-- void setAction( String )
/**
* Set fix date.
*
* @param date
*/
public void setDate( String date )
{
this.date = date;
} //-- void setDate( String )
/**
* Set Name of developer who committed the change.
* This can be either the id of the developer,
* as specified in the developers section of the pom.xml file,
* or the name of the developer. If you generate a changes
* report and specify the id of the developer, a link is
* created to that developer in the team-list.html page.
*
* @param dev
*/
public void setDev( String dev )
{
this.dev = dev;
} //-- void setDev( String )
/**
* Set name of the person to be credited for this change. This
* can be used when a patch is submitted by a non-committer.
*
* @param dueTo
*/
public void setDueTo( String dueTo )
{
this.dueTo = dueTo;
} //-- void setDueTo( String )
/**
* Set email of the person to be credited for this change.
*
* @param dueToEmail
*/
public void setDueToEmail( String dueToEmail )
{
this.dueToEmail = dueToEmail;
} //-- void setDueToEmail( String )
/**
* Set a list of contributors for this issue.
*
* @param dueTos
*/
public void setDueTos( java.util.List dueTos )
{
this.dueTos = dueTos;
} //-- void setDueTos( java.util.List )
/**
* Set a list of fix issues.
*
* @param fixedIssues
*/
public void setFixedIssues( java.util.List fixedIssues )
{
this.fixedIssues = fixedIssues;
} //-- void setFixedIssues( java.util.List )
/**
* Set Id of the issue related to this change. This is the
* id in your issue tracking system.
* The Changes plugin will generate a URL out
* of this id. The URL is constructed using the value of the
* issueLinkTemplate parameter.
* See the changes-report mojo for more
* details.
*
* @param issue
*/
public void setIssue( String issue )
{
this.issue = issue;
} //-- void setIssue( String )
/**
* Set Id of issue tracking system. If empty 'default' value
* will be use.
* The Changes plugin will generate a URL out
* of this id. The URL is constructed using the value of the
* issueLinkTemplatePerSystem parameter.
* See the changes-report mojo for more
* details.
*
* @param system
*/
public void setSystem( String system )
{
this.system = system;
} //-- void setSystem( String )
/**
* Set supported action types are the following:
*
* - add : added functionnality to the
* project.
* - fix : bug fix for the project.
* - update : updated some part of the
* project.
* - remove : removed some functionnality from
* the project.
*
*
* @param type
*/
public void setType( String type )
{
this.type = type;
} //-- void setType( String )
}