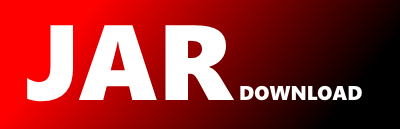
org.apache.maven.plugins.invoker.model.BuildJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-invoker-plugin Show documentation
Show all versions of maven-invoker-plugin Show documentation
The Maven Invoker Plugin is used to run a set of Maven projects. The plugin can determine whether each project
execution is successful, and optionally can verify the output generated from a given project execution.
The newest version!
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello 2.4.0,
// any modifications will be overwritten.
// ==============================================================
package org.apache.maven.plugins.invoker.model;
/**
*
* Describes a build job processed by the Maven Invoker
* Plugin. A build job can consist of a pre-build hook script,
* one ore more invocations of Maven and a post-build hook
* script.
*
*
* @version $Revision$ $Date$
*/
@SuppressWarnings( "all" )
public class BuildJob
implements java.io.Serializable
{
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* The path to the project to build. This path is usually
* relative and can denote both a POM file or a project
* directory.
*/
private String project;
/**
* The name of this build job.
*/
private String name;
/**
* The description of this build job.
*/
private String description;
/**
* The result of this build job.
*/
private String result;
/**
* Any failure message(s) in case this build job failed.
*/
private String failureMessage;
/**
* The number of seconds that this build job took to complete.
*/
private float time = 0.0f;
/**
* The type of the build job.
*/
private String type = "normal";
/**
* BuildJobs will be sorted in the descending order of the
* ordinal. In other words, the BuildJobs with the highest
* numbers will be executed first.
*/
private int ordinal = 0;
/**
* The build log filename.
*/
private String buildlog;
/**
* Field modelEncoding.
*/
private String modelEncoding = "UTF-8";
//-----------/
//- Methods -/
//-----------/
/**
* Get the build log filename.
*
* @return String
*/
public String getBuildlog()
{
return this.buildlog;
} //-- String getBuildlog()
/**
* Get the description of this build job.
*
* @return String
*/
public String getDescription()
{
return this.description;
} //-- String getDescription()
/**
* Get any failure message(s) in case this build job failed.
*
* @return String
*/
public String getFailureMessage()
{
return this.failureMessage;
} //-- String getFailureMessage()
/**
* Get the modelEncoding field.
*
* @return String
*/
public String getModelEncoding()
{
return this.modelEncoding;
} //-- String getModelEncoding()
/**
* Get the name of this build job.
*
* @return String
*/
public String getName()
{
return this.name;
} //-- String getName()
/**
* Get buildJobs will be sorted in the descending order of the
* ordinal. In other words, the BuildJobs with the highest
* numbers will be executed first.
*
* @return int
*/
public int getOrdinal()
{
return this.ordinal;
} //-- int getOrdinal()
/**
* Get the path to the project to build. This path is usually
* relative and can denote both a POM file or a project
* directory.
*
* @return String
*/
public String getProject()
{
return this.project;
} //-- String getProject()
/**
* Get the result of this build job.
*
* @return String
*/
public String getResult()
{
return this.result;
} //-- String getResult()
/**
* Get the number of seconds that this build job took to
* complete.
*
* @return float
*/
public float getTime()
{
return this.time;
} //-- float getTime()
/**
* Get the type of the build job.
*
* @return String
*/
public String getType()
{
return this.type;
} //-- String getType()
/**
* Set the build log filename.
*
* @param buildlog a buildlog object.
*/
public void setBuildlog( String buildlog )
{
this.buildlog = buildlog;
} //-- void setBuildlog( String )
/**
* Set the description of this build job.
*
* @param description a description object.
*/
public void setDescription( String description )
{
this.description = description;
} //-- void setDescription( String )
/**
* Set any failure message(s) in case this build job failed.
*
* @param failureMessage a failureMessage object.
*/
public void setFailureMessage( String failureMessage )
{
this.failureMessage = failureMessage;
} //-- void setFailureMessage( String )
/**
* Set the modelEncoding field.
*
* @param modelEncoding a modelEncoding object.
*/
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
} //-- void setModelEncoding( String )
/**
* Set the name of this build job.
*
* @param name a name object.
*/
public void setName( String name )
{
this.name = name;
} //-- void setName( String )
/**
* Set buildJobs will be sorted in the descending order of the
* ordinal. In other words, the BuildJobs with the highest
* numbers will be executed first.
*
* @param ordinal a ordinal object.
*/
public void setOrdinal( int ordinal )
{
this.ordinal = ordinal;
} //-- void setOrdinal( int )
/**
* Set the path to the project to build. This path is usually
* relative and can denote both a POM file or a project
* directory.
*
* @param project a project object.
*/
public void setProject( String project )
{
this.project = project;
} //-- void setProject( String )
/**
* Set the result of this build job.
*
* @param result a result object.
*/
public void setResult( String result )
{
this.result = result;
} //-- void setResult( String )
/**
* Set the number of seconds that this build job took to
* complete.
*
* @param time a time object.
*/
public void setTime( float time )
{
this.time = time;
} //-- void setTime( float )
/**
* Set the type of the build job.
*
* @param type a type object.
*/
public void setType( String type )
{
this.type = type;
} //-- void setType( String )
/**
* Creates a new empty build job.
*/
public BuildJob()
{
// enables no-arg construction
}
/**
* Creates a new build job with the specified project path.
*
* @param project The path to the project.
*/
public BuildJob( String project )
{
this.project = project;
}
public boolean isNotError()
{
return Result.SUCCESS.equals( result ) || Result.SKIPPED.equals( result );
}
/**
* The various results with which a build job can complete.
*/
public static class Result
{
/**
* The result value corresponding with a successful invocation of Maven and completion of all post-hook scripts.
*/
public static final String SUCCESS = "success";
/**
* The result value corresponding with an invocation that failed before Maven was be invoked.
*/
public static final String FAILURE_PRE_HOOK = "failure-pre-hook";
/**
* The result value corresponding with an invocation that failed while invoking of Maven.
*/
public static final String FAILURE_BUILD = "failure-build";
/**
* The result value corresponding with an invocation that failed after the invocation of Maven.
*/
public static final String FAILURE_POST_HOOK = "failure-post-hook";
/**
* The result value corresponding with an invocation that was skipped.
*/
public static final String SKIPPED = "skipped";
/**
* The result value corresponding with an unexpected error trying to invoke Maven.
*/
public static final String ERROR = "error";
}
/**
* The various types of a build job.
*/
public static class Type
{
/**
* A build job that should be invoked before any non-setup build jobs.
*/
public static final String SETUP = "setup";
/**
* A normal build job.
*/
public static final String NORMAL = "normal";
}
public String toString()
{
StringBuffer buf = new StringBuffer( 128 );
buf.append( "BuildJob[project = \"" );
buf.append( project );
buf.append( "\", type = " );
buf.append( type );
buf.append( ']' );
return buf.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy