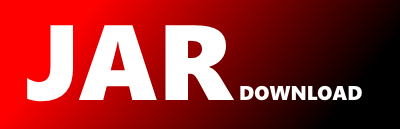
org.apache.maven.plugins.site.render.AbstractSiteRenderingMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-site-plugin Show documentation
Show all versions of maven-site-plugin Show documentation
The Maven Site Plugin is a plugin that generates a site for the current project.
package org.apache.maven.plugins.site.render;
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.doxia.site.decoration.DecorationModel;
import org.apache.maven.doxia.site.decoration.Menu;
import org.apache.maven.doxia.site.decoration.MenuItem;
import org.apache.maven.doxia.siterenderer.DocumentRenderer;
import org.apache.maven.doxia.siterenderer.Renderer;
import org.apache.maven.doxia.siterenderer.RendererException;
import org.apache.maven.doxia.siterenderer.RenderingContext;
import org.apache.maven.doxia.siterenderer.SiteRenderingContext;
import org.apache.maven.doxia.tools.SiteToolException;
import org.apache.maven.execution.MavenSession;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.plugins.site.descriptor.AbstractSiteDescriptorMojo;
import org.apache.maven.reporting.MavenReport;
import org.apache.maven.reporting.exec.MavenReportExecution;
import org.apache.maven.reporting.exec.MavenReportExecutor;
import org.apache.maven.reporting.exec.MavenReportExecutorRequest;
import org.apache.maven.reporting.exec.ReportPlugin;
import org.codehaus.plexus.PlexusConstants;
import org.codehaus.plexus.PlexusContainer;
import org.codehaus.plexus.component.repository.exception.ComponentLookupException;
import org.codehaus.plexus.context.Context;
import org.codehaus.plexus.context.ContextException;
import org.codehaus.plexus.personality.plexus.lifecycle.phase.Contextualizable;
import org.codehaus.plexus.util.ReaderFactory;
import org.codehaus.plexus.util.StringUtils;
/**
* Base class for site rendering mojos.
*
* @author Brett Porter
* @version $Id: AbstractSiteRenderingMojo.java 1729058 2016-02-08 00:11:34Z hboutemy $
*/
public abstract class AbstractSiteRenderingMojo
extends AbstractSiteDescriptorMojo implements Contextualizable
{
/**
* Module type exclusion mappings
* ex: fml -> **/*-m1.fml
(excludes fml files ending in '-m1.fml' recursively)
*
* The configuration looks like this:
*
* <moduleExcludes>
* <moduleType>filename1.ext,**/*sample.ext</moduleType>
* <!-- moduleType can be one of 'apt', 'fml' or 'xdoc'. -->
* <!-- The value is a comma separated list of -->
* <!-- filenames or fileset patterns. -->
* <!-- Here's an example: -->
* <xdoc>changes.xml,navigation.xml</xdoc>
* </moduleExcludes>
*
*/
@Parameter
private Map moduleExcludes;
/**
* The location of a Velocity template file to use. When used, skins and the default templates, CSS and images
* are disabled. It is highly recommended that you package this as a skin instead.
*
* @since 2.0-beta-5
*/
@Parameter( property = "templateFile" )
private File templateFile;
/**
* Additional template properties for rendering the site. See
* Doxia Site Renderer.
*/
@Parameter
private Map attributes;
/**
* Site renderer.
*/
@Component
protected Renderer siteRenderer;
/**
* Reports (Maven 2).
*/
@Parameter( defaultValue = "${reports}", required = true, readonly = true )
protected List reports;
/**
* Alternative directory for xdoc source, useful for m1 to m2 migration
*
* @deprecated use the standard m2 directory layout
*/
@Parameter( defaultValue = "${basedir}/xdocs" )
private File xdocDirectory;
/**
* Directory containing generated documentation.
* This is used to pick up other source docs that might have been generated at build time.
*
* @todo should we deprecate in favour of reports?
*/
@Parameter( alias = "workingDirectory", defaultValue = "${project.build.directory}/generated-site" )
protected File generatedSiteDirectory;
/**
* The current Maven session.
*/
@Parameter( defaultValue = "${session}", readonly = true, required = true )
protected MavenSession mavenSession;
/**
* Configuration section used internally by Maven 3.
* More details available here:
*
* http://maven.apache.org/plugins/maven-site-plugin/maven-3.html#Configuration_formats
*
* Note: using this field is not mandatory with Maven 3 as Maven core injects usual
* <reporting>
section into this field.
*
* @since 3.0-beta-1 (and read-only since 3.3)
*/
@Parameter( readonly = true )
private ReportPlugin[] reportPlugins;
private PlexusContainer container;
/**
* Whether to generate the summary page for project reports: project-info.html.
*
* @since 2.3
*/
@Parameter( property = "generateProjectInfo", defaultValue = "true" )
private boolean generateProjectInfo;
/**
* Specifies the input encoding.
*
* @since 2.3
*/
@Parameter( property = "encoding", defaultValue = "${project.build.sourceEncoding}" )
private String inputEncoding;
/**
* Specifies the output encoding.
*
* @since 2.3
*/
@Parameter( property = "outputEncoding", defaultValue = "${project.reporting.outputEncoding}" )
private String outputEncoding;
/**
* Gets the input files encoding.
*
* @return The input files encoding, never null
.
*/
protected String getInputEncoding()
{
return ( StringUtils.isEmpty( inputEncoding ) ) ? ReaderFactory.FILE_ENCODING : inputEncoding;
}
/**
* Gets the effective reporting output files encoding.
*
* @return The effective reporting output file encoding, never null
.
*/
protected String getOutputEncoding()
{
return ( outputEncoding == null ) ? ReaderFactory.UTF_8 : outputEncoding;
}
/**
* Whether to save Velocity processed Doxia content (*..vm
)
* to ${generatedSiteDirectory}/processed
.
*
* @since 3.5
*/
@Parameter
private boolean saveProcessedContent;
/** {@inheritDoc} */
public void contextualize( Context context )
throws ContextException
{
container = (PlexusContainer) context.get( PlexusConstants.PLEXUS_KEY );
}
protected void checkInputEncoding()
{
if ( StringUtils.isEmpty( inputEncoding ) )
{
getLog().warn( "Input file encoding has not been set, using platform encoding "
+ ReaderFactory.FILE_ENCODING + ", i.e. build is platform dependent!" );
}
}
protected List getReports()
throws MojoExecutionException
{
List allReports;
if ( isMaven3OrMore() )
{
// Maven 3
MavenReportExecutorRequest mavenReportExecutorRequest = new MavenReportExecutorRequest();
mavenReportExecutorRequest.setLocalRepository( localRepository );
mavenReportExecutorRequest.setMavenSession( mavenSession );
mavenReportExecutorRequest.setProject( project );
mavenReportExecutorRequest.setReportPlugins( reportPlugins );
MavenReportExecutor mavenReportExecutor;
try
{
mavenReportExecutor = (MavenReportExecutor) container.lookup( MavenReportExecutor.class.getName() );
}
catch ( ComponentLookupException e )
{
throw new MojoExecutionException( "could not get MavenReportExecutor component", e );
}
allReports = mavenReportExecutor.buildMavenReports( mavenReportExecutorRequest );
}
else
{
// Maven 2
allReports = new ArrayList( reports.size() );
for ( MavenReport report : reports )
{
allReports.add( new MavenReportExecution( report ) );
}
}
// filter out reports that can't be generated
List reportExecutions = new ArrayList( allReports.size() );
for ( MavenReportExecution exec : allReports )
{
if ( exec.canGenerateReport() )
{
reportExecutions.add( exec );
}
}
return reportExecutions;
}
protected SiteRenderingContext createSiteRenderingContext( Locale locale )
throws MojoExecutionException, IOException, MojoFailureException
{
DecorationModel decorationModel = prepareDecorationModel( locale );
if ( attributes == null )
{
attributes = new HashMap();
}
if ( attributes.get( "project" ) == null )
{
attributes.put( "project", project );
}
if ( attributes.get( "inputEncoding" ) == null )
{
attributes.put( "inputEncoding", getInputEncoding() );
}
if ( attributes.get( "outputEncoding" ) == null )
{
attributes.put( "outputEncoding", getOutputEncoding() );
}
// Put any of the properties in directly into the Velocity context
for ( Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy