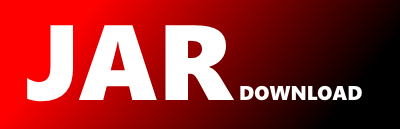
org.apache.maven.plugins.invoker.InvokerProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-invoker-plugin Show documentation
Show all versions of maven-invoker-plugin Show documentation
The Maven Invoker Plugin is used to run a set of Maven projects. The plugin can determine whether each project
execution is successful, and optionally can verify the output generated from a given project execution.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.maven.plugins.invoker;
import java.io.File;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Properties;
import java.util.function.Consumer;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.maven.shared.invoker.InvocationRequest;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Provides a convenient facade around the invoker.properties
.
*
* @author Benjamin Bentmann
*/
class InvokerProperties {
private final Logger logger = LoggerFactory.getLogger(InvokerProperties.class);
private static final String SELECTOR_PREFIX = "selector.";
private static final Pattern ENVIRONMENT_VARIABLES_PATTERN =
Pattern.compile("invoker\\.environmentVariables\\.([A-Za-z][^.]+)(\\.(\\d+))?");
// default values from Mojo configuration
private Boolean defaultDebug;
private Boolean defaultQuiet;
private List defaultGoals;
private List defaultProfiles;
private String defaultMavenOpts;
private Integer defaultTimeoutInSeconds;
private Map defaultEnvironmentVariables;
private File defaultMavenExecutable;
private Boolean defaultUpdateSnapshots;
private String defaultUserPropertiesFiles;
private enum InvocationProperty {
PROJECT("invoker.project"),
BUILD_RESULT("invoker.buildResult"),
GOALS("invoker.goals"),
PROFILES("invoker.profiles"),
MAVEN_EXECUTABLE("invoker.mavenExecutable"),
MAVEN_OPTS("invoker.mavenOpts"),
FAILURE_BEHAVIOR("invoker.failureBehavior"),
NON_RECURSIVE("invoker.nonRecursive"),
OFFLINE("invoker.offline"),
SYSTEM_PROPERTIES_FILE("invoker.systemPropertiesFile"),
USER_PROPERTIES_FILE("invoker.userPropertiesFile"),
DEBUG("invoker.debug"),
QUIET("invoker.quiet"),
SETTINGS_FILE("invoker.settingsFile"),
TIMEOUT_IN_SECONDS("invoker.timeoutInSeconds"),
UPDATE_SNAPSHOTS("invoker.updateSnapshots");
private final String key;
InvocationProperty(final String s) {
this.key = s;
}
@Override
public String toString() {
return key;
}
}
private enum SelectorProperty {
JAVA_VERSION(".java.version"),
MAVEN_VERSION(".maven.version"),
OS_FAMILY(".os.family");
private final String suffix;
SelectorProperty(String suffix) {
this.suffix = suffix;
}
@Override
public String toString() {
return suffix;
}
}
/**
* The invoker properties being wrapped.
*/
private final Properties properties;
/**
* Creates a new facade for the specified invoker properties. The properties will not be copied, so any changes to
* them will be reflected by the facade.
*
* @param properties The invoker properties to wrap, may be null
if none.
*/
InvokerProperties(Properties properties) {
this.properties = (properties != null) ? properties : new Properties();
}
/**
* Default value for debug
* @param defaultDebug a default value
*/
public void setDefaultDebug(boolean defaultDebug) {
this.defaultDebug = defaultDebug;
}
/**
* Default value for quiet
* @param defaultQuiet a default value
*/
public void setDefaultQuiet(boolean defaultQuiet) {
this.defaultQuiet = defaultQuiet;
}
/**
* Default value for goals
* @param defaultGoals a default value
*/
public void setDefaultGoals(List defaultGoals) {
this.defaultGoals = defaultGoals;
}
/**
* Default value for profiles
* @param defaultProfiles a default value
*/
public void setDefaultProfiles(List defaultProfiles) {
this.defaultProfiles = defaultProfiles;
}
/**
* Default value for mavenExecutable
* @param defaultMavenExecutable a default value
*/
public void setDefaultMavenExecutable(String defaultMavenExecutable) {
if (Objects.nonNull(defaultMavenExecutable) && !defaultMavenExecutable.isEmpty()) {
this.defaultMavenExecutable = new File(defaultMavenExecutable);
}
}
/**
* Default value for mavenOpts
* @param defaultMavenOpts a default value
*/
public void setDefaultMavenOpts(String defaultMavenOpts) {
this.defaultMavenOpts = defaultMavenOpts;
}
/**
* Default value for timeoutInSeconds
* @param defaultTimeoutInSeconds a default value
*/
public void setDefaultTimeoutInSeconds(int defaultTimeoutInSeconds) {
this.defaultTimeoutInSeconds = defaultTimeoutInSeconds;
}
/**
* Default value for environmentVariables
* @param defaultEnvironmentVariables a default value
*/
public void setDefaultEnvironmentVariables(Map defaultEnvironmentVariables) {
this.defaultEnvironmentVariables = defaultEnvironmentVariables;
}
/**
* Default value for updateSnapshots
* @param defaultUpdateSnapshots a default value
*/
public void setDefaultUpdateSnapshots(boolean defaultUpdateSnapshots) {
this.defaultUpdateSnapshots = defaultUpdateSnapshots;
}
/**
* Default value for userPropertiesFile
* @param defaultUserPropertiesFiles a default value
*/
public void setDefaultUserPropertiesFiles(String defaultUserPropertiesFiles) {
this.defaultUserPropertiesFiles = defaultUserPropertiesFiles;
}
/**
* Gets the invoker properties being wrapped.
*
* @return The invoker properties being wrapped, never null
.
*/
public Properties getProperties() {
return this.properties;
}
/**
* Gets the name of the corresponding build job.
*
* @return The name of the build job or an empty string if not set.
*/
public String getJobName() {
return this.properties.getProperty("invoker.name", "");
}
/**
* Gets the description of the corresponding build job.
*
* @return The description of the build job or an empty string if not set.
*/
public String getJobDescription() {
return this.properties.getProperty("invoker.description", "");
}
/**
* Get the corresponding ordinal value
*
* @return The ordinal value
*/
public int getOrdinal() {
return Integer.parseInt(this.properties.getProperty("invoker.ordinal", "0"));
}
/**
* Gets the specification of JRE versions on which this build job should be run.
*
* @return The specification of JRE versions or an empty string if not set.
*/
public String getJreVersion() {
return this.properties.getProperty("invoker.java.version", "");
}
/**
* Gets the specification of JRE versions on which this build job should be run.
*
* @return The specification of JRE versions or an empty string if not set.
*/
public String getJreVersion(int index) {
return this.properties.getProperty(SELECTOR_PREFIX + index + SelectorProperty.JAVA_VERSION, getJreVersion());
}
/**
* Gets the specification of Maven versions on which this build job should be run.
*
* @return The specification of Maven versions on which this build job should be run.
* @since 1.5
*/
public String getMavenVersion() {
return this.properties.getProperty("invoker.maven.version", "");
}
/**
* @param index the selector index
* @return The specification of Maven versions on which this build job should be run.
* @since 3.0.0
*/
public String getMavenVersion(int index) {
return this.properties.getProperty(SELECTOR_PREFIX + index + SelectorProperty.MAVEN_VERSION, getMavenVersion());
}
/**
* Gets the specification of OS families on which this build job should be run.
*
* @return The specification of OS families or an empty string if not set.
*/
public String getOsFamily() {
return this.properties.getProperty("invoker.os.family", "");
}
/**
* Gets the specification of OS families on which this build job should be run.
*
* @param index the selector index
* @return The specification of OS families or an empty string if not set.
* @since 3.0.0
*/
public String getOsFamily(int index) {
return this.properties.getProperty(SELECTOR_PREFIX + index + SelectorProperty.OS_FAMILY, getOsFamily());
}
public Collection getToolchains() {
return getToolchains(Pattern.compile("invoker\\.toolchain\\.([^.]+)\\.(.+)"));
}
public Collection getToolchains(int index) {
return getToolchains(Pattern.compile("selector\\." + index + "\\.invoker\\.toolchain\\.([^.]+)\\.(.+)"));
}
private Collection getToolchains(Pattern p) {
Map toolchains = new HashMap<>();
for (Map.Entry
© 2015 - 2024 Weber Informatics LLC | Privacy Policy