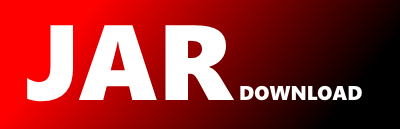
org.apache.maven.plugin.failsafe.util.FailsafeSummaryXmlUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-failsafe-plugin Show documentation
Show all versions of maven-failsafe-plugin Show documentation
Maven Failsafe MOJO in maven-failsafe-plugin.
package org.apache.maven.plugin.failsafe.util;
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import org.apache.commons.io.IOUtils;
import org.apache.maven.surefire.suite.RunResult;
import org.w3c.dom.Node;
import org.xml.sax.InputSource;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathFactory;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Locale;
import static java.lang.Boolean.parseBoolean;
import static java.lang.Integer.parseInt;
import static org.apache.commons.lang3.StringEscapeUtils.escapeXml10;
import static org.apache.commons.lang3.StringEscapeUtils.unescapeXml;
import static org.apache.maven.surefire.util.internal.StringUtils.UTF_8;
import static org.apache.maven.surefire.util.internal.StringUtils.isBlank;
/**
* @author Tibor Digana (tibor17)
* @since 2.20
*/
public final class FailsafeSummaryXmlUtils
{
private static final String FAILSAFE_SUMMARY_XML_SCHEMA_LOCATION =
"https://maven.apache.org/surefire/maven-surefire-plugin/xsd/failsafe-summary.xsd";
private static final String FAILSAFE_SUMMARY_XML_NIL_ATTR =
" xsi:nil=\"true\" xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\"";
private static final String FAILSAFE_SUMMARY_XML_TEMPLATE =
"\n"
+ "\n"
+ " %d \n"
+ " %d \n"
+ " %d \n"
+ " %d \n"
+ " %s \n"
+ " ";
private FailsafeSummaryXmlUtils()
{
throw new IllegalStateException( "No instantiable constructor." );
}
public static RunResult toRunResult( File failsafeSummaryXml ) throws Exception
{
XPathFactory xpathFactory = XPathFactory.newInstance();
XPath xpath = xpathFactory.newXPath();
FileInputStream is = new FileInputStream( failsafeSummaryXml );
try
{
Node root = (Node) xpath.evaluate( "/", new InputSource( is ), XPathConstants.NODE );
String completed = xpath.evaluate( "/failsafe-summary/completed", root );
String errors = xpath.evaluate( "/failsafe-summary/errors", root );
String failures = xpath.evaluate( "/failsafe-summary/failures", root );
String skipped = xpath.evaluate( "/failsafe-summary/skipped", root );
String failureMessage = xpath.evaluate( "/failsafe-summary/failureMessage", root );
String timeout = xpath.evaluate( "/failsafe-summary/@timeout", root );
return new RunResult( parseInt( completed ), parseInt( errors ), parseInt( failures ), parseInt( skipped ),
isBlank( failureMessage ) ? null : unescapeXml( failureMessage ),
parseBoolean( timeout )
);
}
finally
{
is.close();
}
}
public static void fromRunResultToFile( RunResult fromRunResult, File toFailsafeSummaryXml )
throws IOException
{
String failure = fromRunResult.getFailure();
String xml = String.format( Locale.ROOT, FAILSAFE_SUMMARY_XML_TEMPLATE,
fromRunResult.getFailsafeCode(),
String.valueOf( fromRunResult.isTimeout() ),
fromRunResult.getCompletedCount(),
fromRunResult.getErrors(),
fromRunResult.getFailures(),
fromRunResult.getSkipped(),
isBlank( failure ) ? FAILSAFE_SUMMARY_XML_NIL_ATTR : "",
isBlank( failure ) ? "" : escapeXml10( failure )
);
FileOutputStream os = new FileOutputStream( toFailsafeSummaryXml );
try
{
IOUtils.write( xml, os, UTF_8 );
}
finally
{
os.close();
}
}
public static void writeSummary( RunResult mergedSummary, File mergedSummaryFile, boolean inProgress )
throws Exception
{
if ( !mergedSummaryFile.getParentFile().isDirectory() )
{
//noinspection ResultOfMethodCallIgnored
mergedSummaryFile.getParentFile().mkdirs();
}
if ( mergedSummaryFile.exists() && inProgress )
{
RunResult runResult = toRunResult( mergedSummaryFile );
mergedSummary = mergedSummary.aggregate( runResult );
}
fromRunResultToFile( mergedSummary, mergedSummaryFile );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy